KDEUI
#include <ktabwidget.h>
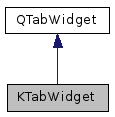
Public Slots | |
virtual void | moveTab (int, int) |
virtual QT_MOC_COMPAT void | removePage (QWidget *w) |
virtual void | removeTab (int index) |
void | setAutomaticResizeTabs (bool enable) |
QT_MOC_COMPAT void | setCloseButtonEnabled (bool) |
QT_MOC_COMPAT void | setHoverCloseButton (bool enable) |
QT_MOC_COMPAT void | setHoverCloseButtonDelayed (bool delayed) |
QT_MOC_COMPAT void | setTabCloseActivatePrevious (bool previous) |
QT_MOC_COMPAT void | setTabReorderingEnabled (bool enable) |
Signals | |
void | closeRequest (QWidget *) |
void | contextMenu (const QPoint &) |
void | contextMenu (QWidget *, const QPoint &) |
void | initiateDrag (QWidget *) |
void | mouseDoubleClick () |
void | mouseDoubleClick (QWidget *) |
void | mouseMiddleClick () |
void | mouseMiddleClick (QWidget *) |
void | movedTab (int, int) |
void | receivedDropEvent (QDropEvent *) |
void | receivedDropEvent (QWidget *, QDropEvent *) |
void | testCanDecode (const QDragMoveEvent *e, bool &accept) |
Public Member Functions | |
KTabWidget (QWidget *parent=0, Qt::WindowFlags flags=0) | |
virtual | ~KTabWidget () |
bool | automaticResizeTabs () const |
bool | hoverCloseButton () const |
bool | hoverCloseButtonDelayed () const |
bool | isCloseButtonEnabled () const |
bool | isTabBarHidden () const |
bool | isTabReorderingEnabled () const |
void | setTabBarHidden (bool hide) |
void | setTabText (int, const QString &) |
void | setTabTextColor (int index, const QColor &color) |
bool | tabCloseActivatePrevious () const |
QString | tabText (int) const |
QColor | tabTextColor (int index) const |
Protected Slots | |
virtual void | closeRequest (int) |
virtual void | contextMenu (int, const QPoint &) |
virtual void | initiateDrag (int) |
virtual void | mouseDoubleClick (int) |
virtual void | mouseMiddleClick (int) |
virtual void | receivedDropEvent (int, QDropEvent *) |
virtual void | wheelDelta (int) |
Protected Member Functions | |
void | currentChanged (int) |
virtual void | dragEnterEvent (QDragEnterEvent *) |
virtual void | dragMoveEvent (QDragMoveEvent *) |
virtual void | dropEvent (QDropEvent *) |
virtual void | mouseDoubleClickEvent (QMouseEvent *) |
virtual void | mousePressEvent (QMouseEvent *) |
virtual void | mouseReleaseEvent (QMouseEvent *) |
virtual void | resizeEvent (QResizeEvent *) |
int | tabBarWidthForMaxChars (int) |
virtual void | tabInserted (int) |
virtual void | tabRemoved (int) |
virtual void | wheelEvent (QWheelEvent *) |
Properties | |
bool | automaticResizeTabs |
bool | closeButtonEnabled |
bool | hoverCloseButton |
bool | hoverCloseButtonDelayed |
bool | tabCloseActivatePrevious |
bool | tabReorderingEnabled |
Detailed Description
A widget containing multiple tabs.
It extends the Qt QTabWidget, providing extra optionally features such as close buttons when you hover over the icon in the tab, and also adds functionality such as responding to mouse wheel scroll events to switch the active tab.
It is recommended to use KTabWidget instead of QTabWidget unless you have a good reason not to.
See also the QTabWidget documentation.
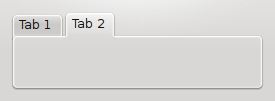
Definition at line 44 of file ktabwidget.h.
Constructor & Destructor Documentation
|
explicit |
Creates a new tab widget.
- Parameters
-
parent The parent widgets. flags The Qt window flags
- See also
- QWidget.
Definition at line 233 of file ktabwidget.cpp.
|
virtual |
Destroys the tab widget.
Definition at line 254 of file ktabwidget.cpp.
Member Function Documentation
bool KTabWidget::automaticResizeTabs | ( | ) | const |
Returns true if calling setTitle() will resize tabs to the width of the tab bar.
|
signal |
The close button of a widget's tab was clicked.
This signal is only possible after you have called setCloseButtonEnabled( true ).
|
protectedvirtualslot |
Definition at line 697 of file ktabwidget.cpp.
|
signal |
The right mouse button was pressed over empty space besides tabbar.
The right mouse button was pressed over a widget.
|
protectedvirtualslot |
Definition at line 541 of file ktabwidget.cpp.
|
protected |
- Deprecated:
- This method has no effect and should not be called
Definition at line 721 of file ktabwidget.cpp.
|
protectedvirtual |
Definition at line 428 of file ktabwidget.cpp.
|
protectedvirtual |
Definition at line 443 of file ktabwidget.cpp.
|
protectedvirtual |
Definition at line 458 of file ktabwidget.cpp.
bool KTabWidget::hoverCloseButton | ( | ) | const |
Returns true if the close button is shown on tabs when mouse is hovering over them.
- Deprecated:
- Use QTabWidget::tabsClosable() instead.
bool KTabWidget::hoverCloseButtonDelayed | ( | ) | const |
Returns true if the close button is shown on tabs after a delay.
- Deprecated:
- Use QTabWidget::setTabsClosable() instead.
|
signal |
Request to start a drag operation on the given tab.
|
protectedvirtualslot |
Definition at line 536 of file ktabwidget.cpp.
bool KTabWidget::isCloseButtonEnabled | ( | ) | const |
Returns true if the close button is shown on tabs.
- Since
- 4.1
- Deprecated:
- Use QTabWidget::tabsClosable() instead.
Definition at line 665 of file ktabwidget.cpp.
bool KTabWidget::isTabBarHidden | ( | ) | const |
Returns true if the tabbar was hidden by a call to setTabBarHidden( true ).
Returns false if the widget itself is hidden, but no call to setTabBarHidden( true ) has been made.
Definition at line 304 of file ktabwidget.cpp.
bool KTabWidget::isTabReorderingEnabled | ( | ) | const |
Returns true if tab ordering with the middle mouse button has been enabled.
- Deprecated:
- Use QTabWidget::isMovable() instead.
Definition at line 327 of file ktabwidget.cpp.
|
signal |
A double left mouse button click was performed over empty space besides tabbar.
The signal is emitted on the second press of the mouse button, before the release.
|
signal |
A double left mouse button click was performed over the widget.
The signal is emitted on the second press of the mouse button, before the release.
|
protectedvirtualslot |
Definition at line 546 of file ktabwidget.cpp.
|
protectedvirtual |
Definition at line 494 of file ktabwidget.cpp.
|
signal |
A middle mouse button click was performed over empty space besides tabbar.
The signal is emitted on the release of the mouse button.
|
signal |
A middle mouse button click was performed over the widget.
The signal is emitted on the release of the mouse button.
|
protectedvirtualslot |
Definition at line 551 of file ktabwidget.cpp.
|
protectedvirtual |
Definition at line 507 of file ktabwidget.cpp.
|
protectedvirtual |
Definition at line 519 of file ktabwidget.cpp.
|
signal |
A tab was moved from first to second index.
This signal is only possible after you have called setTabReorderingEnabled( true ).
|
virtualslot |
Move a widget's tab from first to second specified index and emit signal movedTab( int, int ) afterwards.
Definition at line 556 of file ktabwidget.cpp.
|
signal |
Received an event in the empty space beside tabbar.
Usually creates a new tab. This signal is only possible after testCanDecode and positive accept result.
|
signal |
Received an drop event on given widget's tab.
This signal is only possible after testCanDecode and positive accept result.
|
protectedvirtualslot |
Definition at line 531 of file ktabwidget.cpp.
|
virtualslot |
Removes the widget, reimplemented for internal reasons (keeping labels in sync).
- Deprecated:
- since 4.0
Definition at line 600 of file ktabwidget.cpp.
|
virtualslot |
Removes the widget, reimplemented for internal reasons (keeping labels in sync).
Definition at line 613 of file ktabwidget.cpp.
|
protectedvirtual |
Definition at line 702 of file ktabwidget.cpp.
|
slot |
If enable is true, tabs will be resized to the width of the tab bar.
Does not work reliably with "QTabWidget* foo=new KTabWidget()" and if you change tabs via the tabbar or by accessing tabs directly.
Definition at line 671 of file ktabwidget.cpp.
|
slot |
If enabled, a close button is available for each tab.
The signal KTabWidget::closeRequest() is emitted, if the close button has been clicked.
- Since
- 4.1
- Deprecated:
- Use QTabWidget::setTabsClosable() instead.
Definition at line 658 of file ktabwidget.cpp.
|
slot |
If enable is true, a close button will be shown on mouse hover over tab icons which will emit signal closeRequest( QWidget * ) when pressed.
- Deprecated:
- Use QTabWidget::setTabsClosable() instead.
Definition at line 626 of file ktabwidget.cpp.
|
slot |
If delayed is true, a close button will be shown on mouse hover over tab icons after mouse double click delay else immediately.
- Deprecated:
- Use QTabWidget::setTabsClosable() instead.
Definition at line 642 of file ktabwidget.cpp.
void KTabWidget::setTabBarHidden | ( | bool | hide | ) |
If hide is true, the tabbar is hidden along with any corner widgets.
Definition at line 284 of file ktabwidget.cpp.
|
slot |
If previous is true, closing the current tab activates the previous active tab instead of the one to the right.
- Deprecated:
- Use tabBar()->setSelectionBehaviorOnRemove() instead.
Definition at line 334 of file ktabwidget.cpp.
|
slot |
If enable is true, tab reordering with middle button will be enabled.
Note that once enabled you shouldn't rely on previously queried currentPageIndex() or indexOf( QWidget * ) values anymore.
You can connect to signal movedTab(int, int) which will notify you from which index to which index a tab has been moved.
- Deprecated:
- Use QTabWidget::setMovable() instead.
Note, however, that QTabWidget::setMovable(true) disables dragging tabs out of the KTabBar (e.g., dragging the tab URL from Konqueror to another application)!
Definition at line 320 of file ktabwidget.cpp.
void KTabWidget::setTabText | ( | int | index, |
const QString & | text | ||
) |
Reimplemented for internal reasons.
Definition at line 398 of file ktabwidget.cpp.
void KTabWidget::setTabTextColor | ( | int | index, |
const QColor & | color | ||
) |
Set the tab of the given widget to color.
This is simply a convenience method for QTabBar::setTabTextColor.
Definition at line 309 of file ktabwidget.cpp.
|
protected |
Definition at line 347 of file ktabwidget.cpp.
bool KTabWidget::tabCloseActivatePrevious | ( | ) | const |
Returns true if closing the current tab activates the previous actice tab instead of the one to the right.
- Deprecated:
- Use tabBar()->selectionBehaviorOnRemove() instead.
|
protectedvirtual |
Definition at line 708 of file ktabwidget.cpp.
|
protectedvirtual |
Definition at line 713 of file ktabwidget.cpp.
QString KTabWidget::tabText | ( | int | index | ) | const |
Reimplemented for internal reasons.
virtual void insertTab( QWidget *, const QString &, int index = -1 );
! Reimplemented for internal reasons.
virtual void insertTab( QWidget *child, const QIcon& iconset, const QString &label, int index = -1 ); ! Reimplemented for internal reasons.
virtual void insertTab( QWidget *, QTab *, int index = -1 ); Reimplemented for internal reasons.
Definition at line 378 of file ktabwidget.cpp.
QColor KTabWidget::tabTextColor | ( | int | index | ) | const |
Returns the tab color for the given widget.
This is simply a convenience method for QTabBar::tabTextColor.
Definition at line 314 of file ktabwidget.cpp.
|
signal |
Connect to this and set accept to true if you can and want to decode the event.
|
protectedvirtualslot |
Definition at line 477 of file ktabwidget.cpp.
|
protectedvirtual |
Definition at line 469 of file ktabwidget.cpp.
Property Documentation
|
readwrite |
Definition at line 54 of file ktabwidget.h.
|
readwrite |
Definition at line 51 of file ktabwidget.h.
|
readwrite |
Definition at line 49 of file ktabwidget.h.
|
readwrite |
Definition at line 50 of file ktabwidget.h.
|
readwrite |
Definition at line 52 of file ktabwidget.h.
|
readwrite |
Definition at line 48 of file ktabwidget.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:49:18 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.