KDEUI
#include <dialog.h>
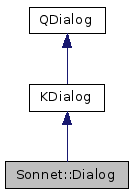
Public Slots | |
void | setBuffer (const QString &) |
![]() | |
void | delayedDestruct () |
void | enableButton (ButtonCode id, bool state) |
void | enableButtonApply (bool state) |
void | enableButtonCancel (bool state) |
void | enableButtonOk (bool state) |
void | enableLinkedHelp (bool state) |
bool | isDetailsWidgetVisible () const |
virtual void | setCaption (const QString &caption) |
virtual void | setCaption (const QString &caption, bool modified) |
void | setDetailsWidget (QWidget *detailsWidget) |
void | setDetailsWidgetVisible (bool visible) |
void | setHelp (const QString &anchor, const QString &appname=QString()) |
void | setHelpLinkText (const QString &text) |
virtual void | setPlainCaption (const QString &caption) |
Signals | |
void | autoCorrect (const QString ¤tWord, const QString &replaceWord) |
void | cancel () |
void | done (const QString &newBuffer) |
void | languageChanged (const QString &language) |
void | misspelling (const QString &word, int start) |
void | replace (const QString &oldWord, int start, const QString &newWord) |
void | spellCheckStatus (const QString &) |
void | stop () |
![]() | |
void | aboutToShowDetails () |
void | applyClicked () |
void | buttonClicked (KDialog::ButtonCode button) |
void | cancelClicked () |
void | closeClicked () |
void | defaultClicked () |
void | finished () |
void | helpClicked () |
void | hidden () |
void | layoutHintChanged () |
void | noClicked () |
void | okClicked () |
void | resetClicked () |
void | tryClicked () |
void | user1Clicked () |
void | user2Clicked () |
void | user3Clicked () |
void | yesClicked () |
Additional Inherited Members | |
![]() | |
enum | ButtonCode { None = 0x00000000, Help = 0x00000001, Default = 0x00000002, Ok = 0x00000004, Apply = 0x00000008, Try = 0x00000010, Cancel = 0x00000020, Close = 0x00000040, No = 0x00000080, Yes = 0x00000100, Reset = 0x00000200, Details = 0x00000400, User1 = 0x00001000, User2 = 0x00002000, User3 = 0x00004000, NoDefault = 0x00008000 } |
enum | ButtonPopupMode { InstantPopup = 0, DelayedPopup = 1 } |
enum | CaptionFlag { NoCaptionFlags = 0, AppNameCaption = 1, ModifiedCaption = 2, HIGCompliantCaption = AppNameCaption } |
![]() | |
static bool | avoidArea (QWidget *widget, const QRect &area, int screen=-1) |
static void | centerOnScreen (QWidget *widget, int screen=-1) |
static int | groupSpacingHint () |
static QString | makeStandardCaption (const QString &userCaption, QWidget *window=0, CaptionFlags flags=HIGCompliantCaption) |
static int | marginHint () |
static void | resizeLayout (QWidget *widget, int margin, int spacing) |
static void | resizeLayout (QLayout *lay, int margin, int spacing) |
static void | setAllowEmbeddingInGraphicsView (bool allowEmbedding) |
static int | spacingHint () |
![]() | |
virtual void | slotButtonClicked (int button) |
void | updateGeometry () |
![]() | |
KDialog (KDialogPrivate &dd, QWidget *parent, Qt::WindowFlags flags=0) | |
virtual void | closeEvent (QCloseEvent *e) |
virtual void | hideEvent (QHideEvent *) |
virtual void | keyPressEvent (QKeyEvent *) |
![]() | |
KDialogPrivate *const | d_ptr |
Detailed Description
Spellcheck dialog.
You can change buffer inside a slot connected to done() signal and spellcheck will continue with new data automatically.
Constructor & Destructor Documentation
Sonnet::Dialog::Dialog | ( | BackgroundChecker * | checker, |
QWidget * | parent | ||
) |
Definition at line 97 of file dialog.cpp.
Sonnet::Dialog::~Dialog | ( | ) |
Definition at line 122 of file dialog.cpp.
Member Function Documentation
void Sonnet::Dialog::activeAutoCorrect | ( | bool | _active | ) |
Definition at line 175 of file dialog.cpp.
|
signal |
QString Sonnet::Dialog::buffer | ( | ) | const |
Definition at line 266 of file dialog.cpp.
|
signal |
|
signal |
The dialog won't be closed if you setBuffer() in slot connected to this signal.
Also emitted after stop() signal
|
signal |
Emitted when the user changes the language used for spellchecking, which is shown in a combobox of this dialog.
- Parameters
-
dictionary the new language the user selected
- Since
- 4.1
|
signal |
QString Sonnet::Dialog::originalBuffer | ( | ) | const |
Definition at line 261 of file dialog.cpp.
|
slot |
Definition at line 271 of file dialog.cpp.
void Sonnet::Dialog::setSpellCheckContinuedAfterReplacement | ( | bool | b | ) |
Controls whether the spell checking is continued after the replacement of a misspelled word has been performed.
By default it is continued.
- Since
- 4.4
Definition at line 193 of file dialog.cpp.
void Sonnet::Dialog::show | ( | ) |
Definition at line 307 of file dialog.cpp.
void Sonnet::Dialog::showProgressDialog | ( | int | timeout = 500 | ) |
Controls whether an (indefinite) progress dialog is shown when the spell checking takes longer than the given time to complete.
By default no progress dialog is shown. If the progress dialog is set to be shown, no time consuming operation (for example, showing a notification message) should be performed in a slot connected to the 'done' signal as this might trigger the progress dialog unnecessarily.
- Parameters
-
timeout time after which the progress dialog should appear; a negative value can be used to hide it
- Since
- 4.4
Definition at line 183 of file dialog.cpp.
void Sonnet::Dialog::showSpellCheckCompletionMessage | ( | bool | b = true | ) |
Controls whether a message box indicating the completion of the spell checking is shown or not.
By default it is not shown.
- Since
- 4.4
Definition at line 188 of file dialog.cpp.
|
signal |
Signal sends when spell checking is finished/stopped/completed.
- Since
- 4.1
|
signal |
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:49:18 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.