Konsole
#include <ViewContainer.h>
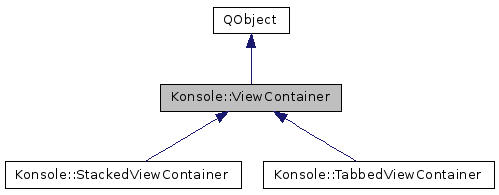
Public Types | |
enum | Feature { QuickNewView = 1, QuickCloseView = 2 } |
enum | MoveDirection { MoveViewLeft, MoveViewRight } |
enum | NavigationPosition { NavigationPositionTop, NavigationPositionBottom, NavigationPositionLeft, NavigationPositionRight } |
enum | NavigationVisibility { AlwaysShowNavigation, ShowNavigationAsNeeded, AlwaysHideNavigation } |
Signals | |
void | activeViewChanged (QWidget *view) |
void | destroyed (ViewContainer *container) |
void | empty (ViewContainer *container) |
void | moveViewRequest (int index, int id, bool &success, TabbedViewContainer *sourceContainer) |
void | newViewRequest () |
void | newViewRequest (Profile::Ptr) |
void | viewAdded (QWidget *view, ViewProperties *properties) |
void | viewRemoved (QWidget *view) |
Protected Member Functions | |
virtual void | addViewWidget (QWidget *view, int index)=0 |
virtual void | moveViewWidget (int fromIndex, int toIndex) |
virtual void | navigationPositionChanged (NavigationPosition) |
virtual void | navigationTextModeChanged (bool) |
virtual void | navigationVisibilityChanged (NavigationVisibility) |
virtual void | removeViewWidget (QWidget *view)=0 |
QList< QWidget * > | widgetsForItem (ViewProperties *item) const |
Detailed Description
An interface for container widgets which can hold one or more views.
The container widget typically displays a list of the views which it has and provides a means of switching between them.
Subclasses should reimplement the addViewWidget() and removeViewWidget() functions to actually add or remove view widgets from the container widget, as well as updating any navigation aids.
Definition at line 64 of file ViewContainer.h.
Member Enumeration Documentation
Enum describing extra UI features which can be provided by the container.
Enumerator | |
---|---|
QuickNewView |
Provides a button which can be clicked to create new views quickly. When the button is clicked, a newViewRequest() signal is emitted. |
QuickCloseView |
Provides a button which can be clicked to close views quickly. |
Definition at line 237 of file ViewContainer.h.
This enum describes the directions in which views can be re-arranged within the container using the moveActiveView() method.
Enumerator | |
---|---|
MoveViewLeft |
Moves the view to the left. |
MoveViewRight |
Moves the view to the right. |
Definition at line 219 of file ViewContainer.h.
This enum describes the options for positioning the container's navigation widget.
Definition at line 73 of file ViewContainer.h.
This enum describes the options for showing or hiding the container's navigation widget.
Definition at line 106 of file ViewContainer.h.
Constructor & Destructor Documentation
ViewContainer::ViewContainer | ( | NavigationPosition | position, |
QObject * | parent | ||
) |
Constructs a new view container with the specified parent.
- Parameters
-
position The initial position of the navigation widget parent The parent object of the container
Definition at line 54 of file ViewContainer.cpp.
|
virtual |
Called when the ViewContainer is destroyed.
When reimplementing this in subclasses, use object->deleteLater() to delete any widgets or other objects instead of 'delete object'.
Definition at line 62 of file ViewContainer.cpp.
Member Function Documentation
void ViewContainer::activateLastView | ( | ) |
Changes the active view to the last view.
Definition at line 226 of file ViewContainer.cpp.
void ViewContainer::activateNextView | ( | ) |
Changes the active view to the next view.
Definition at line 209 of file ViewContainer.cpp.
void ViewContainer::activatePreviousView | ( | ) |
Changes the active view to the previous view.
Definition at line 231 of file ViewContainer.cpp.
|
pure virtual |
Returns the view which currently has the focus or 0 if none of the child views have the focus.
Implemented in Konsole::StackedViewContainer, and Konsole::TabbedViewContainer.
|
signal |
Emitted when the active view changes.
void ViewContainer::addView | ( | QWidget * | view, |
ViewProperties * | navigationItem, | ||
int | index = -1 |
||
) |
Adds a new view to the container widget.
Definition at line 138 of file ViewContainer.cpp.
|
protectedpure virtual |
Performs the task of adding the view widget to the container widget.
Implemented in Konsole::StackedViewContainer, and Konsole::TabbedViewContainer.
|
pure virtual |
Returns the widget which contains the view widgets.
Implemented in Konsole::StackedViewContainer, and Konsole::TabbedViewContainer.
|
signal |
Emitted when the container is deleted.
|
signal |
Emitted when the container has no more children.
ViewContainer::Features ViewContainer::features | ( | ) | const |
Returns a bitwise-OR of enabled extra UI features.
See setFeatures()
Definition at line 79 of file ViewContainer.cpp.
void ViewContainer::moveActiveView | ( | MoveDirection | direction | ) |
Moves the active view within the container and updates the order in which the views are shown in the container's navigation widget.
The default implementation does nothing.
Definition at line 83 of file ViewContainer.cpp.
|
signal |
Emitted when the user requests to move a view from another container into this container.
If 'success' is set to true by a connected slot then the original view will be removed.
- Parameters
-
index Index at which to insert the new view in the container or -1 to append it. This index should be passed to addView() when the new view has been created. id The identifier of the view. success The slot handling this signal should set this to true if the new view was successfully created. sourceContainer Initial move event Tabbed view container.
|
protectedvirtual |
Rearranges the order of widgets in the container.
- Parameters
-
fromIndex Current index of the widget to move toIndex New index for the widget
Reimplemented in Konsole::TabbedViewContainer.
Definition at line 74 of file ViewContainer.cpp.
ViewContainer::NavigationPosition ViewContainer::navigationPosition | ( | ) | const |
Returns the position of the navigation widget with respect to the main content area.
Definition at line 111 of file ViewContainer.cpp.
|
inlineprotectedvirtual |
Called when the navigation position changes to re-layout the container and place the navigation widget in the specified position.
Reimplemented in Konsole::TabbedViewContainer.
Definition at line 325 of file ViewContainer.h.
|
inlineprotectedvirtual |
Reimplemented in Konsole::TabbedViewContainer.
Definition at line 327 of file ViewContainer.h.
ViewContainer::NavigationVisibility ViewContainer::navigationVisibility | ( | ) | const |
Returns the current mode for controlling the visibility of the the view container's navigation widget.
Definition at line 128 of file ViewContainer.cpp.
|
inlineprotectedvirtual |
Called when the navigation display mode changes.
See setNavigationVisibility
Reimplemented in Konsole::TabbedViewContainer.
Definition at line 318 of file ViewContainer.h.
|
signal |
Emitted when the user requests to open a new view.
|
signal |
Requests creation of a new view, with the selected profile.
void ViewContainer::removeView | ( | QWidget * | view | ) |
Removes a view from the container.
Definition at line 174 of file ViewContainer.cpp.
|
protectedpure virtual |
Performs the task of removing the view widget from the container widget.
Implemented in Konsole::StackedViewContainer, and Konsole::TabbedViewContainer.
IncrementalSearchBar * ViewContainer::searchBar | ( | ) |
- Returns
- the search widget for this view
Definition at line 194 of file ViewContainer.cpp.
|
pure virtual |
Changes the focus to the specified view and updates navigation aids to reflect the change.
Implemented in Konsole::StackedViewContainer, and Konsole::TabbedViewContainer.
|
virtual |
Sets which additional features are enabled in this container.
The default implementation does thing. Sub-classes should re-implement this to hide or show the relevant parts of their UI
Reimplemented in Konsole::TabbedViewContainer.
Definition at line 75 of file ViewContainer.cpp.
void ViewContainer::setNavigationPosition | ( | NavigationPosition | position | ) |
Sets the position of the navigation widget with respect to the main content area.
Depending on the ViewContainer subclass, not all positions from the NavigationPosition enum may be supported. A list of supported positions can be obtained by calling supportedNavigationPositions()
ViewContainer sub-classes should re-implement the navigationPositionChanged() method to respond to changes of this property.
Definition at line 115 of file ViewContainer.cpp.
void ViewContainer::setNavigationTextMode | ( | bool | mode | ) |
Sets the navigation text mode If mode is true, use the width of the title; otherwise use the default width calculations.
Definition at line 133 of file ViewContainer.cpp.
void ViewContainer::setNavigationVisibility | ( | NavigationVisibility | mode | ) |
Definition at line 106 of file ViewContainer.cpp.
|
inlinevirtual |
Sets the menu to be shown when the new view button is clicked.
Only valid if the QuickNewView feature is enabled. The default implementation does nothing.
Reimplemented in Konsole::TabbedViewContainer.
Definition at line 261 of file ViewContainer.h.
|
inlinevirtual |
Sets the stylesheet for visual appearance.
The default implementation does nothing.
Reimplemented in Konsole::TabbedViewContainer.
Definition at line 172 of file ViewContainer.h.
|
inlinevirtual |
Returns a bitwise-OR of supported extra UI features.
The default implementation returns 0 (no extra features)
Reimplemented in Konsole::TabbedViewContainer.
Definition at line 255 of file ViewContainer.h.
|
virtual |
Returns the list of supported navigation positions.
The supported positions will depend upon the type of the navigation widget used by the ViewContainer subclass.
The base implementation returns one item, NavigationPositionTop
Reimplemented in Konsole::TabbedViewContainer.
Definition at line 124 of file ViewContainer.cpp.
|
signal |
Emitted when a view is added to the container.
ViewProperties * ViewContainer::viewProperties | ( | QWidget * | view | ) | const |
Returns the ViewProperties instance associated with a particular view in the container.
Definition at line 248 of file ViewContainer.cpp.
|
signal |
Emitted when a view is removed from the container.
Returns a list of the contained views.
Definition at line 189 of file ViewContainer.cpp.
|
protected |
Returns the widgets which are associated with a particular navigation item.
Definition at line 255 of file ViewContainer.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:31:25 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.