KDEUI
#include <kconfigdialogmanager.h>
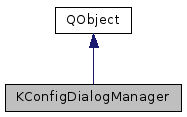
Public Slots | |
void | updateSettings () |
void | updateWidgets () |
void | updateWidgetsDefault () |
Signals | |
void | settingsChanged () |
void | settingsChanged (QWidget *widget) |
void | widgetModified () |
Public Member Functions | |
KConfigDialogManager (QWidget *parent, KCoreConfigSkeleton *conf) | |
KConfigDialogManager (QWidget *parent, KConfigSkeleton *conf) | |
~KConfigDialogManager () | |
void | addWidget (QWidget *widget) |
bool | hasChanged () const |
bool | isDefault () const |
Static Public Member Functions | |
static QHash< QString, QByteArray > * | changedMap () |
static QHash< QString, QByteArray > * | propertyMap () |
Protected Member Functions | |
QByteArray | getCustomProperty (const QWidget *widget) const |
QByteArray | getUserProperty (const QWidget *widget) const |
void | init (bool trackChanges) |
bool | parseChildren (const QWidget *widget, bool trackChanges) |
QVariant | property (QWidget *w) const |
void | setProperty (QWidget *w, const QVariant &v) |
void | setupWidget (QWidget *widget, KConfigSkeletonItem *item) |
Static Protected Member Functions | |
static void | initMaps () |
Detailed Description
Provides a means of automatically retrieving, saving and resetting KConfigSkeleton based settings in a dialog.
The KConfigDialogManager class provides a means of automatically retrieving, saving and resetting basic settings. It also can emit signals when settings have been changed (settings were saved) or modified (the user changes a checkbox from on to off).
The names of the widgets to be managed have to correspond to the names of the configuration entries in the KConfigSkeleton object plus an additional "kcfg_" prefix. For example a widget named "kcfg_MyOption" would be associated to the configuration entry "MyOption".
New widgets can be added to the map using the static functions propertyMap() and changedMap(). Note that you can't just add any class. The class must have a matching Q_PROPERTY(...) macro defined, and a signal which emitted when the property changed. Note: by default, the property which defined as "USER true" is used.
For example (note that KColorButton is already added and it doesn't need to manually added):
kcolorbutton.h defines the following property:
and signal:
To add KColorButton the following code would be inserted in the main:
If you want to use a widget's property that is not the USER property, you can define which property to use in the widget's kcfg_property:
Alternatively you can set the kcfg_property using designer.
Definition at line 85 of file kconfigdialogmanager.h.
Constructor & Destructor Documentation
KConfigDialogManager::KConfigDialogManager | ( | QWidget * | parent, |
KCoreConfigSkeleton * | conf | ||
) |
Constructor.
- Parameters
-
parent Dialog widget to manage conf Object that contains settings
Definition at line 69 of file kconfigdialogmanager.cpp.
KConfigDialogManager::KConfigDialogManager | ( | QWidget * | parent, |
KConfigSkeleton * | conf | ||
) |
Constructor.
- Parameters
-
parent Dialog widget to manage conf Object that contains settings
Definition at line 77 of file kconfigdialogmanager.cpp.
KConfigDialogManager::~KConfigDialogManager | ( | ) |
Destructor.
Definition at line 85 of file kconfigdialogmanager.cpp.
Member Function Documentation
void KConfigDialogManager::addWidget | ( | QWidget * | widget | ) |
Add additional widgets to manage.
- Parameters
-
widget Additional widget to manage, inlcuding all its children
Definition at line 177 of file kconfigdialogmanager.cpp.
Retrieve the widget change map.
Definition at line 162 of file kconfigdialogmanager.cpp.
|
protected |
Find the property to use for a widget by querying the kcfg_property property of the widget.
Like a widget can use a property other than the USER property.
- Since
- 4.3
Definition at line 436 of file kconfigdialogmanager.cpp.
|
protected |
Finds the USER property name using Qt's MetaProperty system, and caches it in the property map (the cache could be retrieved by propertyMap() ).
Definition at line 407 of file kconfigdialogmanager.cpp.
bool KConfigDialogManager::hasChanged | ( | ) | const |
Returns whether the current state of the known widgets are different from the state in the config object.
Definition at line 517 of file kconfigdialogmanager.cpp.
|
protected |
- Parameters
-
trackChanges - If any changes by the widgets should be tracked set true. This causes the emitting the modified() signal when something changes. TODO:
- Returns
- bool - True if any setting was changed from the default.
Definition at line 168 of file kconfigdialogmanager.cpp.
|
staticprotected |
Initializes the property maps.
Definition at line 90 of file kconfigdialogmanager.cpp.
bool KConfigDialogManager::isDefault | ( | ) | const |
Returns whether the current state of the known widgets are the same as the default state in the config object.
Definition at line 539 of file kconfigdialogmanager.cpp.
Recursive function that finds all known children.
Goes through the children of widget and if any are known and not being ignored, stores them in currentGroup. Also checks if the widget should be disabled because it is set immutable.
- Parameters
-
widget - Parent of the children to look at. trackChanges - If true then tracks any changes to the children of widget that are known.
- Returns
- bool - If a widget was set to something other than its default.
Definition at line 225 of file kconfigdialogmanager.cpp.
Retrieve a property.
Definition at line 489 of file kconfigdialogmanager.cpp.
Retrieve the property map.
Definition at line 156 of file kconfigdialogmanager.cpp.
Set a property.
Definition at line 450 of file kconfigdialogmanager.cpp.
|
signal |
One or more of the settings have been saved (such as when the user clicks on the Apply button).
This is only emitted by updateSettings() whenever one or more setting were changed and consequently saved.
|
signal |
TODO: Verify One or more of the settings have been changed.
- Parameters
-
widget - The widget group (pass in via addWidget()) that contains the one or more modified setting.
- See also
- settingsChanged()
|
protected |
Setup secondary widget properties.
Definition at line 182 of file kconfigdialogmanager.cpp.
|
slot |
Traverse the specified widgets, saving the settings of all known widgets in the settings object.
Example use: User clicks Ok or Apply button in a configure dialog.
Definition at line 378 of file kconfigdialogmanager.cpp.
|
slot |
Traverse the specified widgets, sets the state of all known widgets according to the state in the settings object.
Example use: Initialisation of dialog. Example use: User clicks Reset button in a configure dialog.
Definition at line 332 of file kconfigdialogmanager.cpp.
|
slot |
Traverse the specified widgets, sets the state of all known widgets according to the default state in the settings object.
Example use: User clicks Defaults button in a configure dialog.
Definition at line 371 of file kconfigdialogmanager.cpp.
|
signal |
If retrieveSettings() was told to track changes then if any known setting was changed this signal will be emitted.
Note that a settings can be modified several times and might go back to the original saved state. hasChanged() will tell you if anything has actually changed from the saved values.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:49:17 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.