KDEUI
#include <kmessagewidget.h>
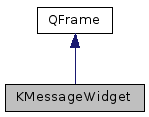
Public Types | |
enum | MessageType { Positive, Information, Warning, Error } |
Public Slots | |
void | animatedHide () |
void | animatedShow () |
void | setCloseButtonVisible (bool visible) |
void | setIcon (const QIcon &icon) |
void | setMessageType (KMessageWidget::MessageType type) |
void | setText (const QString &text) |
void | setWordWrap (bool wordWrap) |
Signals | |
void | linkActivated (const QString &contents) |
void | linkHovered (const QString &contents) |
Public Member Functions | |
KMessageWidget (QWidget *parent=0) | |
KMessageWidget (const QString &text, QWidget *parent=0) | |
~KMessageWidget () | |
void | addAction (QAction *action) |
int | heightForWidth (int width) const |
QIcon | icon () const |
bool | isCloseButtonVisible () const |
MessageType | messageType () const |
QSize | minimumSizeHint () const |
void | removeAction (QAction *action) |
QSize | sizeHint () const |
QString | text () const |
bool | wordWrap () const |
Protected Member Functions | |
bool | event (QEvent *event) |
void | paintEvent (QPaintEvent *event) |
void | resizeEvent (QResizeEvent *event) |
void | showEvent (QShowEvent *event) |
Properties | |
bool | closeButtonVisible |
QIcon | icon |
MessageType | messageType |
QString | text |
bool | wordWrap |
Detailed Description
A widget to provide feedback or propose opportunistic interactions.
KMessageWidget can be used to provide inline positive or negative feedback, or to implement opportunistic interactions.
As a feedback widget, KMessageWidget provides a less intrusive alternative to "OK Only" message boxes. If you do not need the modalness of KMessageBox, consider using KMessageWidget instead.
Negative feedback
The KMessageWidget can be used as a secondary indicator of failure: the first indicator is usually the fact the action the user expected to happen did not happen.
Example: User fills a form, clicks "Submit".
- Expected feedback: form closes
- First indicator of failure: form stays there
- Second indicator of failure: a KMessageWidget appears on top of the form, explaining the error condition
When used to provide negative feedback, KMessageWidget should be placed close to its context. In the case of a form, it should appear on top of the form entries.
KMessageWidget should get inserted in the existing layout. Space should not be reserved for it, otherwise it becomes "dead space", ignored by the user. KMessageWidget should also not appear as an overlay to prevent blocking access to elements the user needs to interact with to fix the failure.
Positive feedback
KMessageWidget can be used for positive feedback but it shouldn't be overused. It is often enough to provide feedback by simply showing the results of an action.
Examples of acceptable uses:
- Confirm success of "critical" transactions
- Indicate completion of background tasks
Example of inadapted uses:
- Indicate successful saving of a file
- Indicate a file has been successfully removed
Opportunistic interaction
Opportunistic interaction is the situation where the application suggests to the user an action he could be interested in perform, either based on an action the user just triggered or an event which the application noticed.
Example of acceptable uses:
- A browser can propose remembering a recently entered password
- A music collection can propose ripping a CD which just got inserted
- A chat application may notify the user a "special friend" just connected
- Since
- 4.7
Definition at line 92 of file kmessagewidget.h.
Member Enumeration Documentation
Enumerator | |
---|---|
Positive | |
Information | |
Warning | |
Error |
Definition at line 103 of file kmessagewidget.h.
Constructor & Destructor Documentation
|
explicit |
Constructs a KMessageWidget with the specified parent.
Definition at line 210 of file kmessagewidget.cpp.
Definition at line 217 of file kmessagewidget.cpp.
KMessageWidget::~KMessageWidget | ( | ) |
Definition at line 225 of file kmessagewidget.cpp.
Member Function Documentation
void KMessageWidget::addAction | ( | QAction * | action | ) |
Definition at line 386 of file kmessagewidget.cpp.
|
slot |
Hide the widget using an animation, unless KGlobalSettings::graphicsEffectLevel() does not allow simple effects.
Definition at line 422 of file kmessagewidget.cpp.
|
slot |
Show the widget using an animation, unless KGlobalSettings::graphicsEffectLevel() does not allow simple effects.
Definition at line 398 of file kmessagewidget.cpp.
|
protected |
Definition at line 314 of file kmessagewidget.cpp.
int KMessageWidget::heightForWidth | ( | int | width | ) | const |
Definition at line 331 of file kmessagewidget.cpp.
QIcon KMessageWidget::icon | ( | ) | const |
The icon shown on the left of the text.
By default, no icon is shown.
- Since
- 4.11
bool KMessageWidget::isCloseButtonVisible | ( | ) | const |
Definition at line 375 of file kmessagewidget.cpp.
|
signal |
This signal is emitted when the user clicks a link in the text label.
The URL referred to by the href anchor is passed in contents.
- Parameters
-
contents text of the href anchor
- See also
- QLabel::linkActivated()
- Since
- 4.10
|
signal |
This signal is emitted when the user hovers over a link in the text label.
The URL referred to by the href anchor is passed in contents.
- Parameters
-
contents text of the href anchor
- See also
- QLabel::linkHovered()
- Since
- 4.11
MessageType KMessageWidget::messageType | ( | ) | const |
QSize KMessageWidget::minimumSizeHint | ( | ) | const |
Definition at line 308 of file kmessagewidget.cpp.
|
protected |
Definition at line 337 of file kmessagewidget.cpp.
void KMessageWidget::removeAction | ( | QAction * | action | ) |
Definition at line 392 of file kmessagewidget.cpp.
|
protected |
Definition at line 322 of file kmessagewidget.cpp.
|
slot |
Definition at line 380 of file kmessagewidget.cpp.
|
slot |
Define an icon to be shown on the left of the text.
- Since
- 4.11
Definition at line 447 of file kmessagewidget.cpp.
|
slot |
Definition at line 253 of file kmessagewidget.cpp.
|
slot |
Definition at line 235 of file kmessagewidget.cpp.
|
slot |
Definition at line 359 of file kmessagewidget.cpp.
|
protected |
Definition at line 347 of file kmessagewidget.cpp.
QSize KMessageWidget::sizeHint | ( | void | ) | const |
Definition at line 302 of file kmessagewidget.cpp.
QString KMessageWidget::text | ( | ) | const |
bool KMessageWidget::wordWrap | ( | ) | const |
Property Documentation
|
readwrite |
Definition at line 99 of file kmessagewidget.h.
|
readwrite |
Definition at line 101 of file kmessagewidget.h.
|
readwrite |
Definition at line 100 of file kmessagewidget.h.
|
readwrite |
Definition at line 97 of file kmessagewidget.h.
|
readwrite |
Definition at line 98 of file kmessagewidget.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:49:17 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.