Nepomuk-Core
#include <Nepomuk2/Query/Term>
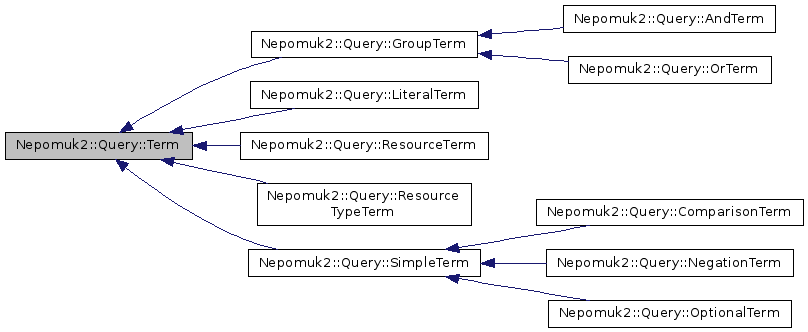
Public Types | |
enum | Type { Invalid, Literal, Resource, And, Or, Comparison, ResourceType, Negation, Optional } |
Static Public Member Functions | |
static Term | fromString (const QString &s) |
static Term | fromVariant (const Variant &variant) |
Related Functions | |
(Note that these are not member functions.) | |
static Term | fromProperty (const Types::Property &property, const Variant &variant) |
Detailed Description
The base class for all term types.
Queries are build from Term instances. A Term can have one of multiple types and subterms. See Term::Type for details on the different Term types.
- Since
- 4.4
Member Enumeration Documentation
The type of a term identifying its meaning.
- See also
- type()
Enumerator | |
---|---|
Invalid |
An invalid term matching nothing. |
Literal |
A literal term is the simplest form of Term. It simply contains a literal value.
|
Resource |
A resource term matches one resource by URI.
|
And |
Match all resources that match all sub terms.
|
Or |
Match all resources that match one of the sub terms.
|
Comparison |
A comparison. The comparison operator needs to be specified in addition. A single subterm specifies the resource or value to match.
|
ResourceType |
Matches all resources of a specific type.
|
Negation |
A negation term inverts the meaning of its sub term.
|
Optional |
An optional term which marks its sub term as optional.
|
Constructor & Destructor Documentation
Nepomuk2::Query::Term::Term | ( | const Term & | other | ) |
Member Function Documentation
|
static |
Parse a Term that has been encoded as a string via toString().
- Warning
- This method can NOT parse SPARQL syntax.
- See also
- toString()
- Since
- 4.5
|
static |
Construct a Term from a Variant value.
This is a convenience method that simplifies handling Nepomuk values. However, list variants are not supported and will result in an invalid Term.
- Returns
- A ResourceTerm in case
variant
is a resource, a LiteralTerm ifvariant
is a supported literal value, or an invalid Term ifvariant
is invalid or a list.
- Since
- 4.6
bool Nepomuk2::Query::Term::isAndTerm | ( | ) | const |
bool Nepomuk2::Query::Term::isComparisonTerm | ( | ) | const |
- Returns
true
if this term is a ComparisonTerm.
bool Nepomuk2::Query::Term::isLiteralTerm | ( | ) | const |
- Returns
true
if this term is a LiteralTerm.
bool Nepomuk2::Query::Term::isNegationTerm | ( | ) | const |
- Returns
true
if this term is a NegationTerm.
bool Nepomuk2::Query::Term::isOptionalTerm | ( | ) | const |
bool Nepomuk2::Query::Term::isOrTerm | ( | ) | const |
bool Nepomuk2::Query::Term::isResourceTerm | ( | ) | const |
- Returns
true
if this term is a ResourceTerm.
bool Nepomuk2::Query::Term::isResourceTypeTerm | ( | ) | const |
- Returns
true
if this term is a ResourceTypeTerm.
bool Nepomuk2::Query::Term::isValid | ( | ) | const |
bool Nepomuk2::Query::Term::operator!= | ( | const Term & | term | ) | const |
QDebug Nepomuk2::Query::Term::operator<< | ( | QDebug | dbg | ) | const |
Nepomuk2::Query::Term & Nepomuk2::Query::Term::operator= | ( | const Term & | other | ) |
bool Nepomuk2::Query::Term::operator== | ( | const Term & | term | ) | const |
Nepomuk2::Query::Term Nepomuk2::Query::Term::optimized | ( | ) | const |
Optimizes the term without changing its meaning.
This removes redundant terms such as NegationTerm and OptionalTerm nestings and flattens AndTerm and OrTerm hierarchies.
- Returns
- An optimized version of this term.
- Since
- 4.6
- See also
- Query::optimized()
void Nepomuk2::Query::Term::setUserData | ( | const QString & | key, |
const QVariant & | value | ||
) |
Nepomuk2::Query::AndTerm Nepomuk2::Query::Term::toAndTerm | ( | ) | const |
Nepomuk2::Query::AndTerm & Nepomuk2::Query::Term::toAndTerm | ( | ) |
Nepomuk2::Query::ComparisonTerm Nepomuk2::Query::Term::toComparisonTerm | ( | ) | const |
Interpret this term as a ComparisonTerm.
- Returns
- A copy of this term if its type is Comparison, a new ComparisonTerm otherwise.
Nepomuk2::Query::ComparisonTerm & Nepomuk2::Query::Term::toComparisonTerm | ( | ) |
Convert this term into a ComparisonTerm.
If type is not Comparison it will be changed and the result is a new ComparisonTerm.
- Returns
- A reference to this term as a ComparisonTerm.
Nepomuk2::Query::LiteralTerm Nepomuk2::Query::Term::toLiteralTerm | ( | ) | const |
Interpret this term as a LiteralTerm.
- Returns
- A copy of this term if its type is Literal, a new LiteralTerm otherwise.
Nepomuk2::Query::LiteralTerm & Nepomuk2::Query::Term::toLiteralTerm | ( | ) |
Convert this term into a LiteralTerm.
If type is not Literal it will be changed and the result is a new LiteralTerm.
- Returns
- A reference to this term as a LiteralTerm.
Nepomuk2::Query::NegationTerm Nepomuk2::Query::Term::toNegationTerm | ( | ) | const |
Interpret this term as a NegationTerm.
- Returns
- A copy of this term if its type is Negation, a new NegationTerm otherwise.
Nepomuk2::Query::NegationTerm & Nepomuk2::Query::Term::toNegationTerm | ( | ) |
Convert this term into a NegationTerm.
If type is not Negation it will be changed and the result is a new NegationTerm.
- Returns
- A reference to this term as a NegationTerm.
Nepomuk2::Query::OptionalTerm Nepomuk2::Query::Term::toOptionalTerm | ( | ) | const |
Interpret this term as a OptionalTerm.
- Returns
- A copy of this term if its type is Optional, a new OptionalTerm otherwise.
- Since
- 4.5
Nepomuk2::Query::OptionalTerm & Nepomuk2::Query::Term::toOptionalTerm | ( | ) |
Convert this term into a OptionalTerm.
If type is not Optional it will be changed and the result is a new OptionalTerm.
- Returns
- A reference to this term as a OptionalTerm.
- Since
- 4.5
Nepomuk2::Query::OrTerm Nepomuk2::Query::Term::toOrTerm | ( | ) | const |
Nepomuk2::Query::OrTerm & Nepomuk2::Query::Term::toOrTerm | ( | ) |
Nepomuk2::Query::ResourceTerm Nepomuk2::Query::Term::toResourceTerm | ( | ) | const |
Interpret this term as a ResourceTerm.
- Returns
- A copy of this term if its type is Resource, a new ResourceTerm otherwise.
Nepomuk2::Query::ResourceTerm & Nepomuk2::Query::Term::toResourceTerm | ( | ) |
Convert this term into a ResourceTerm.
If type is not Resource it will be changed and the result is a new ResourceTerm.
- Returns
- A reference to this term as a ResourceTerm.
Nepomuk2::Query::ResourceTypeTerm Nepomuk2::Query::Term::toResourceTypeTerm | ( | ) | const |
Interpret this term as a ResourceTypeTerm.
- Returns
- A copy of this term if its type is ClassType, a new ResourceTypeTerm otherwise.
Nepomuk2::Query::ResourceTypeTerm & Nepomuk2::Query::Term::toResourceTypeTerm | ( | ) |
Convert this term into a ResourceTypeTerm.
If type is not ClassType it will be changed and the result is a new ResourceTypeTerm.
- Returns
- A reference to this term as a ResourceTypeTerm.
QString Nepomuk2::Query::Term::toString | ( | ) | const |
Encode the Term in a string.
Be aware that this does NOT create a SPARQL query. The returned string can be used to serialize terms that can later be read via fromString().
- See also
- fromString()
- Since
- 4.5
Nepomuk2::Query::Term::Type Nepomuk2::Query::Term::type | ( | ) | const |
QVariant Nepomuk2::Query::Term::userData | ( | const QString & | key | ) | const |
Friends And Related Function Documentation
|
related |
Create a term using a Types::Property and a Variant.
Sadly this cannot be modelled as an operator since it would clash with Entity::operator==().
- Parameters
-
property The property to be used in the ComparisonTerm. variant The value to be compared to. Either ResourceTerm or LiteralTerm is used. List variants (Variant::isList()) are handled via an AndTerm meaning all values need to match.
- Since
- 4.6
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:48:09 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.