KDEUI
#include <kplotwidget.h>
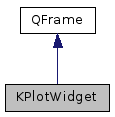
Public Types | |
enum | Axis { LeftAxis = 0, BottomAxis, RightAxis, TopAxis } |
Public Slots | |
void | setObjectToolTipShown (bool show) |
void | setShowGrid (bool show) |
Public Member Functions | |
KPlotWidget (QWidget *parent=0) | |
virtual | ~KPlotWidget () |
void | addPlotObject (KPlotObject *object) |
void | addPlotObjects (const QList< KPlotObject * > &objects) |
bool | antialiasing () const |
KPlotAxis * | axis (Axis type) |
const KPlotAxis * | axis (Axis type) const |
QColor | backgroundColor () const |
int | bottomPadding () const |
void | clearSecondaryLimits () |
QRectF | dataRect () const |
QColor | foregroundColor () const |
QColor | gridColor () const |
bool | isGridShown () const |
bool | isObjectToolTipShown () const |
int | leftPadding () const |
QPointF | mapToWidget (const QPointF &p) const |
void | maskAlongLine (const QPointF &p1, const QPointF &p2, float value=1.0) |
void | maskRect (const QRectF &r, float value=1.0) |
virtual QSize | minimumSizeHint () const |
QRect | pixRect () const |
void | placeLabel (QPainter *painter, KPlotPoint *pp) |
QList< KPlotObject * > | plotObjects () const |
void | removeAllPlotObjects () |
void | replacePlotObject (int i, KPlotObject *o) |
void | resetPlot () |
void | resetPlotMask () |
int | rightPadding () const |
QRectF | secondaryDataRect () const |
void | setAntialiasing (bool b) |
void | setBackgroundColor (const QColor &bg) |
void | setBottomPadding (int padding) |
void | setDefaultPaddings () |
void | setForegroundColor (const QColor &fg) |
void | setGridColor (const QColor &gc) |
void | setLeftPadding (int padding) |
void | setLimits (double x1, double x2, double y1, double y2) |
void | setRightPadding (int padding) |
void | setSecondaryLimits (double x1, double x2, double y1, double y2) |
void | setTopPadding (int padding) |
virtual QSize | sizeHint () const |
int | topPadding () const |
Protected Member Functions | |
virtual void | drawAxes (QPainter *p) |
virtual bool | event (QEvent *) |
virtual void | paintEvent (QPaintEvent *) |
QList< KPlotPoint * > | pointsUnderPoint (const QPoint &p) const |
virtual void | resizeEvent (QResizeEvent *) |
void | setPixRect () |
Properties | |
QColor | backgroundColor |
int | bottomPadding |
QColor | foregroundColor |
bool | grid |
QColor | gridColor |
int | leftPadding |
bool | objectToolTip |
int | rightPadding |
int | topPadding |
Detailed Description
Generic data plotting widget.
Widget for drawing plots. The basic idea behind KPlotWidget is that you don't have to worry about any transformation from your data's natural units to screen pixel coordinates; this is handled internally by the widget.
Data to be plotted are represented by one or more instances of KPlotObject. KPlotObject contains a list of QPointFs to be plotted (again, in the data's natural units), as well as information about how the data are to be rendered in the plot (i.e., as separate points or connected by lines? With what color and point style? etc). See KPlotObject for more information.
KPlotWidget automatically adds axis labels with tickmarks and tick labels. These are encapsulated in the KPlotAxis class. All you have to do is set the limits of the plotting area in data units, and KPlotWidget wil figure out the optimal positions and labels for the tickmarks on the axes.
Example of usage:
- Note
- KPlotWidget will take care of the objects added to it, so when clearing the objects list (eg with removeAllPlotObjects()) any previous reference to a KPlotObject already added to a KPlotWidget will be invalid.
- Version
- 1.1
Definition at line 80 of file kplotwidget.h.
Member Enumeration Documentation
enum KPlotWidget::Axis |
The four types of plot axes.
Enumerator | |
---|---|
LeftAxis |
the left axis |
BottomAxis |
the bottom axis |
RightAxis |
the right axis |
TopAxis |
the top axis |
Definition at line 106 of file kplotwidget.h.
Constructor & Destructor Documentation
|
explicit |
|
virtual |
Destructor.
Definition at line 120 of file kplotwidget.cpp.
Member Function Documentation
void KPlotWidget::addPlotObject | ( | KPlotObject * | object | ) |
Add an item to the list of KPlotObjects to be plotted.
- Note
- do not use this multiple time if many objects have to be added, addPlotObjects() is strongly suggested in this case
- Parameters
-
object the KPlotObject to be added
Definition at line 210 of file kplotwidget.cpp.
void KPlotWidget::addPlotObjects | ( | const QList< KPlotObject * > & | objects | ) |
Add more than one KPlotObject at one time.
- Parameters
-
objects the list of KPlotObjects to be added
Definition at line 219 of file kplotwidget.cpp.
bool KPlotWidget::antialiasing | ( | ) | const |
- Returns
- whether the antialiasing is active Antialiasing is not active by default.
Definition at line 323 of file kplotwidget.cpp.
- Returns
- the axis of the specified
type
, or 0 if no axis has been set.
- See also
- Axis
Definition at line 345 of file kplotwidget.cpp.
- Returns
- the axis of the specified
type
, or 0 if no axis has been set.
- See also
- Axis
Definition at line 351 of file kplotwidget.cpp.
QColor KPlotWidget::backgroundColor | ( | ) | const |
- Returns
- the background color of the plot.
The default color is black.
int KPlotWidget::bottomPadding | ( | ) | const |
- Returns
- the number of pixels below the plot area. Padding values are set to -1 by default; if unchanged, this function will try to guess a good value, based on whether ticklabels and/or axis labels are to be drawn.
void KPlotWidget::clearSecondaryLimits | ( | ) |
Unset the secondary limits, so the top and right axes show the same tickmarks as the bottom and left axes (no tickmark labels will be drawn for the top and right axes in this case)
Definition at line 192 of file kplotwidget.cpp.
QRectF KPlotWidget::dataRect | ( | ) | const |
- Returns
- the rectangle representing the boundaries of the current plot, in natural data units.
- See also
- setLimits()
Definition at line 200 of file kplotwidget.cpp.
|
protectedvirtual |
Draws the plot axes and axis labels.
Internal use only; one should simply call update() to draw the widget with axes and all objects.
- Parameters
-
p pointer to the painter on which we are drawing
Definition at line 695 of file kplotwidget.cpp.
|
protectedvirtual |
Generic event handler.
Definition at line 375 of file kplotwidget.cpp.
QColor KPlotWidget::foregroundColor | ( | ) | const |
- Returns
- the foreground color, used for axes, tickmarks and associated labels.
The default color is white.
QColor KPlotWidget::gridColor | ( | ) | const |
- Returns
- the grid color.
The default color is gray.
bool KPlotWidget::isGridShown | ( | ) | const |
- Returns
- whether the grid lines are shown Grid lines are not shown by default.
Definition at line 313 of file kplotwidget.cpp.
bool KPlotWidget::isObjectToolTipShown | ( | ) | const |
- Returns
- whether the tooltip for the point objects is shown. Tooltips are enabled by default.
Definition at line 318 of file kplotwidget.cpp.
int KPlotWidget::leftPadding | ( | ) | const |
- Returns
- the number of pixels to the left of the plot area.
Padding values are set to -1 by default; if unchanged, this function will try to guess a good value, based on whether ticklabels and/or axis labels need to be drawn.
QPointF KPlotWidget::mapToWidget | ( | const QPointF & | p | ) | const |
Map a coordinate.
- Parameters
-
p from the data rect to the physical pixel rect. Used mainly when drawing. p the point to be converted, in natural data units
- Returns
- the coordinate in the pixel coordinate system
Definition at line 405 of file kplotwidget.cpp.
void KPlotWidget::maskAlongLine | ( | const QPointF & | p1, |
const QPointF & | p2, | ||
float | value = 1.0 |
||
) |
Indicate that object labels should try to avoid the line joining the two given points (in pixel coordinates).
- Note
- You should not normally call this function directly. It is called by KPlotObject when lines are drawn in the plot.
- Parameters
-
p1 the starting point for the line p2 the ending point for the line value Allows you to determine how strongly the line should be avoided. Larger values are avoided more strongly.
Definition at line 427 of file kplotwidget.cpp.
void KPlotWidget::maskRect | ( | const QRectF & | r, |
float | value = 1.0 |
||
) |
Indicate that object labels should try to avoid the given rectangle in the plot.
The rectangle is in pixel coordinates.
- Note
- You should not normally call this function directly. It is called by KPlotObject when points, bars and labels are drawn.
- Parameters
-
r the rectangle defining the region in the plot that text labels should avoid (in pixel coordinates) value Allows you to determine how strongly the rectangle should be avoided. Larger values are avoided more strongly.
Definition at line 412 of file kplotwidget.cpp.
|
virtual |
- Returns
- suggested minimum size for the plot widget
Definition at line 125 of file kplotwidget.cpp.
|
protectedvirtual |
The paint event handler, executed when update() or repaint() is called.
Definition at line 667 of file kplotwidget.cpp.
QRect KPlotWidget::pixRect | ( | ) | const |
- Returns
- the rectangle representing the boundaries of the current plot, in screen pixel units.
Definition at line 357 of file kplotwidget.cpp.
void KPlotWidget::placeLabel | ( | QPainter * | painter, |
KPlotPoint * | pp | ||
) |
Place an object label optimally in the plot.
This function will attempt to place the label as close as it can to the point to which the label belongs, while avoiding overlap with regions of the plot that have been masked.
- Note
- You should not normally call this function directly. It is called internally in KPlotObject::draw().
- Parameters
-
painter Pointer to the painter on which to draw the label pp pointer to the KPlotPoint whose label is to be drawn.
Definition at line 489 of file kplotwidget.cpp.
QList< KPlotObject * > KPlotWidget::plotObjects | ( | ) | const |
- Returns
- the current list of plot objects
Definition at line 234 of file kplotwidget.cpp.
|
protected |
- Returns
- a list of points in the plot which are within 4 pixels of the screen position given as an argument.
- Parameters
-
p The screen position from which to check for plot points.
Definition at line 362 of file kplotwidget.cpp.
void KPlotWidget::removeAllPlotObjects | ( | ) |
Remove and delete all items from the list of KPlotObjects.
Definition at line 239 of file kplotwidget.cpp.
void KPlotWidget::replacePlotObject | ( | int | i, |
KPlotObject * | o | ||
) |
Replace an item in the KPlotObject list.
- Parameters
-
i the index of the item to be replaced o pointer to the replacement KPlotObject
Definition at line 272 of file kplotwidget.cpp.
void KPlotWidget::resetPlot | ( | ) |
Clear the object list, reset the data limits, and remove axis labels.
Definition at line 256 of file kplotwidget.cpp.
void KPlotWidget::resetPlotMask | ( | ) |
Reset the mask used for non-overlapping labels so that all regions of the plot area are considered empty.
Definition at line 249 of file kplotwidget.cpp.
|
protectedvirtual |
The resize event handler, called when the widget is resized.
Definition at line 392 of file kplotwidget.cpp.
int KPlotWidget::rightPadding | ( | ) | const |
- Returns
- the number of pixels to the right of the plot area. Padding values are set to -1 by default; if unchanged, this function will try to guess a good value, based on whether ticklabels and/or axis labels are to be drawn.
QRectF KPlotWidget::secondaryDataRect | ( | ) | const |
- Returns
- the rectangle representing the boundaries of the secondary data limits, if they have been set. Otherwise, this function behaves the same as dataRect().
- See also
- setSecondaryLimits()
Definition at line 205 of file kplotwidget.cpp.
void KPlotWidget::setAntialiasing | ( | bool | b | ) |
Toggle antialiased drawing.
- Parameters
-
b if true, the plot graphics will be antialiased.
Definition at line 328 of file kplotwidget.cpp.
void KPlotWidget::setBackgroundColor | ( | const QColor & | bg | ) |
Set the background color.
- Parameters
-
bg the new background color
Definition at line 296 of file kplotwidget.cpp.
void KPlotWidget::setBottomPadding | ( | int | padding | ) |
Set the number of pixels below the plot area.
Set this to -1 to revert to automatic determination of padding values.
Definition at line 959 of file kplotwidget.cpp.
void KPlotWidget::setDefaultPaddings | ( | ) |
Revert all four padding values to -1, so that they will be automatically determined.
Definition at line 964 of file kplotwidget.cpp.
void KPlotWidget::setForegroundColor | ( | const QColor & | fg | ) |
Set the foreground color.
- Parameters
-
fg the new foreground color
Definition at line 301 of file kplotwidget.cpp.
void KPlotWidget::setGridColor | ( | const QColor & | gc | ) |
void KPlotWidget::setLeftPadding | ( | int | padding | ) |
Set the number of pixels to the left of the plot area.
Set this to -1 to revert to automatic determination of padding values.
Definition at line 944 of file kplotwidget.cpp.
Set new data limits for the plot.
- Parameters
-
x1 the minimum X value in data units x2 the maximum X value in data units y1 the minimum Y value in data units y2 the maximum Y value in data units
Definition at line 135 of file kplotwidget.cpp.
|
slot |
Toggle the display of a tooltip for point objects.
- Parameters
-
show whether show the tooltip.
- See also
- isObjectToolTipShown()
Definition at line 339 of file kplotwidget.cpp.
|
protected |
Synchronize the PixRect with the current widget size and padding settings.
Definition at line 398 of file kplotwidget.cpp.
void KPlotWidget::setRightPadding | ( | int | padding | ) |
Set the number of pixels to the right of the plot area.
Set this to -1 to revert to automatic determination of padding values.
Definition at line 949 of file kplotwidget.cpp.
Reset the secondary data limits, which control the values displayed along the top and right axes.
All data points are plotted using the coordinates defined by setLimits(), so this function is only useful for showing alternate tickmark labels along the top and right edges. For example, if you were plotting temperature on the X-axis, you could use Centigrade units for the primary (bottom) axis, using setLimits( 0.0, 100.0, 0.0, 1.0 ). If you also wanted to show Farenheit units along the secondary (top) axis, you would additionally use setSecondaryLimits( 32.0, 212.0, 0.0, 1.0 ). The data added to the plot would have x-coordinates in Centigrade degrees.
- Parameters
-
x1 the minimum X value in secondary data units x2 the maximum X value in secondary data units y1 the minimum Y value in secondary data units y2 the maximum Y value in secondary data units
- See also
- setLimits()
Definition at line 169 of file kplotwidget.cpp.
|
slot |
Toggle whether grid lines are drawn at major tickmarks.
- Parameters
-
show if true, grid lines will be drawn.
- See also
- isGridShown()
Definition at line 334 of file kplotwidget.cpp.
void KPlotWidget::setTopPadding | ( | int | padding | ) |
Set the number of pixels above the plot area.
Set this to -1 to revert to automatic determination of padding values.
Definition at line 954 of file kplotwidget.cpp.
|
virtual |
- Returns
- suggested size for the plot widget
Definition at line 130 of file kplotwidget.cpp.
int KPlotWidget::topPadding | ( | ) | const |
- Returns
- the number of pixels above the plot area. Padding values are set to -1 by default; if unchanged, this function will try to guess a good value, based on whether ticklabels and/or axis labels are to be drawn.
Property Documentation
|
readwrite |
Definition at line 86 of file kplotwidget.h.
|
read |
Definition at line 85 of file kplotwidget.h.
|
readwrite |
Definition at line 87 of file kplotwidget.h.
|
readwrite |
Definition at line 89 of file kplotwidget.h.
|
readwrite |
Definition at line 88 of file kplotwidget.h.
|
read |
Definition at line 82 of file kplotwidget.h.
|
readwrite |
Definition at line 90 of file kplotwidget.h.
|
read |
Definition at line 83 of file kplotwidget.h.
|
read |
Definition at line 84 of file kplotwidget.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:49:18 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.