KFile
#include <kfileplacesmodel.h>
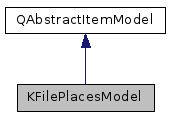
Public Types | |
enum | AdditionalRoles { UrlRole = 0x069CD12B, HiddenRole = 0x0741CAAC, SetupNeededRole = 0x059A935D, FixedDeviceRole = 0x332896C1, CapacityBarRecommendedRole = 0x1548C5C4 } |
Signals | |
void | errorMessage (const QString &message) |
void | setupDone (const QModelIndex &index, bool success) |
Public Member Functions | |
KFilePlacesModel (QObject *parent=0) | |
~KFilePlacesModel () | |
void | addPlace (const QString &text, const KUrl &url, const QString &iconName=QString(), const QString &appName=QString()) |
void | addPlace (const QString &text, const KUrl &url, const QString &iconName, const QString &appName, const QModelIndex &after) |
KBookmark | bookmarkForIndex (const QModelIndex &index) const |
QModelIndex | closestItem (const KUrl &url) const |
int | columnCount (const QModelIndex &parent=QModelIndex()) const |
QVariant | data (const QModelIndex &index, int role) const |
Solid::Device | deviceForIndex (const QModelIndex &index) const |
bool | dropMimeData (const QMimeData *data, Qt::DropAction action, int row, int column, const QModelIndex &parent) |
void | editPlace (const QModelIndex &index, const QString &text, const KUrl &url, const QString &iconName=QString(), const QString &appName=QString()) |
QAction * | ejectActionForIndex (const QModelIndex &index) const |
Qt::ItemFlags | flags (const QModelIndex &index) const |
int | hiddenCount () const |
KIcon | icon (const QModelIndex &index) const |
QModelIndex | index (int row, int column, const QModelIndex &parent=QModelIndex()) const |
bool | isDevice (const QModelIndex &index) const |
bool | isHidden (const QModelIndex &index) const |
QMimeData * | mimeData (const QModelIndexList &indexes) const |
QStringList | mimeTypes () const |
QModelIndex | parent (const QModelIndex &child) const |
void | removePlace (const QModelIndex &index) const |
void | requestEject (const QModelIndex &index) |
void | requestSetup (const QModelIndex &index) |
void | requestTeardown (const QModelIndex &index) |
int | rowCount (const QModelIndex &parent=QModelIndex()) const |
void | setPlaceHidden (const QModelIndex &index, bool hidden) |
bool | setupNeeded (const QModelIndex &index) const |
Qt::DropActions | supportedDropActions () const |
QAction * | teardownActionForIndex (const QModelIndex &index) const |
QString | text (const QModelIndex &index) const |
KUrl | url (const QModelIndex &index) const |
Detailed Description
This class is a list view model.
Each entry represents a "place" where user can access files. Only revelant when used with QListView or QTableView.
Definition at line 40 of file kfileplacesmodel.h.
Member Enumeration Documentation
Enumerator | |
---|---|
UrlRole | |
HiddenRole | |
SetupNeededRole | |
FixedDeviceRole | |
CapacityBarRecommendedRole |
Definition at line 44 of file kfileplacesmodel.h.
Constructor & Destructor Documentation
KFilePlacesModel::KFilePlacesModel | ( | QObject * | parent = 0 | ) |
Definition at line 93 of file kfileplacesmodel.cpp.
KFilePlacesModel::~KFilePlacesModel | ( | ) |
Definition at line 178 of file kfileplacesmodel.cpp.
Member Function Documentation
void KFilePlacesModel::addPlace | ( | const QString & | text, |
const KUrl & | url, | ||
const QString & | iconName = QString() , |
||
const QString & | appName = QString() |
||
) |
Definition at line 640 of file kfileplacesmodel.cpp.
void KFilePlacesModel::addPlace | ( | const QString & | text, |
const KUrl & | url, | ||
const QString & | iconName, | ||
const QString & | appName, | ||
const QModelIndex & | after | ||
) |
Definition at line 646 of file kfileplacesmodel.cpp.
KBookmark KFilePlacesModel::bookmarkForIndex | ( | const QModelIndex & | index | ) | const |
Definition at line 232 of file kfileplacesmodel.cpp.
QModelIndex KFilePlacesModel::closestItem | ( | const KUrl & | url | ) | const |
Returns the closest item for the URL url.
The closest item is defined as item which is equal to the URL or at least is a parent URL. If there are more than one possible parent URL candidates, the item which covers the bigger range of the URL is returned.
Example: the url is '/home/peter/Documents/Music'. Available items are:
- /home/peter
- /home/peter/Documents
The returned item will the one for '/home/peter/Documents'.
Definition at line 287 of file kfileplacesmodel.cpp.
int KFilePlacesModel::columnCount | ( | const QModelIndex & | parent = QModelIndex() | ) | const |
Get the number of columns for a model index.
Definition at line 280 of file kfileplacesmodel.cpp.
QVariant KFilePlacesModel::data | ( | const QModelIndex & | index, |
int | role | ||
) | const |
Get a visible data based on Qt role for the given index.
Return the device information for the give index.
- Parameters
-
index The QModelIndex which contains the row, column to fetch the data. role The Interview data role(ex: Qt::DisplayRole).
- Returns
- the data for the given index and role.
Definition at line 246 of file kfileplacesmodel.cpp.
Solid::Device KFilePlacesModel::deviceForIndex | ( | const QModelIndex & | index | ) | const |
Definition at line 218 of file kfileplacesmodel.cpp.
bool KFilePlacesModel::dropMimeData | ( | const QMimeData * | data, |
Qt::DropAction | action, | ||
int | row, | ||
int | column, | ||
const QModelIndex & | parent | ||
) |
Definition at line 540 of file kfileplacesmodel.cpp.
void KFilePlacesModel::editPlace | ( | const QModelIndex & | index, |
const QString & | text, | ||
const KUrl & | url, | ||
const QString & | iconName = QString() , |
||
const QString & | appName = QString() |
||
) |
Definition at line 665 of file kfileplacesmodel.cpp.
QAction * KFilePlacesModel::ejectActionForIndex | ( | const QModelIndex & | index | ) | const |
Definition at line 777 of file kfileplacesmodel.cpp.
|
signal |
Qt::ItemFlags KFilePlacesModel::flags | ( | const QModelIndex & | index | ) | const |
Definition at line 489 of file kfileplacesmodel.cpp.
int KFilePlacesModel::hiddenCount | ( | ) | const |
Definition at line 719 of file kfileplacesmodel.cpp.
KIcon KFilePlacesModel::icon | ( | const QModelIndex & | index | ) | const |
Definition at line 193 of file kfileplacesmodel.cpp.
QModelIndex KFilePlacesModel::index | ( | int | row, |
int | column, | ||
const QModelIndex & | parent = QModelIndex() |
||
) | const |
Get the children model index for the given row and column.
Definition at line 255 of file kfileplacesmodel.cpp.
bool KFilePlacesModel::isDevice | ( | const QModelIndex & | index | ) | const |
Definition at line 208 of file kfileplacesmodel.cpp.
bool KFilePlacesModel::isHidden | ( | const QModelIndex & | index | ) | const |
Definition at line 203 of file kfileplacesmodel.cpp.
QMimeData * KFilePlacesModel::mimeData | ( | const QModelIndexList & | indexes | ) | const |
Definition at line 516 of file kfileplacesmodel.cpp.
QStringList KFilePlacesModel::mimeTypes | ( | ) | const |
Definition at line 507 of file kfileplacesmodel.cpp.
QModelIndex KFilePlacesModel::parent | ( | const QModelIndex & | child | ) | const |
Get the parent QModelIndex for the given model child.
Definition at line 266 of file kfileplacesmodel.cpp.
void KFilePlacesModel::removePlace | ( | const QModelIndex & | index | ) | const |
Definition at line 687 of file kfileplacesmodel.cpp.
void KFilePlacesModel::requestEject | ( | const QModelIndex & | index | ) |
Definition at line 805 of file kfileplacesmodel.cpp.
void KFilePlacesModel::requestSetup | ( | const QModelIndex & | index | ) |
Definition at line 823 of file kfileplacesmodel.cpp.
void KFilePlacesModel::requestTeardown | ( | const QModelIndex & | index | ) |
Definition at line 792 of file kfileplacesmodel.cpp.
int KFilePlacesModel::rowCount | ( | const QModelIndex & | parent = QModelIndex() | ) | const |
Get the number of rows for a model index.
Definition at line 272 of file kfileplacesmodel.cpp.
void KFilePlacesModel::setPlaceHidden | ( | const QModelIndex & | index, |
bool | hidden | ||
) |
Definition at line 703 of file kfileplacesmodel.cpp.
|
signal |
bool KFilePlacesModel::setupNeeded | ( | const QModelIndex & | index | ) | const |
Definition at line 188 of file kfileplacesmodel.cpp.
Qt::DropActions KFilePlacesModel::supportedDropActions | ( | ) | const |
Definition at line 484 of file kfileplacesmodel.cpp.
QAction * KFilePlacesModel::teardownActionForIndex | ( | const QModelIndex & | index | ) | const |
Definition at line 733 of file kfileplacesmodel.cpp.
QString KFilePlacesModel::text | ( | const QModelIndex & | index | ) | const |
Definition at line 198 of file kfileplacesmodel.cpp.
KUrl KFilePlacesModel::url | ( | const QModelIndex & | index | ) | const |
Definition at line 183 of file kfileplacesmodel.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:52:28 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.