KIO
#include <kbookmark.h>
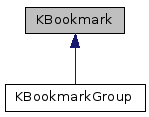
Classes | |
class | List |
Public Types | |
enum | MetaDataOverwriteMode { OverwriteMetaData, DontOverwriteMetaData } |
Static Public Member Functions | |
static QString | commonParent (const QString &A, const QString &B) |
static QString | nextAddress (const QString &address) |
static QString | parentAddress (const QString &address) |
static uint | positionInParent (const QString &address) |
static QString | previousAddress (const QString &address) |
static KBookmark | standaloneBookmark (const QString &text, const KUrl &url, const QString &icon=QString()) |
Protected Attributes | |
QDomElement | element |
Detailed Description
Definition at line 34 of file kbookmark.h.
Member Enumeration Documentation
Enumerator | |
---|---|
OverwriteMetaData | |
DontOverwriteMetaData |
Definition at line 38 of file kbookmark.h.
Constructor & Destructor Documentation
KBookmark::KBookmark | ( | ) |
Constructs a null bookmark, i.e.
a bookmark for which isNull() returns true If you want to create a new bookmark use eitehr KBookmarkGroup.addBookmark or if you want an interactive dialog use KBookmarkDialog.
Definition at line 275 of file kbookmark.cc.
|
explicit |
Creates the KBookmark wrapper for.
- Parameters
-
elem Mostly for internal usage.
Definition at line 279 of file kbookmark.cc.
Member Function Documentation
QString KBookmark::address | ( | ) | const |
Return the "address" of this bookmark in the whole tree.
This is used when telling other processes about a change in a given bookmark. The encoding of the address is "/4/2", for instance, to designate the 2nd child inside the 4th child of the root bookmark.
Definition at line 471 of file kbookmark.cc.
- Returns
- the common parent of both addresses which has the greatest depth
Definition at line 512 of file kbookmark.cc.
QString KBookmark::description | ( | ) | const |
QString KBookmark::fullText | ( | ) | const |
Text shown for the bookmark, not truncated.
You should not use this - this is mainly for keditbookmarks.
Definition at line 311 of file kbookmark.cc.
bool KBookmark::hasParent | ( | ) | const |
- Returns
- true if bookmark is contained by a QDomDocument, if not it is most likely that it has become separated and is thus invalid and/or has been deleted from the bookmarks.
Definition at line 300 of file kbookmark.cc.
QString KBookmark::icon | ( | ) | const |
- Returns
- the pixmap file for this bookmark (i.e. the name of the icon)
Definition at line 348 of file kbookmark.cc.
QDomElement KBookmark::internalElement | ( | ) | const |
for KEditBookmarks
Definition at line 496 of file kbookmark.cc.
bool KBookmark::isGroup | ( | ) | const |
Whether the bookmark is a group or a normal bookmark.
Definition at line 283 of file kbookmark.cc.
bool KBookmark::isNull | ( | ) | const |
- Returns
- true if this is a null bookmark. This will never be the case for a real bookmark (in a menu), but it's used for instance as the end condition for KBookmarkGroup::next()
Definition at line 295 of file kbookmark.cc.
bool KBookmark::isSeparator | ( | ) | const |
Whether the bookmark is a separator.
Definition at line 290 of file kbookmark.cc.
- Returns
- the metadata container node for a certain matadata owner
- Since
- 4.1
Definition at line 578 of file kbookmark.cc.
Get the value of a specific metadata item (owner = "http://www.kde.org").
- Parameters
-
key Name of the metadata item
- Returns
- Value of the metadata item. QString() is returned in case the specified key does not exist.
Definition at line 585 of file kbookmark.cc.
QString KBookmark::mimeType | ( | ) | const |
- Returns
- address of next sibling (e.g. /4/5/2 -> /4/5/3) This doesn't check whether it actually exists
Definition at line 572 of file kbookmark.cc.
Comparison operator.
Definition at line 610 of file kbookmark.cc.
- Returns
- address of parent
Definition at line 554 of file kbookmark.cc.
KBookmarkGroup KBookmark::parentGroup | ( | ) | const |
- Returns
- the group containing this bookmark
Definition at line 460 of file kbookmark.cc.
void KBookmark::populateMimeData | ( | QMimeData * | mimeData | ) | const |
Adds this bookmark into the given QMimeData.
WARNING: do not call this method multiple times, use KBookmark::List::populateMimeData instead.
- Parameters
-
mimeData the QMimeData instance used to drag or copy this bookmark
Definition at line 659 of file kbookmark.cc.
int KBookmark::positionInParent | ( | ) | const |
Return the position in the parent, i.e.
the last number in the address
Definition at line 491 of file kbookmark.cc.
|
static |
- Returns
- position in parent (e.g. /4/5/2 -> 2)
Definition at line 559 of file kbookmark.cc.
- Returns
- address of previous sibling (e.g. /4/5/2 -> /4/5/1) Returns QString() for a first child
Definition at line 564 of file kbookmark.cc.
void KBookmark::setDescription | ( | const QString & | description | ) |
Set the description of the bookmark.
- Parameters
-
description
- Since
- 4.4
Definition at line 412 of file kbookmark.cc.
void KBookmark::setFullText | ( | const QString & | fullText | ) |
Set the text shown for the bookmark.
- Parameters
-
fullText the new bookmark title
Definition at line 321 of file kbookmark.cc.
void KBookmark::setIcon | ( | const QString & | icon | ) |
Set the icon name of the bookmark.
- Parameters
-
icon the new icon name for this bookmark
Definition at line 391 of file kbookmark.cc.
void KBookmark::setMetaDataItem | ( | const QString & | key, |
const QString & | value, | ||
MetaDataOverwriteMode | mode = OverwriteMetaData |
||
) |
Change the value of a specific metadata item, or create the given item if it doesn't exist already (owner = "http://www.kde.org").
- Parameters
-
key Name of the metadata item to change value Value to use for the specified metadata item mode Whether to overwrite the item's value if it exists already or not.
Definition at line 597 of file kbookmark.cc.
void KBookmark::setMimeType | ( | const QString & | mimeType | ) |
Set the Mime-Type of this item.
- Parameters
-
Mime-Type
- Since
- 4.1
Definition at line 436 of file kbookmark.cc.
void KBookmark::setShowInToolbar | ( | bool | show | ) |
Set whether this bookmark is show in a filterd toolbar.
Definition at line 455 of file kbookmark.cc.
void KBookmark::setUrl | ( | const KUrl & | url | ) |
Set the URL of the bookmark.
- Parameters
-
url the new bookmark URL
Definition at line 343 of file kbookmark.cc.
bool KBookmark::showInToolbar | ( | ) | const |
- Returns
- if the bookmark should be shown in the toolbar (used by the filtered toolbar)
Definition at line 443 of file kbookmark.cc.
|
static |
Creates a stand alone bookmark.
This is fairly expensive since a new QDom Tree is build.
Definition at line 501 of file kbookmark.cc.
QString KBookmark::text | ( | ) | const |
Text shown for the bookmark If bigger than 40, the text is shortened by replacing middle characters with "..." (see KStringHandler::csqueeze)
Definition at line 306 of file kbookmark.cc.
KBookmarkGroup KBookmark::toGroup | ( | ) | const |
Convert this to a group - do this only if isGroup() returns true.
Definition at line 465 of file kbookmark.cc.
void KBookmark::updateAccessMetadata | ( | ) |
Updates the bookmarks access metadata Call when a user accesses the bookmark.
Definition at line 535 of file kbookmark.cc.
KUrl KBookmark::url | ( | ) | const |
URL contained by the bookmark.
Definition at line 338 of file kbookmark.cc.
Member Data Documentation
|
protected |
Definition at line 332 of file kbookmark.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:50:03 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.