KIO
#include <kpropertiesdialog.h>
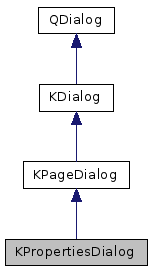
Public Slots | |
virtual void | slotCancel () |
virtual void | slotOk () |
Signals | |
void | applied () |
void | canceled () |
void | leaveModality () |
void | propertiesClosed () |
void | saveAs (const KUrl &oldUrl, KUrl &newUrl) |
Public Member Functions | |
KPropertiesDialog (const KFileItem &item, QWidget *parent=0) | |
KPropertiesDialog (const KFileItemList &_items, QWidget *parent=0) | |
KPropertiesDialog (const KUrl &_url, QWidget *parent=0) | |
KPropertiesDialog (const KUrl &_tempUrl, const KUrl &_currentDir, const QString &_defaultName, QWidget *parent=0) | |
KPropertiesDialog (const QString &title, QWidget *parent=0) | |
virtual | ~KPropertiesDialog () |
void | abortApplying () |
KUrl | currentDir () const |
QString | defaultName () const |
void | insertPlugin (KPropertiesDialogPlugin *plugin) |
KFileItem & | item () |
KFileItemList | items () const |
KUrl | kurl () const |
void | rename (const QString &_name) |
void | setFileNameReadOnly (bool ro) |
void | setFileSharingPage (QWidget *page) |
void | showFileSharingPage () |
void | updateUrl (const KUrl &_newUrl) |
Static Public Member Functions | |
static bool | canDisplay (const KFileItemList &_items) |
static bool | showDialog (const KFileItem &item, QWidget *parent=0, bool modal=true) |
static bool | showDialog (const KUrl &_url, QWidget *parent=0, bool modal=true) |
static bool | showDialog (const KFileItemList &_items, QWidget *parent=0, bool modal=true) |
Detailed Description
The main properties dialog class.
A Properties Dialog is a dialog which displays various information about a particular file or URL, or several files or URLs. This main class holds various related classes, which are instantiated in the form of tab entries in the tabbed dialog that this class provides. The various tabs themselves will let the user view, and sometimes change, information about the file or URL.
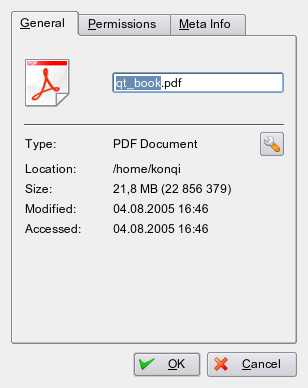
The best way to display the properties dialog is to use showDialog(). Otherwise, you should use (void)new KPropertiesDialog(...) It will take care of deleting itself when closed.
If you are looking for more flexibility, see KFileMetaInfo and KFileMetaInfoWidget.
Definition at line 57 of file kpropertiesdialog.h.
Constructor & Destructor Documentation
Brings up a Properties dialog, as shown above.
This is the normal constructor for file-manager type applications, where you have a KFileItem instance to work with. Normally you will use this method rather than the one below.
- Parameters
-
item file item whose properties should be displayed. parent is the parent of the dialog widget. name is the internal name.
Definition at line 207 of file kpropertiesdialog.cpp.
|
explicit |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.You use this constructor for cases where you have a number of items, rather than a single item.
Be careful which methods you use when passing a list of files or URLs, since some of them will only work on the first item in a list.
- Parameters
-
_items list of file items whose properties should be displayed. parent is the parent of the dialog widget. name is the internal name.
Definition at line 231 of file kpropertiesdialog.cpp.
Brings up a Properties dialog.
Convenience constructor for non-file-manager applications, where you have a KUrl rather than a KFileItem or KFileItemList.
- Parameters
-
_url the URL whose properties should be displayed parent is the parent of the dialog widget. name is the internal name.
IMPORTANT: This constructor, together with exec(), leads to a grave display bug (due to KIO::stat() being run before the dialog has all the necessary information). Do not use this combination for now. TODO: Check if the above is still true with Qt4. For local files with a known mimetype, simply create a KFileItem and pass it to the other constructor.
Definition at line 249 of file kpropertiesdialog.cpp.
KPropertiesDialog::KPropertiesDialog | ( | const KUrl & | _tempUrl, |
const KUrl & | _currentDir, | ||
const QString & | _defaultName, | ||
QWidget * | parent = 0 |
||
) |
Creates a properties dialog for a new .desktop file (whose name is not known yet), based on a template.
Special constructor for "File / New" in file-manager type applications.
- Parameters
-
_tempUrl template used for reading only _currentDir directory where the file will be written to _defaultName something to put in the name field, like mimetype.desktop parent is the parent of the dialog widget. name is the internal name.
Definition at line 264 of file kpropertiesdialog.cpp.
Creates an empty properties dialog (for applications that want use a standard dialog, but for things not doable via the plugin-mechanism).
- Parameters
-
title is the string display as the "filename" in the caption of the dialog. parent is the parent of the dialog widget. name is the internal name. modal tells the dialog whether it should be modal.
Definition at line 222 of file kpropertiesdialog.cpp.
|
virtual |
Cleans up the properties dialog and frees any associated resources, including the dialog itself.
Note that when a properties dialog is closed it cleans up and deletes itself.
Definition at line 377 of file kpropertiesdialog.cpp.
Member Function Documentation
void KPropertiesDialog::abortApplying | ( | ) |
To abort applying changes.
Definition at line 594 of file kpropertiesdialog.cpp.
|
signal |
This signal is emitted when the properties changes are applied (for example, with the OK button)
|
signal |
This signal is emitted when the properties changes are aborted (for example, with the Cancel button)
|
static |
Determine whether there are any property pages available for the given file items.
- Parameters
-
_items the list of items to check.
- Returns
- true if there are any property pages, otherwise false.
Definition at line 419 of file kpropertiesdialog.cpp.
KUrl KPropertiesDialog::currentDir | ( | ) | const |
If the dialog is being built from a template, this method returns the current directory.
If no template, it returns QString(). See the template form of the constructor.
- Returns
- the current directory or QString()
Definition at line 409 of file kpropertiesdialog.cpp.
QString KPropertiesDialog::defaultName | ( | ) | const |
If the dialog is being built from a template, this method returns the default name.
If no template, it returns QString(). See the template form of the constructor.
- Returns
- the default name or QString()
Definition at line 414 of file kpropertiesdialog.cpp.
void KPropertiesDialog::insertPlugin | ( | KPropertiesDialogPlugin * | plugin | ) |
Adds a "3rd party" properties plugin to the dialog.
Useful for extending the properties mechanism.
To create a new plugin type, inherit from the base class KPropertiesDialogPlugin and implement all the methods. If you define a service .desktop file for your plugin, you do not need to call insertPlugin().
- Parameters
-
plugin is a pointer to the KPropertiesDialogPlugin. The Properties dialog will do destruction for you. The KPropertiesDialogPlugin must have been created with the KPropertiesDialog as its parent.
- See also
- KPropertiesDialogPlugin
Definition at line 386 of file kpropertiesdialog.cpp.
KFileItem & KPropertiesDialog::item | ( | ) |
- Returns
- the file item for which the dialog is shown
Warning: this method returns the first item of the list. This means that you should use this only if you are sure the dialog is used for a single item. Otherwise, you probably want items() instead.
Definition at line 399 of file kpropertiesdialog.cpp.
KFileItemList KPropertiesDialog::items | ( | ) | const |
- Returns
- the items for which the dialog is shown
Definition at line 404 of file kpropertiesdialog.cpp.
KUrl KPropertiesDialog::kurl | ( | ) | const |
The URL of the file that has its properties being displayed.
This is only valid if the KPropertiesDialog was created/shown for one file or URL.
- Returns
- a parsed URL.
Definition at line 394 of file kpropertiesdialog.cpp.
|
signal |
|
signal |
This signal is emitted when the Properties Dialog is closed (for example, with OK or Cancel buttons)
void KPropertiesDialog::rename | ( | const QString & | _name | ) |
Renames the item to the specified name.
This can only be called if the dialog applies to a single file or URL.
- Parameters
-
_name new filename, encoded.
- See also
- FilePropsDialogPlugin::applyChanges
Definition at line 572 of file kpropertiesdialog.cpp.
Emitted before changes to oldUrl
are saved as newUrl
.
The receiver may change newUrl
to point to an alternative save location.
void KPropertiesDialog::setFileNameReadOnly | ( | bool | ro | ) |
Call this to make the filename lineedit readonly, to prevent the user from renaming the file.
- Parameters
-
ro true if the lineedit should be read only
Definition at line 366 of file kpropertiesdialog.cpp.
void KPropertiesDialog::setFileSharingPage | ( | QWidget * | page | ) |
Sets the file sharing page.
This page is shown when calling showFileSharingPage().
- Parameters
-
page the page to set
- See also
- showFileSharingPage
Definition at line 361 of file kpropertiesdialog.cpp.
|
static |
Immediately displays a Properties dialog using constructor with the same parameters.
On MS Windows, if item
points to a local file, native (non modal) property dialog is displayed (parent
and modal
are ignored in this case).
- Returns
- true on successful dialog displaying (can be false on win32).
Definition at line 281 of file kpropertiesdialog.cpp.
|
static |
Immediately displays a Properties dialog using constructor with the same parameters.
On MS Windows, if _url
points to a local file, native (non modal) property dialog is displayed (parent
and modal
are ignored in this case).
- Returns
- true on successful dialog displaying (can be false on win32).
Definition at line 301 of file kpropertiesdialog.cpp.
|
static |
Immediately displays a Properties dialog using constructor with the same parameters.
On MS Windows, if _items
has one element and this element points to a local file, native (non modal) property dialog is displayed (parent
and modal
are ignored in this case).
- Returns
- true on successful dialog displaying (can be false on win32).
Definition at line 318 of file kpropertiesdialog.cpp.
void KPropertiesDialog::showFileSharingPage | ( | ) |
Shows the page that was previously set by setFileSharingPage(), or does nothing if no page was set yet.
- See also
- setFileSharingPage
Definition at line 353 of file kpropertiesdialog.cpp.
|
virtualslot |
Called when the user presses 'Cancel'.
Definition at line 476 of file kpropertiesdialog.cpp.
|
virtualslot |
Called when the user presses 'Ok'.
Definition at line 430 of file kpropertiesdialog.cpp.
void KPropertiesDialog::updateUrl | ( | const KUrl & | _newUrl | ) |
Updates the item URL (either called by rename or because a global apps/mimelnk desktop file is being saved) Can only be called if the dialog applies to a single file or URL.
- Parameters
-
_newUrl the new URL
Definition at line 548 of file kpropertiesdialog.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:50:04 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.