KDEUI
#include <kfontdialog.h>
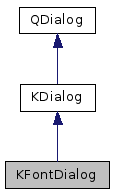
Signals | |
void | fontSelected (const QFont &font) |
![]() | |
void | aboutToShowDetails () |
void | applyClicked () |
void | buttonClicked (KDialog::ButtonCode button) |
void | cancelClicked () |
void | closeClicked () |
void | defaultClicked () |
void | finished () |
void | helpClicked () |
void | hidden () |
void | layoutHintChanged () |
void | noClicked () |
void | okClicked () |
void | resetClicked () |
void | tryClicked () |
void | user1Clicked () |
void | user2Clicked () |
void | user3Clicked () |
void | yesClicked () |
Public Member Functions | |
KFontDialog (QWidget *parent=0, const KFontChooser::DisplayFlags &flags=KFontChooser::NoDisplayFlags, const QStringList &fontlist=QStringList(), Qt::CheckState *sizeIsRelativeState=0) | |
~KFontDialog () | |
QFont | font () const |
void | setFont (const QFont &font, bool onlyFixed=false) |
void | setSizeIsRelative (Qt::CheckState relative) |
Qt::CheckState | sizeIsRelative () const |
![]() | |
KDialog (QWidget *parent=0, Qt::WindowFlags flags=0) | |
~KDialog () | |
KPushButton * | button (ButtonCode id) const |
KIcon | buttonIcon (ButtonCode id) const |
QString | buttonText (ButtonCode id) const |
QString | buttonToolTip (ButtonCode id) const |
QString | buttonWhatsThis (ButtonCode id) const |
ButtonCode | defaultButton () const |
QString | helpLinkText () const |
void | incrementInitialSize (const QSize &size) |
bool | isButtonEnabled (ButtonCode id) const |
QWidget * | mainWidget () |
virtual QSize | minimumSizeHint () const |
void | restoreDialogSize (const KConfigGroup &config) |
void | saveDialogSize (KConfigGroup &config, KConfigGroup::WriteConfigFlags options=KConfigGroup::Normal) const |
void | setButtonFocus (ButtonCode id) |
void | setButtonGuiItem (ButtonCode id, const KGuiItem &item) |
void | setButtonIcon (ButtonCode id, const KIcon &icon) |
void | setButtonMenu (ButtonCode id, QMenu *menu, ButtonPopupMode popupmode=InstantPopup) |
void | setButtons (ButtonCodes buttonMask) |
void | setButtonsOrientation (Qt::Orientation orientation) |
void | setButtonText (ButtonCode id, const QString &text) |
void | setButtonToolTip (ButtonCode id, const QString &text) |
void | setButtonWhatsThis (ButtonCode id, const QString &text) |
void | setDefaultButton (ButtonCode id) |
void | setEscapeButton (ButtonCode id) |
void | setInitialSize (const QSize &size) |
void | setMainWidget (QWidget *widget) |
void | showButton (ButtonCode id, bool state) |
void | showButtonSeparator (bool state) |
virtual QSize | sizeHint () const |
Static Public Member Functions | |
static int | getFont (QFont &theFont, const KFontChooser::DisplayFlags &flags=KFontChooser::NoDisplayFlags, QWidget *parent=0L, Qt::CheckState *sizeIsRelativeState=0L) |
static int | getFontAndText (QFont &theFont, QString &theString, const KFontChooser::DisplayFlags &flags=KFontChooser::NoDisplayFlags, QWidget *parent=0L, Qt::CheckState *sizeIsRelativeState=0L) |
static int | getFontDiff (QFont &theFont, KFontChooser::FontDiffFlags &diffFlags, const KFontChooser::DisplayFlags &flags=KFontChooser::NoDisplayFlags, QWidget *parent=0L, Qt::CheckState *sizeIsRelativeState=0L) |
![]() | |
static bool | avoidArea (QWidget *widget, const QRect &area, int screen=-1) |
static void | centerOnScreen (QWidget *widget, int screen=-1) |
static int | groupSpacingHint () |
static QString | makeStandardCaption (const QString &userCaption, QWidget *window=0, CaptionFlags flags=HIGCompliantCaption) |
static int | marginHint () |
static void | resizeLayout (QWidget *widget, int margin, int spacing) |
static void | resizeLayout (QLayout *lay, int margin, int spacing) |
static void | setAllowEmbeddingInGraphicsView (bool allowEmbedding) |
static int | spacingHint () |
Additional Inherited Members | |
![]() | |
enum | ButtonCode { None = 0x00000000, Help = 0x00000001, Default = 0x00000002, Ok = 0x00000004, Apply = 0x00000008, Try = 0x00000010, Cancel = 0x00000020, Close = 0x00000040, No = 0x00000080, Yes = 0x00000100, Reset = 0x00000200, Details = 0x00000400, User1 = 0x00001000, User2 = 0x00002000, User3 = 0x00004000, NoDefault = 0x00008000 } |
enum | ButtonPopupMode { InstantPopup = 0, DelayedPopup = 1 } |
enum | CaptionFlag { NoCaptionFlags = 0, AppNameCaption = 1, ModifiedCaption = 2, HIGCompliantCaption = AppNameCaption } |
![]() | |
void | delayedDestruct () |
void | enableButton (ButtonCode id, bool state) |
void | enableButtonApply (bool state) |
void | enableButtonCancel (bool state) |
void | enableButtonOk (bool state) |
void | enableLinkedHelp (bool state) |
bool | isDetailsWidgetVisible () const |
virtual void | setCaption (const QString &caption) |
virtual void | setCaption (const QString &caption, bool modified) |
void | setDetailsWidget (QWidget *detailsWidget) |
void | setDetailsWidgetVisible (bool visible) |
void | setHelp (const QString &anchor, const QString &appname=QString()) |
void | setHelpLinkText (const QString &text) |
virtual void | setPlainCaption (const QString &caption) |
![]() | |
virtual void | slotButtonClicked (int button) |
void | updateGeometry () |
![]() | |
KDialog (KDialogPrivate &dd, QWidget *parent, Qt::WindowFlags flags=0) | |
virtual void | closeEvent (QCloseEvent *e) |
virtual void | hideEvent (QHideEvent *) |
virtual void | keyPressEvent (QKeyEvent *) |
![]() | |
KDialogPrivate *const | d_ptr |
Detailed Description
A font selection dialog.
The KFontDialog provides a dialog for interactive font selection. It is basically a thin wrapper around the KFontChooser widget, which can also be used standalone. In most cases, the simplest use of this class is the static method KFontDialog::getFont(), which pops up the dialog, allows the user to select a font, and returns when the dialog is closed.
Example:
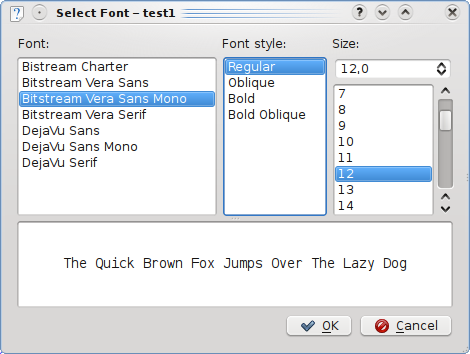
Definition at line 56 of file kfontdialog.h.
Constructor & Destructor Documentation
|
explicit |
Constructs a font selection dialog.
- Parameters
-
parent The parent widget of the dialog, if any. flags Defines how the font chooser is displayed.
- See also
- KFontChooser::DisplayFlags
- Parameters
-
fontlist a list of fonts to display, in XLFD format. If no list is formatted, the internal KDE font list is used. If that has not been created, X is queried, and all fonts available on the system are displayed. sizeIsRelativeState If not zero the widget will show a checkbox where the user may choose whether the font size is to be interpreted as relative size. Initial state of this checkbox will be set according to *sizeIsRelativeState, user choice may be retrieved by calling sizeIsRelative().
Definition at line 68 of file kfontdialog.cpp.
KFontDialog::~KFontDialog | ( | ) |
Definition at line 87 of file kfontdialog.cpp.
Member Function Documentation
QFont KFontDialog::font | ( | ) | const |
- Returns
- The currently selected font in the dialog.
Definition at line 97 of file kfontdialog.cpp.
|
signal |
Emitted whenever the currently selected font changes.
Connect to this to monitor the font as it is selected if you are not running modal.
|
static |
Creates a modal font dialog, lets the user choose a font, and returns when the dialog is closed.
- Parameters
-
theFont a reference to the font to write the chosen font into. flags Defines how the font chooser is displayed.
- See also
- KFontChooser::DisplayFlags
- Parameters
-
parent Parent widget of the dialog. Specifying a widget different from 0 (Null) improves centering (looks better). makeFrame Draws a frame with titles around the contents. sizeIsRelativeState If not zero the widget will show a checkbox where the user may choose whether the font size is to be interpreted as relative size. Initial state of this checkbox will be set according to *sizeIsRelativeState and user choice will be returned therein.
- Returns
- QDialog::result().
Definition at line 136 of file kfontdialog.cpp.
|
static |
When you are not only interested in the font selected, but also in the example string typed in, you can call this method.
- Parameters
-
theFont a reference to the font to write the chosen font into. theString a reference to the example text that was typed. flags Defines how the font chooser is displayed.
- See also
- KFontChooser::DisplayFlags
- Parameters
-
parent Parent widget of the dialog. Specifying a widget different from 0 (Null) improves centering (looks better). sizeIsRelativeState If not zero the widget will show a checkbox where the user may choose whether the font size is to be interpreted as relative size. Initial state of this checkbox will be set according to *sizeIsRelativeState and user choice will be returned therein.
- Returns
- The result of the dialog.
Definition at line 157 of file kfontdialog.cpp.
|
static |
Creates a modal font difference dialog, lets the user choose a selection of changes that should be made to a set of fonts, and returns when the dialog is closed.
Useful for choosing slight adjustments to the font set when the user would otherwise have to manually edit a number of fonts.
- Parameters
-
theFont a reference to the font to write the chosen font into. flags Defines how the font chooser is displayed.
- See also
- KFontChooser::DisplayFlags
- Parameters
-
diffFlags a reference to the int into which the chosen difference selection bitmask should be written. Check the returned bitmask like: if ( diffFlags & KFontChooser::FontDiffFamily )[...]if ( diffFlags & KFontChooser::FontDiffStyle )[...]if ( diffFlags & KFontChooser::FontDiffSize )[...]parent Parent widget of the dialog. Specifying a widget different from 0 (Null) improves centering (looks better). sizeIsRelativeState If not zero the widget will show a checkbox where the user may choose whether the font size is to be interpreted as relative size. Initial state of this checkbox will be set according to *sizeIsRelativeState and user choice will be returned therein.
- Returns
- QDialog::result().
Definition at line 113 of file kfontdialog.cpp.
Sets the currently selected font in the dialog.
- Parameters
-
font The font to select. onlyFixed readjust the font list to display only fixed width fonts if true, or vice-versa
Definition at line 92 of file kfontdialog.cpp.
void KFontDialog::setSizeIsRelative | ( | Qt::CheckState | relative | ) |
Sets the state of the checkbox indicating whether the font size is to be interpreted as relative size.
NOTE: If parameter sizeIsRelative was not set in the constructor of the dialog this setting will be ignored.
Definition at line 102 of file kfontdialog.cpp.
Qt::CheckState KFontDialog::sizeIsRelative | ( | ) | const |
- Returns
- Whether the font size is to be interpreted as relative size (default: false)
Definition at line 107 of file kfontdialog.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:49:17 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.