KSpeech
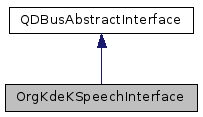
Public Slots | |
QString | applicationName () |
bool | autoConfigureTalkersOn () |
void | changeJobTalker (int jobNum, const QString &talker) |
int | defaultPriority () |
QString | defaultTalker () |
bool | filteringOn () |
int | getCurrentJob () |
int | getJobCount (int priority) |
QByteArray | getJobInfo (int jobNum) |
QStringList | getJobNumbers (int priority) |
QString | getJobSentence (int jobNum, int sentenceNum) |
int | getJobState (int jobNum) |
int | getSentenceCount (int jobNum) |
int | getTalkerCapabilities1 (const QString &talker) |
int | getTalkerCapabilities2 (const QString &talker) |
QStringList | getTalkerCodes () |
QStringList | getTalkerVoices (const QString &talker) |
QString | htmlFilterXsltFile () |
bool | isApplicationPaused () |
bool | isSpeaking () const |
bool | isSystemManager () |
void | kttsdExit () |
void | moveJobLater (int jobNum) |
int | moveRelSentence (int jobNum, int n) |
void | pause () |
void | reinit () |
void | removeAllJobs () |
void | removeJob (int jobNum) |
void | resume () |
int | say (const QString &text, int options) |
int | sayClipboard () |
int | sayFile (const QString &filename, const QString &encoding) |
QString | sentenceDelimiter () |
void | setApplicationName (const QString &applicationName) |
void | setAutoConfigureTalkersOn (bool autoConfigureTalkersOn) |
void | setCallingAppId (const QString &appId) |
void | setDefaultPriority (int defaultPriority) |
void | setDefaultTalker (const QString &defaultTalker) |
void | setFilteringOn (bool filteringOn) |
void | setHtmlFilterXsltFile (const QString &htmlFilterXsltFile) |
void | setIsSystemManager (bool isSystemManager) |
void | setSentenceDelimiter (const QString &sentenceDelimiter) |
void | setSsmlFilterXsltFile (const QString &ssmlFilterXsltFile) |
void | showManagerDialog () |
QString | ssmlFilterXsltFile () |
QString | talkerToTalkerId (const QString &talker) |
QString | version () const |
Signals | |
void | jobStateChanged (const QString &appId, int jobNum, int state) |
void | kttsdExiting () |
void | kttsdStarted () |
void | marker (const QString &appId, int jobNum, int markerType, const QString &markerData) |
Properties | |
bool | isSpeaking |
QString | version |
Detailed Description
D-Bus Interface to KSpeech.
#include "kspeechinterface.h"
The D-Bus interface to KSpeech. Applications may speak text using this class, or by sending D-Bus messages to application "org.kde.kttsd", object path "/KSpeech", interface "org.kde.KSpeech".
You must generate the kspeechinterface.h file using the qdbusxml2cpp utility. See Using OrgKdeKSpeechInterface for more information.
Definition at line 787 of file Mainpage.dox.
Member Function Documentation
|
slot |
Returns the friendly display name for the application.
- Returns
- Application display name.
If application has not provided a friendly name, the D-Bus connection name is returned.
- See also
- setApplicationName
|
slot |
Returns whether KTTSD will automatically attempt to configure new talkers to meet required talker attributes.
- Returns
- True if KTTSD will autoconfigure talkers.
|
slot |
Change the talker of an already-submitted job.
- Parameters
-
jobNum Job Number. If 0, the last job submitted by the application. talker Desired new talker.
|
slot |
Returns the default priority for speech jobs submitted by the application.
- Returns
- Default job priority. See KSpeech::JobPriority.
|
slot |
Returns the default talker for the application.
- Returns
- Talker.
The default is "", which uses the default talker configured by user.
|
slot |
Returns whether speech jobs for this application are filtered using configured filter plugins.
- Returns
- True if filtering is on.
Filtering is on by default.
- See also
- setFilteringOn
- Filters
|
slot |
Returns the job number of the currently speaking job (any application).
- Returns
- Job Number
|
slot |
Returns the number of jobs belonging to the application with the specified job priority.
- Parameters
-
priority Job Priority. See KSpeech::JobPriority.
- Returns
- Number of jobs.
If priority is KSpeech::jpAll, returns the number of jobs belonging to the application (all priorities).
When called from a System Manager, returns count of all jobs of the specified priority for all applications.
- See also
- Speech Jobs and Priorities
|
slot |
Get information about a job.
- Parameters
-
jobNum Job Number. If 0, the last job submitted by the application.
- Returns
- A QDataStream containing information about the job. Blank if no such job.
The stream contains the following elements:
- int priority Job Type.
- int state Job state.
- QString appId D-Bus senderId of the application that requested the speech job.
- QString talker Talker code as requested by application.
- int sentenceNum Current sentence being spoken. Sentences are numbered starting at 1.
- int sentenceCount Total number of sentences in the job.
- QString applicationName Application's friendly name (if provided by app)
If the job is currently filtering, waits for that to finish before returning.
The following sample code will decode the stream:
QByteArray jobInfo = m_kspeech->getJobInfo(jobNum); if (jobInfo != QByteArray()) { QDataStream stream(&jobInfo, QIODevice::ReadOnly); qint32 priority; qint32 state; QString talker; qint32 sentenceNum; qint32 sentenceCount; QString applicationName; stream >> priority; stream >> state; stream >> appId; stream >> talker; stream >> sentenceNum; stream >> sentenceCount; stream >> applicationName; };
|
slot |
Returns a list job numbers for the jobs belonging to the application with the specified priority.
- Parameters
-
priority Job Priority. See KSpeech::JobPriority.
- Returns
- List of job numbers. Note that the numbers are strings.
If priority is KSpeech::jpAll, returns the job numbers belonging to the application (all priorities).
When called from a System Manager, returns job numbers of the specified priority for all applications.
- See also
- Speech Jobs and Priorities
|
slot |
Return a sentence of a job.
- Parameters
-
jobNum Job Number. If 0, the last job submitted by the application. sentenceNum Sentence Number. Sentence numbers start at 1.
- Returns
- The specified sentence in the specified job. If no such job or sentence, returns "".
|
slot |
Returns the state of a job.
- Parameters
-
jobNum Job Number. If 0, the last job submitted by the application.
- Returns
- Job state. See KSpeech::JobState.
|
slot |
Returns the number of sentences in a job.
- Parameters
-
jobNum Job Number. If 0, the last job submitted by the application.
- Returns
- Number of sentences in the job.
|
slot |
Returns a bitarray giving the capabilities of a talker.
- Parameters
-
talker Talker. Example: "en".
- Returns
- A word with bits set according to the capabilities of the talker.
- See also
- getTalkerCapabilities2
|
slot |
Returns a bitarray giving the capabilities of a talker.
- Parameters
-
talker Talker. Example: "en".
- Returns
- A word with bits set according to the capabilities of the talker.
- See also
- getTalkerCapabilities1
|
slot |
Return a list of full Talker Codes for configured talkers.
- Returns
- List of Talker codes.
|
slot |
Return a list of the voice codes of voices available in the synthesizer corresponding to a talker.
- Parameters
-
talker Talker. Example: "synthesizer='Festival'"
- Returns
- List of voice codes.
Voice codes are synthesizer specific.
|
slot |
Returns the full path name to XSLT file used to convert HTML markup to speakable form.
- Returns
- XSLT filename.
- See also
- setHtmlFilterXsltFile
- Filters
|
slot |
|
slot |
Returns true if KTTSD is currently speaking.
- Returns
- True if currently speaking.
|
slot |
Returns whether this is a System Manager application.
- Returns
- True if the application is a System Manager.
- See also
- setIsSystemManager
|
signal |
This signal is emitted each time the state of a job changes.
- Parameters
-
appId The D-Bus connection name of the application that submitted the job. jobNum Job Number. state Job state. KSpeech::JobState.
|
slot |
Shuts down KTTSD.
Do not call this!
|
signal |
This signal is emitted just before KTTS exits.
|
signal |
This signal is emitted when KTTSD starts.
|
signal |
This signal is emitted when a marker is processed.
Currently only emits mtSentenceBegin and mtSentenceEnd.
- Parameters
-
appId The D-Bus connection name of the application that submitted the job. jobNum Job Number of the job emitting the marker. markerType The type of marker. See KSpeech::MarkerType. Currently either mtSentenceBegin or mtSentenceEnd. markerData Data for the marker. Currently, this is the sentence number of the sentence begun or ended. Sentence numbers begin at 1.
|
slot |
Move a job one position down in the queue so that it is spoken later.
If the job is already speaking, it is stopped and will resume when processing next gets to it.
- Parameters
-
jobNum Job Number. If 0, the last job submitted by the application.
Since there is only one ScreenReaderOutput, this method is meaningless for ScreenReaderOutput jobs.
|
slot |
Advance or rewind N sentences in a job.
- Parameters
-
jobNum Job Number. If 0, the last job submitted by the application. n Number of sentences to advance (positive) or rewind (negative) in the job.
- Returns
- Number of the sentence actually moved to. Sentence numbers are numbered starting at 1.
If no such job, does nothing and returns 0. If n is zero, returns the current sentence number of the job. Does not affect the current speaking/not-speaking state of the job.
Since ScreenReaderOutput jobs are not split into sentences, this method is meaningless for ScreenReaderOutput jobs.
|
slot |
Pauses speech jobs belonging to the application.
When called by a System Manager, pauses all jobs of all applications.
- See also
- isApplicationPaused
|
slot |
Cause KTTSD to re-read its configuration.
|
slot |
Removes all jobs belonging to the application.
When called from a System Manager, removes all jobs of all applications.
- See also
- removeJob
|
slot |
Removes the specified job.
If the job is speaking, it is stopped.
- Parameters
-
jobNum Job Number. If 0, the last job submitted by the application.
- See also
- removeAllJobs
|
slot |
Resumes speech jobs belonging to the application.
When called by a System Manager, resumes all jobs of all applications.
|
slot |
Creates and starts a speech job.
The job is created at the application's default job priority using the default talker.
- Parameters
-
text The text to be spoken. options Speech options. See KSpeech::SayOptions.
- Returns
- Job Number for the new job.
- See also
- Speech Jobs and Priorities
|
slot |
Submits a speech job from the contents of the clipboard.
The job is spoken using application's default talker.
- Returns
- Job Number for the new job.
|
slot |
Creates and starts a speech job from a specified file.
- Parameters
-
filename Full path name of the file. encoding The encoding of the file. Default UTF-8.
- Returns
- Job Number for the new job.
The job is spoken using application's default talker.
- See also
- defaultTalker
Plain text is parsed into individual sentences using the current sentence delimiter. Call setSentenceDelimiter to change the sentence delimiter prior to calling sayFile. Call getSentenceCount to retrieve the sentence count after calling sayFile.
The text may contain speech mark language, such as SMML, provided that the speech plugin/engine support it. In this case, sentence parsing follows the semantics of the markup language.
- See also
- Speech Jobs and Priorities
|
slot |
Returns the regular expression used to perform Sentence Boundary Detection (SBD) for the application.
- Returns
- Sentence delimiter regular expression.
The default sentence delimiter is
([\\.\\?\\!\\:\\;])(\\s|$|(\\n *\\n))
Note that backward slashes must be escaped.
- See also
- setSentenceDelimiter
- Sentence Parsing
|
slot |
Sets a friendly display name for the application.
- Parameters
-
applicationName Friendly name for the application.
- See also
- applicationName
|
slot |
Sets whether KTTSD will automatically attempt to configure new talkers to meet required talker attributes.
- Parameters
-
autoConfigureTalkersOn True to enable auto configuration.
|
slot |
Called by DBusAdaptor so that KTTSD knows the application that called it.
- Parameters
-
appId D-Bus connection name that called KSpeech.
|
slot |
Sets the default priority for speech jobs submitted by the application.
- Parameters
-
defaultPriority Default job priority. See KSpeech::JobPriority.
|
slot |
Sets the default talker for the application.
- Parameters
-
defaultTalker Default talker. Example: "en".
|
slot |
Sets whether speech jobs for this application are filtered using configured filter plugins.
- Parameters
-
filteringOn True to set filtering on.
- See also
- filteringOn
- Filters
|
slot |
Sets the full path name to an XSLT file used to convert HTML markup to speakable form.
- Parameters
-
htmlFilterXsltFile XSLT filename.
- See also
- htmlFilterXsltFile
- Filters
|
slot |
Sets whether this is a System Manager application.
- Parameters
-
isSystemManager True if this is a System Manager.
System Managers are used to control and configure overall TTS output. When True, many of the KSpeech methods alter their behavior.
- See also
- isSystemManager
|
slot |
Sets the regular expression used to perform Sentence Boundary Detection (SBD) for the application.
- Parameters
-
sentenceDelimiter Sentence delimiter regular expression.
- See also
- sentenceDelimiter
- Sentence Parsing
|
slot |
Sets the full path name to XSLT file used to convert SSML markup to a speakable form.
- Parameters
-
ssmlFilterXsltFile XSLT filename.
- See also
- ssmlFilterXsltFile
- Filters
|
slot |
Display the KttsMgr program so that user can configure KTTS options.
Only one instance of KttsMgr is displayed.
|
slot |
Returns the full path name to XSLT file used to convert SSML markup to a speakable form.
- Returns
- XSLT filename.
- See also
- setSsmlFilterXsltFile
- Filters
|
slot |
Given a talker, returns the Talker ID for the talker.
that will speak the job.
- Parameters
-
talker Talker. Example: "en".
- Returns
- Talker ID. A Talker ID is an internally-assigned identifier for a talker.
This method is normally used only by System Managers.
|
slot |
Returns the version number of KTTSD.
- Returns
- Version number string.
Property Documentation
|
read |
Definition at line 791 of file Mainpage.dox.
|
read |
Definition at line 792 of file Mainpage.dox.
The documentation for this class was generated from the following file:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:52:17 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.