KIO
#include <accessmanager.h>
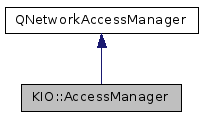
Public Types | |
enum | Attribute { MetaData = QNetworkRequest::User, KioError } |
Public Member Functions | |
AccessManager (QObject *parent) | |
virtual | ~AccessManager () |
WId | cookieJarWindowid () const |
bool | isExternalContentAllowed () const |
KIO::MetaData & | requestMetaData () |
KIO::MetaData & | sessionMetaData () |
void | setCookieJarWindowId (WId id) |
void | setEmitReadyReadOnMetaDataChange (bool) |
void | setExternalContentAllowed (bool allowed) |
void | setWindow (QWidget *widget) |
QWidget * | window () const |
Static Public Member Functions | |
static void | putReplyOnHold (QNetworkReply *reply) |
Protected Member Functions | |
virtual QNetworkReply * | createRequest (Operation op, const QNetworkRequest &req, QIODevice *outgoingData=0) |
Detailed Description
A KDE implementation of QNetworkAccessManager.
Use this class instead of QNetworkAccessManager if you want to integrate with KDE's KIO and KCookieJar modules for network operations and cookie handling respectively.
Here is a simple example that shows how to set the QtWebKit module to use KDE's KIO for its network operations:
To access member functions in the cookiejar class at a later point in your code simply downcast the pointer returned by QWebPage::networkAccessManager as follows:
Please note that this class is in the KIO namespace for backward compatablity. You should use KIO::Integration::AccessManager to access this class in your code.
IMPORTANTThis class is not a replacement for the standard KDE API. It should ONLY be used to provide KDE integration in applications that cannot use the standard KDE API directly.
- Deprecated:
- Use the KIO::Integration::AccessManager typedef to access this class instead.
- Since
- 4.3
Definition at line 73 of file accessmanager.h.
Member Enumeration Documentation
Extensions to QNetworkRequest::Attribute enums.
- Since
- 4.3.2
Enumerator | |
---|---|
MetaData | |
KioError |
< Used to send KIO MetaData back and forth. type: QVariant::Map. Used to send KIO error codes that cannot be mapped into QNetworkReply::NetworkError. type: QVariant::Int |
Definition at line 81 of file accessmanager.h.
Constructor & Destructor Documentation
AccessManager::AccessManager | ( | QObject * | parent | ) |
Constructor.
Definition at line 115 of file accessmanager.cpp.
|
virtual |
Destructor.
Definition at line 122 of file accessmanager.cpp.
Member Function Documentation
WId AccessManager::cookieJarWindowid | ( | ) | const |
Returns the cookiejar's window id.
This is a convenience function that returns the window id associated with the cookiejar. Note that this function will return a 0 if the cookiejar is not of type KIO::Integration::CookieJar or a window id has not yet been set.
- Since
- 4.4
Definition at line 173 of file accessmanager.cpp.
|
protectedvirtual |
Reimplemented for internal reasons, the API is not affected.
- See also
- QNetworkAccessManager::createRequest
Definition at line 213 of file accessmanager.cpp.
bool AccessManager::isExternalContentAllowed | ( | ) | const |
Returns true if external content is going to be fetched.
- See also
- setExternalContentAllowed
Definition at line 132 of file accessmanager.cpp.
|
static |
Puts the ioslave associated with the given reply
on hold.
This function is intended to make possible the implementation of the special case mentioned in KIO::get's documentation within the KIO-QNAM integration.
- See also
- KIO::get.
- Since
- 4.6
Definition at line 198 of file accessmanager.cpp.
KIO::MetaData & AccessManager::requestMetaData | ( | ) |
Returns a reference to the temporary meta data container.
See kdelibs/kio/DESIGN.metadata for list of supported KIO meta data.
Use this function when you want to set per request KIO meta data that will be removed after it has been sent once.
- Since
- 4.4
Definition at line 188 of file accessmanager.cpp.
KIO::MetaData & AccessManager::sessionMetaData | ( | ) |
Returns a reference to the persistent meta data container.
See kdelibs/kio/DESIGN.metadata for list of supported KIO meta data.
Use this function when you want to set per session KIO meta data that will be sent with every request.
Unlike requestMetaData
, the meta data values set using the reference returned by this function will not be deleted and will be sent with every request.
- Since
- 4.4
Definition at line 193 of file accessmanager.cpp.
void AccessManager::setCookieJarWindowId | ( | WId | id | ) |
Sets the cookiejar's window id to id
.
This is a convenience function that allows you to set the cookiejar's window id. Note that this function does nothing unless the cookiejar in use is of type KIO::Integration::CookieJar.
By default the cookiejar's window id is set to false. Make sure you call this function and set the window id to its proper value when create an instance of this object. Otherwise, the KDE cookiejar will not be able to properly manage session based cookies.
- Since
- 4.4
- Deprecated:
- Use setWindow
Definition at line 138 of file accessmanager.cpp.
void AccessManager::setEmitReadyReadOnMetaDataChange | ( | bool | enable | ) |
Sets the network reply object to emit readyRead when it receives meta data.
Meta data is any information that is not the actual content itself, e.g. HTTP response headers of the HTTP protocol.
Calling this function will force the code connecting to QNetworkReply's readyRead signal to prematurely start dealing with the content that might not yet have arrived. However, it is essential to make the put ioslave on hold functionality of KIO work in libraries like QtWebKit.
- See also
- QNetworkReply::metaDataChanged
- Since
- 4.7
Definition at line 208 of file accessmanager.cpp.
void AccessManager::setExternalContentAllowed | ( | bool | allowed | ) |
Set allowed
to false if you don't want any external content to be fetched.
By default external content is fetched.
Definition at line 127 of file accessmanager.cpp.
void AccessManager::setWindow | ( | QWidget * | widget | ) |
Sets the window associated with this network access manager.
Note that widget
will be used as a parent for dialogs in KIO as well as the cookie jar. If widget
is not a window, this function will invoke QWidget::window() to obtain the window for the given widget.
- See also
- KIO::Integration::CookieJar::setWindow.
- Since
- 4.7
Definition at line 154 of file accessmanager.cpp.
QWidget * AccessManager::window | ( | ) | const |
Returns the window associated with this network access manager.
- See also
- setWindow
- Since
- 4.7
Definition at line 183 of file accessmanager.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:50:04 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.