KSpeech
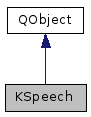
Detailed Description
Constants and Enums for the KSpeech D-Bus interface.
#include "kspeech.h"
Note: Applications do not use this class directly. Instead, use the OrgKdeKSpeechInterface object as described in Calling KTTSD from a Program.
See also Introduction to the KSpeech D-Bus Interface
Definition at line 651 of file Mainpage.dox.
Member Enumeration Documentation
enum KSpeech::JobPriority |
The priority of jobs submitted by OrgKdeKSpeechInterface::say.
Enumerator | |
---|---|
jpAll |
All priorities. Used for information retrieval only. |
jpScreenReaderOutput |
Screen Reader job. |
jpWarning |
Warning job. |
jpMessage |
Message job. |
jpText |
Text job. |
Definition at line 660 of file Mainpage.dox.
enum KSpeech::JobState |
Job states returned by method OrgKdeKSpeechInterface::getJobState.
Definition at line 673 of file Mainpage.dox.
enum KSpeech::MarkerType |
Types of markers emitted by OrgKdeKSpeechInterface::marker signal.
Enumerator | |
---|---|
mtSentenceBegin | |
mtSentenceEnd | |
mtWordBegin | |
mtPhonemeBegin | |
mtCustom |
Definition at line 763 of file Mainpage.dox.
enum KSpeech::SayOptions |
Hints about text content when sending via OrgKdeKSpeechInterface::say.
Definition at line 689 of file Mainpage.dox.
Flags for synthesizer/talker capabilities returned by OrgKdeKSpeechInterface::getTalkerCapabilities1.
All items marked FALSE are hard-coded off at this time.
Definition at line 707 of file Mainpage.dox.
All items marked FALSE are hard-coded off at this time.
Definition at line 745 of file Mainpage.dox.
The documentation for this class was generated from the following file:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:52:17 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.