KIO
#include <tcpslavebase.h>
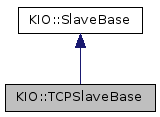
Public Member Functions | |
TCPSlaveBase (const QByteArray &protocol, const QByteArray &poolSocket, const QByteArray &appSocket, bool autoSsl=false) | |
virtual | ~TCPSlaveBase () |
![]() | |
SlaveBase (const QByteArray &protocol, const QByteArray &pool_socket, const QByteArray &app_socket) | |
virtual | ~SlaveBase () |
MetaData | allMetaData () const |
bool | cacheAuthentication (const AuthInfo &info) |
bool | canResume (KIO::filesize_t offset) |
void | canResume () |
bool | checkCachedAuthentication (AuthInfo &info) |
virtual void | chmod (const KUrl &url, int permissions) |
virtual void | chown (const KUrl &url, const QString &owner, const QString &group) |
virtual void | close () |
virtual void | closeConnection () |
KConfigGroup * | config () |
void | connected () |
void | connectSlave (const QString &path) |
int | connectTimeout () |
virtual void | copy (const KUrl &src, const KUrl &dest, int permissions, JobFlags flags) |
void | data (const QByteArray &data) |
void | dataReq () |
virtual void | del (const KUrl &url, bool isfile) |
void | disconnectSlave () |
virtual void | dispatch (int command, const QByteArray &data) |
void | dispatchLoop () |
virtual void | dispatchOpenCommand (int command, const QByteArray &data) |
void | dropNetwork (const QString &host=QString()) |
void | error (int _errid, const QString &_text) |
void | errorPage () |
void | exit () |
void | finished () |
virtual void | get (const KUrl &url) |
bool | hasMetaData (const QString &key) const |
void | infoMessage (const QString &msg) |
virtual void | listDir (const KUrl &url) |
void | listEntries (const UDSEntryList &_entry) |
void | listEntry (const UDSEntry &_entry, bool ready) |
void | lookupHost (const QString &host) |
int | messageBox (MessageBoxType type, const QString &text, const QString &caption=QString(), const QString &buttonYes=i18n("&Yes"), const QString &buttonNo=i18n("&No")) |
int | messageBox (const QString &text, MessageBoxType type, const QString &caption=QString(), const QString &buttonYes=i18n("&Yes"), const QString &buttonNo=i18n("&No"), const QString &dontAskAgainName=QString()) |
QString | metaData (const QString &key) const |
void | mimeType (const QString &_type) |
virtual void | mimetype (const KUrl &url) |
virtual void | mkdir (const KUrl &url, int permissions) |
virtual void | multiGet (const QByteArray &data) |
void | needSubUrlData () |
virtual void | open (const KUrl &url, QIODevice::OpenMode mode) |
virtual void | openConnection () |
void | opened () |
bool | openPasswordDialog (KIO::AuthInfo &info, const QString &errorMsg=QString()) |
void | position (KIO::filesize_t _pos) |
void | processedPercent (float percent) |
void | processedSize (KIO::filesize_t _bytes) |
int | proxyConnectTimeout () |
virtual void | put (const KUrl &url, int permissions, JobFlags flags) |
virtual void | read (KIO::filesize_t size) |
int | readData (QByteArray &buffer) |
int | readTimeout () |
void | redirection (const KUrl &_url) |
KRemoteEncoding * | remoteEncoding () |
virtual void | rename (const KUrl &src, const KUrl &dest, JobFlags flags) |
virtual void | reparseConfiguration () |
bool | requestNetwork (const QString &host=QString()) |
int | responseTimeout () |
virtual void | seek (KIO::filesize_t offset) |
void | sendAndKeepMetaData () |
void | sendMetaData () |
virtual void | setHost (const QString &host, quint16 port, const QString &user, const QString &pass) |
void | setKillFlag () |
virtual void | setLinkDest (const KUrl &url, const QString &target) |
void | setMetaData (const QString &key, const QString &value) |
virtual void | setModificationTime (const KUrl &url, const QDateTime &mtime) |
virtual void | setSubUrl (const KUrl &url) |
void | setTimeoutSpecialCommand (int timeout, const QByteArray &data=QByteArray()) |
virtual void | slave_status () |
void | slaveStatus (const QString &host, bool connected) |
virtual void | special (const QByteArray &data) |
void | speed (unsigned long _bytes_per_second) |
virtual void | stat (const KUrl &url) |
void | statEntry (const UDSEntry &_entry) |
virtual void | symlink (const QString &target, const KUrl &dest, JobFlags flags) |
void | totalSize (KIO::filesize_t _bytes) |
int | waitForAnswer (int expected1, int expected2, QByteArray &data, int *pCmd=0) |
int | waitForHostInfo (QHostInfo &info) |
void | warning (const QString &msg) |
bool | wasKilled () const |
virtual void | write (const QByteArray &data) |
void | written (KIO::filesize_t _bytes) |
Protected Types | |
enum | SslResultDetail { ResultOk = 1, ResultOverridden = 2, ResultFailed = 4, ResultFailedEarly = 8 } |
![]() | |
enum | VirtualFunctionId { AppConnectionMade = 0 } |
Protected Member Functions | |
bool | atEnd () const |
bool | connectToHost (const QString &protocol, const QString &host, quint16 port) |
int | connectToHost (const QString &host, quint16 port, QString *errorString=0) |
void | disconnectFromHost () |
bool | isAutoSsl () const |
bool | isConnected () const |
bool | isUsingSsl () const |
quint16 | port () const |
ssize_t | read (char *data, ssize_t len) |
ssize_t | readLine (char *data, ssize_t len) |
void | setBlocking (bool b) |
QIODevice * | socket () const |
bool | startSsl () |
virtual void | virtual_hook (int id, void *data) |
bool | waitForResponse (int t) |
ssize_t | write (const char *data, ssize_t len) |
Additional Inherited Members | |
![]() | |
enum | MessageBoxType { QuestionYesNo = 1, WarningYesNo = 2, WarningContinueCancel = 3, WarningYesNoCancel = 4, Information = 5, SSLMessageBox = 6 } |
![]() | |
MetaData | mIncomingMetaData |
MetaData | mOutgoingMetaData |
QByteArray | mProtocol |
Detailed Description
There are two classes that specifies the protocol between application (job) and kioslave.
SlaveInterface is the class to use on the application end, SlaveBase is the one to use on the slave end.
Slave implementations should simply inherit SlaveBase
A call to foo() results in a call to slotFoo() on the other end.
Definition at line 46 of file tcpslavebase.h.
Member Enumeration Documentation
|
protected |
Enumerator | |
---|---|
ResultOk | |
ResultOverridden | |
ResultFailed | |
ResultFailedEarly |
Definition at line 63 of file tcpslavebase.h.
Constructor & Destructor Documentation
TCPSlaveBase::TCPSlaveBase | ( | const QByteArray & | protocol, |
const QByteArray & | poolSocket, | ||
const QByteArray & | appSocket, | ||
bool | autoSsl = false |
||
) |
Constructor.
- Parameters
-
autoSsl if true, will automatically invoke startSsl() right after connecting. In the absence of errors the use of SSL will therefore be transparent to higher layers.
Definition at line 194 of file tcpslavebase.cpp.
|
virtual |
Definition at line 214 of file tcpslavebase.cpp.
Member Function Documentation
|
protected |
Returns true when end of data is reached.
Definition at line 493 of file tcpslavebase.cpp.
|
protected |
Performs the initial TCP connection stuff and/or SSL handshaking as necessary.
- Parameters
-
protocol the protocol being used host hostname port port number
- Returns
- on succes, true is returned. on failure, false is returned and an appropriate error message is sent to the application.
Definition at line 302 of file tcpslavebase.cpp.
|
protected |
Connects to the specified host and port.
- Parameters
-
host host name port port number errorString if not NULL, this string will contain error information on why the connection request failed.
- Returns
- on success, 0 is returned. on failure, a KIO::Error code is returned. errorString, if not NULL, will contain the appropriate error message that can be sent back to the client.
- Since
- 4.7.2
Definition at line 315 of file tcpslavebase.cpp.
|
protected |
Close the connection and forget non-permanent data like the peer host.
Definition at line 455 of file tcpslavebase.cpp.
|
protected |
Will start SSL after connecting?
- Returns
- if so, true is returned. if not, false is returned.
Definition at line 478 of file tcpslavebase.cpp.
|
protected |
Determines whether or not we are still connected to the remote machine.
return true
if the socket is still active or false otherwise.
Definition at line 964 of file tcpslavebase.cpp.
|
protected |
Is the current connection using SSL?
- Returns
- if so, true is returned. if not, false is returned.
Definition at line 483 of file tcpslavebase.cpp.
|
protected |
the current port for this service
Definition at line 488 of file tcpslavebase.cpp.
|
protected |
Definition at line 252 of file tcpslavebase.cpp.
|
protected |
Same as read() except it reads data one line at a time.
Definition at line 278 of file tcpslavebase.cpp.
|
protected |
Sets the mode of the connection to blocking or non-blocking.
Be sure to call this function before calling connectToHost. Otherwise, this setting will not have any effect until the next connectToHost
.
- Parameters
-
b true to make the connection a blocking one, false otherwise.
Definition at line 979 of file tcpslavebase.cpp.
|
protected |
Return the socket object, if the class ever needs to do anything to it.
Definition at line 188 of file tcpslavebase.cpp.
|
protected |
Start using SSL on the connection.
You can use it right after connecting for classic, transparent to the protocol SSL. Calling it later can be used to implement e.g. SMTP's STARTTLS feature.
- Returns
- on success, true is returned. on failure, false is returned.
Definition at line 498 of file tcpslavebase.cpp.
|
protectedvirtual |
Reimplemented from KIO::SlaveBase.
Definition at line 988 of file tcpslavebase.cpp.
|
protected |
Wait for incoming data on the socket for the period specified by t
.
- Parameters
-
t length of time in seconds that we should monitor the socket before timing out.
- Returns
- true if any data arrived on the socket before the timeout value was reached, false otherwise.
Definition at line 971 of file tcpslavebase.cpp.
|
protected |
Definition at line 220 of file tcpslavebase.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:50:05 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.