KDEUI
#include <kreplace.h>
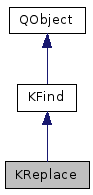
Signals | |
void | replace (const QString &text, int replacementIndex, int replacedLength, int matchedLength) |
![]() | |
void | dialogClosed () |
void | findNext () |
void | highlight (const QString &text, int matchingIndex, int matchedLength) |
void | highlight (int id, int matchingIndex, int matchedLength) |
void | optionsChanged () |
Public Member Functions | |
KReplace (const QString &pattern, const QString &replacement, long options, QWidget *parent=0) | |
KReplace (const QString &pattern, const QString &replacement, long options, QWidget *parent, QWidget *replaceDialog) | |
virtual | ~KReplace () |
void | closeReplaceNextDialog () |
virtual void | displayFinalDialog () const |
int | numReplacements () const |
Result | replace () |
KDialog * | replaceNextDialog (bool create=false) |
virtual void | resetCounts () |
virtual bool | shouldRestart (bool forceAsking=false, bool showNumMatches=true) const |
![]() | |
KFind (const QString &pattern, long options, QWidget *parent) | |
KFind (const QString &pattern, long options, QWidget *parent, QWidget *findDialog) | |
virtual | ~KFind () |
void | closeFindNextDialog () |
Result | find () |
KDialog * | findNextDialog (bool create=false) |
int | index () const |
bool | needData () const |
int | numMatches () const |
long | options () const |
QString | pattern () const |
void | setData (const QString &data, int startPos=-1) |
void | setData (int id, const QString &data, int startPos=-1) |
virtual void | setOptions (long options) |
void | setPattern (const QString &pattern) |
virtual bool | validateMatch (const QString &text, int index, int matchedlength) |
Static Public Member Functions | |
static int | replace (QString &text, const QString &pattern, const QString &replacement, int index, long options, int *replacedLength) |
static int | replace (QString &text, const QRegExp &pattern, const QString &replacement, int index, long options, int *replacedLength) |
![]() | |
static int | find (const QString &text, const QString &pattern, int index, long options, int *matchedlength) |
static int | find (const QString &text, const QRegExp &pattern, int index, long options, int *matchedlength) |
Additional Inherited Members | |
![]() | |
enum | Options { WholeWordsOnly = 1, FromCursor = 2, SelectedText = 4, CaseSensitive = 8, FindBackwards = 16, RegularExpression = 32, FindIncremental = 64, MinimumUserOption = 65536 } |
enum | Result { NoMatch, Match } |
![]() | |
QWidget * | dialogsParent () const |
QWidget * | parentWidget () const |
Detailed Description
A generic implementation of the "replace" function.
Detail:
This class includes prompt handling etc. Also provides some static functions which can be used to create custom behavior instead of using the class directly.
Example:
To use the class to implement a complete replace feature:
In the slot connect to the replace action, after using KReplaceDialog:
Then initialize the variables determining the "current position" (to the cursor, if the option FromCursor is set, to the beginning of the selection if the option SelectedText is set, and to the beginning of the document otherwise). Initialize the "end of search" variables as well (end of doc or end of selection). Swap begin and end if FindBackwards. Finally, call slotReplaceNext();
Don't forget delete m_find in the destructor of your class, unless you gave it a parent widget on construction.
Definition at line 96 of file kreplace.h.
Constructor & Destructor Documentation
KReplace::KReplace | ( | const QString & | pattern, |
const QString & | replacement, | ||
long | options, | ||
QWidget * | parent = 0 |
||
) |
Only use this constructor if you don't use KFindDialog, or if you use it as a modal dialog.
Definition at line 91 of file kreplace.cpp.
KReplace::KReplace | ( | const QString & | pattern, |
const QString & | replacement, | ||
long | options, | ||
QWidget * | parent, | ||
QWidget * | replaceDialog | ||
) |
This is the recommended constructor if you also use KReplaceDialog (non-modal).
You should pass the pointer to it here, so that when a message box appears it has the right parent. Don't worry about deletion, KReplace will notice if the find dialog is closed.
Definition at line 97 of file kreplace.cpp.
|
virtual |
Definition at line 103 of file kreplace.cpp.
Member Function Documentation
void KReplace::closeReplaceNextDialog | ( | ) |
Close the "replace next?" dialog.
The application should do this when the last match was hit. If the application deletes the KReplace, then "find previous" won't be possible anymore.
Definition at line 369 of file kreplace.cpp.
|
virtual |
Displays the final dialog telling the user how many replacements were made.
Call either this or shouldRestart().
Reimplemented from KFind.
Definition at line 133 of file kreplace.cpp.
int KReplace::numReplacements | ( | ) | const |
Return the number of replacements made (i.e.
the number of times the replace signal was emitted). Can be used in a dialog box to tell the user how many replacements were made. The final dialog does so already, unless you used setDisplayFinalDialog(false).
Definition at line 108 of file kreplace.cpp.
KFind::Result KReplace::replace | ( | ) |
Walk the text fragment (e.g.
kwrite line, kspread cell) looking for matches. For each match, if prompt-on-replace is specified, emits the highlight() signal and displays the prompt-for-replace dialog before doing the replace.
Definition at line 162 of file kreplace.cpp.
|
static |
Search the given string, replaces with the given replacement string, and returns whether a match was found.
If one is, the replacement string length is also returned.
A performance optimised version of the function is provided for use with regular expressions.
- Parameters
-
text The string to search. pattern The pattern to look for. replacement The replacement string to insert into the text. index The starting index into the string. options The options to use. replacedLength Output parameter, contains the length of the replaced string. Not always the same as replacement.length(), when backreferences are used.
- Returns
- The index at which a match was found, or -1 if no match was found.
Definition at line 234 of file kreplace.cpp.
|
static |
Definition at line 250 of file kreplace.cpp.
|
signal |
Connect to this slot to implement updating of replaced text during the replace operation.
Extra care must be taken to properly implement the "no prompt-on-replace" case. For instance highlight isn't emitted in that case (some code might rely on it), and for performance reasons one should repaint after replace() ONLY if prompt-on-replace was selected.
- Parameters
-
text The text, in which the replacement has already been done. replacementIndex Starting index of the matched substring replacedLength Length of the replacement string matchedLength Length of the matched string
Return (or create) the dialog that shows the "find next?" prompt.
Usually you don't need to call this. One case where it can be useful, is when the user selects the "Find" menu item while a find operation is under way. In that case, the program may want to call setActiveWindow() on that dialog.
Definition at line 113 of file kreplace.cpp.
|
virtual |
Call this to reset the numMatches & numReplacements counts.
Can be useful if reusing the same KReplace for different operations, or when restarting from the beginning of the document.
Reimplemented from KFind.
Definition at line 322 of file kreplace.cpp.
Returns true if we should restart the search from scratch.
Can ask the user, or return false (if we already searched/replaced the whole document without the PromptOnReplace option).
- Parameters
-
forceAsking set to true if the user modified the document during the search. In that case it makes sense to restart the search again. showNumMatches set to true if the dialog should show the number of matches. Set to false if the application provides a "find previous" action, in which case the match count will be erroneous when hitting the end, and we could even be hitting the beginning of the document (so not all matches have even been seen).
Reimplemented from KFind.
Definition at line 328 of file kreplace.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:49:18 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.