KDEUI
#include <khistorycombobox.h>
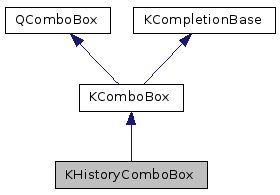
Public Slots | |
void | addToHistory (const QString &item) |
void | clearHistory () |
![]() | |
void | rotateText (KCompletionBase::KeyBindingType type) |
void | setCompletedItems (const QStringList &items, bool autosubject=true) |
virtual void | setCompletedText (const QString &) |
void | setCurrentItem (const QString &item, bool insert=false, int index=-1) |
Signals | |
void | cleared () |
![]() | |
void | aboutToShowContextMenu (QMenu *p) |
void | completion (const QString &) |
void | completionModeChanged (KGlobalSettings::Completion) |
void | returnPressed () |
void | returnPressed (const QString &) |
void | substringCompletion (const QString &) |
void | textRotation (KCompletionBase::KeyBindingType) |
Public Member Functions | |
KHistoryComboBox (QWidget *parent=0) | |
KHistoryComboBox (bool useCompletion, QWidget *parent=0) | |
~KHistoryComboBox () | |
QStringList | historyItems () const |
KPixmapProvider * | pixmapProvider () const |
bool | removeFromHistory (const QString &item) |
void | reset () |
void | setHistoryItems (const QStringList &items) |
void | setHistoryItems (const QStringList &items, bool setCompletionList) |
void | setPixmapProvider (KPixmapProvider *prov) |
![]() | |
KComboBox (QWidget *parent=0) | |
KComboBox (bool rw, QWidget *parent=0) | |
virtual | ~KComboBox () |
void | addUrl (const KUrl &url) |
void | addUrl (const QIcon &icon, const KUrl &url) |
bool | autoCompletion () const |
void | changeURL (const KUrl &url, int index) |
void | changeURL (const QPixmap &pixmap, const KUrl &url, int index) |
void | changeUrl (int index, const KUrl &url) |
void | changeUrl (int index, const QIcon &icon, const KUrl &url) |
KCompletionBox * | completionBox (bool create=true) |
bool | contains (const QString &text) const |
int | cursorPosition () const |
virtual bool | eventFilter (QObject *, QEvent *) |
void | insertURL (const KUrl &url, int index=-1) |
void | insertURL (const QPixmap &pixmap, const KUrl &url, int index=-1) |
void | insertUrl (int index, const KUrl &url) |
void | insertUrl (int index, const QIcon &icon, const KUrl &url) |
virtual void | setAutoCompletion (bool autocomplete) |
virtual void | setContextMenuEnabled (bool showMenu) |
void | setEditable (bool editable) |
void | setEditUrl (const KUrl &url) |
virtual void | setLineEdit (QLineEdit *) |
void | setTrapReturnKey (bool trap) |
void | setUrlDropsEnabled (bool enable) |
bool | trapReturnKey () const |
bool | urlDropsEnabled () const |
![]() | |
KCompletionBase () | |
virtual | ~KCompletionBase () |
KGlobalSettings::Completion | completionMode () const |
KCompletion * | completionObject (bool hsig=true) |
KCompletion * | compObj () const |
bool | emitSignals () const |
KShortcut | getKeyBinding (KeyBindingType item) const |
bool | handleSignals () const |
bool | isCompletionObjectAutoDeleted () const |
void | setAutoDeleteCompletionObject (bool autoDelete) |
virtual void | setCompletedItems (const QStringList &items, bool autoSuggest=true)=0 |
virtual void | setCompletedText (const QString &text)=0 |
virtual void | setCompletionMode (KGlobalSettings::Completion mode) |
virtual void | setCompletionObject (KCompletion *compObj, bool hsig=true) |
void | setEnableSignals (bool enable) |
virtual void | setHandleSignals (bool handle) |
bool | setKeyBinding (KeyBindingType item, const KShortcut &key) |
void | useGlobalKeyBindings () |
Protected Member Functions | |
void | insertItems (const QStringList &items) |
virtual void | keyPressEvent (QKeyEvent *) |
bool | useCompletion () const |
virtual void | wheelEvent (QWheelEvent *ev) |
![]() | |
virtual void | create (WId=0, bool initializeWindow=true, bool destroyOldWindow=true) |
virtual QSize | minimumSizeHint () const |
virtual void | setCompletedText (const QString &, bool) |
![]() | |
KCompletionBase * | delegate () const |
KeyBindingMap | getKeyBindings () const |
void | setDelegate (KCompletionBase *delegate) |
virtual void | virtual_hook (int id, void *data) |
Properties | |
QStringList | historyItems |
![]() | |
bool | autoCompletion |
bool | trapReturnKey |
bool | urlDropsEnabled |
Additional Inherited Members | |
![]() | |
typedef QMap< KeyBindingType, KShortcut > | KeyBindingMap |
enum | KeyBindingType { TextCompletion, PrevCompletionMatch, NextCompletionMatch, SubstringCompletion } |
![]() | |
virtual void | makeCompletion (const QString &) |
Detailed Description
A combobox for offering a history and completion.
A combobox which implements a history like a unix shell. You can navigate through all the items by using the Up or Down arrows (configurable of course). Additionally, weighted completion is available. So you should load and save the completion list to preserve the weighting between sessions.
KHistoryComboBox obeys the HISTCONTROL environment variable to determine whether duplicates in the history should be tolerated in addToHistory() or not. During construction of KHistoryComboBox, duplicates will be disabled when HISTCONTROL is set to "ignoredups" or "ignoreboth". Otherwise, duplicates are enabled by default.
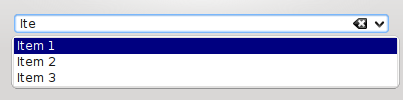
Definition at line 48 of file khistorycombobox.h.
Constructor & Destructor Documentation
|
explicit |
Constructs a "read-write" combobox.
A read-only history combobox doesn't make much sense, so it is only available as read-write. Completion will be used automatically for the items in the combo.
The insertion-policy is set to NoInsert, you have to add the items yourself via the slot addToHistory. If you want every item added, use
Use QComboBox::setMaxCount() to limit the history.
parent
the parent object of this widget.
Definition at line 66 of file khistorycombobox.cpp.
Same as the previous constructor, but additionally has the option to specify whether you want to let KHistoryComboBox handle completion or not.
If set to true
, KHistoryComboBox will sync the completion to the contents of the combobox.
Definition at line 73 of file khistorycombobox.cpp.
KHistoryComboBox::~KHistoryComboBox | ( | ) |
Destructs the combo, the completion-object and the pixmap-provider.
Definition at line 107 of file khistorycombobox.cpp.
Member Function Documentation
|
slot |
Adds an item to the end of the history list and to the completion list.
If maxCount() is reached, the first item of the list will be removed.
If the last inserted item is the same as item
, it will not be inserted again.
If duplicatesEnabled() is false, any equal existing item will be removed before item
is added.
Note: By using this method and not the Q and KComboBox insertItem() methods, you make sure that the combobox stays in sync with the completion. It would be annoying if completion would give an item not in the combobox, and vice versa.
- See also
- removeFromHistory
- QComboBox::setDuplicatesEnabled
Definition at line 183 of file khistorycombobox.cpp.
|
signal |
Emitted when the history was cleared by the entry in the popup menu.
|
slot |
Clears the history and the completion list.
Definition at line 163 of file khistorycombobox.cpp.
QStringList KHistoryComboBox::historyItems | ( | ) | const |
Returns the list of history items.
Empty, when this is not a read-write combobox.
- See also
- setHistoryItems
|
protected |
Inserts items
into the combo, honoring pixmapProvider() Does not update the completionObject.
Note: duplicatesEnabled() is not honored here.
Called from setHistoryItems() and setPixmapProvider()
Definition at line 395 of file khistorycombobox.cpp.
|
protectedvirtual |
Handling key-events, the shortcuts to rotate the items.
Definition at line 340 of file khistorycombobox.cpp.
KPixmapProvider * KHistoryComboBox::pixmapProvider | ( | ) | const |
- Returns
- the current pixmap provider.
- See also
- setPixmapProvider
- KPixmapProvider
Definition at line 438 of file khistorycombobox.cpp.
Removes all items named item
.
- Returns
true
if at least one item was removed.
- See also
- addToHistory
Definition at line 235 of file khistorycombobox.cpp.
void KHistoryComboBox::reset | ( | ) |
Resets the current position of the up/down history.
Call this when you manually call setCurrentItem() or clearEdit().
Definition at line 443 of file khistorycombobox.cpp.
void KHistoryComboBox::setHistoryItems | ( | const QStringList & | items | ) |
Inserts items
into the combobox.
items
might get truncated if it is longer than maxCount()
- See also
- historyItems
Definition at line 113 of file khistorycombobox.cpp.
void KHistoryComboBox::setHistoryItems | ( | const QStringList & | items, |
bool | setCompletionList | ||
) |
Inserts items
into the combobox.
items
might get truncated if it is longer than maxCount()
Set setCompletionList
to true, if you don't have a list of completions. This tells KHistoryComboBox to use all the items for the completion object as well. You won't have the benefit of weighted completion though, so normally you should do something like
Be sure to use different names for saving with KConfig if you have more than one KHistoryComboBox.
Note: When setCompletionList
is true, the items are inserted into the KCompletion object with mode KCompletion::Insertion and the mode is set to KCompletion::Weighted afterwards.
Definition at line 118 of file khistorycombobox.cpp.
void KHistoryComboBox::setPixmapProvider | ( | KPixmapProvider * | prov | ) |
Sets a pixmap provider, so that items in the combobox can have a pixmap.
KPixmapProvider is just an abstract class with the one pure virtual method KPixmapProvider::pixmapFor(). This method is called whenever an item is added to the KHistoryComboBoxBox. Implement it to return your own custom pixmaps, or use the KUrlPixmapProvider from libkio, which uses KMimeType::pixmapForUrl to resolve icons.
Set prov
to 0L if you want to disable pixmaps. Default no pixmaps.
- See also
- pixmapProvider
Definition at line 377 of file khistorycombobox.cpp.
|
protected |
- Returns
- if we can modify the completion object or not.
Definition at line 158 of file khistorycombobox.cpp.
|
protectedvirtual |
Handling wheel-events, to rotate the items.
Reimplemented from KComboBox.
Definition at line 352 of file khistorycombobox.cpp.
Property Documentation
|
readwrite |
Definition at line 51 of file khistorycombobox.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:49:17 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.