KDEUI
#include <klineedit.h>
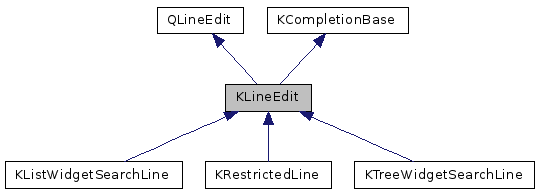
Public Slots | |
virtual void | clear () |
bool | passwordMode () const |
void | rotateText (KCompletionBase::KeyBindingType type) |
void | setCompletedItems (const QStringList &items, bool autoSuggest=true) |
virtual void | setCompletedText (const QString &) |
void | setPasswordMode (bool b=true) |
virtual void | setReadOnly (bool) |
void | setSqueezedText (const QString &text) |
virtual void | setText (const QString &) |
Signals | |
void | aboutToShowContextMenu (QMenu *menu) |
void | clearButtonClicked () |
void | completion (const QString &) |
void | completionBoxActivated (const QString &) |
void | completionModeChanged (KGlobalSettings::Completion) |
void | returnPressed (const QString &) |
void | substringCompletion (const QString &) |
void | textRotation (KCompletionBase::KeyBindingType) |
QT_MOC_COMPAT void | userTextChanged (const QString &) |
Protected Slots | |
virtual void | makeCompletion (const QString &) |
void | userCancelled (const QString &cancelText) |
Protected Member Functions | |
bool | autoSuggest () const |
virtual void | contextMenuEvent (QContextMenuEvent *) |
virtual void | create (WId=0, bool initializeWindow=true, bool destroyOldWindow=true) |
QMenu * | createStandardContextMenu () |
virtual void | dropEvent (QDropEvent *) |
virtual bool | event (QEvent *) |
virtual void | focusInEvent (QFocusEvent *ev) |
virtual void | focusOutEvent (QFocusEvent *ev) |
virtual void | keyPressEvent (QKeyEvent *) |
virtual void | mouseDoubleClickEvent (QMouseEvent *) |
virtual void | mousePressEvent (QMouseEvent *) |
virtual void | mouseReleaseEvent (QMouseEvent *) |
virtual void | paintEvent (QPaintEvent *ev) |
virtual void | resizeEvent (QResizeEvent *) |
virtual void | setCompletedText (const QString &, bool) |
void | setUserSelection (bool userSelection) |
![]() | |
KCompletionBase * | delegate () const |
KeyBindingMap | getKeyBindings () const |
void | setDelegate (KCompletionBase *delegate) |
virtual void | virtual_hook (int id, void *data) |
Properties | |
QString | clickMessage |
bool | contextMenuEnabled |
bool | passwordMode |
bool | showClearButton |
bool | squeezedTextEnabled |
bool | trapEnterKeyEvent |
bool | urlDropsEnabled |
Additional Inherited Members | |
![]() | |
typedef QMap< KeyBindingType, KShortcut > | KeyBindingMap |
enum | KeyBindingType { TextCompletion, PrevCompletionMatch, NextCompletionMatch, SubstringCompletion } |
Detailed Description
An enhanced QLineEdit widget for inputting text.
Detail
This widget inherits from QLineEdit and implements the following additional functionalities: a completion object that provides both automatic and manual text completion as well as multiple match iteration features, configurable key-bindings to activate these features and a popup-menu item that can be used to allow the user to set text completion modes on the fly based on their preference.
To support these new features KLineEdit also emits a few more additional signals. These are: completion( const QString& ), textRotation( KeyBindingType ), and returnPressed( const QString& ). The completion signal can be connected to a slot that will assist the user in filling out the remaining text. The text rotation signal is intended to be used to iterate through the list of all possible matches whenever there is more than one match for the entered text. The returnPressed( const QString& )
signals are the same as QLineEdit's except it provides the current text in the widget as its argument whenever appropriate.
This widget by default creates a completion object when you invoke the completionObject( bool ) member function for the first time or use setCompletionObject( KCompletion*, bool ) to assign your own completion object. Additionally, to make this widget more functional, KLineEdit will by default handle the text rotation and completion events internally when a completion object is created through either one of the methods mentioned above. If you do not need this functionality, simply use KCompletionBase::setHandleSignals( bool ) or set the boolean parameter in the above functions to false.
The default key-bindings for completion and rotation is determined from the global settings in KStandardShortcut. These values, however, can be overridden locally by invoking KCompletionBase::setKeyBinding(). The values can easily be reverted back to the default setting, by simply calling useGlobalSettings(). An alternate method would be to default individual key-bindings by using setKeyBinding() with the default second argument.
If EchoMode
for this widget is set to something other than QLineEdit::Normal
, the completion mode will always be defaulted to KGlobalSettings::CompletionNone. This is done purposefully to guard against protected entries such as passwords being cached in KCompletion's list. Hence, if the EchoMode
is not QLineEdit::Normal, the completion mode is automatically disabled.
A read-only KLineEdit will have the same background color as a disabled KLineEdit, but its foreground color will be the one used for the read-write mode. This differs from QLineEdit's implementation and is done to give visual distinction between the three different modes: disabled, read-only, and read-write.
KLineEdit has also a password mode which depends of globals KDE settings. Use KLineEdit::setPasswordMode instead of QLineEdit::echoMode property to have a password field.
Usage
To enable the basic completion feature:
To use a customized completion objects or your own completion object:
Note if you specify your own completion object you have to either delete it when you don't need it anymore, or you can tell KLineEdit to delete it for you:
Miscellaneous function calls :
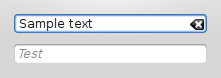
Definition at line 149 of file klineedit.h.
Constructor & Destructor Documentation
Constructs a KLineEdit object with a default text, a parent, and a name.
- Parameters
-
string Text to be shown in the edit widget. parent The parent widget of the line edit.
Definition at line 232 of file klineedit.cpp.
|
explicit |
Constructs a line edit.
- Parameters
-
parent The parent widget of the line edit.
Definition at line 238 of file klineedit.cpp.
|
virtual |
Destructor.
Definition at line 245 of file klineedit.cpp.
Member Function Documentation
|
signal |
Emitted before the context menu is displayed.
The signal allows you to add your own entries into the the context menu that is created on demand.
NOTE: Do not store the pointer to the QMenu provided through since it is created and deleted on demand.
- Parameters
-
p the context menu about to be displayed
|
protected |
Whether in current state text should be auto-suggested.
Definition at line 1726 of file klineedit.cpp.
|
virtualslot |
Reimplemented to workaround a buggy QLineEdit::clear() (changing the clipboard to the text we just had in the lineedit)
Definition at line 1698 of file klineedit.cpp.
|
signal |
Emitted when the user clicked on the clear button.
QSize KLineEdit::clearButtonUsedSize | ( | ) | const |
QString KLineEdit::clickMessage | ( | ) | const |
- Returns
- the message set with setClickMessage
|
signal |
Emitted when the completion key is pressed.
Please note that this signal is not emitted if the completion mode is set to CompletionNone
or EchoMode
is normal.
KCompletionBox * KLineEdit::completionBox | ( | bool | create = true | ) |
- Returns
- the completion-box, that is used in completion mode KGlobalSettings::CompletionPopup. This method will create a completion-box if none is there, yet.
- Parameters
-
create Set this to false if you don't want the box to be created i.e. to test if it is available.
Definition at line 1629 of file klineedit.cpp.
|
signal |
Emitted whenever the completion box is activated.
|
signal |
Emitted when the user changed the completion mode by using the popupmenu.
|
protectedvirtual |
Re-implemented for internal reasons.
API not affected.
See QLineEdit::contextMenuEvent().
Reimplemented in KTreeWidgetSearchLine.
Definition at line 1261 of file klineedit.cpp.
|
virtual |
Reimplemented for internal reasons, the API is not affected.
Definition at line 657 of file klineedit.cpp.
|
protectedvirtual |
Reimplemented for internal reasons, the API is not affected.
Definition at line 1655 of file klineedit.cpp.
|
protected |
Re-implemented for internal reasons.
API not affected.
See QLineEdit::createStandardContextMenu().
Definition at line 1180 of file klineedit.cpp.
void KLineEdit::doCompletion | ( | const QString & | txt | ) |
Do completion now.
This is called automatically when typing a key for instance. Emits completion() and/or calls makeCompletion(), depending on emitSignals and handleSignals.
- Since
- 4.2.1
Definition at line 1860 of file klineedit.cpp.
|
protectedvirtual |
Re-implemented to handle URI drops.
See QLineEdit::dropEvent().
Definition at line 1321 of file klineedit.cpp.
|
protectedvirtual |
Re-implemented for internal reasons.
API not affected.
Reimplemented in KTreeWidgetSearchLine, and KListWidgetSearchLine.
Definition at line 1358 of file klineedit.cpp.
|
protectedvirtual |
Definition at line 1805 of file klineedit.cpp.
|
protectedvirtual |
Definition at line 1810 of file klineedit.cpp.
bool KLineEdit::isClearButtonShown | ( | ) | const |
- Returns
- whether or not the clear button is shown
Definition at line 310 of file klineedit.cpp.
bool KLineEdit::isContextMenuEnabled | ( | ) | const |
Returns true
when the context menu is enabled.
- Deprecated:
- use contextMenuPolicy
Definition at line 1830 of file klineedit.cpp.
bool KLineEdit::isSqueezedTextEnabled | ( | ) | const |
Returns true if text squeezing is enabled.
This is only valid when the widget is in read-only mode.
Definition at line 564 of file klineedit.cpp.
|
protectedvirtual |
Re-implemented for internal reasons.
API not affected.
See QLineEdit::keyPressEvent().
Reimplemented in KRestrictedLine.
Definition at line 702 of file klineedit.cpp.
|
protectedvirtualslot |
Completes the remaining text with a matching one from a given list.
Definition at line 482 of file klineedit.cpp.
|
protectedvirtual |
Re-implemented for internal reasons.
API not affected.
See QWidget::mouseDoubleClickEvent().
Definition at line 1102 of file klineedit.cpp.
|
protectedvirtual |
Re-implemented for internal reasons.
API not affected.
See QLineEdit::mousePressEvent().
Definition at line 1113 of file klineedit.cpp.
|
protectedvirtual |
Re-implemented for internal reasons.
API not affected.
See QLineEdit::mouseReleaseEvent().
Definition at line 1144 of file klineedit.cpp.
QString KLineEdit::originalText | ( | ) | const |
Returns the original text if text squeezing is enabled.
If the widget is not in "read-only" mode, this function returns the same thing as QLineEdit::text().
- See also
- QLineEdit
Definition at line 1713 of file klineedit.cpp.
|
protectedvirtual |
Definition at line 1731 of file klineedit.cpp.
|
slot |
- Returns
- returns true if the lineedit is set to password mode echoing
|
protectedvirtual |
Re-implemented for internal reasons.
API not affected.
See QLineEdit::resizeEvent().
Definition at line 692 of file klineedit.cpp.
|
signal |
Emitted when the user presses the return key.
The argument is the current text. Note that this signal is not emitted if the widget's EchoMode
is set to QLineEdit::EchoMode.
|
slot |
Iterates through all possible matches of the completed text or the history list.
This function simply iterates over all possible matches in case multiple matches are found as a result of a text completion request. It will have no effect if only a single match is found.
- Parameters
-
type The key-binding invoked.
Definition at line 461 of file klineedit.cpp.
void KLineEdit::setClearButtonShown | ( | bool | show | ) |
This makes the line edit display an icon on one side of the line edit which, when clicked, clears the contents of the line edit.
This is useful for such things as location or search bars.
Definition at line 284 of file klineedit.cpp.
void KLineEdit::setClickMessage | ( | const QString & | msg | ) |
This makes the line edit display a grayed-out hinting text as long as the user didn't enter any text.
It is often used as indication about the purpose of the line edit.
Definition at line 1815 of file klineedit.cpp.
|
slot |
Same as the above function except it allows you to temporarily turn off text completion in CompletionPopupAuto mode.
- Parameters
-
items list of completion matches to be shown in the completion box. autoSuggest true if you want automatic text completion (suggestion) enabled.
Definition at line 1567 of file klineedit.cpp.
|
virtualslot |
See KCompletionBase::setCompletedText.
Definition at line 451 of file klineedit.cpp.
This function simply sets the lineedit text and highlights the text appropriately if the boolean value is set to true.
- Parameters
-
text marked
Definition at line 432 of file klineedit.cpp.
void KLineEdit::setCompletionBox | ( | KCompletionBox * | box | ) |
Set the completion-box to be used in completion mode KGlobalSettings::CompletionPopup.
This will do nothing if a completion-box already exists.
- Parameters
-
box The KCompletionBox to set
Definition at line 1431 of file klineedit.cpp.
|
virtual |
Re-implemented from KCompletionBase for internal reasons.
This function is re-implemented in order to make sure that the EchoMode is acceptable before we set the completion mode.
See KCompletionBase::setCompletionMode
Reimplemented from KCompletionBase.
Definition at line 400 of file klineedit.cpp.
void KLineEdit::setCompletionModeDisabled | ( | KGlobalSettings::Completion | mode, |
bool | disable = true |
||
) |
Disables completion modes by makeing them non-checkable.
The context menu allows to change the completion mode. This method allows to disable some modes.
Definition at line 427 of file klineedit.cpp.
|
virtual |
Reimplemented for internal reasons, the API is not affected.
Reimplemented from KCompletionBase.
Definition at line 1640 of file klineedit.cpp.
|
virtual |
Enables/disables the popup (context) menu.
This method only works if this widget is editable, i.e. read-write and allows you to enable/disable the context menu. It does nothing if invoked for a none-editable combo-box.
By default, the context menu is created if this widget is editable. Call this function with the argument set to false to disable the popup menu.
- Parameters
-
showMenu If true
, show the context menu.
- Deprecated:
- use setContextMenuPolicy
Definition at line 1823 of file klineedit.cpp.
|
slot |
set the line edit in password mode.
this change the EchoMode according to KDE preferences.
- Parameters
-
b true to set in password mode
Definition at line 1836 of file klineedit.cpp.
|
virtualslot |
Sets the lineedit to read-only.
Similar to QLineEdit::setReadOnly but also takes care of the background color, and the clear button.
Definition at line 521 of file klineedit.cpp.
|
slot |
Squeezes text
into the line edit.
This can only be used with read-only line-edits.
Definition at line 553 of file klineedit.cpp.
void KLineEdit::setSqueezedTextEnabled | ( | bool | enable | ) |
Enable text squeezing whenever the supplied text is too long.
Only works for "read-only" mode.
Note that once text squeezing is enabled, QLineEdit::text() and QLineEdit::displayText() return the squeezed text. If you want the original text, use originalText.
- See also
- QLineEdit
Definition at line 559 of file klineedit.cpp.
|
virtualslot |
Re-implemented to enable text squeezing.
API is not affected.
Definition at line 569 of file klineedit.cpp.
void KLineEdit::setTrapReturnKey | ( | bool | trap | ) |
By default, KLineEdit recognizes Key_Return
and Key_Enter
and emits the returnPressed() signals, but it also lets the event pass, for example causing a dialog's default-button to be called.
Call this method with trap
= true
to make KLineEdit
stop these events. The signals will still be emitted of course.
- See also
- trapReturnKey()
Definition at line 1416 of file klineedit.cpp.
void KLineEdit::setUrl | ( | const KUrl & | url | ) |
Sets url
into the lineedit.
It uses KUrl::prettyUrl() so that the url is properly decoded for displaying.
Definition at line 1426 of file klineedit.cpp.
void KLineEdit::setUrlDropsEnabled | ( | bool | enable | ) |
Enables/Disables handling of URL drops.
If enabled and the user drops an URL, the decoded URL will be inserted. Otherwise the default behavior of QLineEdit is used, which inserts the encoded URL.
- Parameters
-
enable If true
, insert decoded URLs
Definition at line 1406 of file klineedit.cpp.
|
protected |
Sets the widget in userSelection mode or in automatic completion selection mode.
This changes the colors of selections.
Definition at line 1661 of file klineedit.cpp.
|
signal |
Emitted when the shortcut for substring completion is pressed.
|
signal |
Emitted when the text rotation key-bindings are pressed.
The argument indicates which key-binding was pressed. In KLineEdit's case this can be either one of two values: PrevCompletionMatch or NextCompletionMatch. See KCompletionBase::setKeyBinding for details.
Note that this signal is not emitted if the completion mode is set to KGlobalSettings::CompletionNone
or echoMode()
is not normal.
bool KLineEdit::trapReturnKey | ( | ) | const |
- Returns
true
if keyevents ofKey_Return
orKey_Enter
will be stopped or if they will be propagated.
- See also
- setTrapReturnKey ()
Definition at line 1421 of file klineedit.cpp.
bool KLineEdit::urlDropsEnabled | ( | ) | const |
Returns true
when decoded URL drops are enabled.
|
protectedslot |
Resets the current displayed text.
Call this function to revert a text completion if the user cancels the request. Mostly applies to popup completions.
Definition at line 1465 of file klineedit.cpp.
QString KLineEdit::userText | ( | ) | const |
Returns the text as given by the user (i.e.
not autocompleted) if the widget has autocompletion disabled, this function returns the same as QLineEdit::text().
- Since
- 4.2.2
Definition at line 1721 of file klineedit.cpp.
|
signal |
Emitted when the text is changed NOT by the suggested autocompletion: either when the user is physically typing keys, or when the text is changed programmatically, for example, by calling setText().
But not when automatic completion changes the text temporarily.
- Since
- 4.2.2
- Deprecated:
- since 4.5. You probably want to connect to textEdited() instead, which is emitted whenever the text is actually changed by the user (by typing or accepting autocompletion), without side effects from suggested autocompletion either. userTextChanged isn't needed anymore.
Property Documentation
|
readwrite |
Definition at line 161 of file klineedit.h.
|
readwrite |
Definition at line 156 of file klineedit.h.
|
readwrite |
Definition at line 163 of file klineedit.h.
|
readwrite |
Definition at line 162 of file klineedit.h.
|
readwrite |
Definition at line 160 of file klineedit.h.
|
readwrite |
Definition at line 159 of file klineedit.h.
|
readwrite |
Definition at line 158 of file klineedit.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:49:17 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.