KIO
#include <krun.h>
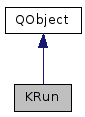
Classes | |
class | KRunPrivate |
Signals | |
void | error () |
void | finished () |
Public Member Functions | |
KRun (const KUrl &url, QWidget *window, mode_t mode=0, bool isLocalFile=false, bool showProgressInfo=true, const QByteArray &asn=QByteArray()) | |
virtual | ~KRun () |
void | abort () |
bool | autoDelete () const |
bool | hasError () const |
bool | hasFinished () const |
void | setAutoDelete (bool b) |
void | setEnableExternalBrowser (bool b) |
void | setPreferredService (const QString &desktopEntryName) |
void | setRunExecutables (bool b) |
void | setSuggestedFileName (const QString &fileName) |
QString | suggestedFileName () const |
QWidget * | window () const |
Static Public Member Functions | |
static QString | binaryName (const QString &execLine, bool removePath) |
static bool | checkStartupNotify (const QString &binName, const KService *service, bool *silent_arg, QByteArray *wmclass_arg) |
static bool | displayOpenWithDialog (const KUrl::List &lst, QWidget *window, bool tempFiles=false, const QString &suggestedFileName=QString(), const QByteArray &asn=QByteArray()) |
static bool | isExecutable (const QString &serviceType) |
static bool | isExecutableFile (const KUrl &url, const QString &mimetype) |
static QStringList | processDesktopExec (const KService &_service, const KUrl::List &_urls, bool tempFiles=false, const QString &suggestedFileName=QString()) |
static bool | run (const KService &service, const KUrl::List &urls, QWidget *window, bool tempFiles=false, const QString &suggestedFileName=QString(), const QByteArray &asn=QByteArray()) |
static bool | run (const QString &exec, const KUrl::List &urls, QWidget *window, const QString &name=QString(), const QString &icon=QString(), const QByteArray &asn=QByteArray()) |
static bool | runCommand (const QString &cmd, QWidget *window) |
static bool | runCommand (const QString &cmd, QWidget *window, const QString &workingDirectory) |
static bool | runCommand (const QString &cmd, const QString &execName, const QString &icon, QWidget *window, const QByteArray &asn=QByteArray()) |
static bool | runCommand (const QString &cmd, const QString &execName, const QString &icon, QWidget *window, const QByteArray &asn, const QString &workingDirectory) |
static bool | runUrl (const KUrl &url, const QString &mimetype, QWidget *window, bool tempFile=false, bool runExecutables=true, const QString &suggestedFileName=QString(), const QByteArray &asn=QByteArray()) |
static void | shellQuote (QString &str) |
Protected Slots | |
void | mimeTypeDetermined (const QString &mimeType) |
void | slotScanFinished (KJob *) |
void | slotScanMimeType (KIO::Job *, const QString &type) |
virtual void | slotStatResult (KJob *) |
void | slotTimeout () |
Protected Member Functions | |
bool | doScanFile () const |
virtual void | foundMimeType (const QString &type) |
virtual void | init () |
bool | initializeNextAction () const |
bool | isDirectory () const |
bool | isLocalFile () const |
KIO::Job * | job () |
virtual void | killJob () |
mode_t | mode () const |
bool | progressInfo () const |
virtual void | scanFile () |
void | setDoScanFile (bool scanFile) |
void | setError (bool error) |
void | setFinished (bool finished) |
void | setInitializeNextAction (bool initialize) |
void | setIsDirecory (bool isDirectory) |
void | setIsLocalFile (bool isLocalFile) |
void | setJob (KIO::Job *job) |
void | setMode (mode_t mode) |
void | setProgressInfo (bool progressInfo) |
void | setUrl (const KUrl &url) |
QTimer & | timer () |
KUrl | url () const |
Detailed Description
To open files with their associated applications in KDE, use KRun.
It can execute any desktop entry, as well as any file, using the default application or another application "bound" to the file type (or URL protocol).
In that example, the mimetype of the file is not known by the application, so a KRun instance must be created. It will determine the mimetype by itself. If the mimetype is known, or if you even know the service (application) to use for this file, use one of the static methods.
By default KRun uses auto deletion. It causes the KRun instance to delete itself when the it finished its task. If you allocate the KRun object on the stack you must disable auto deletion, otherwise it will crash.
Opens files with their associated applications in KDE
Constructor & Destructor Documentation
KRun::KRun | ( | const KUrl & | url, |
QWidget * | window, | ||
mode_t | mode = 0 , |
||
bool | isLocalFile = false , |
||
bool | showProgressInfo = true , |
||
const QByteArray & | asn = QByteArray() |
||
) |
- Parameters
-
url the URL of the file or directory to 'run' window The top-level widget of the app that invoked this object. It is used to make sure private information like passwords are properly handled per application. mode The st_mode
field ofstruct stat
. If you don't know this set it to 0.isLocalFile If this parameter is set to false
thenurl
is examined to find out whether it is a local URL or not. This flag is just used to improve speed, since the function KUrl::isLocalFile is a bit slow.showProgressInfo Whether to show progress information when determining the type of the file (i.e. when using KIO::stat and KIO::mimetype) Before you set this to false to avoid a dialog box, think about a very slow FTP server... It is always better to provide progress info in such cases. asn Application startup notification id, if available (otherwise "").
|
virtual |
Member Function Documentation
void KRun::abort | ( | ) |
bool KRun::autoDelete | ( | ) | const |
Given a full command line (e.g.
the Exec= line from a .desktop file), extract the name of the binary being run.
- Parameters
-
execLine the full command line removePath if true, remove a (relative or absolute) path. E.g. /usr/bin/ls becomes ls.
- Returns
- the name of the binary to run
|
static |
Display the Open-With dialog for those URLs, and run the chosen application.
- Parameters
-
lst the list of applications to run window The top-level widget of the app that invoked this object. tempFiles if true and lst are local files, they will be deleted when the application exits. suggestedFileName see setSuggestedFileName asn Application startup notification id, if any (otherwise "").
- Returns
- false if the dialog was canceled
|
protected |
Returns whether the file shall be scanned.
- Deprecated:
- not useful in public API
|
signal |
Emitted when the operation had an error.
- See also
- hasError()
|
signal |
Emitted when the operation finished.
This signal is emitted in all cases of completion, whether successful or with error.
- See also
- hasFinished()
|
protectedvirtual |
Called if the mimetype has been detected.
The function runs the application associated with this mimetype. Reimplement this method to implement a different behavior, like opening the component for displaying the URL embedded.
Important: call setFinished(true) once you are done! Usually at the end of the foundMimeType reimplementation, but if the reimplementation is asynchronous (e.g. uses KIO jobs) then it can be called later instead.
Note: if that service failed, we'll go to runUrl below to maybe find another service, even though an error dialog box was already displayed. That's good if runUrl tries another service, but it's not good if it tries the same one :}
bool KRun::hasError | ( | ) | const |
bool KRun::hasFinished | ( | ) | const |
Returns true if the KRun instance has finished.
- Returns
- true if the KRun instance has finished
- See also
- finished()
|
protectedvirtual |
|
protected |
- Deprecated:
- not useful in public API
|
protected |
Returns whether the url
of mimetype
is executable.
To be executable the file must pass the following rules:
- Must reside on the local filesystem.
- Must be marked as executable for the user by the filesystem.
- The mime type must inherit application/x-executable or application/x-executable-script. To allow a script to run when the above rules are satisfied add the entry to the mimetype's desktop file.X-KDE-IsAlso=application/x-executable-script
|
protected |
|
protectedvirtual |
|
protectedslot |
|
protected |
|
static |
Processes a Exec= line as found in .desktop files.
- Parameters
-
_service the service to extract information from. _urls The urls the service should open. tempFiles if true and urls are local files, they will be deleted when the application exits. suggestedFileName see setSuggestedFileName
- Returns
- a list of arguments suitable for KProcess::setProgram().
|
protected |
|
static |
Open a list of URLs with a certain service (application).
- Parameters
-
service the service to run urls the list of URLs, can be empty (app launched without argument) window The top-level widget of the app that invoked this object. tempFiles if true and urls are local files, they will be deleted when the application exits. suggestedFileName see setSuggestedFileName asn Application startup notification id, if any (otherwise "").
- Returns
true
on success,false
on error
|
static |
Open a list of URLs with an executable.
- Parameters
-
exec the name of the executable, for example "/usr/bin/netscape %u". Don't forget to include the u if you know that the applications supports URLs. Otherwise, non-local urls will first be downloaded to a temp file (using kioexec). urls the list of URLs to open, can be empty (app launched without argument) window The top-level widget of the app that invoked this object. name the logical name of the application, for example "Netscape 4.06". icon the icon which should be used by the application. asn Application startup notification id, if any (otherwise "").
- Returns
true
on success,false
on error
Run the given shell command and notifies KDE of the starting of the application.
If the program to be called doesn't exist, an error box will be displayed.
Use only when you know the full command line. Otherwise use the other static methods, or KRun's constructor.
cmd
must be a shell command. You must not append "&" to it, since the function will do that for you.
- Parameters
-
window The top-level widget of the app that invoked this object.
- Returns
true
on success,false
on error
|
static |
Same as the other runCommand(), but it also takes the name of the binary, to display an error message in case it couldn't find it.
- Parameters
-
cmd must be a shell command. You must not append "&" to it, since the function will do that for you. execName the name of the executable icon icon for app starting notification window The top-level widget of the app that invoked this object. asn Application startup notification id, if any (otherwise "").
- Returns
true
on success,false
on error
|
static |
Overload that also takes a working directory, so that a command like "kwrite file.txt" finds file.txt from the right place.
- Parameters
-
workingDirectory the working directory for the started process. The default (if passing an empty string) is the user's document path.
- Since
- 4.4
|
static |
Open the given URL.
This function is used after the mime type is found out. It will search for all services which can handle the mime type and call run() afterwards.
- Parameters
-
url the URL to open mimetype the mime type of the resource window The top-level widget of the app that invoked this object. tempFile if true and url is a local file, it will be deleted when the launched application exits. runExecutables if false then local .desktop files, executables and shell scripts will not be run. See also isExecutable(). suggestedFileName see setSuggestedFileName asn Application startup notification id, if any (otherwise "").
- Returns
true
on success,false
on error
|
protectedvirtual |
void KRun::setAutoDelete | ( | bool | b | ) |
|
protected |
Indicate that the next action is to scan the file.
- Deprecated:
- not useful in public API
void KRun::setEnableExternalBrowser | ( | bool | b | ) |
|
protected |
|
protected |
|
protected |
- Deprecated:
- not useful in public API
|
protected |
Sets whether it is a directory.
- Deprecated:
- typo in the name, and not useful as a public method
|
protected |
|
protected |
|
protected |
void KRun::setPreferredService | ( | const QString & | desktopEntryName | ) |
Set the preferred service for opening this URL, after its mimetype will have been found by KRun.
IMPORTANT: the service is only used if its configuration says it can handle this mimetype. This is used for instance for the X-KDE-LastOpenedWith key in the recent documents list, or for the app selection in KParts::BrowserOpenOrSaveQuestion.
- Parameters
-
desktopEntryName the desktopEntryName of the service, e.g. "kate".
|
protected |
void KRun::setRunExecutables | ( | bool | b | ) |
Sets whether executables, .desktop files or shell scripts should be run by KRun.
This is enabled by default.
- Parameters
-
b whether to run executable files or not.
- See also
- isExecutable()
void KRun::setSuggestedFileName | ( | const QString & | fileName | ) |
Sets the file name to use in the case of downloading the file to a tempfile in order to give to a non-url-aware application.
Some apps rely on the extension to determine the mimetype of the file. Usually the file name comes from the URL, but in the case of the HTTP Content-Disposition header, we need to override the file name.
|
protected |
|
static |
Quotes a string for the shell.
An empty string will not be quoted.
- Deprecated:
- Use KShell::quoteArg() instead. Note that this function behaves differently for empty arguments and returns the result differently.
- Parameters
-
str the string to quote. The quoted string will be written here
|
protectedslot |
|
protectedvirtualslot |
|
protectedslot |
QString KRun::suggestedFileName | ( | ) | const |
|
protected |
Returns the timer object.
- Deprecated:
- setFinished(true) now takes care of the timer().start(0), so this can be removed.
QWidget * KRun::window | ( | ) | const |
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:50:04 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.