KDECore
netsupp.h File Reference
#include <kdecore_export.h>
#include <config.h>
#include <config-network.h>
#include <sys/socket.h>
#include <netdb.h>
Include dependency graph for netsupp.h:
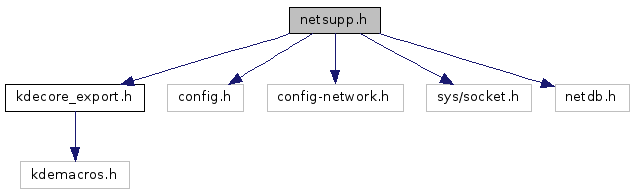
This graph shows which files directly or indirectly include this file:
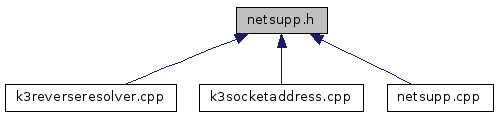
Go to the source code of this file.
Classes | |
struct | addrinfo |
struct | kde_addrinfo |
struct | kde_in6_addr |
struct | kde_sockaddr_in6 |
Namespaces | |
KDE | |
Macros | |
#define | AF_LOCAL AF_UNIX |
#define | AI_CANONNAME 2 /* Request for canonical name. */ |
#define | AI_NUMERICHOST 4 /* Don't use name resolution. */ |
#define | AI_PASSIVE 1 /* Socket address is intended for `bind'. */ |
#define | EAI_ADDRFAMILY 1 /* Address family for NAME not supported. */ |
#define | EAI_AGAIN 2 /* Temporary failure in name resolution. */ |
#define | EAI_BADFLAGS 3 /* Invalid value for `ai_flags' field. */ |
#define | EAI_FAIL 4 /* Non-recoverable failure in name res. */ |
#define | EAI_FAMILY 5 /* `ai_family' not supported. */ |
#define | EAI_MEMORY 6 /* Memory allocation failure. */ |
#define | EAI_NODATA 7 /* No address associated with NAME. */ |
#define | EAI_NONAME 8 /* NAME or SERVICE is unknown. */ |
#define | EAI_SERVICE 9 /* SERVICE not supported for `ai_socktype'. */ |
#define | EAI_SOCKTYPE 10 /* `ai_socktype' not supported. */ |
#define | EAI_SYSTEM 11 /* System error returned in `errno'. */ |
#define | freeaddrinfo KDE::freeaddrinfo |
#define | getaddrinfo KDE::getaddrinfo |
#define | getnameinfo KDE::getnameinfo |
#define | inet_ntop KDE::inet_ntop |
#define | inet_pton KDE::inet_pton |
#define | KAI_LOCALUNIX 1 /* data contains a Unix addrinfo allocated by us */ |
#define | KAI_QDNS 2 /* data contains data derived from QDns */ |
#define | KAI_SYSTEM 0 /* data is all-system */ |
#define | KDE_IN6_ARE_ADDR_EQUAL(a, b) |
#define | KDE_IN6_IS_ADDR_LINKLOCAL(a) ((((quint32 *) (a))[0] & htonl (0xffc00000)) == htonl (0xfe800000)) |
#define | KDE_IN6_IS_ADDR_LOOPBACK(a) |
#define | KDE_IN6_IS_ADDR_MC_GLOBAL(a) (KDE_IN6_IS_ADDR_MULTICAST(a) && ((((quint8 *) (a))[1] & 0xf) == 0xe)) |
#define | KDE_IN6_IS_ADDR_MC_LINKLOCAL(a) (KDE_IN6_IS_ADDR_MULTICAST(a) && ((((quint8 *) (a))[1] & 0xf) == 0x2)) |
#define | KDE_IN6_IS_ADDR_MC_NODELOCAL(a) (KDE_IN6_IS_ADDR_MULTICAST(a) && ((((quint8 *) (a))[1] & 0xf) == 0x1)) |
#define | KDE_IN6_IS_ADDR_MC_ORGLOCAL(a) (KDE_IN6_IS_ADDR_MULTICAST(a) && ((((quint8 *) (a))[1] & 0xf) == 0x8)) |
#define | KDE_IN6_IS_ADDR_MC_SITELOCAL(a) (KDE_IN6_IS_ADDR_MULTICAST(a) && ((((quint8 *) (a))[1] & 0xf) == 0x5)) |
#define | KDE_IN6_IS_ADDR_MULTICAST(a) (((u_int8_t *) (a))[0] == 0xff) |
#define | KDE_IN6_IS_ADDR_SITELOCAL(a) ((((quint32 *) (a))[0] & htonl (0xffc00000)) == htonl (0xfec00000)) |
#define | KDE_IN6_IS_ADDR_UNSPECIFIED(a) |
#define | KDE_IN6_IS_ADDR_V4COMPAT(a) |
#define | KDE_IN6_IS_ADDR_V4MAPPED(a) |
#define | NI_DGRAM 16 /* Look up UDP service rather than TCP. */ |
#define | NI_MAXHOST 1025 |
#define | NI_MAXSERV 32 |
#define | NI_NAMEREQD 8 /* Don't return numeric addresses. */ |
#define | NI_NOFQDN 4 /* Only return nodename portion. */ |
#define | NI_NUMERICHOST 1 /* Don't try to look up hostname. */ |
#define | NI_NUMERICSERV 2 /* Don't convert port number to name. */ |
#define | PF_LOCAL PF_UNIX |
Functions | |
void | KDE::freeaddrinfo (struct addrinfo *ai) |
char * | gai_strerror (int errorcode) |
int | KDE::getaddrinfo (const char *name, const char *service, const struct addrinfo *hint, struct addrinfo **result) |
int | KDE::getnameinfo (const struct sockaddr *sa, kde_socklen_t salen, char *host, size_t hostlen, char *serv, size_t servlen, int flags) |
const char * | KDE::inet_ntop (int af, const void *cp, char *buf, size_t len) |
int | KDE::inet_pton (int af, const char *cp, void *buf) |
void | kde_freeaddrinfo (struct kde_addrinfo *p) |
int | kde_getaddrinfo (const char *name, const char *service, const struct addrinfo *hint, struct kde_addrinfo **result) |
Macro Definition Documentation
#define AI_PASSIVE 1 /* Socket address is intended for `bind'. */ |
#define EAI_ADDRFAMILY 1 /* Address family for NAME not supported. */ |
#define EAI_AGAIN 2 /* Temporary failure in name resolution. */ |
#define EAI_BADFLAGS 3 /* Invalid value for `ai_flags' field. */ |
#define EAI_FAIL 4 /* Non-recoverable failure in name res. */ |
#define EAI_NODATA 7 /* No address associated with NAME. */ |
#define EAI_SERVICE 9 /* SERVICE not supported for `ai_socktype'. */ |
#define EAI_SOCKTYPE 10 /* `ai_socktype' not supported. */ |
#define EAI_SYSTEM 11 /* System error returned in `errno'. */ |
#define KAI_LOCALUNIX 1 /* data contains a Unix addrinfo allocated by us */ |
#define KAI_QDNS 2 /* data contains data derived from QDns */ |
#define KDE_IN6_ARE_ADDR_EQUAL | ( | a, | |
b | |||
) |
#define KDE_IN6_IS_ADDR_LINKLOCAL | ( | a | ) | ((((quint32 *) (a))[0] & htonl (0xffc00000)) == htonl (0xfe800000)) |
#define KDE_IN6_IS_ADDR_LOOPBACK | ( | a | ) |
#define KDE_IN6_IS_ADDR_MC_GLOBAL | ( | a | ) | (KDE_IN6_IS_ADDR_MULTICAST(a) && ((((quint8 *) (a))[1] & 0xf) == 0xe)) |
#define KDE_IN6_IS_ADDR_MC_LINKLOCAL | ( | a | ) | (KDE_IN6_IS_ADDR_MULTICAST(a) && ((((quint8 *) (a))[1] & 0xf) == 0x2)) |
#define KDE_IN6_IS_ADDR_MC_NODELOCAL | ( | a | ) | (KDE_IN6_IS_ADDR_MULTICAST(a) && ((((quint8 *) (a))[1] & 0xf) == 0x1)) |
#define KDE_IN6_IS_ADDR_MC_ORGLOCAL | ( | a | ) | (KDE_IN6_IS_ADDR_MULTICAST(a) && ((((quint8 *) (a))[1] & 0xf) == 0x8)) |
#define KDE_IN6_IS_ADDR_MC_SITELOCAL | ( | a | ) | (KDE_IN6_IS_ADDR_MULTICAST(a) && ((((quint8 *) (a))[1] & 0xf) == 0x5)) |
#define KDE_IN6_IS_ADDR_MULTICAST | ( | a | ) | (((u_int8_t *) (a))[0] == 0xff) |
#define KDE_IN6_IS_ADDR_SITELOCAL | ( | a | ) | ((((quint32 *) (a))[0] & htonl (0xffc00000)) == htonl (0xfec00000)) |
#define KDE_IN6_IS_ADDR_UNSPECIFIED | ( | a | ) |
#define KDE_IN6_IS_ADDR_V4COMPAT | ( | a | ) |
#define KDE_IN6_IS_ADDR_V4MAPPED | ( | a | ) |
#define NI_DGRAM 16 /* Look up UDP service rather than TCP. */ |
#define NI_NAMEREQD 8 /* Don't return numeric addresses. */ |
#define NI_NUMERICHOST 1 /* Don't try to look up hostname. */ |
#define NI_NUMERICSERV 2 /* Don't convert port number to name. */ |
Function Documentation
char* gai_strerror | ( | int | errorcode | ) |
Definition at line 890 of file netsupp.cpp.
void kde_freeaddrinfo | ( | struct kde_addrinfo * | p | ) |
Definition at line 101 of file netsupp.cpp.
int kde_getaddrinfo | ( | const char * | name, |
const char * | service, | ||
const struct addrinfo * | hint, | ||
struct kde_addrinfo ** | result | ||
) |
Definition at line 230 of file netsupp.cpp.
This file is part of the KDE documentation.
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:47:10 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:47:10 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.