KParts
#include <part.h>
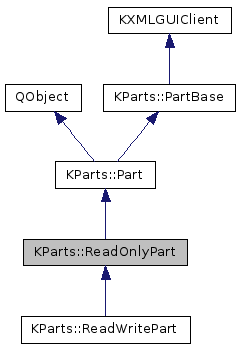
Public Slots | |
virtual bool | openUrl (const KUrl &url) |
Signals | |
void | canceled (const QString &errMsg) |
void | completed () |
void | completed (bool pendingAction) |
void | started (KIO::Job *) |
void | urlChanged (const KUrl &url) |
![]() | |
void | setStatusBarText (const QString &text) |
void | setWindowCaption (const QString &caption) |
Protected Member Functions | |
ReadOnlyPart (ReadOnlyPartPrivate &dd, QObject *parent) | |
void | abortLoad () |
virtual void | guiActivateEvent (GUIActivateEvent *event) |
bool | isLocalFileTemporary () const |
QString | localFilePath () const |
virtual bool | openFile () |
void | setLocalFilePath (const QString &localFilePath) |
void | setLocalFileTemporary (bool temp) |
void | setUrl (const KUrl &url) |
![]() | |
Part (PartPrivate &dd, QObject *parent) | |
virtual void | customEvent (QEvent *event) |
QWidget * | hostContainer (const QString &containerName) |
void | loadPlugins () |
virtual void | partActivateEvent (PartActivateEvent *event) |
virtual void | partSelectEvent (PartSelectEvent *event) |
virtual void | setWidget (QWidget *widget) |
![]() | |
PartBase (PartBasePrivate &dd) | |
void | loadPlugins (QObject *parent, KXMLGUIClient *parentGUIClient, const KComponentData &componentData) |
virtual void | setComponentData (const KComponentData &componentData) |
virtual void | setComponentData (const KComponentData &componentData, bool loadPlugins) |
void | setPluginInterfaceVersion (int version) |
void | setPluginLoadingMode (PluginLoadingMode loadingMode) |
![]() | |
void | loadStandardsXmlFile () |
virtual void | setDOMDocument (const QDomDocument &document, bool merge=false) |
virtual void | setLocalXMLFile (const QString &file) |
virtual void | setXML (const QString &document, bool merge=false) |
virtual void | setXMLFile (const QString &file, bool merge=false, bool setXMLDoc=true) |
virtual void | stateChanged (const QString &newstate, ReverseStateChange reverse=StateNoReverse) |
virtual void | virtual_hook (int id, void *data) |
Properties | |
KUrl | url |
Additional Inherited Members | |
![]() | |
enum | ReverseStateChange |
![]() | |
static QString | findMostRecentXMLFile (const QStringList &files, QString &doc) |
![]() | |
enum | PluginLoadingMode { DoNotLoadPlugins = 0, LoadPlugins = 1, LoadPluginsIfEnabled = 2 } |
![]() | |
void | slotWidgetDestroyed () |
![]() | |
PartBasePrivate * | d_ptr |
Detailed Description
Base class for any "viewer" part.
This class takes care of network transparency for you, in the simplest way (downloading to a temporary file, then letting the part load from the temporary file). To use the built-in network transparency, you only need to implement openFile(), not openUrl().
To implement network transparency differently (e.g. for progressive loading, like a web browser does for instance), or to prevent network transparency (but why would you do that?), you can override openUrl().
KParts Application can use the signals to show feedback while the URL is being loaded.
ReadOnlyPart handles the window caption by setting it to the current URL (set in openUrl(), and each time the part is activated). If you want another caption, set it in openFile() and (if the part might ever be used with a part manager) in guiActivateEvent()
Constructor & Destructor Documentation
|
explicit |
|
protected |
Member Function Documentation
OpenUrlArguments KParts::ReadOnlyPart::arguments | ( | ) | const |
BrowserExtension * ReadOnlyPart::browserExtension | ( | ) | const |
|
signal |
Emit this if loading is canceled by the user or by an error.
- Parameters
-
errMsg the error message, empty if the user canceled the loading voluntarily.
bool ReadOnlyPart::closeStream | ( | ) |
|
virtual |
Called when closing the current url (e.g.
document), for instance when switching to another url (note that openUrl() calls it automatically in this case). If the current URL is not fully loaded yet, aborts loading. Deletes the temporary file used when the url is remote.
- Returns
- always true, but the return value exists for reimplementations
Reimplemented in KParts::ReadWritePart.
|
signal |
Emit this when you have completed loading data.
Hosting apps will want to know when the process of loading the data is finished, so that they can access the data when everything is loaded.
|
signal |
Same as the above signal except it indicates whether there is a pending action to be executed on a delay timer.
An example of this is the meta-refresh tags on web pages used to reload/redirect after a certain period of time. This signal is useful if you want to give the user the ability to cancel such pending actions.
pendingAction
true if a pending action exists, false otherwise.
|
protectedvirtual |
Reimplemented from Part, so that the window caption is set to the current url (decoded) when the part is activated This is the usual behavior in 99% of the apps Reimplement if you don't like it - test for event->activated() !
Technical note : this is done with GUIActivateEvent and not with PartActivateEvent because it's handled by the mainwindow (which gets the even after the PartActivateEvent events have been sent)
Reimplemented from KParts::Part.
|
protected |
bool ReadOnlyPart::isProgressInfoEnabled | ( | ) | const |
|
protected |
|
protectedvirtual |
Initiate sending data to this part.
This is an alternative to openUrl, which allows the user of the part to load the data itself, and send it progressively to the part.
- Parameters
-
mimeType the type of data that is going to be sent to this part. url the URL representing this data. Although not directly used, every ReadOnlyPart has a URL (see url()), so this simply sets it.
- Returns
- true if the part supports progressive loading and accepts data, false otherwise.
Only reimplement openUrl if you don't want the network transparency support to download from the url into a temporary file (when the url isn't local).
Otherwise, reimplement openFile() only .
If you reimplement it, don't forget to set the caption, usually with emit setWindowCaption( url.prettyUrl() );
void KParts::ReadOnlyPart::setArguments | ( | const OpenUrlArguments & | arguments | ) |
|
protected |
|
protected |
void ReadOnlyPart::setProgressInfoEnabled | ( | bool | show | ) |
|
protected |
|
signal |
The part emits this when starting data.
If using a KIO::Job, it sets the job in the signal, so that progress information can be shown. Otherwise, job is 0.
KUrl KParts::ReadOnlyPart::url | ( | ) | const |
Returns the URL currently opened in this part.
- Returns
- The current URL.
|
signal |
Emitted by the part when url() changes.
- Since
- 4.10
bool ReadOnlyPart::writeStream | ( | const QByteArray & | data | ) |
Property Documentation
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:50:42 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.