KIO
#include <kdirlister.h>
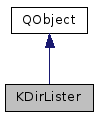
Classes | |
class | Private |
Public Types | |
enum | OpenUrlFlag { NoFlags = 0x0, Keep = 0x1, Reload = 0x2 } |
enum | WhichItems { AllItems = 0, FilteredItems = 1 } |
Signals | |
void | canceled () |
void | canceled (const KUrl &_url) |
void | clear () |
void | clear (const KUrl &_url) |
void | completed () |
void | completed (const KUrl &_url) |
QT_MOC_COMPAT void | deleteItem (const KFileItem &_fileItem) |
void | infoMessage (const QString &msg) |
void | itemsAdded (const KUrl &directoryUrl, const KFileItemList &items) |
void | itemsDeleted (const KFileItemList &items) |
void | itemsFilteredByMime (const KFileItemList &items) |
void | newItems (const KFileItemList &items) |
void | percent (int percent) |
void | processedSize (KIO::filesize_t size) |
void | redirection (const KUrl &_url) |
void | redirection (const KUrl &oldUrl, const KUrl &newUrl) |
void | refreshItems (const QList< QPair< KFileItem, KFileItem > > &items) |
void | speed (int bytes_per_second) |
void | started (const KUrl &_url) |
void | totalSize (KIO::filesize_t size) |
Static Public Member Functions | |
static KFileItem | cachedItemForUrl (const KUrl &url) |
Protected Types | |
enum | Changes { NONE =0, NAME_FILTER =1, MIME_FILTER =2, DOT_FILES =4, DIR_ONLY_MODE =8 } |
Protected Member Functions | |
virtual bool | doMimeFilter (const QString &mime, const QStringList &filters) const |
virtual bool | doNameFilter (const QString &name, const QList< QRegExp > &filters) const |
virtual void | handleError (KIO::Job *) |
virtual bool | matchesFilter (const KFileItem &) const |
virtual bool | matchesMimeFilter (const KFileItem &) const |
Properties | |
bool | autoErrorHandlingEnabled |
bool | autoUpdate |
bool | delayedMimeTypes |
bool | dirOnlyMode |
QStringList | mimeFilter |
QString | nameFilter |
bool | showingDotFiles |
Detailed Description
Helper class for the kiojob used to list and update a directory.
The dir lister deals with the kiojob used to list and update a directory and has signals for the user of this class (e.g. konqueror view or kdesktop) to create/destroy its items when asked.
This class is independent from the graphical representation of the dir (icon container, tree view, ...) and it stores the items (as KFileItems).
Typical usage :
- Create an instance.
- Connect to at least update, clear, itemsAdded, and itemsDeleted.
- Call openUrl - the signals will be called.
- Reuse the instance when opening a new url (openUrl).
- Destroy the instance when not needed anymore (usually destructor).
Advanced usage : call openUrl with OpenUrlFlag::Keep to list directories without forgetting the ones previously read (e.g. for a tree view)
Definition at line 57 of file kdirlister.h.
Member Enumeration Documentation
|
protected |
- Deprecated:
- and unused, ignore this
Enumerator | |
---|---|
NONE | |
NAME_FILTER | |
MIME_FILTER | |
DOT_FILES | |
DIR_ONLY_MODE |
Definition at line 596 of file kdirlister.h.
Enumerator | |
---|---|
NoFlags |
No additional flags specified. |
Keep |
Previous directories aren't forgotten (they are still watched by kdirwatch and their items are kept for this KDirLister). This is useful for e.g. a treeview. |
Reload |
Indicates whether to use the cache or to reread the directory from the disk. Use only when opening a dir not yet listed by this lister without using the cache. Otherwise use updateDirectory. |
Definition at line 72 of file kdirlister.h.
Used by items() and itemsForDir() to specify whether you want all items for a directory or just the filtered ones.
Enumerator | |
---|---|
AllItems | |
FilteredItems |
Definition at line 391 of file kdirlister.h.
Constructor & Destructor Documentation
KDirLister::KDirLister | ( | QObject * | parent = 0 | ) |
Create a directory lister.
Definition at line 2056 of file kdirlister.cpp.
|
virtual |
Destroy the directory lister.
Definition at line 2070 of file kdirlister.cpp.
Member Function Documentation
bool KDirLister::autoErrorHandlingEnabled | ( | ) | const |
Check whether auto error handling is enabled.
If enabled, it will show an error dialog to the user when an error occurs. It is turned on by default.
- Returns
- true if auto error handling is enabled, false otherwise
- See also
- setAutoErrorHandlingEnabled()
bool KDirLister::autoUpdate | ( | ) | const |
Return the KFileItem for the given URL, if we listed it recently and it's still in the cache - which is always the case if a directory view is currently showing this item.
If not, then it might be in the cache, or it might not, in which case you get a null KFileItem. If you really need a KFileItem for this URL in all cases, then use KIO::stat() instead.
- Since
- 4.2
Definition at line 2783 of file kdirlister.cpp.
|
signal |
Tell the view that the user canceled the listing.
No running jobs are left.
|
signal |
Tell the view that the listing of the directory _url
was canceled.
There might be other running jobs left.
- Parameters
-
_url the directory URL
|
signal |
Signal to clear all items.
Make sure to connect to this signal to avoid doubled items.
|
signal |
Signal to empty the directory _url
.
It is only emitted if the lister is holding more than one directory.
- Parameters
-
_url the directory that will be emptied
|
virtual |
Clears the mime based filter.
You need to call emitChanges() afterwards.
- See also
- setMimeFilter
Definition at line 2310 of file kdirlister.cpp.
|
signal |
Tell the view that listing is finished.
There are no jobs running anymore.
|
signal |
Tell the view that the listing of the directory _url
is finished.
There might be other running jobs left.
- Parameters
-
_url the directory URL
bool KDirLister::delayedMimeTypes | ( | ) | const |
- Returns
- true if the "delayed mimetypes" feature was enabled
- See also
- setDelayedMimeTypes
|
signal |
Signals that an item has been deleted.
- Deprecated:
- Don't connect to this signal. Use itemsDeleted instead.
- Parameters
-
_fileItem the fileItem to delete
KUrl::List KDirLister::directories | ( | ) | const |
Returns all URLs that are listed by this KDirLister.
This is only useful if you called openUrl() with OpenUrlFlag::Keep, as it happens in a treeview, for example. (Note that the base url is included in the list as well, of course.)
- Returns
- the list of all listed URLs
Definition at line 2162 of file kdirlister.cpp.
bool KDirLister::dirOnlyMode | ( | ) | const |
Checks whether the KDirLister only lists directories or all files.
By default this option is disabled (all files will be shown).
- Returns
- true if setDirOnlyMode(true) was called
|
protectedvirtual |
Called by the public matchesMimeFilter() to do the actual filtering.
Those methods may be reimplemented to customize filtering.
- Parameters
-
mime the mime type to filter filters the list of mime types to filter
Definition at line 2369 of file kdirlister.cpp.
|
protectedvirtual |
Called by the public matchesFilter() to do the actual filtering.
Those methods may be reimplemented to customize filtering.
- Parameters
-
name the name to filter filters a list of regular expressions for filtering
Definition at line 2360 of file kdirlister.cpp.
|
virtual |
Actually emit the changes made with setShowingDotFiles, setDirOnlyMode, setNameFilter and setMimeFilter.
Definition at line 2167 of file kdirlister.cpp.
Find an item by its name.
- Parameters
-
name the item name
- Returns
- the KFileItem
Definition at line 2260 of file kdirlister.cpp.
Find an item by its URL.
- Parameters
-
_url the item URL
- Returns
- the KFileItem
Definition at line 2250 of file kdirlister.cpp.
|
protectedvirtual |
Reimplement to customize error handling.
Definition at line 2401 of file kdirlister.cpp.
|
signal |
Emitted to display information about running jobs.
Examples of message are "Resolving host", "Connecting to host...", etc.
- Parameters
-
msg the info message
bool KDirLister::isFinished | ( | ) | const |
Returns true if no io operation is currently in progress.
- Returns
- true if finished, false otherwise
Definition at line 2240 of file kdirlister.cpp.
KFileItemList KDirLister::items | ( | WhichItems | which = FilteredItems | ) | const |
Returns the items listed for the current url().
This method will NOT start listing a directory, you should only call this when receiving the finished() signal.
The items in the KFileItemList are copies of the items used by KDirLister.
- Parameters
-
which specifies whether the returned list will contain all entries or only the ones that passed the nameFilter(), mimeFilter(), etc. Note that the latter causes iteration over all the items, filtering them. If this is too slow for you, use the newItems() signal, sending out filtered items in chunks.
- Returns
- the items listed for the current url().
Definition at line 2688 of file kdirlister.cpp.
|
signal |
Signal that new items were found during directory listing.
Alternative signal emitted at the same time as newItems(), but itemsAdded also passes the url of the parent directory.
- Parameters
-
items a list of new items
- Since
- 4.2
|
signal |
Signal that items have been deleted.
- Since
- 4.1.2
- Parameters
-
items the list of deleted items
|
signal |
Send a list of items filtered-out by mime-type.
- Parameters
-
items the list of filtered items
KFileItemList KDirLister::itemsForDir | ( | const KUrl & | dir, |
WhichItems | which = FilteredItems |
||
) | const |
Returns the items listed for the given dir
.
This method will NOT start listing dir
, you should only call this when receiving the finished() signal.
The items in the KFileItemList are copies of the items used by KDirLister.
- Parameters
-
dir specifies the url for which the items should be returned. This is only useful if you use KDirLister with multiple URLs i.e. using bool OpenUrlFlag::Keep in openUrl(). which specifies whether the returned list will contain all entries or only the ones that passed the nameFilter, mimeFilter, etc. Note that the latter causes iteration over all the items, filtering them. If this is too slow for you, use the newItems() signal, sending out filtered items in chunks.
- Returns
- the items listed for
dir
.
Definition at line 2693 of file kdirlister.cpp.
QWidget * KDirLister::mainWindow | ( | ) |
Returns the main window associated with this object.
- Returns
- the associated main window, or 0 if there is none
Definition at line 2683 of file kdirlister.cpp.
Checks whether name
matches a filter in the list of name filters.
- Returns
- true if
name
matches a filter in the list, otherwise false.
- See also
- setNameFilter
Definition at line 2322 of file kdirlister.cpp.
Called for every new item before emitting newItems().
You may reimplement this method in a subclass to implement your own filtering. The default implementation filters out ".." and everything not matching the name filter(s)
- Returns
- true if the item is "ok". false if the item shall not be shown in a view, e.g. files not matching a pattern *.cpp ( KFileItem::isHidden())
- See also
- matchesFilter
- setNameFilter
Definition at line 2335 of file kdirlister.cpp.
Checks whether mime
matches a filter in the list of mime types.
- Parameters
-
mime the mimetype to find in the filter list.
- Returns
- true if
name
matches a filter in the list, otherwise false.
- See also
- setMimeFilter.
Definition at line 2327 of file kdirlister.cpp.
Called for every new item before emitting newItems().
You may reimplement this method in a subclass to implement your own filtering. The default implementation filters out ".." and everything not matching the name filter(s)
- Returns
- true if the item is "ok". false if the item shall not be shown in a view, e.g. files not matching a pattern *.cpp ( KFileItem::isHidden())
- See also
- matchesMimeFilter
- setMimeFilter
Definition at line 2351 of file kdirlister.cpp.
QStringList KDirLister::mimeFilters | ( | ) | const |
Returns the list of mime based filters, as set via setMimeFilter().
- Returns
- the list of mime based filters. Empty, when no mime filter is set.
Definition at line 2317 of file kdirlister.cpp.
QString KDirLister::nameFilter | ( | ) | const |
Returns the current name filter, as set via setNameFilter()
- Returns
- the current name filter, can be QString() if filtering is turned off
|
signal |
Signal new items.
- Parameters
-
items a list of new items
Run the directory lister on the given url.
This method causes KDirLister to emit all the items of _url
, in any case. Depending on _flags, either clear() or clear(const KUrl &) will be emitted first.
The newItems() signal may be emitted more than once to supply you with KFileItems, up until the signal completed() is emitted (and isFinished() returns true).
- Parameters
-
_url the directory URL. _flags whether to keep previous directories, and whether to reload, see OpenUrlFlags
- Returns
- true if successful, false otherwise (e.g. invalid
_url
)
Definition at line 2083 of file kdirlister.cpp.
|
signal |
Progress signal showing the overall progress of the KDirLister.
This allows using a progress bar very easily. (see QProgressBar)
- Parameters
-
percent the progress in percent
|
signal |
Regularly emitted to show the progress of this KDirLister.
- Parameters
-
size the processed size in bytes
|
signal |
Signal a redirection.
Only emitted if there's just one directory to list, i.e. most probably openUrl() has been called without OpenUrlFlag::Keep.
- Parameters
-
_url the new URL
Signal a redirection.
- Parameters
-
oldUrl the original URL newUrl the new URL
Signal an item to refresh (its mimetype/icon/name has changed).
Note: KFileItem::refresh has already been called on those items.
- Parameters
-
items the items to refresh. This is a list of pairs, where the first item in the pair is the OLD item, and the second item is the NEW item. This allows to track which item has changed, especially after a renaming.
KFileItem KDirLister::rootItem | ( | ) | const |
Returns the file item of the URL.
Can return an empty KFileItem.
- Returns
- the file item for url() itself (".")
Definition at line 2245 of file kdirlister.cpp.
Enable or disable auto error handling is enabled.
If enabled, it will show an error dialog to the user when an error occurs. It is turned on by default.
- Parameters
-
enable true to enable auto error handling, false to disable parent the parent widget for the error dialogs, can be 0 for top-level
- See also
- autoErrorHandlingEnabled()
Definition at line 2151 of file kdirlister.cpp.
|
virtual |
Enable/disable automatic directory updating, when a directory changes (using KDirWatch).
- Parameters
-
enable true to enable, false to disable
Definition at line 2109 of file kdirlister.cpp.
void KDirLister::setDelayedMimeTypes | ( | bool | delayedMimeTypes | ) |
Delayed mimetypes feature: If enabled, mime types will be fetched on demand, which leads to a faster initial directory listing, where icons get progressively replaced with the correct one while KMimeTypeResolver is going through the items with unknown or imprecise mimetype (e.g.
files with no extension or an unknown extension).
Definition at line 2722 of file kdirlister.cpp.
|
virtual |
Call this to list only directories.
You need to call emitChanges() afterwards. By default this option is disabled (all files will be shown).
- Parameters
-
dirsOnly true to list only directories
Definition at line 2137 of file kdirlister.cpp.
void KDirLister::setMainWindow | ( | QWidget * | window | ) |
Pass the main window this object is associated with this is used for caching authentication data.
- Parameters
-
window the window to associate with, 0 to disassociate
Definition at line 2678 of file kdirlister.cpp.
void KDirLister::setMimeExcludeFilter | ( | const QStringList & | mimeList | ) |
Filtering should be done with KFileFilter.
This will be implemented in a later revision of KDirLister. This method may be removed then.
Set mime-based exclude filter to only list items not matching the given mimetypes
NOTE: setting the filter does not automatically reload directory. Also calling this function will not affect any named filter already set.
- Parameters
-
mimeList a list of mime-types.
- See also
- clearMimeFilter
- matchesMimeFilter
Definition at line 2300 of file kdirlister.cpp.
|
virtual |
Set mime-based filter to only list items matching the given mimetypes.
NOTE: setting the filter does not automatically reload directory. Also calling this function will not affect any named filter already set.
You need to call emitChanges() afterwards.
- Parameters
-
mimeList a list of mime-types.
- See also
- clearMimeFilter
- matchesMimeFilter
Definition at line 2288 of file kdirlister.cpp.
|
virtual |
Set a name filter to only list items matching this name, e.g.
"*.cpp".
You can set more than one filter by separating them with whitespace, e.g "*.cpp *.h". Note: the directory is not automatically reloaded. You need to call emitChanges() afterwards.
- Parameters
-
filter the new filter, QString() to disable filtering
- See also
- matchesFilter
Definition at line 2268 of file kdirlister.cpp.
|
virtual |
Changes the "is viewing dot files" setting.
You need to call emitChanges() afterwards. By default this option is disabled (hidden files will not be shown).
- Parameters
-
_showDotFiles true to enable showing hidden files, false to disable
- See also
- showingDotFiles()
Definition at line 2123 of file kdirlister.cpp.
bool KDirLister::showingDotFiles | ( | ) | const |
Checks whether hidden files (files beginning with a dot) will be shown.
By default this option is disabled (hidden files will be not shown).
- Returns
- true if dot files are shown, false otherwise
- See also
- setShowingDotFiles()
|
signal |
Emitted to display information about the speed of the jobs.
- Parameters
-
bytes_per_second the speed in bytes/s
|
signal |
Tell the view that we started to list _url
.
NOTE: this does not imply that there is really a job running! I.e. KDirLister::jobs() may return an empty list. In this case the items are taken from the cache.
The view knows that openUrl should start it, so it might seem useless, but the view also needs to know when an automatic update happens.
- Parameters
-
_url the URL to list
|
virtual |
Stop listing all directories currently being listed.
Emits canceled() if there was at least one job running. Emits canceled( const KUrl& ) for each stopped job if there are at least two directories being watched by KDirLister.
Definition at line 2094 of file kdirlister.cpp.
|
virtual |
Stop listing the given directory.
Emits canceled() if the killed job was the last running one. Emits canceled( const KUrl& ) for the killed job if there are at least two directories being watched by KDirLister. No signal is emitted if there was no job running for _url
.
- Parameters
-
_url the directory URL
Definition at line 2099 of file kdirlister.cpp.
|
signal |
Emitted when we know the size of the jobs.
- Parameters
-
size the total size in bytes
|
virtual |
Update the directory _dir
.
This method causes KDirLister to only emit the items of _dir
that actually changed compared to the current state in the cache and updates the cache.
The current implementation calls updateDirectory automatically for local files, using KDirWatch (if autoUpdate() is true), but it might be useful to force an update manually.
- Parameters
-
_dir the directory URL
Definition at line 2235 of file kdirlister.cpp.
KUrl KDirLister::url | ( | ) | const |
Returns the top level URL that is listed by this KDirLister.
It might be different from the one given with openUrl() if there was a redirection. If you called openUrl() with OpenUrlFlag::Keep this is the first url opened (e.g. in a treeview this is the root).
- Returns
- the url used by this instance to list the files.
Definition at line 2157 of file kdirlister.cpp.
Property Documentation
|
read |
Definition at line 66 of file kdirlister.h.
|
readwrite |
Definition at line 63 of file kdirlister.h.
|
readwrite |
Definition at line 67 of file kdirlister.h.
|
readwrite |
Definition at line 65 of file kdirlister.h.
|
readwrite |
Definition at line 69 of file kdirlister.h.
|
readwrite |
Definition at line 68 of file kdirlister.h.
|
readwrite |
Definition at line 64 of file kdirlister.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:50:04 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.