KDEUI
#include <kaction.h>
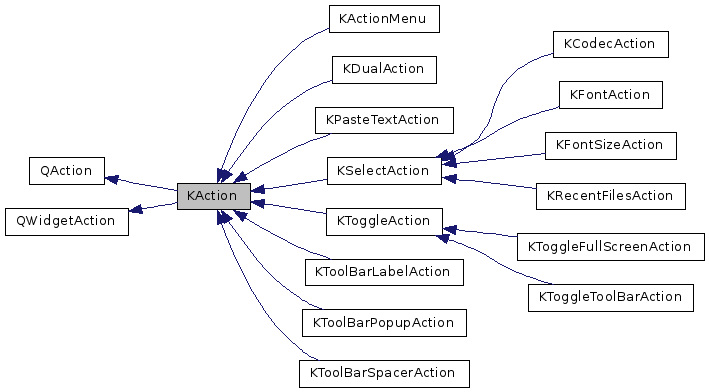
Public Types | |
enum | GlobalShortcutLoading { Autoloading = 0x0, NoAutoloading = 0x4 } |
enum | ShortcutType { ActiveShortcut = 0x1, DefaultShortcut = 0x2 } |
Signals | |
void | authorized (KAuth::Action *action) |
void | globalShortcutChanged (const QKeySequence &) |
void | triggered (Qt::MouseButtons buttons, Qt::KeyboardModifiers modifiers) |
Properties | |
KShortcut | globalShortcut |
bool | globalShortcutAllowed |
bool | globalShortcutEnabled |
KShortcut | shortcut |
bool | shortcutConfigurable |
Detailed Description
Class to encapsulate user-driven action or event.
The KAction class (and derived and super classes) extends QAction, which provides a way to easily encapsulate a "real" user-selected action or event in your program.
For instance, a user may want to paste
the contents of the clipboard, scroll
down
a document, or quit
the application. These are all actions – events that the user causes to happen. The KAction class allows the developer to deal with these actions in an easy and intuitive manner, and conforms to KDE's extended functionality requirements - including supporting multiple user-configurable shortcuts, and KDE named icons. Actions also improve accessibility.
Specifically, QAction (and thus KAction) encapsulates the various attributes of an event/action. For instance, an action might have an icon() that provides a visual representation (a clipboard for a "paste" action or scissors for a "cut" action). The action should also be described by some text(). It will certainly be connected to a method that actually executes
the action! All these attributes are contained within the action object.
The advantage of dealing with actions is that you can manipulate the Action without regard to the GUI representation of it. For instance, in the "normal" way of dealing with actions like "cut", you would manually insert a item for Cut into a menu and a button into a toolbar. If you want to disable the cut action for a moment (maybe nothing is selected), you would have to hunt down the pointer to the menu item and the toolbar button and disable both individually. Setting the menu item and toolbar item up uses very similar code - but has to be done twice!
With the action concept, you simply add the action to whatever GUI element you want. The KAction class will then take care of correctly defining the menu item (with icons, accelerators, text, etc), toolbar button, or other. From then on, if you manipulate the action at all, the effect will propagate through all GUI representations of it. Back to the "cut" example: if you want to disable the Cut Action, you would simply call 'cutAction->setEnabled(false)' and both the menuitem and button would instantly be disabled!
This is the biggest advantage to the action concept – there is a one-to-one relationship between the "real" action and all
GUI representations of it.
KAction emits the hovered() signal on mouseover, and the triggered(bool checked) signal on activation of a corresponding GUI element ( menu item, toolbar button, etc. )
If you are in the situation of wanting to map the triggered() signal of multiple action objects to one slot, with a special argument bound to each action, you have several options:
Using QActionGroup:
- Create a QActionGroup and assign it to each of the actions with setActionGroup(), then
- Connect the QActionGroup::triggered(QAction*) signal to your slot.
Using QSignalMapper:
General Usage
The steps to using actions are roughly as follows:
- Decide which attributes you want to associate with a given action (icons, text, keyboard shortcut, etc)
- Create the action using KAction (or derived or super class).
- Add the action into whatever GUI element you want. Typically, this will be a menu or toolbar.
General Usage
Local shortcuts are active if their context has the focus, global shortcus are active even if the program does not have the focus. If a global shortcut and a local shortcut are ambiguous the global shortcut wins.
- Active shortcuts trigger a KAction if activated.
- Default shortcuts are what the active shortcuts revert to if the user chooses to reset shortcuts to default.
Detailed Example
Here is an example of enabling a "New [document]" action
This section creates our action. Text, Icon and Shortcut will be set from KStandardAction. KStandardAction ensures your application complies to the platform standards. When triggered the fileNew()
slot will be called.
- See also
- KStandardAction for more information.
If you want to create your own actions use
This section creates our action. It displays the text "Quick Connect", uses the Icon "quick-connect" and pressing F6
will trigger it. When invoked, the slot quickConnect() is called.
That just inserted the action into the File menu. The point is, it's not important in which menu it is: all manipulation of the item is done through the newAct object.
And this added the action into the main toolbar as a button.
That's it!
If you want to disable that action sometime later, you can do so with
and both the menuitem in File and the toolbar button will instantly be disabled.
Unlike with previous versions of KDE, the action can simply be deleted when you have finished with it - the destructor takes care of all of the cleanup.
- Warning
- calling QAction::setShortcut() on a KAction may lead to unexpected behavior. There is nothing we can do about it because QAction::setShortcut() is not virtual.
- Note
- if you are using a "standard" action like "new", "paste", "quit", or any other action described in the KDE UI Standards, please use the methods in the KStandardAction class rather than defining your own.
QActions
Mixing QActions and KActions in an application is not a good idea. KShortcutsEditor doesn't handle QActions at all.
Usage Within the XML Framework
If you are using KAction within the context of the XML menu and toolbar building framework, you do not ever have to add your actions to containers manually. The framework does that for you.
- See also
- KStandardAction
Member Enumeration Documentation
An enum about global shortcut setter semantics.
Enumerator | |
---|---|
Autoloading |
Look up the action in global settings (using its main component's name and text()) and set the shortcut as saved there.
|
NoAutoloading |
Prevent autoloading of saved global shortcut for action. |
An enumeration about the two types of shortcuts in a KAction.
Constructor & Destructor Documentation
|
explicit |
Constructs an action.
Definition at line 139 of file kaction.cpp.
Constructs an action with the specified parent and visible text.
- Parameters
-
text The visible text for this action. parent The parent for this action.
Definition at line 145 of file kaction.cpp.
Constructs an action with text and icon; a shortcut may be specified by the ampersand character (e.g.
"&Option" creates a shortcut with key O )
This is the other common KAction constructor used. Use it when you do have a corresponding icon.
- Parameters
-
icon The icon to display. text The text that will be displayed. parent The parent for this action.
Definition at line 152 of file kaction.cpp.
|
virtual |
Standard destructor.
Definition at line 160 of file kaction.cpp.
Member Function Documentation
KAuth::Action * KAction::authAction | ( | ) | const |
Returns the action object associated with this action, or 0 if it does not have one.
- Returns
- the KAuth::Action associated with this action.
Definition at line 385 of file kaction.cpp.
|
signal |
Signal emitted when the action is triggered and authorized.
If the action needs authorization, when the user triggers the action, the authorization process automatically begins. If it succeeds, this signal is emitted. The KAuth::Action object is provided for convenience if you have multiple KAuthorizedAction objects, but of course it's always the same set with setAuthAction().
WARNING: If your action needs authorization you should connect eventual slots processing stuff to this signal, and NOT triggered. Triggered will be emitted even if the user has not been authorized
- Parameters
-
action The object set with setAuthAction()
bool KAction::event | ( | QEvent * | event | ) |
- Reimplemented from superclass.
Definition at line 115 of file kaction.cpp.
void KAction::forgetGlobalShortcut | ( | ) |
Sets the globalShortcutEnabled property to false and sets the global shortcut to an empty shortcut.
This will also wipe out knowlegde about the existence of this action's global shortcut so it will not be considered anymore for shortcut conflict resolution. It will also not be visible anymore in the shortcuts KControl module. This method should not be used unless these effects are explicitly desired.
- Since
- 4.1
Definition at line 312 of file kaction.cpp.
const KShortcut & KAction::globalShortcut | ( | ShortcutTypes | type = ActiveShortcut | ) | const |
Get the global shortcut for this action, if one exists.
Global shortcuts allow your actions to respond to accellerators independently of the focused window. Unlike regular shortcuts, the application's window does not need focus for them to be activated.
- Parameters
-
type the type of shortcut to be returned. Should both be specified, only the active shortcut will be returned. Defaults to the active shortcut, if one exists.
- See also
- KGlobalAccel
- setGlobalShortcut()
Definition at line 229 of file kaction.cpp.
bool KAction::globalShortcutAllowed | ( | ) | const |
Returns true if this action is permitted to have a global shortcut.
Defaults to false. Use isGlobalShortcutEnabled() instead.
|
signal |
Emitted when the global shortcut is changed.
A global shortcut is subject to be changed by the global shortcuts kcm.
bool KAction::isGlobalShortcutEnabled | ( | ) | const |
Definition at line 296 of file kaction.cpp.
bool KAction::isShortcutConfigurable | ( | ) | const |
Returns true if this action's shortcut is configurable.
Definition at line 173 of file kaction.cpp.
KRockerGesture KAction::rockerGesture | ( | ShortcutTypes | type = ActiveShortcut | ) | const |
Definition at line 332 of file kaction.cpp.
void KAction::setAuthAction | ( | KAuth::Action * | action | ) |
Sets the action object associated with this action.
By setting a KAuth::Action, this action will become associated with it, and whenever it gets clicked, it will trigger the authorization and execution process for the action. The signal activated will also be emitted whenever the action gets clicked and the action gets authorized. Pass 0 to this function to disassociate the action
- Parameters
-
action the KAuth::Action to associate with this action.
Definition at line 400 of file kaction.cpp.
void KAction::setAuthAction | ( | const QString & | actionName | ) |
Sets the action object associated with this action.
Overloaded member to allow creating the action by name
- Parameters
-
actionName the name of the action to associate
Definition at line 390 of file kaction.cpp.
void KAction::setGlobalShortcut | ( | const KShortcut & | shortcut, |
ShortcutTypes | type = ShortcutTypes(ActiveShortcut | DefaultShortcut) , |
||
GlobalShortcutLoading | loading = Autoloading |
||
) |
Assign a global shortcut for this action.
Global shortcuts allow an action to respond to key shortcuts independently of the focused window, i.e. the action will trigger if the keys were pressed no matter where in the X session.
The action must have a per main component unique objectName() to enable cross-application bookeeping. If the objectName() is empty this method will do nothing, otherwise the isGlobalShortcutEnabled() property will be set to true and the shortcut will be enabled. It is mandatory that the objectName() doesn't change once isGlobalshortcutEnabled() has become true.
- Note
- KActionCollection::insert(name, action) will set action's objectName to name so you often don't have to set an objectName explicitly.
When an action, identified by main component name and objectName(), is assigned a global shortcut for the first time on a KDE installation the assignment will be saved. The shortcut will then be restored every time setGlobalShortcut() is called with loading
== Autoloading.
If you actually want to change the global shortcut you have to set loading
to NoAutoloading. The new shortcut will be automatically saved again.
- Parameters
-
shortcut global shortcut(s) to assign. Will be ignored unless loading
is set to NoAutoloading or this is the first time ever you call this method (see above).type the type of shortcut to be set, whether the active shortcut, the default shortcut, or both (the default). loading if Autoloading, assign the global shortcut this action has previously had if any. That way user preferences and changes made to avoid clashes will be conserved. if NoAutoloading the given shortcut will be assigned without looking up old values. You should only do this if the user wants to change the shortcut or if you have another very good reason. Key combinations that clash with other shortcuts will be dropped.
- Note
- the default shortcut will never be influenced by autoloading - it will be set as given.
- See also
- globalShortcut()
Definition at line 239 of file kaction.cpp.
void KAction::setGlobalShortcutAllowed | ( | bool | allowed, |
GlobalShortcutLoading | loading = Autoloading |
||
) |
Indicate whether the programmer and/or user may define a global shortcut for this action.
Defaults to false. Note that calling setGlobalShortcut() turns this on automatically.
- Parameters
-
allowed set to true if this action may have a global shortcut, otherwise false. loading if Autoloading, assign to this action the global shortcut it has previously had if any.
Definition at line 302 of file kaction.cpp.
void KAction::setHelpText | ( | const QString & | text | ) |
Sets the help text for the action.
This help text will be set for all help mechanisms:
- the status-bar help text
- the tooltip (for toolbar buttons)
- the "WhatsThis" help text (unless one was already set)
This is more convenient than calling all three methods with the same text, and this level of abstraction can allow to change the default implementation of help one day more easily. Of course you can also call setStatusTip, setToolTip and setWhatsThis separately for more flexibility.
This method is also the easiest way to port from KDE3's KAction::setToolTip.
- Since
- 4.3
Definition at line 377 of file kaction.cpp.
void KAction::setRockerGesture | ( | const KRockerGesture & | gest, |
ShortcutTypes | type = ShortcutTypes(ActiveShortcut | DefaultShortcut) |
||
) |
Definition at line 359 of file kaction.cpp.
void KAction::setShapeGesture | ( | const KShapeGesture & | gest, |
ShortcutTypes | type = ShortcutTypes(ActiveShortcut | DefaultShortcut) |
||
) |
Definition at line 341 of file kaction.cpp.
void KAction::setShortcut | ( | const KShortcut & | shortcut, |
ShortcutTypes | type = ShortcutTypes(ActiveShortcut | DefaultShortcut) |
||
) |
Set the shortcut for this action.
This is preferred over QAction::setShortcut(), as it allows for multiple shortcuts per action.
- Parameters
-
shortcut shortcut(s) to use for this action in its specified shortcutContext() type type of shortcut to be set: active shortcut, default shortcut, or both (the default).
Definition at line 198 of file kaction.cpp.
void KAction::setShortcut | ( | const QKeySequence & | shortcut, |
ShortcutTypes | type = ShortcutTypes(ActiveShortcut | DefaultShortcut) |
||
) |
Definition at line 212 of file kaction.cpp.
void KAction::setShortcutConfigurable | ( | bool | configurable | ) |
Indicate whether the user may configure the action's shortcut.
- Parameters
-
configurable set to true if this shortcut may be configured by the user, otherwise false.
Definition at line 178 of file kaction.cpp.
void KAction::setShortcuts | ( | const QList< QKeySequence > & | shortcuts, |
ShortcutTypes | type = ShortcutTypes(ActiveShortcut | DefaultShortcut) |
||
) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
This function is there to explicitly override QAction::setShortcut(const QList<QKeySequence>&). QAction::setShortcuts() will bypass everything in KAction and may lead to unexpected behavior.
- Parameters
-
shortcut shortcut(s) to use for this action in its specified shortcutContext() type type of shortcut to be set: active shortcut, default shortcut, or both (default argument value).
Definition at line 224 of file kaction.cpp.
KShapeGesture KAction::shapeGesture | ( | ShortcutTypes | type = ActiveShortcut | ) | const |
Definition at line 323 of file kaction.cpp.
KShortcut KAction::shortcut | ( | ShortcutTypes | types = ActiveShortcut | ) | const |
Get the shortcut for this action.
This is preferred over QAction::shortcut(), as it allows for multiple shortcuts per action. The first and second shortcut as reported by shortcuts() will be the primary and alternate shortcut of the shortcut returned.
- Parameters
-
types the type of shortcut to return. Should both be specified, only the active shortcut will be returned. Defaults to the active shortcut, if one exists.
- See also
- shortcuts()
Definition at line 183 of file kaction.cpp.
|
signal |
Emitted when the action is triggered.
Also provides the state of the keyboard modifiers and mouse buttons at the time.
Property Documentation
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:49:17 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.