KIO
#include <metainfojob.h>
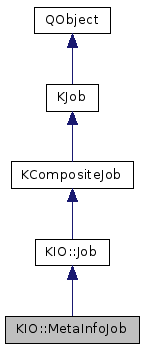
Signals | |
void | failed (const KFileItem &item) |
void | gotMetaInfo (const KFileItem &item) |
![]() | |
void | canceled (KJob *job) |
void | connected (KIO::Job *job) |
![]() | |
void | description (KJob *job, const QString &title, const QPair< QString, QString > &field1=qMakePair(QString(), QString()), const QPair< QString, QString > &field2=qMakePair(QString(), QString())) |
void | finished (KJob *job) |
void | infoMessage (KJob *job, const QString &plain, const QString &rich=QString()) |
void | percent (KJob *job, unsigned long percent) |
void | processedAmount (KJob *job, KJob::Unit unit, qulonglong amount) |
void | processedSize (KJob *job, qulonglong size) |
void | result (KJob *job) |
void | resumed (KJob *job) |
void | speed (KJob *job, unsigned long speed) |
void | suspended (KJob *job) |
void | totalAmount (KJob *job, KJob::Unit unit, qulonglong amount) |
void | totalSize (KJob *job, qulonglong size) |
void | warning (KJob *job, const QString &plain, const QString &rich=QString()) |
Public Member Functions | |
MetaInfoJob (const KFileItemList &items, KFileMetaInfo::WhatFlags w=KFileMetaInfo::Everything, int iocost=3, int cpucost=6, const QStringList &requiredfields=QStringList(), const QStringList &requestedfields=QStringList()) | |
virtual | ~MetaInfoJob () |
void | removeItem (const KFileItem &item) |
![]() | |
virtual | ~Job () |
void | addMetaData (const QString &key, const QString &value) |
void | addMetaData (const QMap< QString, QString > &values) |
QStringList | detailedErrorStrings (const KUrl *reqUrl=0L, int method=-1) const |
QString | errorString () const |
bool | isInteractive () const |
void | mergeMetaData (const QMap< QString, QString > &values) |
MetaData | metaData () const |
MetaData | outgoingMetaData () const |
Job * | parentJob () const |
QString | queryMetaData (const QString &key) |
void | setMetaData (const KIO::MetaData &metaData) |
void | setParentJob (Job *parentJob) |
void | showErrorDialog (QWidget *parent=0) |
void | start () |
JobUiDelegate * | ui () const |
![]() | |
KCompositeJob (QObject *parent=0) | |
virtual | ~KCompositeJob () |
virtual | ~KJob () |
Capabilities | capabilities () const |
int | error () const |
QString | errorText () const |
bool | exec () |
bool | isAutoDelete () const |
bool | isSuspended () const |
KJob (QObject *parent=0) | |
unsigned long | percent () const |
qulonglong | processedAmount (Unit unit) const |
void | setAutoDelete (bool autodelete) |
void | setUiDelegate (KJobUiDelegate *delegate) |
qulonglong | totalAmount (Unit unit) const |
KJobUiDelegate * | uiDelegate () const |
Protected Slots | |
virtual void | slotResult (KJob *job) |
![]() | |
virtual void | slotInfoMessage (KJob *job, const QString &plain, const QString &rich) |
virtual void | slotResult (KJob *job) |
Protected Member Functions | |
void | getMetaInfo () |
![]() | |
Job () | |
Job (JobPrivate &dd) | |
virtual bool | addSubjob (KJob *job) |
virtual bool | doKill () |
virtual bool | doResume () |
virtual bool | doSuspend () |
virtual bool | removeSubjob (KJob *job) |
![]() | |
KCompositeJob (KCompositeJobPrivate &dd, QObject *parent) | |
void | clearSubjobs () |
void | emitPercent (qulonglong processedAmount, qulonglong totalAmount) |
void | emitResult () |
void | emitSpeed (unsigned long speed) |
bool | hasSubjobs () |
KJob (KJobPrivate &dd, QObject *parent) | |
void | setCapabilities (Capabilities capabilities) |
void | setError (int errorCode) |
void | setErrorText (const QString &errorText) |
void | setPercent (unsigned long percentage) |
void | setProcessedAmount (Unit unit, qulonglong amount) |
void | setTotalAmount (Unit unit, qulonglong amount) |
const QList< KJob * > & | subjobs () const |
Additional Inherited Members | |
![]() | |
enum | Capability |
enum | KillVerbosity |
enum | Unit |
![]() | |
bool | kill (KillVerbosity verbosity=Quietly) |
bool | resume () |
bool | suspend () |
![]() | |
KJobPrivate *const | d_ptr |
Detailed Description
MetaInfoJob is a KIO Job to retrieve meta information from files.
KIO Job to retrieve meta information from files.
Definition at line 35 of file metainfojob.h.
Constructor & Destructor Documentation
|
explicit |
Creates a new MetaInfoJob.
- Parameters
-
items A list of KFileItems to get the metainfo for w Flags which serve as a preset which can be customized with other parameters. iocost The allowed cost in terms of io to retrieve the metainfo. The approximate maximum number of bytes to be read is 10^iocost. Negative values mean that there is no limit on the cost. 0 means that no fields other than the required fields will be retrieved. The default value of 3 means about 1024 bytes per file may be read. This is merely a suggestion and not a hard limit. cpucost The allowed cost in terms of cpu to determine the information in the fields. The number mean the amount of instructions allowed is 10^cpucost and is a suggestion only. The default value of 6 means that about a million instructions (10^6) are allowed. This is useful for expensive fields like md5 or thumbnails. requiredfields The names of fields or groups of fields that should be retrieved regardless of cost. requestedfields The names of fields or groups of fields that should be retrieved first.
Definition at line 45 of file metainfojob.cpp.
|
virtual |
Definition at line 68 of file metainfojob.cpp.
Member Function Documentation
|
signal |
Emitted when metainfo for item
could not be extracted, either because a plugin for its MIME type does not exist, or because something went wrong.
- Parameters
-
item the KFileItem of the file that failed
|
protected |
Definition at line 125 of file metainfojob.cpp.
|
signal |
Emitted when the meta info for item
has been successfully retrieved.
- Parameters
-
item the KFileItem describing the fetched item
void MetaInfoJob::removeItem | ( | const KFileItem & | item | ) |
Removes an item from metainfo extraction.
- Parameters
-
item the item that should be removed from the queue
Definition at line 77 of file metainfojob.cpp.
|
protectedvirtualslot |
Definition at line 117 of file metainfojob.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:50:04 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.