KDEUI
#include <kcategorizedview.h>
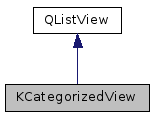
Public Member Functions | |
KCategorizedView (QWidget *parent=0) | |
~KCategorizedView () | |
bool | alternatingBlockColors () const |
QModelIndexList | block (const QString &category) |
QModelIndexList | block (const QModelIndex &representative) |
KCategoryDrawer * | categoryDrawer () const |
int | categorySpacing () const |
bool | collapsibleBlocks () const |
virtual QModelIndex | indexAt (const QPoint &point) const |
virtual void | reset () |
void | setAlternatingBlockColors (bool enable) |
void | setCategoryDrawer (KCategoryDrawer *categoryDrawer) |
void | setCategorySpacing (int categorySpacing) |
void | setCollapsibleBlocks (bool enable) |
void | setGridSize (const QSize &size) |
void | setGridSizeOwn (const QSize &size) |
virtual void | setModel (QAbstractItemModel *model) |
virtual QRect | visualRect (const QModelIndex &index) const |
Protected Slots | |
virtual void | rowsInsertedArtifficial (const QModelIndex &parent, int start, int end) |
virtual void | rowsRemoved (const QModelIndex &parent, int start, int end) |
virtual void | slotLayoutChanged () |
Protected Member Functions | |
virtual void | currentChanged (const QModelIndex ¤t, const QModelIndex &previous) |
virtual void | dataChanged (const QModelIndex &topLeft, const QModelIndex &bottomRight) |
virtual void | dragEnterEvent (QDragEnterEvent *event) |
virtual void | dragLeaveEvent (QDragLeaveEvent *event) |
virtual void | dragMoveEvent (QDragMoveEvent *event) |
virtual void | dropEvent (QDropEvent *event) |
virtual void | leaveEvent (QEvent *event) |
virtual void | mouseMoveEvent (QMouseEvent *event) |
virtual void | mousePressEvent (QMouseEvent *event) |
virtual void | mouseReleaseEvent (QMouseEvent *event) |
virtual QModelIndex | moveCursor (CursorAction cursorAction, Qt::KeyboardModifiers modifiers) |
virtual void | paintEvent (QPaintEvent *event) |
virtual void | resizeEvent (QResizeEvent *event) |
virtual void | rowsAboutToBeRemoved (const QModelIndex &parent, int start, int end) |
virtual void | rowsInserted (const QModelIndex &parent, int start, int end) |
virtual void | setSelection (const QRect &rect, QItemSelectionModel::SelectionFlags flags) |
virtual void | startDrag (Qt::DropActions supportedActions) |
virtual void | updateGeometries () |
Properties | |
bool | alternatingBlockColors |
int | categorySpacing |
bool | collapsibleBlocks |
Detailed Description
Item view for listing items in a categorized fashion optionally.
KCategorizedView basically has the same functionality as QListView, only that it also lets you layout items in a way that they are categorized visually.
For it to work you will need to set a KCategorizedSortFilterProxyModel and a KCategoryDrawer with methods setModel() and setCategoryDrawer() respectively. Also, the model will need to be flagged as categorized with KCategorizedSortFilterProxyModel::setCategorizedModel(true).
The way it works (if categorization enabled):
- When sorting, it does more things than QListView does. It will ask the model for the special role CategorySortRole (@see KCategorizedSortFilterProxyModel). This can return a QString or an int in order to tell the view the order of categories. In this sense, for instance, if we are sorting by name ascending, "A" would be before than "B". If we are sorting by size ascending, 512 bytes would be before 1024 bytes. This way categories are also sorted. - When the view has to paint, it will ask the model with the role CategoryDisplayRole (@see KCategorizedSortFilterProxyModel). It will for instance return "F" for "foo.pdf" if we are sorting by name ascending, or "Small" if a certain item has 100 bytes, for example.
For drawing categories, KCategoryDrawer will be used. You can inherit this class to do your own drawing.
- Note
- All examples cited before talk about filesystems and such, but have present that this is a completely generic class, and it can be used for whatever your purpose is. For instance when talking about animals, you can separate them by "Mammal" and "Oviparous". In this very case, for example, the CategorySortRole and the CategoryDisplayRole could be the same ("Mammal" and "Oviparous").
- There is a really performance boost if CategorySortRole returns an int instead of a QString. Have present that this role is asked (n * log n) times when sorting and compared. Comparing ints is always faster than comparing strings, whithout mattering how fast the string comparison is. Consider thinking of a way of returning ints instead of QStrings if your model can contain a high number of items.
- Warning
- Note that for really drawing items in blocks you will need some things to be done:
- The model set to this view has to be (or inherit if you want to do special stuff in it) KCategorizedSortFilterProxyModel.
- This model needs to be set setCategorizedModel to true.
- Set a category drawer by calling setCategoryDrawer.
Definition at line 79 of file kcategorizedview.h.
Constructor & Destructor Documentation
KCategorizedView::KCategorizedView | ( | QWidget * | parent = 0 | ) |
Definition at line 503 of file kcategorizedview.cpp.
KCategorizedView::~KCategorizedView | ( | ) |
Definition at line 509 of file kcategorizedview.cpp.
Member Function Documentation
bool KCategorizedView::alternatingBlockColors | ( | ) | const |
- Returns
- Whether blocks should be drawn with alternating colors.
- Since
- 4.4
QModelIndexList KCategorizedView::block | ( | const QString & | category | ) |
- Returns
- Block of indexes that are into
category
.
- Since
- 4.5
Definition at line 693 of file kcategorizedview.cpp.
QModelIndexList KCategorizedView::block | ( | const QModelIndex & | representative | ) |
- Returns
- Block of indexes that are represented by
representative
.
- Since
- 4.5
Definition at line 711 of file kcategorizedview.cpp.
KCategoryDrawer * KCategorizedView::categoryDrawer | ( | ) | const |
Returns the current category drawer.
Definition at line 632 of file kcategorizedview.cpp.
int KCategorizedView::categorySpacing | ( | ) | const |
- Returns
- Category spacing. The spacing between categories.
- Since
- 4.4
bool KCategorizedView::collapsibleBlocks | ( | ) | const |
- Returns
- Whether blocks can be collapsed or not.
- Since
- 4.4
|
protectedvirtual |
Reimplemented from QAbstractItemView.
Definition at line 1431 of file kcategorizedview.cpp.
|
protectedvirtual |
Reimplemented from QAbstractItemView.
Definition at line 1437 of file kcategorizedview.cpp.
|
protectedvirtual |
Reimplemented from QAbstractItemView.
Definition at line 1084 of file kcategorizedview.cpp.
|
protectedvirtual |
Reimplemented from QAbstractItemView.
Definition at line 1089 of file kcategorizedview.cpp.
|
protectedvirtual |
Reimplemented from QAbstractItemView.
Definition at line 1078 of file kcategorizedview.cpp.
|
protectedvirtual |
Reimplemented from QAbstractItemView.
Definition at line 1094 of file kcategorizedview.cpp.
|
virtual |
Reimplemented from QAbstractItemView.
Definition at line 716 of file kcategorizedview.cpp.
|
protectedvirtual |
Reimplemented from QWidget.
Definition at line 1056 of file kcategorizedview.cpp.
|
protectedvirtual |
Reimplemented from QWidget.
Definition at line 932 of file kcategorizedview.cpp.
|
protectedvirtual |
Reimplemented from QWidget.
Definition at line 991 of file kcategorizedview.cpp.
|
protectedvirtual |
Reimplemented from QWidget.
Definition at line 1025 of file kcategorizedview.cpp.
|
protectedvirtual |
Reimplemented from QAbstractItemView.
Definition at line 1101 of file kcategorizedview.cpp.
|
protectedvirtual |
Reimplemented from QWidget.
Definition at line 771 of file kcategorizedview.cpp.
|
virtual |
Reimplemented from QAbstractItemView.
Definition at line 765 of file kcategorizedview.cpp.
|
protectedvirtual |
Reimplemented from QWidget.
Definition at line 882 of file kcategorizedview.cpp.
|
protectedvirtual |
Reimplemented from QAbstractItemView.
Definition at line 1218 of file kcategorizedview.cpp.
|
protectedvirtual |
Reimplemented from QAbstractItemView.
Definition at line 1469 of file kcategorizedview.cpp.
|
protectedvirtualslot |
- Warning
- Deprecated since 4.4.
Definition at line 1484 of file kcategorizedview.cpp.
|
protectedvirtualslot |
- Warning
- Deprecated since 4.4.
Definition at line 1495 of file kcategorizedview.cpp.
void KCategorizedView::setAlternatingBlockColors | ( | bool | enable | ) |
Sets whether blocks should be drawn with alternating colors.
- Since
- 4.4
Definition at line 678 of file kcategorizedview.cpp.
void KCategorizedView::setCategoryDrawer | ( | KCategoryDrawer * | categoryDrawer | ) |
The category drawer that will be used for drawing categories.
Definition at line 637 of file kcategorizedview.cpp.
void KCategorizedView::setCategorySpacing | ( | int | categorySpacing | ) |
Stablishes the category spacing.
This is the spacing between categories.
- Since
- 4.4
Definition at line 659 of file kcategorizedview.cpp.
void KCategorizedView::setCollapsibleBlocks | ( | bool | enable | ) |
Sets whether blocks can be collapsed or not.
- Since
- 4.4
Definition at line 688 of file kcategorizedview.cpp.
void KCategorizedView::setGridSize | ( | const QSize & | size | ) |
Calls to setGridSizeOwn().
Definition at line 540 of file kcategorizedview.cpp.
void KCategorizedView::setGridSizeOwn | ( | const QSize & | size | ) |
- Warning
- note that setGridSize is not virtual in the base class (QListView), so if you are calling to this method, make sure you have a KCategorizedView pointer around. This means that something like: lv->setGridSize(mySize);
will not call to the expected setGridSize method. Instead do something like this:
- Note
- this method will call to QListView::setGridSize among other operations.
- Since
- 4.4
Definition at line 545 of file kcategorizedview.cpp.
|
virtual |
Reimplemented from QAbstractItemView.
Definition at line 514 of file kcategorizedview.cpp.
|
protectedvirtual |
Reimplemented from QAbstractItemView.
Definition at line 888 of file kcategorizedview.cpp.
|
protectedvirtualslot |
Reposition items as needed.
Definition at line 1505 of file kcategorizedview.cpp.
|
protectedvirtual |
Reimplemented from QAbstractItemView.
Definition at line 1073 of file kcategorizedview.cpp.
|
protectedvirtual |
Reimplemented from QAbstractItemView.
Definition at line 1340 of file kcategorizedview.cpp.
|
virtual |
Reimplemented from QAbstractItemView.
Definition at line 551 of file kcategorizedview.cpp.
Property Documentation
|
readwrite |
Definition at line 84 of file kcategorizedview.h.
|
readwrite |
Definition at line 83 of file kcategorizedview.h.
|
readwrite |
Definition at line 85 of file kcategorizedview.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:49:17 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.