KDECore
#include <KPluginLoader>
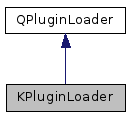
Public Member Functions | |
KPluginLoader (const QString &plugin, const KComponentData &componentdata=KGlobal::mainComponent(), QObject *parent=0) | |
KPluginLoader (const KService &service, const KComponentData &componentdata=KGlobal::mainComponent(), QObject *parent=0) | |
~KPluginLoader () | |
QString | errorString () const |
KPluginFactory * | factory () |
bool | isLoaded () const |
QString | pluginName () const |
quint32 | pluginVersion () const |
Protected Member Functions | |
bool | load () |
Properties | |
QString | fileName |
QString | pluginName |
Related Functions | |
(Note that these are not member functions.) | |
#define | K_EXPORT_PLUGIN(factory) |
#define | K_EXPORT_PLUGIN_VERSION(version) Q_EXTERN_C KDE_EXPORT const quint32 kde_plugin_version = version; |
Detailed Description
This class can be used to dynamically load a plugin library at runtime.
This class makes sure that the Qt and KDE versions used to compile this library aren't newer than the ones currently used.
This class is reentrant, you can load plugins from different threads. You can also have multiple PluginLoaders for one library without negative effects. The object obtained with factory() or the inherited method QPluginLoader::instance() is cached inside the library. If you call factory() or instance() multiple times, you will always get the same object, even from different threads and different KPluginLoader instances. You can delete this object easily, a new one will be created if factory() or instance() is called afterwards. factory() uses instance() internally.
KPluginLoader inherits QPluginLoader::unload(). It is safe to call this method if you loaded a plugin and decide not to use it for some reason. But as soon as you start to use the factory from the plugin, you should stay away from it. It's nearly impossible to keep track of all objects created directly or indirectly from the plugin and all other pointers into plugin code. Using unload() in this case is asking for trouble. If you really need to unload your plugins, you have to take care to convert the clipboard content to text, because the plugin could have registered a custom mime source. You also have to delete the factory of the plugin, otherwise you will create a leak. The destructor of KPluginLoader doesn't call unload.
Sample code:
- See also
- KPluginFactory
Definition at line 79 of file kpluginloader.h.
Constructor & Destructor Documentation
|
explicit |
Used this constructor to load a plugin with a given library name.
Plugin libraries shouldn't have a 'lib' prefix.
errorString() will be set if problems are encountered.
- Parameters
-
plugin The name of the plugin library. componentdata The KStandardDirs object from componentdata is used to search the library. parent A parent object.
Definition at line 133 of file kpluginloader.cpp.
|
explicit |
Used this constructor to load a plugin from a service.
The service must contain a library.
errorString() will be set if problems are encountered.
- Parameters
-
service The service for which the library should be loaded. componentdata The KStandardDirs object from componentdata is used to search the library. parent A parent object.
Definition at line 150 of file kpluginloader.cpp.
KPluginLoader::~KPluginLoader | ( | ) |
Destroys the plugin loader.
Definition at line 180 of file kpluginloader.cpp.
Member Function Documentation
QString KPluginLoader::errorString | ( | ) | const |
Queries the last error.
- Returns
- The description of the last error.
Definition at line 259 of file kpluginloader.cpp.
KPluginFactory * KPluginLoader::factory | ( | ) |
Used to obtain the factory object of the plugin.
You have to give a class which inherits KPluginFactory to K_EXPORT_PLUGIN to use this method.
- Returns
- The factory of the plugin or 0 on error.
Definition at line 185 of file kpluginloader.cpp.
bool KPluginLoader::isLoaded | ( | ) | const |
Definition at line 128 of file kpluginloader.cpp.
|
protected |
Performs the loading of the plugin.
Definition at line 217 of file kpluginloader.cpp.
QString KPluginLoader::pluginName | ( | ) | const |
The name of this plugin as given to the constructor.
- Returns
- the plugin name
quint32 KPluginLoader::pluginVersion | ( | ) | const |
Queries the plugin version.
- Returns
- The version given to K_EXPORT_PLUGIN_VERSION or (quint32) -1 if not set.
Definition at line 269 of file kpluginloader.cpp.
Friends And Related Function Documentation
|
related |
This macro exports the main object of the plugin. Most times, this will be a KPluginFactory or derived class, but any QObject derived class can be used. Take a look at the documentation of Q_EXPORT_PLUGIN2 for some details.
Definition at line 71 of file kexportplugin.h.
|
related |
Use this macro if you want to give your plugin a version number. You can later access the version number with KPluginLoader::pluginVersion()
Definition at line 61 of file kexportplugin.h.
Property Documentation
|
read |
Definition at line 82 of file kpluginloader.h.
|
read |
Definition at line 83 of file kpluginloader.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:47:11 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.