KDEUI
#include <kpassivepopup.h>
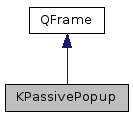
Public Types | |
enum | PopupStyle { Boxed, Balloon, CustomStyle =128 } |
Public Slots | |
void | setPopupStyle (int popupstyle) |
void | setTimeout (int delay) |
virtual void | setVisible (bool visible) |
void | show () |
void | show (const QPoint &p) |
Signals | |
void | clicked () |
void | clicked (const QPoint &pos) |
Public Member Functions | |
KPassivePopup (QWidget *parent=0, Qt::WindowFlags f=0) | |
KPassivePopup (WId parent) | |
virtual | ~KPassivePopup () |
QPoint | anchor () const |
bool | autoDelete () const |
QRect | defaultArea () const |
void | setAnchor (const QPoint &anchor) |
virtual void | setAutoDelete (bool autoDelete) |
void | setView (QWidget *child) |
void | setView (const QString &caption, const QString &text=QString()) |
virtual void | setView (const QString &caption, const QString &text, const QPixmap &icon) |
KVBox * | standardView (const QString &caption, const QString &text, const QPixmap &icon, QWidget *parent=0L) |
int | timeout () const |
QWidget * | view () const |
Static Public Member Functions | |
static KPassivePopup * | message (const QString &text, QWidget *parent) |
static KPassivePopup * | message (const QString &text, QSystemTrayIcon *parent) |
static KPassivePopup * | message (const QString &caption, const QString &text, QWidget *parent) |
static KPassivePopup * | message (const QString &caption, const QString &text, QSystemTrayIcon *parent) |
static KPassivePopup * | message (const QString &caption, const QString &text, const QPixmap &icon, QWidget *parent, int timeout=-1) |
static KPassivePopup * | message (const QString &caption, const QString &text, const QPixmap &icon, QSystemTrayIcon *parent, int timeout=-1) |
static KPassivePopup * | message (const QString &caption, const QString &text, const QPixmap &icon, WId parent, int timeout=-1) |
static KPassivePopup * | message (int popupStyle, const QString &text, QWidget *parent) |
static KPassivePopup * | message (int popupStyle, const QString &text, QSystemTrayIcon *parent) |
static KPassivePopup * | message (int popupStyle, const QString &caption, const QString &text, QSystemTrayIcon *parent) |
static KPassivePopup * | message (int popupStyle, const QString &caption, const QString &text, QWidget *parent) |
static KPassivePopup * | message (int popupStyle, const QString &caption, const QString &text, const QPixmap &icon, QWidget *parent, int timeout=-1) |
static KPassivePopup * | message (int popupStyle, const QString &caption, const QString &text, const QPixmap &icon, QSystemTrayIcon *parent, int timeout=-1) |
static KPassivePopup * | message (int popupStyle, const QString &caption, const QString &text, const QPixmap &icon, WId parent, int timeout=-1) |
Protected Member Functions | |
QPoint | calculateNearbyPoint (const QRect &target) |
virtual void | hideEvent (QHideEvent *) |
virtual void | mouseReleaseEvent (QMouseEvent *e) |
void | moveNear (const QRect &target) |
virtual void | paintEvent (QPaintEvent *pe) |
virtual void | positionSelf () |
void | updateMask () |
Properties | |
bool | autoDelete |
QRect | defaultArea |
int | timeout |
Detailed Description
A dialog-like popup that displays messages without interrupting the user.
The simplest uses of KPassivePopup are by using the various message() static methods. The position the popup appears at depends on the type of the parent window:
- Normal Windows: The popup is placed adjacent to the icon of the window.
- System Tray Windows: The popup is placed adjact to the system tray window itself.
- Skip Taskbar Windows: The popup is placed adjact to the window itself if it is visible, and at the edge of the desktop otherwise.
You also have the option of calling show with a QPoint as a parameter that removes the automatic placing of KPassivePopup and shows it in the point you want.
The most basic use of KPassivePopup displays a popup containing a piece of text:
We can create popups with titles and icons too, as this example shows:
This screenshot shows a popup with both a caption and a main text which is being displayed next to the toolbar icon of the window that triggered it:
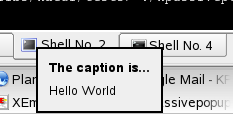
For more control over the popup, you can use the setView(QWidget *) method to create a custom popup.
Definition at line 79 of file kpassivepopup.h.
Member Enumeration Documentation
Styles that a KPassivePopup can have.
Enumerator | |
---|---|
Boxed |
Information will appear in a framed box (default) |
Balloon |
Information will appear in a comic-alike balloon. |
CustomStyle |
Ids greater than this are reserved for use by subclasses. |
Definition at line 90 of file kpassivepopup.h.
Constructor & Destructor Documentation
|
explicit |
Creates a popup for the specified widget.
Definition at line 86 of file kpassivepopup.cpp.
|
explicit |
Creates a popup for the specified window.
Definition at line 93 of file kpassivepopup.cpp.
|
virtual |
Cleans up.
Definition at line 141 of file kpassivepopup.cpp.
Member Function Documentation
QPoint KPassivePopup::anchor | ( | ) | const |
Returns the position to which this popup is anchored.
Definition at line 427 of file kpassivepopup.cpp.
bool KPassivePopup::autoDelete | ( | ) | const |
- Returns
- true if the widget auto-deletes itself when the timeout occurs.
- See also
- setAutoDelete
Calculates the position to place the popup near the specified rectangle.
Definition at line 385 of file kpassivepopup.cpp.
|
signal |
Emitted when the popup is clicked.
|
signal |
Emitted when the popup is clicked.
QRect KPassivePopup::defaultArea | ( | ) | const |
If no relative window (eg taskbar button, system tray window) is available, use this rectangle (pass it to moveNear()).
Basically KWindowSystem::workArea() with width and height set to 0 so that moveNear uses the upper-left position.
- Returns
- The QRect to be passed to moveNear() if no other is available.
|
protectedvirtual |
Reimplemented to destroy the object when autoDelete() is enabled.
Definition at line 314 of file kpassivepopup.cpp.
|
static |
Convenience method that displays popup with the specified message beside the icon of the specified widget.
Note that the returned object is destroyed when it is hidden.
- See also
- setAutoDelete
Definition at line 538 of file kpassivepopup.cpp.
|
static |
Convenience method that displays popup with the specified message beside the icon of the specified QSystemTrayIcon.
Note that the returned object is destroyed when it is hidden.
- See also
- setAutoDelete
Definition at line 562 of file kpassivepopup.cpp.
|
static |
Convenience method that displays popup with the specified caption and message beside the icon of the specified widget.
Note that the returned object is destroyed when it is hidden.
- See also
- setAutoDelete
Definition at line 543 of file kpassivepopup.cpp.
|
static |
Convenience method that displays popup with the specified caption and message beside the icon of the specified QSystemTrayIcon.
Note that the returned object is destroyed when it is hidden.
- See also
- setAutoDelete
Definition at line 567 of file kpassivepopup.cpp.
|
static |
Convenience method that displays popup with the specified icon, caption and message beside the icon of the specified widget.
Note that the returned object is destroyed when it is hidden.
- See also
- setAutoDelete
Definition at line 531 of file kpassivepopup.cpp.
|
static |
Convenience method that displays popup with the specified icon, caption and message beside the icon of the specified QSystemTrayIcon.
Note that the returned object is destroyed when it is hidden.
- See also
- setAutoDelete
Definition at line 555 of file kpassivepopup.cpp.
|
static |
Convenience method that displays popup with the specified icon, caption and message beside the icon of the specified window.
Note that the returned object is destroyed when it is hidden.
- See also
- setAutoDelete
Definition at line 549 of file kpassivepopup.cpp.
|
static |
Convenience method that displays popup with the specified popup-style and message beside the icon of the specified widget.
Note that the returned object is destroyed when it is hidden.
- See also
- setAutoDelete
Definition at line 588 of file kpassivepopup.cpp.
|
static |
Convenience method that displays popup with the specified popup-style and message beside the icon of the specified QSystemTrayIcon.
Note that the returned object is destroyed when it is hidden.
- See also
- setAutoDelete
Definition at line 628 of file kpassivepopup.cpp.
|
static |
Convenience method that displays popup with the specified popup-style, caption and message beside the icon of the specified QSystemTrayIcon.
Note that the returned object is destroyed when it is hidden.
- See also
- setAutoDelete
Definition at line 633 of file kpassivepopup.cpp.
|
static |
Convenience method that displays popup with the specified popup-style, caption and message beside the icon of the specified widget.
Note that the returned object is destroyed when it is hidden.
- See also
- setAutoDelete
Definition at line 593 of file kpassivepopup.cpp.
|
static |
Convenience method that displays popup with the specified popup-style, icon, caption and message beside the icon of the specified widget.
Note that the returned object is destroyed when it is hidden.
- See also
- setAutoDelete
Definition at line 574 of file kpassivepopup.cpp.
|
static |
Convenience method that displays popup with the specified popup-style, icon, caption and message beside the icon of the specified QSystemTrayIcon.
Note that the returned object is destroyed when it is hidden.
- See also
- setAutoDelete
Definition at line 612 of file kpassivepopup.cpp.
|
static |
Convenience method that displays popup with the specified popup-style, icon, caption and message beside the icon of the specified window.
Note that the returned object is destroyed when it is hidden.
- See also
- setAutoDelete
Definition at line 599 of file kpassivepopup.cpp.
|
protectedvirtual |
Reimplemented to detect mouse clicks.
Definition at line 263 of file kpassivepopup.cpp.
|
protected |
Moves the popup to be adjacent to the icon of the specified rectangle.
Definition at line 376 of file kpassivepopup.cpp.
|
protectedvirtual |
Overwrite to paint the border when PopupStyle == Balloon.
Unused if PopupStyle == Boxed
Definition at line 438 of file kpassivepopup.cpp.
|
protectedvirtual |
This method positions the popup.
Definition at line 341 of file kpassivepopup.cpp.
void KPassivePopup::setAnchor | ( | const QPoint & | anchor | ) |
Sets the anchor of this popup.
The popup tries automatically to adjust itself somehow around the point.
Definition at line 432 of file kpassivepopup.cpp.
|
virtual |
Enables / disables auto-deletion of this widget when the timeout occurs.
The default is false. If you use the class-methods message(), auto-deletion is turned on by default.
Definition at line 258 of file kpassivepopup.cpp.
|
slot |
Sets the visual appearance of the popup.
- See also
- PopupStyle
Definition at line 146 of file kpassivepopup.cpp.
|
slot |
Sets the delay for the popup is removed automatically.
Setting the delay to 0 disables the timeout, if you're doing this, you may want to connect the clicked() signal to the hide() slot. Setting the delay to -1 makes it use the default value.
- See also
- timeout
Definition at line 240 of file kpassivepopup.cpp.
void KPassivePopup::setView | ( | QWidget * | child | ) |
Sets the main view to be the specified widget (which must be a child of the popup).
Definition at line 164 of file kpassivepopup.cpp.
Creates a standard view then calls setView(QWidget*) .
Definition at line 225 of file kpassivepopup.cpp.
|
virtual |
Creates a standard view then calls setView(QWidget*) .
Definition at line 178 of file kpassivepopup.cpp.
|
virtualslot |
Definition at line 273 of file kpassivepopup.cpp.
|
slot |
Reimplemented to reposition the popup.
Definition at line 303 of file kpassivepopup.cpp.
|
slot |
Shows the popup in the given point.
Definition at line 308 of file kpassivepopup.cpp.
KVBox * KPassivePopup::standardView | ( | const QString & | caption, |
const QString & | text, | ||
const QPixmap & | icon, | ||
QWidget * | parent = 0L |
||
) |
Returns a widget that is used as standard view if one of the setView() methods taking the QString arguments is used.
You can use the returned widget to customize the passivepopup while keeping the look similar to the "standard" passivepopups.
After customizing the widget, pass it to setView( QWidget* )
- Parameters
-
caption The window caption (title) on the popup text The text for the popup icon The icon to use for the popup parent The parent widget used for the returned KVBox. If left 0L, then "this", i.e. the passive popup object will be used.
- Returns
- a KVBox containing the given arguments, looking like the standard passivepopups.
- See also
- setView( QWidget * )
- setView( const QString&, const QString& )
- setView( const QString&, const QString&, const QPixmap& )
Definition at line 186 of file kpassivepopup.cpp.
int KPassivePopup::timeout | ( | ) | const |
Returns the delay before the popup is removed automatically.
|
protected |
Updates the transparency mask.
Unused if PopupStyle == Boxed
Definition at line 449 of file kpassivepopup.cpp.
QWidget * KPassivePopup::view | ( | ) | const |
Returns the main view.
Definition at line 230 of file kpassivepopup.cpp.
Property Documentation
|
readwrite |
Definition at line 82 of file kpassivepopup.h.
|
read |
Definition at line 84 of file kpassivepopup.h.
|
readwrite |
Definition at line 83 of file kpassivepopup.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:49:18 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.