KDECore
#include <KConfigBase>
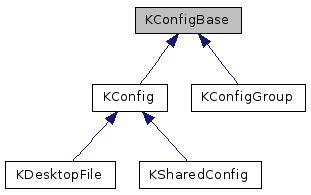
Public Types | |
enum | AccessMode { NoAccess, ReadOnly, ReadWrite } |
enum | WriteConfigFlag { Persistent = 0x01, Global = 0x02, Localized = 0x04, Normal =Persistent } |
Public Member Functions | |
virtual | ~KConfigBase () |
virtual AccessMode | accessMode () const =0 |
void | deleteGroup (const QByteArray &group, WriteConfigFlags flags=Normal) |
void | deleteGroup (const QString &group, WriteConfigFlags flags=Normal) |
void | deleteGroup (const char *group, WriteConfigFlags flags=Normal) |
KConfigGroup | group (const QByteArray &group) |
KConfigGroup | group (const QString &group) |
KConfigGroup | group (const char *group) |
const KConfigGroup | group (const QByteArray &group) const |
const KConfigGroup | group (const QString &group) const |
const KConfigGroup | group (const char *group) const |
virtual QStringList | groupList () const =0 |
bool | hasGroup (const QString &group) const |
bool | hasGroup (const char *group) const |
bool | hasGroup (const QByteArray &group) const |
bool | isGroupImmutable (const QByteArray &aGroup) const |
bool | isGroupImmutable (const QString &aGroup) const |
bool | isGroupImmutable (const char *aGroup) const |
virtual bool | isImmutable () const =0 |
virtual void | markAsClean ()=0 |
virtual void | sync ()=0 |
Protected Member Functions | |
KConfigBase () | |
virtual void | deleteGroupImpl (const QByteArray &group, WriteConfigFlags flags=Normal)=0 |
virtual KConfigGroup | groupImpl (const QByteArray &b)=0 |
virtual const KConfigGroup | groupImpl (const QByteArray &b) const =0 |
virtual bool | hasGroupImpl (const QByteArray &group) const =0 |
virtual bool | isGroupImmutableImpl (const QByteArray &aGroup) const =0 |
virtual void | virtual_hook (int id, void *data) |
Detailed Description
Definition at line 38 of file kconfigbase.h.
Member Enumeration Documentation
Possible return values for accessMode().
Enumerator | |
---|---|
NoAccess | |
ReadOnly | |
ReadWrite |
Definition at line 133 of file kconfigbase.h.
Flags to control write entry.
Definition at line 44 of file kconfigbase.h.
Constructor & Destructor Documentation
|
virtual |
Destructs the KConfigBase object.
Definition at line 105 of file kconfigbase.cpp.
|
protected |
Definition at line 108 of file kconfigbase.cpp.
Member Function Documentation
|
pure virtual |
Returns the access mode of the app-config object.
Possible return values are NoAccess (the application-specific config file could not be opened neither read-write nor read-only), ReadOnly (the application-specific config file is opened read-only, but not read-write) and ReadWrite (the application-specific config file is opened read-write).
- Returns
- the access mode of the app-config object
Implemented in KConfig, and KConfigGroup.
void KConfigBase::deleteGroup | ( | const QByteArray & | group, |
WriteConfigFlags | flags = Normal |
||
) |
Delete aGroup
.
This marks aGroup
as deleted in the config object. This effectively removes any cascaded values from config files earlier in the stack.
Definition at line 74 of file kconfigbase.cpp.
Definition at line 79 of file kconfigbase.cpp.
void KConfigBase::deleteGroup | ( | const char * | group, |
WriteConfigFlags | flags = Normal |
||
) |
Definition at line 84 of file kconfigbase.cpp.
|
protectedpure virtual |
Implemented in KConfigGroup, and KConfig.
KConfigGroup KConfigBase::group | ( | const QByteArray & | group | ) |
Returns an object for the named subgroup.
- Parameters
-
group the group to open. Pass a null string on to the KConfig object to obtain a handle on the root group.
- Returns
- The list of groups.
Definition at line 44 of file kconfigbase.cpp.
KConfigGroup KConfigBase::group | ( | const QString & | group | ) |
Definition at line 49 of file kconfigbase.cpp.
KConfigGroup KConfigBase::group | ( | const char * | group | ) |
Definition at line 54 of file kconfigbase.cpp.
const KConfigGroup KConfigBase::group | ( | const QByteArray & | group | ) | const |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
Definition at line 59 of file kconfigbase.cpp.
const KConfigGroup KConfigBase::group | ( | const QString & | group | ) | const |
Definition at line 64 of file kconfigbase.cpp.
const KConfigGroup KConfigBase::group | ( | const char * | group | ) | const |
Definition at line 69 of file kconfigbase.cpp.
|
protectedpure virtual |
Implemented in KConfigGroup, and KConfig.
|
protectedpure virtual |
Implemented in KConfigGroup, and KConfig.
|
pure virtual |
Returns a list of groups that are known about.
- Returns
- The list of groups.
Implemented in KConfig, and KConfigGroup.
Returns true if the specified group is known about.
- Parameters
-
group The group to search for.
- Returns
- true if the group exists.
Definition at line 29 of file kconfigbase.cpp.
bool KConfigBase::hasGroup | ( | const char * | group | ) | const |
Definition at line 34 of file kconfigbase.cpp.
bool KConfigBase::hasGroup | ( | const QByteArray & | group | ) | const |
Definition at line 39 of file kconfigbase.cpp.
|
protectedpure virtual |
Implemented in KConfigGroup, and KConfig.
bool KConfigBase::isGroupImmutable | ( | const QByteArray & | aGroup | ) | const |
Can changes be made to the entries in aGroup
?
- Parameters
-
aGroup The group to check for immutability.
- Returns
false
if the entries inaGroup
can be modified.
Definition at line 89 of file kconfigbase.cpp.
Definition at line 94 of file kconfigbase.cpp.
bool KConfigBase::isGroupImmutable | ( | const char * | aGroup | ) | const |
Definition at line 100 of file kconfigbase.cpp.
|
protectedpure virtual |
Implemented in KConfigGroup, and KConfig.
|
pure virtual |
Checks whether this configuration object can be modified.
- Returns
- whether changes may be made to this configuration object.
Implemented in KConfigGroup, and KConfig.
|
pure virtual |
Reset the dirty flags of all entries in the entry map, so the values will not be written to disk on a later call to sync().
Implemented in KConfig, and KConfigGroup.
|
pure virtual |
Syncs the configuration object that this group belongs to.
Unrelated concurrent changes to the same file are merged and thus not overwritten. Note however, that this object is not automatically updated with those changes.
Implemented in KConfig, and KConfigGroup.
|
protectedvirtual |
Virtual hook, used to add new "virtual" functions while maintaining binary compatibility.
Unused in this class.
Reimplemented in KConfig.
Definition at line 111 of file kconfigbase.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:47:10 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.