KDEUI
#include <kidentityproxymodel.h>
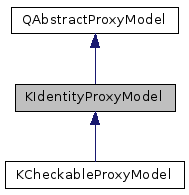
Public Member Functions | |
KIdentityProxyModel (QObject *parent=0) | |
virtual | ~KIdentityProxyModel () |
virtual bool | canFetchMore (const QModelIndex &parent) const |
int | columnCount (const QModelIndex &parent=QModelIndex()) const |
bool | dropMimeData (const QMimeData *data, Qt::DropAction action, int row, int column, const QModelIndex &parent) |
virtual void | fetchMore (const QModelIndex &parent) |
QModelIndex | index (int row, int column, const QModelIndex &parent=QModelIndex()) const |
bool | insertColumns (int column, int count, const QModelIndex &parent=QModelIndex()) |
bool | insertRows (int row, int count, const QModelIndex &parent=QModelIndex()) |
QModelIndex | mapFromSource (const QModelIndex &sourceIndex) const |
QItemSelection | mapSelectionFromSource (const QItemSelection &selection) const |
QItemSelection | mapSelectionToSource (const QItemSelection &selection) const |
QModelIndex | mapToSource (const QModelIndex &proxyIndex) const |
QModelIndexList | match (const QModelIndex &start, int role, const QVariant &value, int hits=1, Qt::MatchFlags flags=Qt::MatchFlags(Qt::MatchStartsWith|Qt::MatchWrap)) const |
virtual QMimeData * | mimeData (const QModelIndexList &indexes) const |
virtual QStringList | mimeTypes () const |
QModelIndex | parent (const QModelIndex &child) const |
bool | removeColumns (int column, int count, const QModelIndex &parent=QModelIndex()) |
bool | removeRows (int row, int count, const QModelIndex &parent=QModelIndex()) |
int | rowCount (const QModelIndex &parent=QModelIndex()) const |
void | setSourceModel (QAbstractItemModel *sourceModel) |
virtual Qt::DropActions | supportedDropActions () const |
Protected Slots | |
virtual void | resetInternalData () |
Protected Member Functions | |
KIdentityProxyModel (KIdentityProxyModelPrivate *privateClass, QObject *parent) | |
Protected Attributes | |
KIdentityProxyModelPrivate *const | d_ptr |
Detailed Description
The KIdentityProxyModel class proxies its source model unmodified.
- Since
- 4.6
KIdentityProxyModel can be used to forward the structure of a source model exactly, with no sorting, filtering or other transformation. This is similar in concept to an identity matrix where A.I = A.
Because it does no sorting or filtering, this class is most suitable to proxy models which transform the data() of the source model. For example, a proxy model could be created to define the font used, or the background colour, or the tooltip etc. This removes the need to implement all data handling in the same class that creates the structure of the model, and can also be used to create re-usable components.
This also provides a way to change the data in the case where a source model is supplied by a third party which can not be modified.
- Since
- 4.6
Definition at line 31 of file kidentityproxymodel.h.
Constructor & Destructor Documentation
|
explicit |
Constructs an identity model with the given parent.
Definition at line 123 of file kidentityproxymodel.cpp.
|
virtual |
Destroys this identity model.
Definition at line 140 of file kidentityproxymodel.cpp.
|
protected |
Definition at line 131 of file kidentityproxymodel.cpp.
Member Function Documentation
|
virtual |
- Reimplemented from superclass.
Definition at line 148 of file kidentityproxymodel.cpp.
int KIdentityProxyModel::columnCount | ( | const QModelIndex & | parent = QModelIndex() | ) | const |
\reimp
Definition at line 159 of file kidentityproxymodel.cpp.
bool KIdentityProxyModel::dropMimeData | ( | const QMimeData * | data, |
Qt::DropAction | action, | ||
int | row, | ||
int | column, | ||
const QModelIndex & | parent | ||
) |
\reimp
Definition at line 181 of file kidentityproxymodel.cpp.
|
virtual |
- Reimplemented from superclass.
Definition at line 170 of file kidentityproxymodel.cpp.
QModelIndex KIdentityProxyModel::index | ( | int | row, |
int | column, | ||
const QModelIndex & | parent = QModelIndex() |
||
) | const |
- Reimplemented from superclass.
Definition at line 192 of file kidentityproxymodel.cpp.
bool KIdentityProxyModel::insertColumns | ( | int | column, |
int | count, | ||
const QModelIndex & | parent = QModelIndex() |
||
) |
- Reimplemented from superclass.
Definition at line 208 of file kidentityproxymodel.cpp.
bool KIdentityProxyModel::insertRows | ( | int | row, |
int | count, | ||
const QModelIndex & | parent = QModelIndex() |
||
) |
- Reimplemented from superclass.
Definition at line 219 of file kidentityproxymodel.cpp.
QModelIndex KIdentityProxyModel::mapFromSource | ( | const QModelIndex & | sourceIndex | ) | const |
- Reimplemented from superclass.
Definition at line 230 of file kidentityproxymodel.cpp.
QItemSelection KIdentityProxyModel::mapSelectionFromSource | ( | const QItemSelection & | selection | ) | const |
- Reimplemented from superclass.
Definition at line 242 of file kidentityproxymodel.cpp.
QItemSelection KIdentityProxyModel::mapSelectionToSource | ( | const QItemSelection & | selection | ) | const |
- Reimplemented from superclass.
Definition at line 263 of file kidentityproxymodel.cpp.
QModelIndex KIdentityProxyModel::mapToSource | ( | const QModelIndex & | proxyIndex | ) | const |
- Reimplemented from superclass.
Definition at line 299 of file kidentityproxymodel.cpp.
QModelIndexList KIdentityProxyModel::match | ( | const QModelIndex & | start, |
int | role, | ||
const QVariant & | value, | ||
int | hits = 1 , |
||
Qt::MatchFlags | flags = Qt::MatchFlags(Qt::MatchStartsWith|Qt::MatchWrap) |
||
) | const |
- Reimplemented from superclass.
Definition at line 310 of file kidentityproxymodel.cpp.
|
virtual |
Definition at line 336 of file kidentityproxymodel.cpp.
|
virtual |
- Reimplemented from superclass.
Definition at line 328 of file kidentityproxymodel.cpp.
QModelIndex KIdentityProxyModel::parent | ( | const QModelIndex & | child | ) | const |
\reimp
Definition at line 352 of file kidentityproxymodel.cpp.
bool KIdentityProxyModel::removeColumns | ( | int | column, |
int | count, | ||
const QModelIndex & | parent = QModelIndex() |
||
) |
- Reimplemented from superclass.
Definition at line 366 of file kidentityproxymodel.cpp.
bool KIdentityProxyModel::removeRows | ( | int | row, |
int | count, | ||
const QModelIndex & | parent = QModelIndex() |
||
) |
- Reimplemented from superclass.
Definition at line 378 of file kidentityproxymodel.cpp.
|
protectedvirtualslot |
This slot is automatically invoked when the model is reset. It can be used to clear any data internal to the proxy at the appropriate time.
- See also
- QAbstractItemModel::internalDataReset()
Definition at line 755 of file kidentityproxymodel.cpp.
int KIdentityProxyModel::rowCount | ( | const QModelIndex & | parent = QModelIndex() | ) | const |
- Reimplemented from superclass.
Definition at line 390 of file kidentityproxymodel.cpp.
void KIdentityProxyModel::setSourceModel | ( | QAbstractItemModel * | sourceModel | ) |
- Reimplemented from superclass.
Definition at line 401 of file kidentityproxymodel.cpp.
|
virtual |
Definition at line 505 of file kidentityproxymodel.cpp.
Member Data Documentation
|
protected |
Definition at line 64 of file kidentityproxymodel.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:49:17 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.