KDEUI
#include <kxmlguiwindow.h>
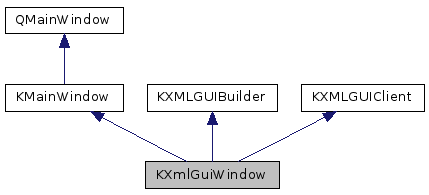
Public Types | |
enum | StandardWindowOption { ToolBar = 1, Keys = 2, StatusBar = 4, Save = 8, Create = 16, Default = ToolBar | Keys | StatusBar | Save | Create } |
![]() | |
enum | ReverseStateChange { StateNoReverse, StateReverse } |
Public Slots | |
virtual void | configureToolbars () |
virtual void | slotStateChanged (const QString &newstate) |
void | slotStateChanged (const QString &newstate, bool reverse) |
![]() | |
void | appHelpActivated (void) |
virtual void | setCaption (const QString &caption) |
virtual void | setCaption (const QString &caption, bool modified) |
virtual void | setPlainCaption (const QString &caption) |
void | setSettingsDirty () |
Protected Slots | |
virtual void | saveNewToolbarConfig () |
![]() | |
void | saveAutoSaveSettings () |
virtual void | showAboutApplication () |
Protected Member Functions | |
virtual bool | event (QEvent *event) |
![]() | |
KMainWindow (KMainWindowPrivate &dd, QWidget *parent, Qt::WindowFlags f) | |
virtual void | closeEvent (QCloseEvent *) |
void | parseGeometry (bool parsewidth) |
virtual bool | queryClose () |
virtual bool | queryExit () |
virtual void | readGlobalProperties (KConfig *sessionConfig) |
virtual void | readProperties (const KConfigGroup &) |
bool | readPropertiesInternal (KConfig *, int) |
void | restoreWindowSize (const KConfigGroup &config) |
virtual void | saveGlobalProperties (KConfig *sessionConfig) |
virtual void | saveProperties (KConfigGroup &) |
void | savePropertiesInternal (KConfig *, int) |
void | saveWindowSize (const KConfigGroup &config) const |
bool | settingsDirty () const |
![]() | |
virtual void | virtual_hook (int id, void *data) |
![]() | |
void | loadStandardsXmlFile () |
virtual void | setComponentData (const KComponentData &componentData) |
virtual void | setDOMDocument (const QDomDocument &document, bool merge=false) |
virtual void | setLocalXMLFile (const QString &file) |
virtual void | setXML (const QString &document, bool merge=false) |
virtual void | setXMLFile (const QString &file, bool merge=false, bool setXMLDoc=true) |
virtual void | stateChanged (const QString &newstate, ReverseStateChange reverse=StateNoReverse) |
virtual void | virtual_hook (int id, void *data) |
Properties | |
QString | autoSaveGroup |
bool | autoSaveSettings |
bool | hasMenuBar |
bool | initialGeometrySet |
bool | standardToolBarMenuEnabled |
![]() | |
QString | autoSaveGroup |
bool | autoSaveSettings |
bool | hasMenuBar |
bool | initialGeometrySet |
Additional Inherited Members | |
![]() | |
static bool | canBeRestored (int number) |
static const QString | classNameOfToplevel (int number) |
static QList< KMainWindow * > | memberList () |
![]() | |
static QString | findMostRecentXMLFile (const QStringList &files, QString &doc) |
![]() | |
KMainWindowPrivate *const | k_ptr |
Detailed Description
KDE top level main window with predefined action layout
Instead of creating a KMainWindow manually and assigning menus, menu entries, toolbar buttons and actions to it by hand, this class can be used to load an rc file to manage the main window's actions.
See http://techbase.kde.org/Development/Tutorials/Using_KActions#XMLGUI for essential information on the XML file format and usage of this class.
- See also
- KMainWindow
Definition at line 61 of file kxmlguiwindow.h.
Member Enumeration Documentation
- See also
- setupGUI()
Enumerator | |
---|---|
ToolBar |
adds action to show/hide the toolbar(s) and adds action to configure the toolbar(s).
|
Keys |
adds action to show the key configure action. |
StatusBar |
adds action to show/hide the statusbar if the statusbar exists.
|
Save |
auto-saves (and loads) the toolbar/menubar/statusbar settings and window size using the default name.
Typically you want to let the default window size be determined by the widgets size hints. Make sure that setupGUI() is called after all the widgets are created ( including setCentralWidget ) so the default size's will be correct.
|
Create |
calls createGUI() once ToolBar, Keys and Statusbar have been taken care of.
NOTE: when using KParts::MainWindow, remove this flag from the setupGUI call, since you'll be using createGUI(part) instead. |
Default |
All the above option (this is the default) |
Definition at line 192 of file kxmlguiwindow.h.
Constructor & Destructor Documentation
|
explicit |
Construct a main window.
- Parameters
-
parent The widget parent. This is usually 0 but it may also be the window group leader. In that case, the KMainWindow becomes sort of a secondary window. f Specify the widget flags. The default is Qt::Window and Qt::WA_DeleteOnClose. Qt::Window indicates that a main window is a toplevel window, regardless of whether it has a parent or not. Qt::WA_DeleteOnClose indicates that a main window is automatically destroyed when its window is closed. Pass 0 if you do not want this behavior.
KMainWindows must be created on the heap with 'new', like:
IMPORTANT: For session management and window management to work properly, all main windows in the application should have a different name. If you don't do it, KMainWindow will create a unique name, but it's recommended to explicitly pass a window name that will also describe the type of the window. If there can be several windows of the same type, append '#' (hash) to the name, and KMainWindow will replace it with numbers to make the names unique. For example, for a mail client which has one main window showing the mails and folders, and which can also have one or more windows for composing mails, the name for the folders window should be e.g. "mainwindow" and for the composer windows "composer#".
Definition at line 88 of file kxmlguiwindow.cpp.
|
virtual |
Destructor.
Will also destroy the toolbars, and menubar if needed.
Definition at line 118 of file kxmlguiwindow.cpp.
Member Function Documentation
|
virtual |
Read settings for statusbar, menubar and toolbar from their respective groups in the config file and apply them.
- Parameters
-
config Config group to read the settings from. forceGlobal see the same argument in KToolBar::applySettings
Reimplemented from KMainWindow.
Definition at line 348 of file kxmlguiwindow.cpp.
|
virtualslot |
Show a standard configure toolbar dialog.
This slot can be connected directly to the action to configure toolbar. This is very simple to do that by adding a single line
Definition at line 161 of file kxmlguiwindow.cpp.
Create a GUI given a local XML file.
In a regular app you usually want to use setupGUI() instead of this one since it does more things for free like setting up the toolbar/shortcut edit actions, etc.
If xmlfile
is NULL, then it will try to construct a local XML filename like appnameui.rc where 'appname' is your app's name. If that file does not exist, then the XML UI code will only use the global (standard) XML file for the layout purposes.
- Parameters
-
xmlfile The local xmlfile (relative or absolute)
Definition at line 232 of file kxmlguiwindow.cpp.
void KXmlGuiWindow::createStandardStatusBarAction | ( | ) |
Sets whether KMainWindow should provide a menu that allows showing/hiding of the statusbar ( using KToggleStatusBarAction ).
The menu / menu item is implemented using xmlgui. It will be inserted in your menu structure in the 'Settings' menu.
Note that you should enable this feature before calling createGUI() ( or similar ).
If an application maintains the action on its own (i.e. never calls this function) a connection needs to be made to let KMainWindow know when that status (hidden/shown) of the statusbar has changed. For example: connect(action, SIGNAL(activated()), kmainwindow, SLOT(setSettingsDirty())); Otherwise the status (hidden/show) of the statusbar might not be saved by KMainWindow.
Definition at line 321 of file kxmlguiwindow.cpp.
|
protectedvirtual |
Reimplemented to catch QEvent::Polish in order to adjust the object name if needed, once all constructor code for the main window has run.
Also reimplemented to catch when a QDockWidget is added or removed.
Reimplemented from KMainWindow.
Definition at line 124 of file kxmlguiwindow.cpp.
|
virtual |
Reimplemented from KXMLGUIBuilder.
Definition at line 359 of file kxmlguiwindow.cpp.
void KXmlGuiWindow::finalizeGUI | ( | bool | force | ) |
Definition at line 337 of file kxmlguiwindow.cpp.
|
virtual |
Definition at line 150 of file kxmlguiwindow.cpp.
bool KXmlGuiWindow::isHelpMenuEnabled | ( | ) | const |
Return true
when the help menu is enabled.
Definition at line 144 of file kxmlguiwindow.cpp.
bool KXmlGuiWindow::isStandardToolBarMenuEnabled | ( | ) | const |
Definition at line 315 of file kxmlguiwindow.cpp.
|
protectedvirtualslot |
Rebuilds the GUI after KEditToolbar changed the toolbar layout.
- See also
- configureToolbars()
Definition at line 174 of file kxmlguiwindow.cpp.
void KXmlGuiWindow::setHelpMenuEnabled | ( | bool | showHelpMenu = true | ) |
Enables the build of a standard help menu when calling createGUI/setupGUI().
The default behavior is to build one, you must call this function to disable it
Definition at line 138 of file kxmlguiwindow.cpp.
void KXmlGuiWindow::setStandardToolBarMenuEnabled | ( | bool | enable | ) |
Sets whether KMainWindow should provide a menu that allows showing/hiding the available toolbars ( using KToggleToolBarAction ) .
In case there is only one toolbar configured a simple 'Show <toolbar name here>' menu item is shown.
The menu / menu item is implemented using xmlgui. It will be inserted in your menu structure in the 'Settings' menu.
If your application uses a non-standard xmlgui resource file then you can specify the exact position of the menu / menu item by adding a <Merge name="StandardToolBarMenuHandler" /> line to the settings menu section of your resource file ( usually appname.rc ).
Note that you should enable this feature before calling createGUI() ( or similar ) .
Definition at line 292 of file kxmlguiwindow.cpp.
void KXmlGuiWindow::setupGUI | ( | StandardWindowOptions | options = Default , |
const QString & | xmlfile = QString() |
||
) |
Configures the current windows and its actions in the typical KDE fashion.
The options are all enabled by default but can be turned off if desired through the params or if the prereqs don't exists.
Typically this function replaces createGUI().
- See also
- StandardWindowOptions
- Note
- Since this method will restore the state of the application (toolbar, dockwindows positions...), you need to have added all your actions to your toolbars etc before calling to this method. (This note is only applicable if you are using the Default or Save flag).
- Warning
- If you are calling createGUI yourself, remember to remove the Create flag from the
options
parameter.
Definition at line 185 of file kxmlguiwindow.cpp.
void KXmlGuiWindow::setupGUI | ( | const QSize & | defaultSize, |
StandardWindowOptions | options = Default , |
||
const QString & | xmlfile = QString() |
||
) |
Configures the current windows and its actions in the typical KDE fashion.
The options are all enabled by default but can be turned off if desired through the params or if the prereqs don't exists.
defaultSize
The default size of the window
Typically this function replaces createGUI().
- See also
- StandardWindowOptions
- Note
- Since this method will restore the state of the application (toolbar, dockwindows positions...), you need to have added all your actions to your toolbars etc before calling to this method. (This note is only applicable if you are using the Default or Save flag).
- Warning
- If you are calling createGUI yourself, remember to remove the Create flag from the
options
parameter. Also, call setupGUI always after you call createGUI.
Definition at line 189 of file kxmlguiwindow.cpp.
void KXmlGuiWindow::setupToolbarMenuActions | ( | ) |
for KToolBar
Definition at line 110 of file kxmlguiwindow.cpp.
|
virtualslot |
Apply a state change.
Enable and disable actions as defined in the XML rc file
Definition at line 280 of file kxmlguiwindow.cpp.
Apply a state change.
Enable and disable actions as defined in the XML rc file, can "reverse" the state (disable the actions which should be enabled, and vice-versa) if specified.
Definition at line 285 of file kxmlguiwindow.cpp.
QAction * KXmlGuiWindow::toolBarMenuAction | ( | ) |
Returns a pointer to the mainwindows action responsible for the toolbars menu.
Definition at line 100 of file kxmlguiwindow.cpp.
Property Documentation
|
read |
Definition at line 67 of file kxmlguiwindow.h.
|
read |
Definition at line 66 of file kxmlguiwindow.h.
|
read |
Definition at line 65 of file kxmlguiwindow.h.
|
read |
Definition at line 70 of file kxmlguiwindow.h.
|
readwrite |
Definition at line 68 of file kxmlguiwindow.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:49:18 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.