KIO
#include <copyjob.h>
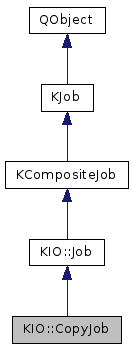
Public Types | |
enum | CopyMode { Copy, Move, Link } |
![]() | |
enum | Capability |
enum | KillVerbosity |
enum | Unit |
Signals | |
void | aboutToCreate (KIO::Job *job, const QList< KIO::CopyInfo > &files) |
void | copying (KIO::Job *job, const KUrl &src, const KUrl &dest) |
void | copyingDone (KIO::Job *job, const KUrl &from, const KUrl &to, time_t mtime, bool directory, bool renamed) |
void | copyingLinkDone (KIO::Job *job, const KUrl &from, const QString &target, const KUrl &to) |
void | creatingDir (KIO::Job *job, const KUrl &dir) |
void | linking (KIO::Job *job, const QString &target, const KUrl &to) |
void | moving (KIO::Job *job, const KUrl &from, const KUrl &to) |
void | processedDirs (KIO::Job *job, unsigned long dirs) |
void | processedFiles (KIO::Job *job, unsigned long files) |
void | renamed (KIO::Job *job, const KUrl &from, const KUrl &to) |
void | totalDirs (KJob *job, unsigned long dirs) |
void | totalFiles (KJob *job, unsigned long files) |
![]() | |
void | canceled (KJob *job) |
void | connected (KIO::Job *job) |
![]() | |
void | description (KJob *job, const QString &title, const QPair< QString, QString > &field1=qMakePair(QString(), QString()), const QPair< QString, QString > &field2=qMakePair(QString(), QString())) |
void | finished (KJob *job) |
void | infoMessage (KJob *job, const QString &plain, const QString &rich=QString()) |
void | percent (KJob *job, unsigned long percent) |
void | processedAmount (KJob *job, KJob::Unit unit, qulonglong amount) |
void | processedSize (KJob *job, qulonglong size) |
void | result (KJob *job) |
void | resumed (KJob *job) |
void | speed (KJob *job, unsigned long speed) |
void | suspended (KJob *job) |
void | totalAmount (KJob *job, KJob::Unit unit, qulonglong amount) |
void | totalSize (KJob *job, qulonglong size) |
void | warning (KJob *job, const QString &plain, const QString &rich=QString()) |
Public Member Functions | |
virtual | ~CopyJob () |
KUrl | destUrl () const |
virtual bool | doSuspend () |
CopyMode | operationMode () const |
void | setAutoRename (bool autoRename) |
void | setAutoSkip (bool autoSkip) |
void | setDefaultPermissions (bool b) |
void | setWriteIntoExistingDirectories (bool overwriteAllDirs) |
KUrl::List | srcUrls () const |
![]() | |
virtual | ~Job () |
void | addMetaData (const QString &key, const QString &value) |
void | addMetaData (const QMap< QString, QString > &values) |
QStringList | detailedErrorStrings (const KUrl *reqUrl=0L, int method=-1) const |
QString | errorString () const |
bool | isInteractive () const |
void | mergeMetaData (const QMap< QString, QString > &values) |
MetaData | metaData () const |
MetaData | outgoingMetaData () const |
Job * | parentJob () const |
QString | queryMetaData (const QString &key) |
void | setMetaData (const KIO::MetaData &metaData) |
void | setParentJob (Job *parentJob) |
void | showErrorDialog (QWidget *parent=0) |
void | start () |
JobUiDelegate * | ui () const |
![]() | |
KCompositeJob (QObject *parent=0) | |
virtual | ~KCompositeJob () |
virtual | ~KJob () |
Capabilities | capabilities () const |
int | error () const |
QString | errorText () const |
bool | exec () |
bool | isAutoDelete () const |
bool | isSuspended () const |
KJob (QObject *parent=0) | |
unsigned long | percent () const |
qulonglong | processedAmount (Unit unit) const |
void | setAutoDelete (bool autodelete) |
void | setUiDelegate (KJobUiDelegate *delegate) |
qulonglong | totalAmount (Unit unit) const |
KJobUiDelegate * | uiDelegate () const |
Protected Slots | |
virtual void | slotResult (KJob *job) |
![]() | |
virtual void | slotInfoMessage (KJob *job, const QString &plain, const QString &rich) |
virtual void | slotResult (KJob *job) |
Protected Member Functions | |
CopyJob (CopyJobPrivate &dd) | |
void | emitResult () |
![]() | |
Job () | |
Job (JobPrivate &dd) | |
virtual bool | addSubjob (KJob *job) |
virtual bool | doKill () |
virtual bool | doResume () |
virtual bool | removeSubjob (KJob *job) |
![]() | |
KCompositeJob (KCompositeJobPrivate &dd, QObject *parent) | |
void | clearSubjobs () |
void | emitPercent (qulonglong processedAmount, qulonglong totalAmount) |
void | emitResult () |
void | emitSpeed (unsigned long speed) |
bool | hasSubjobs () |
KJob (KJobPrivate &dd, QObject *parent) | |
void | setCapabilities (Capabilities capabilities) |
void | setError (int errorCode) |
void | setErrorText (const QString &errorText) |
void | setPercent (unsigned long percentage) |
void | setProcessedAmount (Unit unit, qulonglong amount) |
void | setTotalAmount (Unit unit, qulonglong amount) |
const QList< KJob * > & | subjobs () const |
Additional Inherited Members | |
![]() | |
bool | kill (KillVerbosity verbosity=Quietly) |
bool | resume () |
bool | suspend () |
![]() | |
KJobPrivate *const | d_ptr |
Detailed Description
CopyJob is used to move, copy or symlink files and directories.
Don't create the job directly, but use KIO::copy(), KIO::move(), KIO::link() and friends.
Member Enumeration Documentation
Constructor & Destructor Documentation
|
virtual |
Definition at line 264 of file copyjob.cpp.
|
protected |
Definition at line 256 of file copyjob.cpp.
Member Function Documentation
|
signal |
Emitted when it is known which files / directories are going to be created.
Note that this may still change e.g. when existing files with the same name are discovered.
- Parameters
-
job the job that emitted this signal files a list of items that are about to be created.
The job is copying a file or directory.
Note: This signal is used for progress dialogs, it's not emitted for every file or directory (this would be too slow), but every 200ms.
- Parameters
-
job the job that emitted this signal src the URL of the file or directory that is currently being copied dest the destination of the current operation
|
signal |
The job emits this signal when copying or moving a file or directory successfully finished.
This signal is mainly for the Undo feature. If you simply want to know when a copy job is done, use result().
- Parameters
-
job the job that emitted this signal from the source URL to the destination URL mtime the modification time of the source file, hopefully set on the destination file too (when the kioslave supports it). directory indicates whether a file or directory was successfully copied/moved. true for a directory, false for file renamed indicates that the destination URL was created using a rename operation (i.e. fast directory moving). true if is has been renamed
|
signal |
The job is copying or moving a symbolic link, that points to target.
The new link is created in to
. The existing one is/was in from
. This signal is mainly for the Undo feature.
- Parameters
-
job the job that emitted this signal from the source URL target the target to the destination URL
The job is creating the directory dir
.
This signal is emitted for every directory being created.
- Parameters
-
job the job that emitted this signal dir the directory that is currently being created
KUrl CopyJob::destUrl | ( | ) | const |
|
virtual |
Reimplemented for internal reasons.
Reimplemented from KIO::Job.
Definition at line 484 of file copyjob.cpp.
|
protected |
Definition at line 1745 of file copyjob.cpp.
The job is creating a symbolic link.
Note: This signal is used for progress dialogs, it's not emitted for every file or directory (this would be too slow), but every 200ms.
- Parameters
-
job the job that emitted this signal target the URL of the file or directory that is currently being linked to the destination of the current operation
The job is moving a file or directory.
Note: This signal is used for progress dialogs, it's not emitted for every file or directory (this would be too slow), but every 200ms.
- Parameters
-
job the job that emitted this signal from the URL of the file or directory that is currently being moved to the destination of the current operation
KIO::CopyJob::CopyMode KIO::CopyJob::operationMode | ( | ) | const |
Returns the mode of the operation (copy, move, or link), depending on whether KIO::copy(), KIO::move() or KIO::link() was called.
Definition at line 2140 of file copyjob.cpp.
|
signal |
Sends the number of processed directories.
- Parameters
-
job the job that emitted this signal dirs the number of processed dirs
|
signal |
Sends the number of processed files.
- Parameters
-
job the job that emitted this signal files the number of processed files
The user chose to rename from
to to
.
- Parameters
-
job the job that emitted this signal from the original name to the new name
void KIO::CopyJob::setAutoRename | ( | bool | autoRename | ) |
Rename files automatically when the destination already exists, instead of the default behavior (interactive mode: showing a dialog to the user, non-interactive mode: aborting with an error).
Initially added for a unit test.
- Since
- 4.7
Definition at line 2151 of file copyjob.cpp.
void KIO::CopyJob::setAutoSkip | ( | bool | autoSkip | ) |
Skip copying or moving any file when the destination already exists, instead of the default behavior (interactive mode: showing a dialog to the user, non-interactive mode: aborting with an error).
Initially added for a unit test.
- Since
- 4.2
Definition at line 2145 of file copyjob.cpp.
void KIO::CopyJob::setDefaultPermissions | ( | bool | b | ) |
By default the permissions of the copied files will be those of the source files.
But when copying "template" files to "new" files, people prefer the umask to apply, rather than the template's permissions. For that case, call setDefaultPermissions(true)
Definition at line 2135 of file copyjob.cpp.
void KIO::CopyJob::setWriteIntoExistingDirectories | ( | bool | overwriteAllDirs | ) |
Reuse any directory that already exists, instead of the default behavior (interactive mode: showing a dialog to the user, non-interactive mode: aborting with an error).
- Since
- 4.2
Definition at line 2157 of file copyjob.cpp.
|
protectedvirtualslot |
Definition at line 2080 of file copyjob.cpp.
KUrl::List CopyJob::srcUrls | ( | ) | const |
Returns the list of source URLs.
- Returns
- the list of source URLs.
Definition at line 268 of file copyjob.cpp.
|
signal |
Emitted when the toal number of direcotries is known.
- Parameters
-
job the job that emitted this signal dirs the total number of directories
|
signal |
Emitted when the total number of files is known.
- Parameters
-
job the job that emitted this signal files the total number of files
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:50:04 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.