KDECore
#include <kdirwatch_p.h>
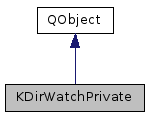
Classes | |
struct | Client |
class | Entry |
Public Types | |
enum | { NoChange =0, Changed =1, Created =2, Deleted =4 } |
typedef QMap< QString, Entry > | EntryMap |
enum | entryMode { UnknownMode = 0, StatMode, DNotifyMode, INotifyMode, FAMMode, QFSWatchMode } |
enum | entryStatus { Normal = 0, NonExistent } |
Public Slots | |
void | famEventReceived () |
void | fswEventReceived (const QString &path) |
void | inotifyEventReceived () |
void | slotRemoveDelayed () |
void | slotRescan () |
Public Member Functions | |
KDirWatchPrivate () | |
~KDirWatchPrivate () | |
void | addEntry (KDirWatch *instance, const QString &_path, Entry *sub_entry, bool isDir, KDirWatch::WatchModes watchModes=KDirWatch::WatchDirOnly) |
void | addWatch (Entry *entry) |
bool | deref () |
void | emitEvent (const Entry *e, int event, const QString &fileName=QString()) |
Entry * | entry (const QString &) |
void | ref () |
void | removeEntries (KDirWatch *) |
bool | removeEntry (KDirWatch *, const QString &, Entry *sub_entry) |
void | removeEntry (KDirWatch *, Entry *e, Entry *sub_entry) |
void | removeWatch (Entry *entry) |
void | resetList (KDirWatch *, bool) |
bool | restartEntryScan (KDirWatch *, Entry *, bool) |
int | scanEntry (Entry *e) |
void | startScan (KDirWatch *, bool, bool) |
void | statistics () |
bool | stopEntryScan (KDirWatch *, Entry *) |
void | stopScan (KDirWatch *) |
void | useFreq (Entry *e, int newFreq) |
bool | useQFSWatch (Entry *e) |
bool | useStat (Entry *) |
Static Public Member Functions | |
static bool | isNoisyFile (const char *filename) |
Public Attributes | |
bool | _isStopped |
bool | delayRemove |
int | freq |
KFileSystemWatcher * | fsWatcher |
EntryMap | m_mapEntries |
int | m_nfsPollInterval |
KDirWatch::Method | m_nfsPreferredMethod |
int | m_PollInterval |
KDirWatch::Method | m_preferredMethod |
int | m_ref |
QSet< Entry * > | removeList |
bool | rescan_all |
QTimer | rescan_timer |
int | statEntries |
QTimer | timer |
Detailed Description
Definition at line 116 of file kdirwatch_p.h.
Member Typedef Documentation
typedef QMap<QString,Entry> KDirWatchPrivate::EntryMap |
Definition at line 191 of file kdirwatch_p.h.
Member Enumeration Documentation
anonymous enum |
Enumerator | |
---|---|
NoChange | |
Changed | |
Created | |
Deleted |
Definition at line 123 of file kdirwatch_p.h.
Enumerator | |
---|---|
UnknownMode | |
StatMode | |
DNotifyMode | |
INotifyMode | |
FAMMode | |
QFSWatchMode |
Definition at line 122 of file kdirwatch_p.h.
Enumerator | |
---|---|
Normal | |
NonExistent |
Definition at line 121 of file kdirwatch_p.h.
Constructor & Destructor Documentation
KDirWatchPrivate::KDirWatchPrivate | ( | ) |
Definition at line 153 of file kdirwatch.cpp.
KDirWatchPrivate::~KDirWatchPrivate | ( | ) |
Definition at line 250 of file kdirwatch.cpp.
Member Function Documentation
void KDirWatchPrivate::addEntry | ( | KDirWatch * | instance, |
const QString & | _path, | ||
Entry * | sub_entry, | ||
bool | isDir, | ||
KDirWatch::WatchModes | watchModes = KDirWatch::WatchDirOnly |
||
) |
Definition at line 763 of file kdirwatch.cpp.
void KDirWatchPrivate::addWatch | ( | Entry * | entry | ) |
Definition at line 919 of file kdirwatch.cpp.
|
inline |
Definition at line 218 of file kdirwatch_p.h.
void KDirWatchPrivate::emitEvent | ( | const Entry * | e, |
int | event, | ||
const QString & | fileName = QString() |
||
) |
Definition at line 1314 of file kdirwatch.cpp.
KDirWatchPrivate::Entry * KDirWatchPrivate::entry | ( | const QString & | _path | ) |
Definition at line 586 of file kdirwatch.cpp.
|
slot |
Definition at line 1658 of file kdirwatch.cpp.
|
slot |
Definition at line 1706 of file kdirwatch.cpp.
|
slot |
Definition at line 271 of file kdirwatch.cpp.
|
static |
Definition at line 1501 of file kdirwatch.cpp.
|
inline |
Definition at line 217 of file kdirwatch_p.h.
void KDirWatchPrivate::removeEntries | ( | KDirWatch * | instance | ) |
Definition at line 1060 of file kdirwatch.cpp.
bool KDirWatchPrivate::removeEntry | ( | KDirWatch * | instance, |
const QString & | _path, | ||
Entry * | sub_entry | ||
) |
Definition at line 994 of file kdirwatch.cpp.
Definition at line 1010 of file kdirwatch.cpp.
void KDirWatchPrivate::removeWatch | ( | Entry * | entry | ) |
Definition at line 967 of file kdirwatch.cpp.
Definition at line 1205 of file kdirwatch.cpp.
Definition at line 1121 of file kdirwatch.cpp.
int KDirWatchPrivate::scanEntry | ( | Entry * | e | ) |
Definition at line 1219 of file kdirwatch.cpp.
|
slot |
Definition at line 1372 of file kdirwatch.cpp.
|
slot |
Definition at line 1387 of file kdirwatch.cpp.
Definition at line 1190 of file kdirwatch.cpp.
void KDirWatchPrivate::statistics | ( | ) |
Definition at line 1665 of file kdirwatch.cpp.
Definition at line 1095 of file kdirwatch.cpp.
void KDirWatchPrivate::stopScan | ( | KDirWatch * | instance | ) |
Definition at line 1182 of file kdirwatch.cpp.
void KDirWatchPrivate::useFreq | ( | Entry * | e, |
int | newFreq | ||
) |
Definition at line 606 of file kdirwatch.cpp.
Definition at line 713 of file kdirwatch.cpp.
Definition at line 734 of file kdirwatch.cpp.
Member Data Documentation
bool KDirWatchPrivate::_isStopped |
Definition at line 268 of file kdirwatch_p.h.
bool KDirWatchPrivate::delayRemove |
Definition at line 242 of file kdirwatch_p.h.
int KDirWatchPrivate::freq |
Definition at line 234 of file kdirwatch_p.h.
KFileSystemWatcher* KDirWatchPrivate::fsWatcher |
Definition at line 264 of file kdirwatch_p.h.
EntryMap KDirWatchPrivate::m_mapEntries |
Definition at line 231 of file kdirwatch_p.h.
int KDirWatchPrivate::m_nfsPollInterval |
Definition at line 236 of file kdirwatch_p.h.
KDirWatch::Method KDirWatchPrivate::m_nfsPreferredMethod |
Definition at line 233 of file kdirwatch_p.h.
int KDirWatchPrivate::m_PollInterval |
Definition at line 236 of file kdirwatch_p.h.
KDirWatch::Method KDirWatchPrivate::m_preferredMethod |
Definition at line 233 of file kdirwatch_p.h.
int KDirWatchPrivate::m_ref |
Definition at line 237 of file kdirwatch_p.h.
Definition at line 241 of file kdirwatch_p.h.
bool KDirWatchPrivate::rescan_all |
Definition at line 244 of file kdirwatch_p.h.
QTimer KDirWatchPrivate::rescan_timer |
Definition at line 245 of file kdirwatch_p.h.
int KDirWatchPrivate::statEntries |
Definition at line 235 of file kdirwatch_p.h.
QTimer KDirWatchPrivate::timer |
Definition at line 230 of file kdirwatch_p.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:47:10 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.