KDEUI
#include <kiconloader.h>
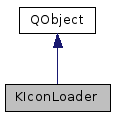
Public Types | |
enum | Context { Any, Action, Application, Device, FileSystem, MimeType, Animation, Category, Emblem, Emote, International, Place, StatusIcon } |
enum | Group { NoGroup =-1, Desktop =0, FirstGroup =0, Toolbar, MainToolbar, Small, Panel, Dialog, LastGroup, User } |
enum | MatchType { MatchExact, MatchBest } |
enum | States { DefaultState, ActiveState, DisabledState, LastState } |
enum | StdSizes { SizeSmall =16, SizeSmallMedium =22, SizeMedium =32, SizeLarge =48, SizeHuge =64, SizeEnormous =128 } |
enum | Type { Fixed, Scalable, Threshold } |
Public Slots | |
void | newIconLoader () |
Signals | |
void | iconLoaderSettingsChanged () |
Static Public Member Functions | |
static KIconLoader * | global () |
static QPixmap | unknown () |
Related Functions | |
(Note that these are not member functions.) | |
QPixmap | BarIcon (const QString &name, int size=0, int state=KIconLoader::DefaultState, const QStringList &overlays=QStringList()) |
QIcon | BarIconSet (const QString &name, int size=0) |
QPixmap | DesktopIcon (const QString &name, int size=0, int state=KIconLoader::DefaultState, const QStringList &overlays=QStringList()) |
QIcon | DesktopIconSet (const QString &name, int size=0) |
int | IconSize (KIconLoader::Group group) |
QPixmap | MainBarIcon (const QString &name, int size=0, int state=KIconLoader::DefaultState, const QStringList &overlays=QStringList()) |
QIcon | MainBarIconSet (const QString &name, int size=0) |
QPixmap | SmallIcon (const QString &name, int size=0, int state=KIconLoader::DefaultState, const QStringList &overlays=QStringList()) |
QIcon | SmallIconSet (const QString &name, int size=0) |
QPixmap | UserIcon (const QString &name, int state=KIconLoader::DefaultState, const QStringList &overlays=QStringList()) |
QIcon | UserIconSet (const QString &name) |
Detailed Description
Iconloader for KDE.
KIconLoader will load the current icon theme and all its base themes. Icons will be searched in any of these themes. Additionally, it caches icons and applies effects according to the user's preferences.
In KDE, it is encouraged to load icons by "Group". An icon group is a location on the screen where icons are being used. Standard groups are: Desktop, Toolbar, MainToolbar, Small and Panel. Each group has some centrally configured properties bound to it, including the icon size and effects. This makes it possible to offer a consistent icon look in all KDE applications.
The standard groups are defined below.
- KIconLoader::Desktop: Icons in the iconview of konqueror, kdesktop and similar apps.
- KIconLoader::Toolbar: Icons in toolbars.
- KIconLoader::MainToolbar: Icons in the main toolbars.
- KIconLoader::Small: Various small (typical 16x16) places: titlebars, listviews and menu entries.
- KIconLoader::Panel: Icons in kicker's panel
The icons are stored on disk in an icon theme or in a standalone directory. The icon theme directories contain multiple sizes and/or depths for the same icon. The iconloader will load the correct one based on the icon group and the current theme. Icon themes are stored globally in share/icons, or, application specific in share/apps/$appdir/icons.
The standalone directories contain just one version of an icon. The directories that are searched are: $appdir/pics and $appdir/toolbar. Icons in these directories can be loaded by using the special group "User".
Definition at line 77 of file kiconloader.h.
Member Enumeration Documentation
enum KIconLoader::Context |
Defines the context of the icon.
Enumerator | |
---|---|
Any |
Some icon with unknown purpose. |
Action |
An action icon (e.g. 'save', 'print'). |
Application |
An icon that represents an application. |
Device |
An icon that represents a device. |
FileSystem |
An icon that represents a file system.
|
MimeType |
An icon that represents a mime type (or file type). |
Animation |
An icon that is animated. |
Category |
An icon that represents a category. |
Emblem |
An icon that adds information to an existing icon. |
Emote |
An icon that expresses an emotion. |
International |
An icon that represents a country's flag. |
Place |
An icon that represents a location (e.g. 'home', 'trash'). |
StatusIcon |
An icon that represents an event. |
Definition at line 91 of file kiconloader.h.
enum KIconLoader::Group |
The group of the icon.
Definition at line 127 of file kiconloader.h.
The type of a match.
Enumerator | |
---|---|
MatchExact |
Only try to find an exact match. |
MatchBest |
Take the best match if there is no exact match. |
Definition at line 119 of file kiconloader.h.
enum KIconLoader::States |
Defines the possible states of an icon.
Enumerator | |
---|---|
DefaultState |
The default state. |
ActiveState |
Icon is active. |
DisabledState |
Icon is disabled. |
LastState |
Last state (last constant) |
Definition at line 171 of file kiconloader.h.
These are the standard sizes for icons.
Definition at line 153 of file kiconloader.h.
enum KIconLoader::Type |
The type of the icon.
Enumerator | |
---|---|
Fixed |
Fixed-size icon. |
Scalable |
Scalable-size icon. |
Threshold |
A threshold icon. |
Definition at line 110 of file kiconloader.h.
Constructor & Destructor Documentation
|
explicit |
Constructs an iconloader.
- Parameters
-
appname Add the data directories of this application to the icon search path for the "User" group. The default argument adds the directories of the current application. dirs the KStandardDirs object to use. If null the global one is used
Usually, you use the default iconloader, which can be accessed via KIconLoader::global(), so you hardly ever have to create an iconloader object yourself. That one is the current KComponentData's (typically KApplication's) iconloader.
Definition at line 424 of file kiconloader.cpp.
|
explicit |
Constructs an iconloader.
- Parameters
-
componentData the KComponentData to use to create this icon loader.
Usually, you use the default iconloader, which can be accessed via KIconLoader::global(), so you hardly ever have to create an iconloader object yourself. That one is the current KComponentData's (typically KApplication's) iconloader.
Definition at line 435 of file kiconloader.cpp.
KIconLoader::~KIconLoader | ( | ) |
Cleanup.
Definition at line 556 of file kiconloader.cpp.
Member Function Documentation
void KIconLoader::addAppDir | ( | const QString & | appname | ) |
Adds appname
to the list of application specific directories.
- Parameters
-
appname The application name.
Definition at line 561 of file kiconloader.cpp.
void KIconLoader::addExtraDesktopThemes | ( | ) |
Adds all the default themes from other desktops at the end of the list of icon themes.
Definition at line 644 of file kiconloader.cpp.
bool KIconLoader::alphaBlending | ( | KIconLoader::Group | group | ) | const |
Checks whether the user wants to blend the icons with the background using the alpha channel information for a given group.
- Parameters
-
group the group to check
- Returns
- true if alpha blending is desired
Definition at line 1492 of file kiconloader.cpp.
int KIconLoader::currentSize | ( | KIconLoader::Group | group | ) | const |
Returns the current size of the icon group.
Using e.g. KIconLoader::SmallIcon will retrieve the icon size that is currently set from System Settings->Appearance->Icon sizes. SmallIcon for instance, would typically be 16x16, but the user could increase it and this setting would change as well.
- Parameters
-
group the group to check.
- Returns
- the current size for an icon group.
Definition at line 1370 of file kiconloader.cpp.
void KIconLoader::drawOverlays | ( | const QStringList & | overlays, |
QPixmap & | pixmap, | ||
KIconLoader::Group | group, | ||
int | state = KIconLoader::DefaultState |
||
) | const |
Draws overlays on the specified pixmap, it takes the width and height of the pixmap into consideration.
- Parameters
-
overlays to draw pixmap to draw on
- Since
- 4.7
Definition at line 699 of file kiconloader.cpp.
bool KIconLoader::extraDesktopThemesAdded | ( | ) | const |
Returns if the default icon themes of other desktops have been added to the list of icon themes where icons are searched.
Definition at line 694 of file kiconloader.cpp.
|
static |
Returns the global icon loader initialized with the global KComponentData.
- Returns
- global icon loader
bool KIconLoader::hasContext | ( | KIconLoader::Context | context | ) | const |
Definition at line 1477 of file kiconloader.cpp.
KIconEffect * KIconLoader::iconEffect | ( | ) | const |
Returns a pointer to the KIconEffect object used by the icon loader.
- Returns
- the KIconEffect.
Definition at line 1487 of file kiconloader.cpp.
|
signal |
Emitted by newIconLoader once the new settings have been loaded.
QString KIconLoader::iconPath | ( | const QString & | name, |
int | group_or_size, | ||
bool | canReturnNull = false |
||
) | const |
Returns the path of an icon.
- Parameters
-
name The name of the icon, without extension. If an absolute path is supplied for this parameter, iconPath will return it directly. group_or_size If positive, search icons whose size is specified by the icon group group_or_size
. If negative, search icons whose size is -group_or_size
. See KIconLoader::Group and KIconLoader::StdSizescanReturnNull Can return a null string? If not, a path to the "unknown" icon will be returned.
- Returns
- the path of an icon, can be null or the "unknown" icon when not found, depending on
canReturnNull
.
Definition at line 1009 of file kiconloader.cpp.
QStringList KIconLoader::loadAnimated | ( | const QString & | name, |
KIconLoader::Group | group, | ||
int | size = 0 |
||
) | const |
Loads an animated icon as a series of still frames.
If you want to load a .mng animation as QMovie instead, please use loadMovie() instead.
- Parameters
-
name The name of the icon. group The icon group. See loadIcon(). size Override the default size for group
. See KIconLoader::StdSizes.
- Returns
- A QStringList containing the absolute path of all the frames making up the animation.
Definition at line 1310 of file kiconloader.cpp.
QPixmap KIconLoader::loadIcon | ( | const QString & | name, |
KIconLoader::Group | group, | ||
int | size = 0 , |
||
int | state = KIconLoader::DefaultState , |
||
const QStringList & | overlays = QStringList() , |
||
QString * | path_store = 0L , |
||
bool | canReturnNull = false |
||
) | const |
Loads an icon.
It will try very hard to find an icon which is suitable. If no exact match is found, a close match is searched. If neither an exact nor a close match is found, a null pixmap or the "unknown" pixmap is returned, depending on the value of the canReturnNull
parameter.
- Parameters
-
name The name of the icon, without extension. group The icon group. This will specify the size of and effects to be applied to the icon. size If nonzero, this overrides the size specified by group
. See KIconLoader::StdSizes.state The icon state: DefaultState
,ActiveState
orDisabledState
. Depending on the user's preferences, the iconloader may apply a visual effect to hint about its state.overlays a list of emblem icons to overlay, by name. the emblem prefix is applied automatically to each name, e.g. "zip" becomes "emblem-zip" path_store If not null, the path of the icon is stored here, if the icon was found. If the icon was not found path_store
is unaltered even if the "unknown" pixmap was returned.canReturnNull Can return a null pixmap? If false, the "unknown" pixmap is returned when no appropriate icon has been found. Note: a null pixmap can still be returned in the event of invalid parameters, such as empty names, negative sizes, and etc.
- Returns
- the QPixmap. Can be null when not found, depending on
canReturnNull
.
Definition at line 1100 of file kiconloader.cpp.
QIcon KIconLoader::loadIconSet | ( | const QString & | name, |
KIconLoader::Group | group, | ||
int | size = 0 , |
||
bool | canReturnNull = false |
||
) |
Creates an icon set, that will do on-demand loading of the icon.
Loading itself is done by calling loadIcon .
- Parameters
-
name The name of the icon, without extension. group The icon group. This will specify the size of and effects to be applied to the icon. size If nonzero, this overrides the size specified by group
. See KIconLoader::StdSizes.canReturnNull Can return a null iconset? If false, iconset containing the "unknown" pixmap is returned when no appropriate icon has been found.
- Returns
- the icon set. Can be null when not found, depending on
canReturnNull
.
- Deprecated:
- use KIcon instead, which uses the iconloader internally
Definition at line 1506 of file kiconloader.cpp.
QPixmap KIconLoader::loadMimeTypeIcon | ( | const QString & | iconName, |
KIconLoader::Group | group, | ||
int | size = 0 , |
||
int | state = KIconLoader::DefaultState , |
||
const QStringList & | overlays = QStringList() , |
||
QString * | path_store = 0 |
||
) | const |
Loads an icon for a mimetype.
This is basically like loadIcon except that extra desktop themes are loaded if necessary.
- Parameters
-
iconName The name of the icon, without extension, usually from KMimeType. group The icon group. This will specify the size of and effects to be applied to the icon. size If nonzero, this overrides the size specified by group
. See KIconLoader::StdSizes.state The icon state: DefaultState
,ActiveState
orDisabledState
. Depending on the user's preferences, the iconloader may apply a visual effect to hint about its state.path_store If not null, the path of the icon is stored here. overlays a list of emblem icons to overlay, by name. the emblem prefix is applied automatically to each name, e.g. "zip" becomes "emblem-zip"
- Returns
- the QPixmap. Can not be null, the "unknown" pixmap is returned when no appropriate icon has been found.
Definition at line 1075 of file kiconloader.cpp.
QMovie * KIconLoader::loadMovie | ( | const QString & | name, |
KIconLoader::Group | group, | ||
int | size = 0 , |
||
QObject * | parent = 0 |
||
) | const |
Loads an animated icon.
- Parameters
-
name The name of the icon. group The icon group. See loadIcon(). size Override the default size for group
. See KIconLoader::StdSizes.parent The parent object of the returned QMovie.
- Returns
- A QMovie object. Can be null if not found or not valid. Ownership is passed to the caller.
Definition at line 1240 of file kiconloader.cpp.
QString KIconLoader::moviePath | ( | const QString & | name, |
KIconLoader::Group | group, | ||
int | size = 0 |
||
) | const |
Returns the path to an animated icon.
- Parameters
-
name The name of the icon. group The icon group. See loadIcon(). size Override the default size for group
. See KIconLoader::StdSizes.
- Returns
- the full path to the movie, ready to be passed to QMovie's constructor. Empty string if not found.
Definition at line 1258 of file kiconloader.cpp.
|
slot |
Re-initialize the global icon loader.
Definition at line 1632 of file kiconloader.cpp.
QStringList KIconLoader::queryIcons | ( | int | group_or_size, |
KIconLoader::Context | context = KIconLoader::Any |
||
) | const |
Queries all available icons for a specific group, having a specific context.
- Parameters
-
group_or_size If positive, search icons whose size is specified by the icon group group_or_size
. If negative, search icons whose size is -group_or_size
. See KIconLoader::Group and KIconLoader::StdSizescontext The icon context.
- Returns
- a list of all icons
Definition at line 1436 of file kiconloader.cpp.
QStringList KIconLoader::queryIconsByContext | ( | int | group_or_size, |
KIconLoader::Context | context = KIconLoader::Any |
||
) | const |
Queries all available icons for a specific context.
- Parameters
-
group_or_size The icon preferred group or size. If available at this group or size, those icons will be returned, in other case, icons of undefined size will be returned. Positive numbers are groups, negative numbers are negated sizes. See KIconLoader::Group and KIconLoader::StdSizes context The icon context.
- Returns
- A QStringList containing the icon names available for that context
Definition at line 1394 of file kiconloader.cpp.
QStringList KIconLoader::queryIconsByDir | ( | const QString & | iconsDir | ) | const |
Returns a list of all icons (*.png or *.xpm extension) in the given directory.
- Parameters
-
iconsDir the directory to search in
- Returns
- A QStringList containing the icon paths
Definition at line 1382 of file kiconloader.cpp.
void KIconLoader::reconfigure | ( | const QString & | _appname, |
KStandardDirs * | _dirs | ||
) |
Called by KComponentData::newIconLoader to reconfigure the icon loader.
- Parameters
-
_appname the new application name _dirs the new standard directories. If 0, the directories from KGlobal will be taken.
Definition at line 446 of file kiconloader.cpp.
KIconTheme * KIconLoader::theme | ( | ) | const |
Returns a pointer to the current theme.
Can be used to query available and default sizes for groups.
- Note
- The KIconTheme will change if reconfigure() is called and therefore it's not recommended to store the pointer anywhere.
- Returns
- a pointer to the current theme. 0 if no theme set.
Definition at line 1363 of file kiconloader.cpp.
|
static |
Returns the unknown icon.
An icon that is used when no other icon can be found.
- Returns
- the unknown pixmap
Definition at line 1604 of file kiconloader.cpp.
Friends And Related Function Documentation
|
related |
Load a toolbar icon.
Definition at line 1538 of file kiconloader.cpp.
|
related |
Load a toolbar icon, and apply the necessary effects to get an IconSet.
- Deprecated:
- use KIcon(name) or KIcon(name,componentData.iconLoader()) instead
Definition at line 1546 of file kiconloader.cpp.
|
related |
Load a desktop icon.
Definition at line 1523 of file kiconloader.cpp.
|
related |
Load a desktop icon, and apply the necessary effects to get an IconSet.
- Deprecated:
- use KIcon(name) or KIcon(name,componentData.iconLoader()) instead
Definition at line 1531 of file kiconloader.cpp.
|
related |
Returns the current icon size for a specific group.
Definition at line 1598 of file kiconloader.cpp.
|
related |
Load a main toolbar icon.
Definition at line 1568 of file kiconloader.cpp.
|
related |
Load a main toolbar icon, and apply the effects to get an IconSet.
- Deprecated:
- use KIcon(name) or KIcon(name,componentData.iconLoader()) instead
Definition at line 1576 of file kiconloader.cpp.
|
related |
Load a small icon.
Definition at line 1553 of file kiconloader.cpp.
|
related |
Load a small icon, and apply the necessary effects to get an IconSet.
- Deprecated:
- use KIcon(name) or KIcon(name,componentData.iconLoader()) instead
Definition at line 1561 of file kiconloader.cpp.
|
related |
Load a user icon. User icons are searched in $appdir/pics.
Definition at line 1583 of file kiconloader.cpp.
|
related |
Load a user icon, and apply the effects to get an IconSet.
- Deprecated:
- use KIcon(name) or KIcon(name,componentData.iconLoader()) instead
Definition at line 1591 of file kiconloader.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:49:17 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.