KDEUI
#include <kprogressdialog.h>
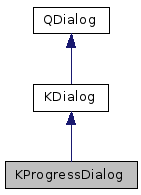
Protected Member Functions | |
virtual void | showEvent (QShowEvent *event) |
![]() | |
KDialog (KDialogPrivate &dd, QWidget *parent, Qt::WindowFlags flags=0) | |
virtual void | closeEvent (QCloseEvent *e) |
virtual void | hideEvent (QHideEvent *) |
virtual void | keyPressEvent (QKeyEvent *) |
Additional Inherited Members | |
![]() | |
enum | ButtonCode { None = 0x00000000, Help = 0x00000001, Default = 0x00000002, Ok = 0x00000004, Apply = 0x00000008, Try = 0x00000010, Cancel = 0x00000020, Close = 0x00000040, No = 0x00000080, Yes = 0x00000100, Reset = 0x00000200, Details = 0x00000400, User1 = 0x00001000, User2 = 0x00002000, User3 = 0x00004000, NoDefault = 0x00008000 } |
enum | ButtonPopupMode { InstantPopup = 0, DelayedPopup = 1 } |
enum | CaptionFlag { NoCaptionFlags = 0, AppNameCaption = 1, ModifiedCaption = 2, HIGCompliantCaption = AppNameCaption } |
![]() | |
void | delayedDestruct () |
void | enableButton (ButtonCode id, bool state) |
void | enableButtonApply (bool state) |
void | enableButtonCancel (bool state) |
void | enableButtonOk (bool state) |
void | enableLinkedHelp (bool state) |
bool | isDetailsWidgetVisible () const |
virtual void | setCaption (const QString &caption) |
virtual void | setCaption (const QString &caption, bool modified) |
void | setDetailsWidget (QWidget *detailsWidget) |
void | setDetailsWidgetVisible (bool visible) |
void | setHelp (const QString &anchor, const QString &appname=QString()) |
void | setHelpLinkText (const QString &text) |
virtual void | setPlainCaption (const QString &caption) |
![]() | |
void | aboutToShowDetails () |
void | applyClicked () |
void | buttonClicked (KDialog::ButtonCode button) |
void | cancelClicked () |
void | closeClicked () |
void | defaultClicked () |
void | finished () |
void | helpClicked () |
void | hidden () |
void | layoutHintChanged () |
void | noClicked () |
void | okClicked () |
void | resetClicked () |
void | tryClicked () |
void | user1Clicked () |
void | user2Clicked () |
void | user3Clicked () |
void | yesClicked () |
![]() | |
static bool | avoidArea (QWidget *widget, const QRect &area, int screen=-1) |
static void | centerOnScreen (QWidget *widget, int screen=-1) |
static int | groupSpacingHint () |
static QString | makeStandardCaption (const QString &userCaption, QWidget *window=0, CaptionFlags flags=HIGCompliantCaption) |
static int | marginHint () |
static void | resizeLayout (QWidget *widget, int margin, int spacing) |
static void | resizeLayout (QLayout *lay, int margin, int spacing) |
static void | setAllowEmbeddingInGraphicsView (bool allowEmbedding) |
static int | spacingHint () |
![]() | |
virtual void | slotButtonClicked (int button) |
void | updateGeometry () |
![]() | |
KDialogPrivate *const | d_ptr |
Detailed Description
A dialog with a progress bar.
KProgressDialog provides a dialog with a text label, a progress bar and an optional cancel button with a KDE look 'n feel.
Since knowing how long it can take to complete an action and it is undesirable to show a dialog for a split second before hiding it, there are a few ways to control the timing behavior of KProgressDialog. There is a time out that can be set before showing the dialog as well as an option to autohide or keep displaying the dialog once complete.
All the functionality of QProgressBar is available through direct access to the progress bar widget via progressBar();
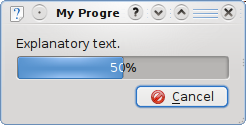
Definition at line 47 of file kprogressdialog.h.
Constructor & Destructor Documentation
|
explicit |
Constructs a KProgressDialog.
- Parameters
-
parent Parent of the widget caption Text to display in window title bar text Text to display in the dialog flags The widget flags
Definition at line 62 of file kprogressdialog.cpp.
KProgressDialog::~KProgressDialog | ( | ) |
Destructor.
Definition at line 93 of file kprogressdialog.cpp.
Member Function Documentation
bool KProgressDialog::allowCancel | ( | ) | const |
Returns true if the dialog can be canceled, false otherwise.
Definition at line 156 of file kprogressdialog.cpp.
bool KProgressDialog::autoClose | ( | ) | const |
Returns true if the dialog will close upon completion, or false otherwise.
Definition at line 186 of file kprogressdialog.cpp.
bool KProgressDialog::autoReset | ( | ) | const |
Returns true if the QProgressBar widget will be reset upon completion, or false otherwise.
Definition at line 196 of file kprogressdialog.cpp.
QString KProgressDialog::buttonText | ( | ) | const |
Returns the text on the cancel button.
Definition at line 212 of file kprogressdialog.cpp.
void KProgressDialog::ignoreCancel | ( | ) |
Ignores the last cancel action if the cancel button was pressed.
Useful for kdialog when combined with a KMessageBox to display a message like "Are you sure you want to cancel?"
Definition at line 129 of file kprogressdialog.cpp.
QString KProgressDialog::labelText | ( | ) | const |
Returns the current dialog text.
Definition at line 176 of file kprogressdialog.cpp.
int KProgressDialog::minimumDuration | ( | ) | const |
Returns the time that must pass before the dialog appears.
- See also
- setMinimumDuration
Definition at line 145 of file kprogressdialog.cpp.
QProgressBar * KProgressDialog::progressBar | ( | ) |
Returns the QProgressBar used in this dialog.
To set the number of steps or other progress bar related settings, access the QProgressBar object directly via this method.
Definition at line 161 of file kprogressdialog.cpp.
const QProgressBar * KProgressDialog::progressBar | ( | ) | const |
Returns the QProgressBar used in this dialog.
To set the number of steps or other progress bar related settings, access the QProgressBar object directly via this method.
Definition at line 166 of file kprogressdialog.cpp.
|
virtual |
Definition at line 114 of file kprogressdialog.cpp.
void KProgressDialog::setAllowCancel | ( | bool | allowCancel | ) |
Sets whether or not the user can cancel the process.
If the dialog is cancellable, the Cancel button will be shown and the user can close the window using the window decorations. If the process is not (or should not be) interuptable, set the dialog to be modal and not cancellable.
The default is true.
- Parameters
-
allowCancel Set to true to make the dialog non-closable
Definition at line 150 of file kprogressdialog.cpp.
void KProgressDialog::setAutoClose | ( | bool | close | ) |
Sets whether the dialog should close automagically when all the steps in the QProgressBar have been completed.
The default is true.
Definition at line 191 of file kprogressdialog.cpp.
void KProgressDialog::setAutoReset | ( | bool | autoReset | ) |
Sets whether the dialog should reset the QProgressBar dialog back to 0 steps compelete when all steps have been completed.
This is useful for KProgressDialogs that will be reused.
The default is false.
Definition at line 201 of file kprogressdialog.cpp.
void KProgressDialog::setButtonText | ( | const QString & | text | ) |
Sets the text to appear on the cancel button.
Definition at line 206 of file kprogressdialog.cpp.
void KProgressDialog::setLabelText | ( | const QString & | text | ) |
Sets the text in the dialog.
- Parameters
-
text the text to display
Definition at line 171 of file kprogressdialog.cpp.
void KProgressDialog::setMinimumDuration | ( | int | ms | ) |
Set the minimum number of milliseconds to wait before actually showing the dialog.
If the expected duration of the task is less than the minimumDuration, the dialog will not appear at all. This prevents the dialog popping up for tasks that are quickly over. For tasks that are expected to exceed the minimumDuration, the dialog will pop up after the minimumDuration time. If set to 0, the dialog is always shown immediately. The default is 2000 milliseconds.
Definition at line 134 of file kprogressdialog.cpp.
void KProgressDialog::showCancelButton | ( | bool | show | ) |
Sets whether the cancel button is visible.
setAllowCancel(false) implies showCancelButton(false)
The default is true.
- Parameters
-
show Whether or not the cancel button should be shown
Definition at line 181 of file kprogressdialog.cpp.
|
protectedvirtual |
Definition at line 108 of file kprogressdialog.cpp.
bool KProgressDialog::wasCancelled | ( | ) | const |
Returns true if the dialog was closed or canceled before completion.
If the dialog is not cancellable it will always return false.
Definition at line 124 of file kprogressdialog.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:49:18 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.