KIO
#include <kurlcombobox.h>
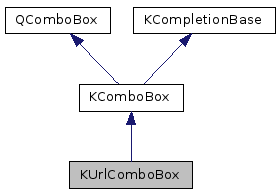
Public Types | |
enum | Mode { Files = -1, Directories = 1, Both = 0 } |
enum | OverLoadResolving { RemoveTop, RemoveBottom } |
![]() | |
typedef QMap< KeyBindingType, KShortcut > | KeyBindingMap |
enum | KeyBindingType |
Signals | |
void | urlActivated (const KUrl &url) |
![]() | |
void | aboutToShowContextMenu (QMenu *p) |
void | completion (const QString &) |
void | completionModeChanged (KGlobalSettings::Completion) |
void | returnPressed (const QString &) |
void | returnPressed () |
void | substringCompletion (const QString &) |
void | textRotation (KCompletionBase::KeyBindingType) |
Public Member Functions | |
KUrlComboBox (Mode mode, QWidget *parent=0) | |
KUrlComboBox (Mode mode, bool rw, QWidget *parent=0) | |
~KUrlComboBox () | |
void | addDefaultUrl (const KUrl &url, const QString &text=QString()) |
void | addDefaultUrl (const KUrl &url, const QIcon &icon, const QString &text=QString()) |
int | maxItems () const |
void | removeUrl (const KUrl &url, bool checkDefaultURLs=true) |
virtual void | setCompletionObject (KCompletion *compObj, bool hsig=true) |
void | setDefaults () |
void | setMaxItems (int) |
void | setUrl (const KUrl &url) |
void | setUrls (const QStringList &urls) |
void | setUrls (const QStringList &urls, OverLoadResolving remove) |
QStringList | urls () const |
![]() | |
KComboBox (QWidget *parent=0) | |
KComboBox (bool rw, QWidget *parent=0) | |
virtual | ~KComboBox () |
virtual | ~KCompletionBase () |
void | addUrl (const KUrl &url) |
void | addUrl (const QIcon &icon, const KUrl &url) |
bool | autoCompletion () const |
void | changeURL (const QPixmap &pixmap, const KUrl &url, int index) |
void | changeUrl (int index, const KUrl &url) |
void | changeUrl (int index, const QIcon &icon, const KUrl &url) |
void | changeURL (const KUrl &url, int index) |
KCompletionBox * | completionBox (bool create=true) |
KGlobalSettings::Completion | completionMode () const |
KCompletion * | completionObject (bool hsig=true) |
KCompletion * | compObj () const |
bool | contains (const QString &text) const |
int | cursorPosition () const |
bool | emitSignals () const |
virtual bool | eventFilter (QObject *, QEvent *) |
KShortcut | getKeyBinding (KeyBindingType item) const |
bool | handleSignals () const |
void | insertURL (const QPixmap &pixmap, const KUrl &url, int index=-1) |
void | insertUrl (int index, const QIcon &icon, const KUrl &url) |
void | insertUrl (int index, const KUrl &url) |
void | insertURL (const KUrl &url, int index=-1) |
bool | isCompletionObjectAutoDeleted () const |
KCompletionBase () | |
virtual void | setAutoCompletion (bool autocomplete) |
void | setAutoDeleteCompletionObject (bool autoDelete) |
virtual void | setCompletedItems (const QStringList &items, bool autoSuggest=true)=0 |
virtual void | setCompletedText (const QString &text)=0 |
virtual void | setCompletionMode (KGlobalSettings::Completion mode) |
virtual void | setContextMenuEnabled (bool showMenu) |
void | setEditable (bool editable) |
void | setEditUrl (const KUrl &url) |
void | setEnableSignals (bool enable) |
virtual void | setHandleSignals (bool handle) |
bool | setKeyBinding (KeyBindingType item, const KShortcut &key) |
virtual void | setLineEdit (QLineEdit *) |
void | setTrapReturnKey (bool trap) |
void | setUrlDropsEnabled (bool enable) |
bool | trapReturnKey () const |
bool | urlDropsEnabled () const |
void | useGlobalKeyBindings () |
Protected Member Functions | |
virtual void | mouseMoveEvent (QMouseEvent *event) |
virtual void | mousePressEvent (QMouseEvent *event) |
![]() | |
virtual void | create (WId=0, bool initializeWindow=true, bool destroyOldWindow=true) |
KCompletionBase * | delegate () const |
KeyBindingMap | getKeyBindings () const |
virtual QSize | minimumSizeHint () const |
virtual void | setCompletedText (const QString &, bool) |
void | setDelegate (KCompletionBase *delegate) |
virtual void | virtual_hook (int id, void *data) |
virtual void | wheelEvent (QWheelEvent *ev) |
Properties | |
int | maxItems |
QStringList | urls |
![]() | |
bool | autoCompletion |
bool | trapReturnKey |
bool | urlDropsEnabled |
Additional Inherited Members | |
![]() | |
void | rotateText (KCompletionBase::KeyBindingType type) |
void | setCompletedItems (const QStringList &items, bool autosubject=true) |
virtual void | setCompletedText (const QString &) |
void | setCurrentItem (const QString &item, bool insert=false, int index=-1) |
![]() | |
virtual void | makeCompletion (const QString &) |
Detailed Description
This combobox shows a number of recent URLs/directories, as well as some default directories.
It will manage the default dirs root-directory, home-directory and Desktop-directory, as well as a number of URLs set via setUrls() and one additional entry to be set via setUrl().
This widget forces the layout direction to be Qt::LeftToRight instead of inheriting the layout direction like a normal widget. This means that even in RTL desktops the widget will be displayed in LTR mode, as generally URLs are LTR by nature.
A combo box showing a number of recent URLs/directories
Definition at line 47 of file kurlcombobox.h.
Member Enumeration Documentation
enum KUrlComboBox::Mode |
This enum describes which kind of items is shown in the combo box.
Enumerator | |
---|---|
Files | |
Directories | |
Both |
Definition at line 57 of file kurlcombobox.h.
This Enumeration is used in setUrl() to determine which items will be removed when the given list is larger than maxItems().
- RemoveTop means that items will be removed from top
- RemoveBottom means, that items will be removed from the bottom
Enumerator | |
---|---|
RemoveTop | |
RemoveBottom |
Definition at line 65 of file kurlcombobox.h.
Constructor & Destructor Documentation
Constructs a KUrlComboBox.
- Parameters
-
mode is either Files, Directories or Both and controls the following behavior: - Files all inserted URLs will be treated as files, therefore the url shown in the combo will never show a trailing / the icon will be the one associated with the file's mimetype.
- Directories all inserted URLs will be treated as directories, will have a trailing slash in the combobox. The current directory will show the "open folder" icon, other directories the "folder" icon.
- Both Don't mess with anything, just show the url as given.
parent The parent object of this widget.
Definition at line 75 of file kurlcombobox.cpp.
Definition at line 82 of file kurlcombobox.cpp.
KUrlComboBox::~KUrlComboBox | ( | ) |
Destructs the combo box.
Definition at line 89 of file kurlcombobox.cpp.
Member Function Documentation
Adds a url that will always be shown in the combobox, it can't be "rotated away".
Default urls won't be returned in urls() and don't have to be set via setUrls(). If you want to specify a special pixmap, use the overloaded method with the pixmap parameter. Default URLs will be inserted into the combobox by setDefaults()
Definition at line 134 of file kurlcombobox.cpp.
void KUrlComboBox::addDefaultUrl | ( | const KUrl & | url, |
const QIcon & | icon, | ||
const QString & | text = QString() |
||
) |
Adds a url that will always be shown in the combobox, it can't be "rotated away".
Default urls won't be returned in urls() and don't have to be set via setUrls(). If you don't need to specify a pixmap, use the overloaded method without the pixmap parameter. Default URLs will be inserted into the combobox by setDefaults()
Definition at line 140 of file kurlcombobox.cpp.
int KUrlComboBox::maxItems | ( | ) | const |
- Returns
- the maximum of items the combobox handles.
- See also
- setMaxItems
|
protectedvirtual |
Definition at line 403 of file kurlcombobox.cpp.
|
protectedvirtual |
Definition at line 386 of file kurlcombobox.cpp.
Removes any occurrence of url
.
If checkDefaultUrls
is false default-urls won't be removed.
Definition at line 355 of file kurlcombobox.cpp.
|
virtual |
Reimplemented from KComboBox (from KCompletion)
Reimplemented from KComboBox.
Definition at line 375 of file kurlcombobox.cpp.
void KUrlComboBox::setDefaults | ( | ) |
Clears all items and inserts the default urls into the combo.
Will be called implicitly upon the first call to setUrls() or setUrl()
- See also
- addDefaultUrl
Definition at line 157 of file kurlcombobox.cpp.
void KUrlComboBox::setMaxItems | ( | int | max | ) |
Sets how many items should be handled and displayed by the combobox.
- See also
- maxItems
Definition at line 329 of file kurlcombobox.cpp.
void KUrlComboBox::setUrl | ( | const KUrl & | url | ) |
Sets the current url.
This combo handles exactly one url additionally to the default items and those set via setUrls(). So you can call setUrl() as often as you want, it will always replace the previous one set via setUrl(). If url
is already in the combo, the last item will stay there and the existing item becomes the current item. The current item will always have the open-directory-pixmap as icon.
Note that you won't receive any signals, e.g. textChanged(), returnPressed() or activated() upon calling this method.
Definition at line 241 of file kurlcombobox.cpp.
void KUrlComboBox::setUrls | ( | const QStringList & | urls | ) |
Inserts urls
into the combobox below the "default urls" (see addDefaultUrl).
If the list of urls contains more items than maxItems, the first items will be stripped.
Definition at line 169 of file kurlcombobox.cpp.
void KUrlComboBox::setUrls | ( | const QStringList & | urls, |
OverLoadResolving | remove | ||
) |
Inserts urls
into the combobox below the "default urls" (see addDefaultUrl).
If the list of urls contains more items than maxItems, the remove
parameter determines whether the first or last items will be stripped.
Definition at line 174 of file kurlcombobox.cpp.
|
signal |
Emitted when an item was clicked at.
- Parameters
-
url is the url of the now current item. If it is a local url, it won't have a protocol (file:/), otherwise it will.
QStringList KUrlComboBox::urls | ( | ) | const |
- Returns
- a list of all urls currently handled. The list contains at most maxItems() items. Use this to save the list of urls in a config-file and reinsert them via setUrls() next time. Note that all default urls set via addDefaultUrl() are not returned, they will automatically be set via setUrls() or setUrl(). You will always get fully qualified urls, i.e. with protocol like file:/
Property Documentation
|
readwrite |
Definition at line 51 of file kurlcombobox.h.
|
readwrite |
Definition at line 50 of file kurlcombobox.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:50:04 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.