KDECore
#include <klocalsocket.h>
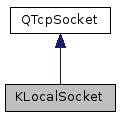
Public Types | |
enum | LocalSocketType { UnixSocket, AbstractUnixSocket, UnknownLocalSocketType = -1 } |
Public Member Functions | |
KLocalSocket (QObject *parent=0) | |
virtual | ~KLocalSocket () |
void | connectToPath (const QString &path, OpenMode mode=ReadWrite) |
void | connectToPath (const QString &path, LocalSocketType type, OpenMode mode=ReadWrite) |
void | disconnectFromPath () |
QString | localPath () const |
LocalSocketType | localSocketType () const |
QString | peerPath () const |
Protected Slots | |
void | connectToHostImplementation (const QString &hostName, quint16 port, OpenMode mode) |
void | disconnectFromHostImplementation () |
Detailed Description
KLocalSocket allows one to create and use local (Unix) sockets.
On some platforms, local sockets are a kind of streaming socket that can be used to transmit and receive data just like Internet (TCP) streaming sockets. The difference is that they remain local to the host running them and cannot be accessed externally. They are also very fast and (in theory) consume less resources than standard TCP sockets.
KLocalSocket supports two kinds of local socket types (see KLocalSocket::LocalSocketType):
- Unix sockets (UnixSocket): standard Unix sockets whose names are file paths and obey filesystem restrictions
- Abstract Unix sockets (AbstractUnixSocket): similar to Unix sockets, but they don't exist as entries in the filesystem and, thus, aren't restricted by its permissions
Definition at line 51 of file klocalsocket.h.
Member Enumeration Documentation
Defines the local socket type.
See KLocalSocket for more information
Enumerator | |
---|---|
UnixSocket |
Unix sockets. |
AbstractUnixSocket |
Abstract Unix sockets. |
UnknownLocalSocketType |
Definition at line 59 of file klocalsocket.h.
Constructor & Destructor Documentation
|
explicit |
Creates a KLocalSocket object with parent
as the parent object.
- Parameters
-
parent the parent object
Definition at line 44 of file klocalsocket.cpp.
|
virtual |
Destroys the KLocalSocket object and frees up any resources associated.
If the socket is open, it will be closed.
Definition at line 49 of file klocalsocket.cpp.
Member Function Documentation
|
protectedslot |
Definition at line 67 of file klocalsocket.cpp.
void KLocalSocket::connectToPath | ( | const QString & | path, |
OpenMode | mode = ReadWrite |
||
) |
Opens a connection to a listening Unix socket at path
.
Use waitForConnection() to find out if the connection succeeded or not.
- Parameters
-
path the Unix socket to connect to mode the mode to use when opening (see QIODevice::OpenMode)
Definition at line 55 of file klocalsocket.cpp.
void KLocalSocket::connectToPath | ( | const QString & | path, |
LocalSocketType | type, | ||
OpenMode | mode = ReadWrite |
||
) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts. Opens a connection to a listening local socket at address path
.
Use waitForConnection() to find out if the connection succeeded or not.
- Parameters
-
path the local socket address to connect to type the local socket type to use mode the mode to use when opening (see QIODevice::OpenMode)
Definition at line 61 of file klocalsocket.cpp.
|
protectedslot |
Definition at line 81 of file klocalsocket.cpp.
void KLocalSocket::disconnectFromPath | ( | ) |
Disconnects the socket from its server.
Definition at line 90 of file klocalsocket.cpp.
QString KLocalSocket::localPath | ( | ) | const |
Returns the local address of this socket, when connected.
Returns QString() if not connected.
Most of the time, the socket has no local address.
Definition at line 101 of file klocalsocket.cpp.
KLocalSocket::LocalSocketType KLocalSocket::localSocketType | ( | ) | const |
Returns the socket type for this socket, when connected.
Returns UnknownLocalSocketType if not connected.
Definition at line 96 of file klocalsocket.cpp.
QString KLocalSocket::peerPath | ( | ) | const |
Returns the peer address of this socket.
That is, the address that this socket connected to (see connectToPath). Returns QString() if not connected.
Definition at line 106 of file klocalsocket.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:47:11 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.