KDEUI
#include <ktimecombobox.h>
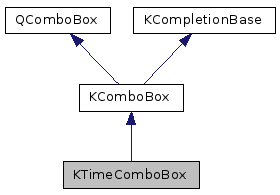
Public Types | |
enum | Option { EditTime = 0x0001, SelectTime = 0x0002, ForceTime = 0x0004, WarnOnInvalid = 0x0008 } |
![]() | |
typedef QMap< KeyBindingType, KShortcut > | KeyBindingMap |
enum | KeyBindingType { TextCompletion, PrevCompletionMatch, NextCompletionMatch, SubstringCompletion } |
Public Slots | |
void | setDisplayFormat (KLocale::TimeFormatOptions formatOptions) |
void | setMaximumTime (const QTime &maxTime, const QString &maxWarnMsg=QString()) |
void | setMinimumTime (const QTime &minTime, const QString &minWarnMsg=QString()) |
void | setOptions (Options options) |
void | setTime (const QTime &time) |
void | setTimeList (QList< QTime > timeList, const QString &minWarnMsg=QString(), const QString &maxWarnMsg=QString()) |
void | setTimeListInterval (int minutes) |
![]() | |
void | rotateText (KCompletionBase::KeyBindingType type) |
void | setCompletedItems (const QStringList &items, bool autosubject=true) |
virtual void | setCompletedText (const QString &) |
void | setCurrentItem (const QString &item, bool insert=false, int index=-1) |
Signals | |
void | timeChanged (const QTime &time) |
void | timeEdited (const QTime &time) |
void | timeEntered (const QTime &time) |
![]() | |
void | aboutToShowContextMenu (QMenu *p) |
void | completion (const QString &) |
void | completionModeChanged (KGlobalSettings::Completion) |
void | returnPressed () |
void | returnPressed (const QString &) |
void | substringCompletion (const QString &) |
void | textRotation (KCompletionBase::KeyBindingType) |
Public Member Functions | |
KTimeComboBox (QWidget *parent=0) | |
virtual | ~KTimeComboBox () |
KLocale::TimeFormatOptions | displayFormat () const |
bool | isNull () const |
bool | isValid () const |
QTime | maximumTime () const |
QTime | minimumTime () const |
Options | options () const |
void | resetMaximumTime () |
void | resetMinimumTime () |
void | resetTimeRange () |
void | setTimeRange (const QTime &minTime, const QTime &maxTime, const QString &minWarnMsg=QString(), const QString &maxWarnMsg=QString()) |
QTime | time () const |
QList< QTime > | timeList () const |
int | timeListInterval () const |
![]() | |
KComboBox (QWidget *parent=0) | |
KComboBox (bool rw, QWidget *parent=0) | |
virtual | ~KComboBox () |
void | addUrl (const KUrl &url) |
void | addUrl (const QIcon &icon, const KUrl &url) |
bool | autoCompletion () const |
void | changeURL (const KUrl &url, int index) |
void | changeURL (const QPixmap &pixmap, const KUrl &url, int index) |
void | changeUrl (int index, const KUrl &url) |
void | changeUrl (int index, const QIcon &icon, const KUrl &url) |
KCompletionBox * | completionBox (bool create=true) |
bool | contains (const QString &text) const |
int | cursorPosition () const |
void | insertURL (const KUrl &url, int index=-1) |
void | insertURL (const QPixmap &pixmap, const KUrl &url, int index=-1) |
void | insertUrl (int index, const KUrl &url) |
void | insertUrl (int index, const QIcon &icon, const KUrl &url) |
virtual void | setAutoCompletion (bool autocomplete) |
virtual void | setContextMenuEnabled (bool showMenu) |
void | setEditable (bool editable) |
void | setEditUrl (const KUrl &url) |
virtual void | setLineEdit (QLineEdit *) |
void | setTrapReturnKey (bool trap) |
void | setUrlDropsEnabled (bool enable) |
bool | trapReturnKey () const |
bool | urlDropsEnabled () const |
![]() | |
KCompletionBase () | |
virtual | ~KCompletionBase () |
KGlobalSettings::Completion | completionMode () const |
KCompletion * | completionObject (bool hsig=true) |
KCompletion * | compObj () const |
bool | emitSignals () const |
KShortcut | getKeyBinding (KeyBindingType item) const |
bool | handleSignals () const |
bool | isCompletionObjectAutoDeleted () const |
void | setAutoDeleteCompletionObject (bool autoDelete) |
virtual void | setCompletedItems (const QStringList &items, bool autoSuggest=true)=0 |
virtual void | setCompletedText (const QString &text)=0 |
virtual void | setCompletionMode (KGlobalSettings::Completion mode) |
virtual void | setCompletionObject (KCompletion *compObj, bool hsig=true) |
void | setEnableSignals (bool enable) |
virtual void | setHandleSignals (bool handle) |
bool | setKeyBinding (KeyBindingType item, const KShortcut &key) |
void | useGlobalKeyBindings () |
Protected Member Functions | |
virtual void | assignTime (const QTime &time) |
virtual bool | eventFilter (QObject *object, QEvent *event) |
virtual void | focusInEvent (QFocusEvent *event) |
virtual void | focusOutEvent (QFocusEvent *event) |
virtual void | hidePopup () |
virtual void | keyPressEvent (QKeyEvent *event) |
virtual void | mousePressEvent (QMouseEvent *event) |
virtual void | resizeEvent (QResizeEvent *event) |
virtual void | showPopup () |
virtual void | wheelEvent (QWheelEvent *event) |
![]() | |
virtual void | create (WId=0, bool initializeWindow=true, bool destroyOldWindow=true) |
virtual QSize | minimumSizeHint () const |
virtual void | setCompletedText (const QString &, bool) |
![]() | |
KCompletionBase * | delegate () const |
KeyBindingMap | getKeyBindings () const |
void | setDelegate (KCompletionBase *delegate) |
virtual void | virtual_hook (int id, void *data) |
Properties | |
QTime | maximumTime |
QTime | minimumTime |
Options | options |
QTime | time |
int | timeListInterval |
![]() | |
bool | autoCompletion |
bool | trapReturnKey |
bool | urlDropsEnabled |
Additional Inherited Members | |
![]() | |
virtual void | makeCompletion (const QString &) |
Detailed Description
Definition at line 32 of file ktimecombobox.h.
Member Enumeration Documentation
Options provided by the widget.
- See also
- options
- setOptions
Definition at line 50 of file ktimecombobox.h.
Constructor & Destructor Documentation
|
explicit |
Create a new KTimeComboBox widget.
Definition at line 280 of file ktimecombobox.cpp.
|
virtual |
Destroy the widget.
Definition at line 296 of file ktimecombobox.cpp.
Member Function Documentation
|
protectedvirtual |
Assign the time for the widget.
Virtual to allow sub-classes to apply extra validation rules.
- Parameters
-
time the new time
Definition at line 323 of file ktimecombobox.cpp.
KLocale::TimeFormatOptions KTimeComboBox::displayFormat | ( | ) | const |
Return the currently set time format.
By default this is the Short Time
- Returns
- the currently set time format
Definition at line 408 of file ktimecombobox.cpp.
Re-implemented for internal reasons.
API not affected.
Reimplemented from KComboBox.
Definition at line 474 of file ktimecombobox.cpp.
|
protectedvirtual |
Definition at line 531 of file ktimecombobox.cpp.
|
protectedvirtual |
Definition at line 504 of file ktimecombobox.cpp.
|
protectedvirtual |
Definition at line 516 of file ktimecombobox.cpp.
bool KTimeComboBox::isNull | ( | ) | const |
Return if the current user input is null.
- See also
- isValid()
- Returns
- if the current user input is null
Definition at line 336 of file ktimecombobox.cpp.
bool KTimeComboBox::isValid | ( | ) | const |
Return if the current user input is valid.
If the user input is null then it is not valid
- See also
- isNull()
- Returns
- if the current user input is valid
Definition at line 328 of file ktimecombobox.cpp.
|
protectedvirtual |
Definition at line 479 of file ktimecombobox.cpp.
QTime KTimeComboBox::maximumTime | ( | ) | const |
Return the current maximum time.
- Returns
- the current maximum time
QTime KTimeComboBox::minimumTime | ( | ) | const |
Return the current minimum time.
- Returns
- the current minimum time
|
protectedvirtual |
Definition at line 521 of file ktimecombobox.cpp.
Options KTimeComboBox::options | ( | ) | const |
Return the currently set widget options.
- Returns
- the currently set widget options
void KTimeComboBox::resetMaximumTime | ( | ) |
Reset the maximum time to the default of 23:59:59.999.
Definition at line 380 of file ktimecombobox.cpp.
void KTimeComboBox::resetMinimumTime | ( | ) |
Reset the minimum time to the default of 00:00:00.000.
Definition at line 365 of file ktimecombobox.cpp.
void KTimeComboBox::resetTimeRange | ( | ) |
Reset the minimum and maximum time to the default values.
Definition at line 403 of file ktimecombobox.cpp.
|
protectedvirtual |
Definition at line 536 of file ktimecombobox.cpp.
|
slot |
Sets the time format to display.
By default is the Short Time format.
- Parameters
-
format the time format to use
Definition at line 413 of file ktimecombobox.cpp.
|
slot |
Set the maximum allowed time.
If the time is invalid, or less than current minimum, then the maximum will not be set.
- Parameters
-
maxTime the maximum time maxWarnMsg the maximum warning message
Definition at line 375 of file ktimecombobox.cpp.
|
slot |
Set the minimum allowed time.
If the time is invalid, or greater than current maximum, then the minimum will not be set.
- Parameters
-
minTime the minimum time minWarnMsg the minimum warning message
Definition at line 360 of file ktimecombobox.cpp.
|
slot |
Set the new widget options.
- Parameters
-
options the new widget options
Definition at line 346 of file ktimecombobox.cpp.
|
slot |
Set the currently selected time.
You can set an invalid time or a time outside the valid range, validity checking is only done via isValid().
- Parameters
-
time the new time
Definition at line 307 of file ktimecombobox.cpp.
|
slot |
Set the list of times able to be selected from the drop-down.
Setting the time list will override any time interval previously set via setTimeListInterval().
Any invalid or duplicate times will be ignored, and the list will be sorted.
The minimum and maximum time will automatically be set to the earliest and latest value in the list.
- See also
- timeList()
- Parameters
-
timeList the list of times able to be selected minWarnMsg the minimum warning message maxWarnMsg the maximum warning message
Definition at line 457 of file ktimecombobox.cpp.
|
slot |
Set the interval between times able to be selected from the drop-down.
The combo drop-down will be populated with times every
- Parameters
-
minutes apart, starting from the minimumTime() and ending at maximumTime().
If the ForceInterval option is set then any time manually typed into the combo line edit will be forced to the nearest interval.
This interval must be an exact divisor of the valid time range hours. For example with the default 24 hour range interval
must divide 1440 minutes exactly, meaning 1, 6 and 90 are valid but 7, 31 and 91 are not.
Setting the time list interval will override any time list previously set via setTimeList().
- See also
- timeListInterval()
- Parameters
-
minutes the time list interval to display
Definition at line 427 of file ktimecombobox.cpp.
void KTimeComboBox::setTimeRange | ( | const QTime & | minTime, |
const QTime & | maxTime, | ||
const QString & | minWarnMsg = QString() , |
||
const QString & | maxWarnMsg = QString() |
||
) |
Set the minimum and maximum time range.
If either time is invalid, or min > max then the range will not be set.
- Parameters
-
minTime the minimum time maxTime the maximum time minWarnMsg the minimum warning message maxWarnMsg the maximum warning message
Definition at line 385 of file ktimecombobox.cpp.
|
protectedvirtual |
Definition at line 511 of file ktimecombobox.cpp.
QTime KTimeComboBox::time | ( | ) | const |
Return the currently selected time.
- Returns
- the currently selected time
|
signal |
Signal if the time has been changed either manually by the user or programatically.
The returned time may be invalid.
- Parameters
-
time the new time
|
signal |
Signal if the time is being manually edited by the user.
The returned time may be invalid.
- Parameters
-
time the new time
|
signal |
Signal if the time has been manually entered or selected by the user.
The returned time may be invalid.
- Parameters
-
time the new time
QList< QTime > KTimeComboBox::timeList | ( | ) | const |
Return the list of times able to be selected in the drop-down.
- Returns
- the select time list
Definition at line 446 of file ktimecombobox.cpp.
int KTimeComboBox::timeListInterval | ( | ) | const |
Return the interval between select time list entries if set by setTimeListInterval().
Returns -1 if not set.
- See also
- setTimeListInterval()
- Returns
- the select time list interval in minutes
|
protectedvirtual |
Reimplemented from KComboBox.
Definition at line 526 of file ktimecombobox.cpp.
Property Documentation
|
readwrite |
Definition at line 38 of file ktimecombobox.h.
|
readwrite |
Definition at line 37 of file ktimecombobox.h.
|
readwrite |
Definition at line 40 of file ktimecombobox.h.
|
readwrite |
Definition at line 36 of file ktimecombobox.h.
|
readwrite |
Definition at line 39 of file ktimecombobox.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:49:18 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.