KDECore
#include <kservicegroup.h>
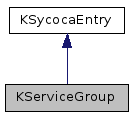
Public Types | |
enum | EntriesOption { NoOptions = 0x0, SortEntries = 0x1, ExcludeNoDisplay = 0x2, AllowSeparators = 0x4, SortByGenericName = 0x8 } |
typedef QList< SPtr > | List |
typedef KSharedPtr< KServiceGroup > | Ptr |
typedef KSharedPtr< KSycocaEntry > | SPtr |
![]() | |
typedef QList< Ptr > | List |
typedef KSharedPtr< KSycocaEntry > | Ptr |
Static Public Member Functions | |
static Ptr | baseGroup (const QString &baseGroupName) |
static Ptr | childGroup (const QString &parent) |
static Ptr | group (const QString &relPath) |
static Ptr | root () |
![]() | |
static void | read (QDataStream &s, QString &str) |
static void | read (QDataStream &s, QStringList &list) |
Protected Member Functions | |
void | addEntry (const KSycocaEntry::Ptr &entry) |
![]() | |
KSycocaEntry (KSycocaEntryPrivate &d) | |
Additional Inherited Members | |
![]() | |
KSycocaEntryPrivate * | d_ptr |
![]() | |
enum | KSycocaType |
Detailed Description
KServiceGroup represents a group of service, for example screensavers.
This class is typically used like this:
Represents a group of services
Definition at line 62 of file kservicegroup.h.
Member Typedef Documentation
typedef QList<SPtr> KServiceGroup::List |
Definition at line 68 of file kservicegroup.h.
typedef KSharedPtr<KServiceGroup> KServiceGroup::Ptr |
Definition at line 66 of file kservicegroup.h.
typedef KSharedPtr<KSycocaEntry> KServiceGroup::SPtr |
Definition at line 67 of file kservicegroup.h.
Member Enumeration Documentation
options for groupEntries and serviceEntries
Enumerator | |
---|---|
NoOptions | |
SortEntries | |
ExcludeNoDisplay | |
AllowSeparators | |
SortByGenericName |
Definition at line 205 of file kservicegroup.h.
Constructor & Destructor Documentation
KServiceGroup::KServiceGroup | ( | const QString & | name | ) |
Construct a dummy servicegroup indexed with name
.
- Parameters
-
name the name of the service group
Definition at line 34 of file kservicegroup.cpp.
Construct a service and take all information from a config file.
- Parameters
-
_fullpath full path to the config file _relpath relative path to the config file
Definition at line 39 of file kservicegroup.cpp.
KServiceGroup::KServiceGroup | ( | QDataStream & | _str, |
int | offset, | ||
bool | deep | ||
) |
construct a service from a stream. The stream must already be positionned at the correct offset
Definition at line 82 of file kservicegroup.cpp.
|
virtual |
Definition at line 90 of file kservicegroup.cpp.
Member Function Documentation
|
protected |
Add a service to this group
Definition at line 263 of file kservicegroup.cpp.
bool KServiceGroup::allowInline | ( | ) | const |
- Returns
- true if we allow to inline menu.
Definition at line 198 of file kservicegroup.cpp.
|
static |
Returns the group for the given baseGroupName.
Can return 0L if the directory (or the .directory file) was deleted.
- Returns
- the base group with the given name, or 0 if not available.
This mechanism was fragile and isn't used in kde4 anymore.
- Deprecated:
- Use a servicetype and a proper trader query instead, for a better way of finding related services.
Definition at line 705 of file kservicegroup.cpp.
QString KServiceGroup::baseGroupName | ( | ) | const |
Returns a non-empty string if the group is a special base group.
By default, "Settings/" is the kcontrol base group ("settings") and "System/Screensavers/" is the screensavers base group ("screensavers"). This allows moving the groups without breaking those apps.
The base group is defined by the X-KDE-BaseGroup key in the .directory file.
- Returns
- the base group name, or null if no base group
Definition at line 730 of file kservicegroup.cpp.
QString KServiceGroup::caption | ( | ) | const |
Returns the caption of this group.
- Returns
- the caption of this group
Definition at line 99 of file kservicegroup.cpp.
int KServiceGroup::childCount | ( | ) | const |
Returns the total number of displayable services in this group and any of its subgroups.
- Returns
- the number of child services
Definition at line 117 of file kservicegroup.cpp.
|
static |
Returns the group of services that have X-KDE-ParentApp equal to parent
(siblings).
- Parameters
-
parent the name of the service's parent
- Returns
- the services group
Definition at line 725 of file kservicegroup.cpp.
QString KServiceGroup::comment | ( | ) | const |
Returns the comment about this service group.
- Returns
- the descriptive comment for the group, if there is one, or QString() if not set
Definition at line 111 of file kservicegroup.cpp.
QString KServiceGroup::directoryEntryPath | ( | ) | const |
Returns a path to the .directory file describing this service group.
The path is either absolute or relative to the "apps" resource.
Definition at line 736 of file kservicegroup.cpp.
KServiceGroup::List KServiceGroup::entries | ( | bool | sorted, |
bool | excludeNoDisplay, | ||
bool | allowSeparators, | ||
bool | sortByGenericName = false |
||
) |
List of all Services and ServiceGroups within this ServiceGroup.
- Parameters
-
sorted true to sort items excludeNoDisplay true to exclude items marked "NoDisplay" allowSeparators true to allow separator items to be included sortByGenericName true to sort GenericName+Name instead of Name+GenericName
- Returns
- the list of entries
Definition at line 356 of file kservicegroup.cpp.
KServiceGroup::List KServiceGroup::entries | ( | bool | sorted, |
bool | excludeNoDisplay | ||
) |
Definition at line 349 of file kservicegroup.cpp.
KServiceGroup::List KServiceGroup::entries | ( | bool | sorted = false | ) |
List of all Services and ServiceGroups within this ServiceGroup.
- Parameters
-
sorted true to sort items
- Returns
- the list of entried
Definition at line 342 of file kservicegroup.cpp.
|
static |
Returns the group with the given relative path.
- Parameters
-
relPath the path of the service group
- Returns
- the group with the given relative path name.
Definition at line 718 of file kservicegroup.cpp.
QList< KServiceGroup::Ptr > KServiceGroup::groupEntries | ( | EntriesOptions | options = ExcludeNoDisplay | ) |
subgroups for this service group
Definition at line 305 of file kservicegroup.cpp.
QString KServiceGroup::icon | ( | ) | const |
Returns the name of the icon associated with the group.
- Returns
- the name of the icon associated with the group, or QString() if not set
Definition at line 105 of file kservicegroup.cpp.
bool KServiceGroup::inlineAlias | ( | ) | const |
- Returns
- true to show an inline alias item into menu
Definition at line 162 of file kservicegroup.cpp.
int KServiceGroup::inlineValue | ( | ) | const |
- Returns
- inline limite value
Definition at line 186 of file kservicegroup.cpp.
QStringList KServiceGroup::layoutInfo | ( | ) | const |
Returns information related to the layout of services in this group.
Definition at line 697 of file kservicegroup.cpp.
bool KServiceGroup::noDisplay | ( | ) | const |
Returns true if the NoDisplay flag was set, i.e.
if this group should be hidden from menus, while still being in ksycoca.
- Returns
- true to hide this service group, false to display it
Definition at line 210 of file kservicegroup.cpp.
QString KServiceGroup::relPath | ( | ) | const |
Returns the relative path of the service group.
- Returns
- the service group's relative path
Definition at line 94 of file kservicegroup.cpp.
|
static |
Returns the root service group.
- Returns
- the root service group
Definition at line 712 of file kservicegroup.cpp.
KService::List KServiceGroup::serviceEntries | ( | EntriesOptions | options = ExcludeNoDisplay | ) |
entries of this service group
Definition at line 322 of file kservicegroup.cpp.
void KServiceGroup::setAllowInline | ( | bool | _b | ) |
Definition at line 204 of file kservicegroup.cpp.
void KServiceGroup::setInlineAlias | ( | bool | _b | ) |
Definition at line 168 of file kservicegroup.cpp.
void KServiceGroup::setInlineValue | ( | int | _val | ) |
Definition at line 192 of file kservicegroup.cpp.
void KServiceGroup::setLayoutInfo | ( | const QStringList & | layout | ) |
Sets information related to the layout of services in this group.
Definition at line 691 of file kservicegroup.cpp.
void KServiceGroup::setShowEmptyMenu | ( | bool | b | ) |
Definition at line 174 of file kservicegroup.cpp.
void KServiceGroup::setShowInlineHeader | ( | bool | _b | ) |
Definition at line 180 of file kservicegroup.cpp.
bool KServiceGroup::showEmptyMenu | ( | ) | const |
Return true if we want to display empty menu entry.
- Returns
- true to show this service group as menu entry is empty, false to hide it
Definition at line 156 of file kservicegroup.cpp.
bool KServiceGroup::showInlineHeader | ( | ) | const |
- Returns
- true to show an inline header into menu
Definition at line 150 of file kservicegroup.cpp.
QStringList KServiceGroup::suppressGenericNames | ( | ) | const |
Returns a list of untranslated generic names that should be be suppressed when showing this group.
E.g. The group "Games/Arcade" might want to suppress the generic name "Arcade Game" since it's redundant in this particular context.
Definition at line 216 of file kservicegroup.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:47:11 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.