Nepomuk-Core
#include <Nepomuk2/Resource>
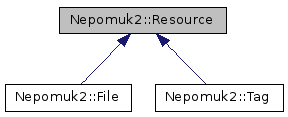
Public Member Functions | |
Resource () | |
Resource (const Resource &) | |
Resource (const QString &pathOrIdentifier, const QUrl &type=QUrl()) | |
Resource (const QUrl &uri, const QUrl &type=QUrl()) | |
Resource (ResourceData *) | |
virtual | ~Resource () |
void | addIdentifier (const QString &value) |
void | addIsRelated (const Resource &value) |
void | addProperty (const QUrl &uri, const Variant &value) |
void | addSymbol (const QString &value) |
void | addTag (const Tag &value) |
void | addType (const QUrl &type) |
QString | description () const |
bool | exists () const |
QString | genericDescription () const |
QString | genericIcon () const |
QString | genericLabel () const |
bool | hasProperty (const QUrl &uri) const |
bool | hasProperty (const Types::Property &p, const Variant &v) const |
bool | hasType (const QUrl &typeUri) const |
QStringList | identifiers () const |
void | increaseUsageCount () |
bool | isFile () const |
QList< Resource > | isRelatedOf () const |
QList< Resource > | isRelateds () const |
bool | isValid () const |
QString | label () const |
bool | operator!= (const Resource &) const |
Resource & | operator= (const Resource &other) |
Resource & | operator= (const QUrl &uri) |
bool | operator== (const Resource &) const |
QHash< QUrl, Variant > | properties () const |
Variant | property (const QUrl &uri) const |
quint32 | rating () const |
void | remove () |
void | removeProperty (const QUrl &uri) |
void | removeProperty (const QUrl &uri, const Variant &value) |
void | setDescription (const QString &value) |
void | setIdentifiers (const QStringList &value) |
void | setIsRelateds (const QList< Resource > &value) |
void | setLabel (const QString &value) |
void | setProperty (const QUrl &uri, const Variant &value) |
void | setRating (const quint32 &value) |
void | setSymbols (const QStringList &value) |
void | setTags (const QList< Tag > &value) |
void | setTypes (const QList< QUrl > &types) |
void | setWatchEnabled (bool status) |
QStringList | symbols () const |
QList< Tag > | tags () const |
File | toFile () const |
QUrl | type () const |
QList< QUrl > | types () const |
QUrl | uri () const |
int | usageCount () const |
bool | watchEnabled () |
Static Public Member Functions | |
static Resource | fromResourceUri (const KUrl &uri, const Nepomuk2::Types::Class &type=Nepomuk2::Types::Class()) |
Detailed Description
Resource is the central object type in Nepomuk.
It represents a piece of information of any kind.
Resources are identified by their unique URI (which correlates directly with the URI in the local NEPOMUK RDF storage.
Resource objects with the same URI share their data.
All methods in Resource are thread-safe.
See Using Nepomuk for details on how to use Resource.
- See also
- ResourceManager
Special case: file URLs
file:/
URLs are handled as a special case in Nepomuk. Starting with KDE 4.4 they are no longer used to identify the Nepomuk resource but only stored as nie:url property. All resources have nepomuk:/res/
<UUID> URIs. The Resource constructors handle this automatically. Thus, one can still use file URLs to construct the objects. But be aware of the following example:
Here fileUrl
and fileResUri
are NOT equal. The latter is the resource URI of the form nepomuk:/res/
<UUID>.
Definition at line 93 of file resource.h.
Constructor & Destructor Documentation
Nepomuk2::Resource::Resource | ( | ) |
Creates an empty invalid Resource.
An invalid resource will become valid (i.e. get a new random URI) once setProperty is called.
Definition at line 52 of file resource.cpp.
Nepomuk2::Resource::Resource | ( | const Resource & | res | ) |
Copy constructor.
Definition at line 67 of file resource.cpp.
Nepomuk2::Resource::Resource | ( | const QString & | pathOrIdentifier, |
const QUrl & | type = QUrl() |
||
) |
Creates a new Resource object.
The actual resource data is loaded on demand. Thus, it is possible to work with Resources as if they were in memory all the time.
- Parameters
-
pathOrIdentifier The path to a file or an arbitrary identifier of the resource. The following cases are handled: - A local file path is converted to a local file URL
- A URI which already exist in Nepomuk results in loading of that particular resource.
- A string which already exists as the nao:identifier of a resource results in loading of that particular resource.
- Any other string is used as nao:identifier for a new resource. This resource can later be loaded again by using the same identifier with this constructor.
type The URI identifying the type of the resource. If it is empty Resource falls back to http://www.w3.org/2000/01/rdf-schema#Resource or in case the resource already exists the type will be read from the store.
Example:
The best way to understand the path or identifier system is through tags. There are two ways to create a resource that represents an existing tag. The first is the low level one: use the unique URI of the tag with the Resource(QUrl,QUrl) constructor. The second one is to use this constructor with the name of the tag as its identifier:
This will result in Resource loading the tag with nao:identifier "Nepomuk".
Definition at line 82 of file resource.cpp.
Nepomuk2::Resource::Resource | ( | const QUrl & | uri, |
const QUrl & | type = QUrl() |
||
) |
Creates a new Resource object.
- Parameters
-
uri The URI of the resource. If no resource with this URI exists, a new one is created. Using an empty QUrl will result in a new resource with a random URI being created on the first call to setProperty.
See the Special case: file URLs Special file URL handling.
- Parameters
-
type The URI identifying the type of the resource. If it is empty Resource falls back to http://www.w3.org/2000/01/rdf-schema#Resource or in case the resource already exists the type will be read from the store.
Definition at line 97 of file resource.cpp.
Nepomuk2::Resource::Resource | ( | Nepomuk2::ResourceData * | data | ) |
Constructor used internally.
Definition at line 113 of file resource.cpp.
|
virtual |
Destructor.
Definition at line 125 of file resource.cpp.
Member Function Documentation
void Nepomuk2::Resource::addIdentifier | ( | const QString & | value | ) |
Add a value to property 'identifier'.
Definition at line 528 of file resource.cpp.
void Nepomuk2::Resource::addIsRelated | ( | const Resource & | value | ) |
Add a value to property 'isRelated'.
Definition at line 589 of file resource.cpp.
void Nepomuk2::Resource::addProperty | ( | const QUrl & | uri, |
const Variant & | value | ||
) |
Add a property value to the existing values.
- Parameters
-
uri The URI identifying the property. value The value of the property (i.e. the object of the RDF triple(s))
- Since
- 4.3
Definition at line 276 of file resource.cpp.
void Nepomuk2::Resource::addSymbol | ( | const QString & | value | ) |
Add a value to property 'Symbol'.
Each resource can have a symbol assigned. For now this is a simple string which can either be the path to an actual pixmap file or just the name of an icon as defined by the freedesktop.org standard.
- Since
- 4.10
Definition at line 670 of file resource.cpp.
void Nepomuk2::Resource::addTag | ( | const Tag & | value | ) |
Add a value to property 'Tag'.
Each Resource can be tagged with an arbitrary number of Tags. This allows a simple grouping of resources.
Definition at line 561 of file resource.cpp.
void Nepomuk2::Resource::addType | ( | const QUrl & | type | ) |
QString Nepomuk2::Resource::description | ( | ) | const |
Get property 'description'.
Everything can be annotated with a simple string comment.
Definition at line 504 of file resource.cpp.
bool Nepomuk2::Resource::exists | ( | ) | const |
- Returns
- true if this resource (i.e. the uri of this resource) exists in the local NEPOMUK RDF store.
Definition at line 322 of file resource.cpp.
|
static |
Allows to quickly load a resource from its resource URI without any additional checks.
This is mostly used for optimized code within Nepomuk.
In most situations the construtor Resource( QUrl, QUrl ) is better suited.
- Since
- 4.5
Definition at line 743 of file resource.cpp.
QString Nepomuk2::Resource::genericDescription | ( | ) | const |
Tries very hard to find a suitable human-readable description of the resource.
This description is supposed to be longer than genericLabel() and includes such properties as nao:description, xesam:comment, rdfs:comment
- Returns
- A human readable description of the resource or an empty string if none could be found.
Definition at line 396 of file resource.cpp.
QString Nepomuk2::Resource::genericIcon | ( | ) | const |
Tries very hard to find an icon suitable for this resource.
- Returns
- An icon name to be used with KIcon or an empty string if none was found.
Definition at line 409 of file resource.cpp.
QString Nepomuk2::Resource::genericLabel | ( | ) | const |
Tries very hard to find a suitable human-readable label for this resource.
It looks for properties such as nao:prefLabel, rdfs:label, or nao:identifier, or even the fileName of File resources.
- Returns
- A human readable label or if all fails the URI of the resource.
Definition at line 341 of file resource.cpp.
bool Nepomuk2::Resource::hasProperty | ( | const QUrl & | uri | ) | const |
Check if property identified by uri is defined for this resource.
- Parameters
-
uri The URI identifying the property.
- Returns
- true if property uri has a value set.
Definition at line 240 of file resource.cpp.
bool Nepomuk2::Resource::hasProperty | ( | const Types::Property & | p, |
const Variant & | v | ||
) | const |
Check if the resource has a property p
with value v
.
- Since
- 4.5
Definition at line 252 of file resource.cpp.
bool Nepomuk2::Resource::hasType | ( | const QUrl & | typeUri | ) | const |
Check if the resource is of a certain type.
The type hierarchy is checked including subclass relations.
Definition at line 218 of file resource.cpp.
QStringList Nepomuk2::Resource::identifiers | ( | ) | const |
Get property 'identifier'.
Definition at line 516 of file resource.cpp.
void Nepomuk2::Resource::increaseUsageCount | ( | ) |
Increase the usage count of this resource and also update the last used date to the current date and time.
- Since
- 4.5
Definition at line 693 of file resource.cpp.
bool Nepomuk2::Resource::isFile | ( | ) | const |
- Returns
true
if this resource represents a file. Use toFile() to retrieve the corresponding file resource which provides convinience methods to handle file resources.
- Since
- 4.6
Definition at line 707 of file resource.cpp.
QList< Nepomuk2::Resource > Nepomuk2::Resource::isRelatedOf | ( | ) | const |
Get all resources that have this resource set as property 'isRelated'.
- See also
- ResourceManager::allResourcesWithProperty
Definition at line 676 of file resource.cpp.
QList< Nepomuk2::Resource > Nepomuk2::Resource::isRelateds | ( | ) | const |
Get property 'isRelated'.
Definition at line 572 of file resource.cpp.
bool Nepomuk2::Resource::isValid | ( | ) | const |
- Returns
- true if this Resource object is valid, i.e. has a proper URI and type and can be synced with the local NEPOMUK RDF store.
An invalid resource will become valid (i.e. get a new random URI) once setProperty is called.
Definition at line 334 of file resource.cpp.
QString Nepomuk2::Resource::label | ( | ) | const |
Get property 'label'.
Definition at line 600 of file resource.cpp.
bool Nepomuk2::Resource::operator!= | ( | const Resource & | other | ) | const |
Nepomuk2::Resource & Nepomuk2::Resource::operator= | ( | const Resource & | other | ) |
Makes this instance of Resource a copy of other.
Definition at line 141 of file resource.cpp.
Nepomuk2::Resource & Nepomuk2::Resource::operator= | ( | const QUrl & | uri | ) |
Same as operator=( Resource( uri ) )
Definition at line 160 of file resource.cpp.
bool Nepomuk2::Resource::operator== | ( | const Resource & | other | ) | const |
Operator to compare two Resource objects.
Definition at line 459 of file resource.cpp.
QHash< QUrl, Nepomuk2::Variant > Nepomuk2::Resource::properties | ( | ) | const |
- Returns
- A list of all defined properties
Definition at line 230 of file resource.cpp.
Nepomuk2::Variant Nepomuk2::Resource::property | ( | const QUrl & | uri | ) | const |
Retrieve the value of property uri.
If the property is not defined for this resource an invalid, empty Variant object is returned.
- Parameters
-
uri The URI identifying the property.
Definition at line 264 of file resource.cpp.
quint32 Nepomuk2::Resource::rating | ( | ) | const |
Get property 'Rating'.
Definition at line 612 of file resource.cpp.
void Nepomuk2::Resource::remove | ( | ) |
Remove this resource completely.
CAUTION: After calling this method the resource will have been removed from the store without any trace.
Definition at line 313 of file resource.cpp.
void Nepomuk2::Resource::removeProperty | ( | const QUrl & | uri | ) |
Remove property uri from this resource object.
- Parameters
-
uri The URI identifying the property.
Definition at line 294 of file resource.cpp.
void Nepomuk2::Resource::removeProperty | ( | const QUrl & | uri, |
const Variant & | value | ||
) |
Remove value from property uri of this resource object.
- Parameters
-
uri The URI identifying the property. value The value to remove
- Since
- 4.3
Definition at line 303 of file resource.cpp.
void Nepomuk2::Resource::setDescription | ( | const QString & | value | ) |
Set property 'description'.
Everything can be annotated with a simple string comment.
Definition at line 510 of file resource.cpp.
void Nepomuk2::Resource::setIdentifiers | ( | const QStringList & | value | ) |
Set property 'identifier'.
Definition at line 522 of file resource.cpp.
void Nepomuk2::Resource::setIsRelateds | ( | const QList< Resource > & | value | ) |
Set property 'isRelated'.
Definition at line 583 of file resource.cpp.
void Nepomuk2::Resource::setLabel | ( | const QString & | value | ) |
Set property 'label'.
Definition at line 606 of file resource.cpp.
void Nepomuk2::Resource::setProperty | ( | const QUrl & | uri, |
const Variant & | value | ||
) |
Set a property of the resource.
- Parameters
-
uri The URI identifying the property. value The value of the property (i.e. the object of the RDF triple(s))
Definition at line 285 of file resource.cpp.
void Nepomuk2::Resource::setRating | ( | const quint32 & | value | ) |
Set property 'Rating'.
Definition at line 618 of file resource.cpp.
void Nepomuk2::Resource::setSymbols | ( | const QStringList & | value | ) |
Set property 'Symbol'.
Each resource can have a symbol assigned. For now this is a simple string which can either be the path to an actual pixmap file or just the name of an icon as defined by the freedesktop.org standard.
- Since
- 4.10
Definition at line 659 of file resource.cpp.
void Nepomuk2::Resource::setTags | ( | const QList< Tag > & | value | ) |
Set property 'Tag'.
Each Resource can be tagged with an arbitrary number of Tags. This allows a simple grouping of resources.
Definition at line 545 of file resource.cpp.
void Nepomuk2::Resource::setTypes | ( | const QList< QUrl > & | types | ) |
Set the types of the resource.
Previous types will be overwritten.
- Since
- 4.2
Definition at line 200 of file resource.cpp.
void Nepomuk2::Resource::setWatchEnabled | ( | bool | status | ) |
Enables automatic updates of the internal cache using a ResourceWatcher.
Definition at line 725 of file resource.cpp.
QStringList Nepomuk2::Resource::symbols | ( | ) | const |
Get property 'Symbol'.
Each resource can have a symbol assigned. For now this is a simple string which can either be the path to an actual pixmap file or just the name of an icon as defined by the freedesktop.org standard.
- Since
- 4.10
Definition at line 623 of file resource.cpp.
QList< Nepomuk2::Tag > Nepomuk2::Resource::tags | ( | ) | const |
Get property 'Tag'.
Each Resource can be tagged with an arbitrary number of Tags. This allows a simple grouping of resources.
Definition at line 534 of file resource.cpp.
Nepomuk2::File Nepomuk2::Resource::toFile | ( | ) | const |
Convert this resource into a File resource to have access to the convinience methods provided by the File class.
- Since
- 4.6
Definition at line 720 of file resource.cpp.
QUrl Nepomuk2::Resource::type | ( | ) | const |
The main type of the resource.
Nepomuk tries hard to make this the type furthest down the hierarchy. In case the resource has only one type, this is no problem. However, if the resource has multiple types from different type hierarchies, there is no guarantee which one will be used here.
Definition at line 178 of file resource.cpp.
QList< QUrl > Nepomuk2::Resource::types | ( | ) | const |
- Returns
- The list of all stored types for this resource. This may also include types that lie in the same hierachy.
Definition at line 190 of file resource.cpp.
QUrl Nepomuk2::Resource::uri | ( | ) | const |
The URI of the resource, uniquely identifying it.
This URI in most cases is a virtual one which has been created from a generic base namespace and some identifier.
The most important thing to remember is that the URI of for example a file does not necessarily have a relation to its local path.
- Returns
- The resource URI of the resource or an empty url if the resource does not exist() yet.
- See also
- getIdentifiers()
Definition at line 166 of file resource.cpp.
int Nepomuk2::Resource::usageCount | ( | ) | const |
- Returns
- The usage count stored for this resource.
- See also
- increaseUsageCount()
- Since
- 4.5
Definition at line 687 of file resource.cpp.
bool Nepomuk2::Resource::watchEnabled | ( | ) |
- Returns
true
if this resource will automatically update its cache when the data is changed by some other application
Definition at line 732 of file resource.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:48:09 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.