Nepomuk-Core
#include <resourcewatcher.h>
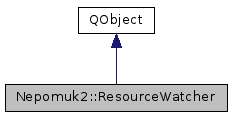
Public Slots | |
void | addProperty (const Types::Property &property) |
void | addResource (const Nepomuk2::Resource &res) |
void | addResource (const QUrl &resUri) |
void | addType (const Types::Class &type) |
QList< Types::Property > | properties () const |
int | propertyCount () const |
void | removeProperty (const Types::Property &property) |
void | removeResource (const Nepomuk2::Resource &res) |
void | removeResource (const QUrl &resUri) |
void | removeType (const Types::Class &type) |
int | resourceCount () const |
QList< Nepomuk2::Resource > | resources () const |
void | setProperties (const QList< Types::Property > &properties_) |
void | setResources (const QList< Nepomuk2::Resource > &resources_) |
void | setTypes (const QList< Types::Class > &types_) |
bool | start () |
void | stop () |
int | typeCount () const |
QList< Types::Class > | types () const |
Signals | |
void | propertyAdded (const Nepomuk2::Resource &resource, const Nepomuk2::Types::Property &property, const QVariant &value) |
void | propertyChanged (const Nepomuk2::Resource &resource, const Nepomuk2::Types::Property &property, const QVariantList &addedValues, const QVariantList &removedValues) |
void | propertyRemoved (const Nepomuk2::Resource &resource, const Nepomuk2::Types::Property &property, const QVariant &value) |
void | resourceCreated (const Nepomuk2::Resource &resource, const QList< QUrl > &types) |
void | resourceRemoved (const QUrl &uri, const QList< QUrl > &types) |
void | resourceTypeAdded (const Nepomuk2::Resource &res, const Nepomuk2::Types::Class &type) |
void | resourceTypeRemoved (const Nepomuk2::Resource &res, const Nepomuk2::Types::Class &type) |
Public Member Functions | |
ResourceWatcher (QObject *parent=0) | |
virtual | ~ResourceWatcher () |
Detailed Description
Selectively monitor the nepomuk repository for changes.
Resources may be monitored on the basis of types, properties, and uris.
Changes may be monitored in one of the following ways:
- By resources - Specify the exact resources that should be watched. Any changes made to the specified resources (Excluding Resource Metadata) will be notified through the propertyAdded() and propertyRemoved() signals. Notifications will also be sent if any of the watched resources is deleted.
- By resources and properties - Specify the exact resources and their properties. Any changes made to the specified resources which touch one of the specified properties will be notified through the propertyAdded() and propertyRemoved() signals.
- By types - Specific types may be specified via add/setType. If types are set, then notifications will be sent for all new resources of that type. This includes property changes and resource creation and removal. TODO: add flags that allow to only watch for resource creation and removal.
- By types and properties - Both the types and properties may be specified. Notifications will be sent for property changes in resource with the specified types.
Resource Watcher Usage Example
The following code creates a new ResourceWatcher, configures it to listen to changes on the nmm:performer
property on one specific resource res
.
Definition at line 81 of file resourcewatcher.h.
Constructor & Destructor Documentation
Nepomuk2::ResourceWatcher::ResourceWatcher | ( | QObject * | parent = 0 | ) |
Create a new ResourceWatcher instance.
This instance will not emit any signals before it has been configured and started.
Definition at line 72 of file resourcewatcher.cpp.
|
virtual |
Destructor.
Definition at line 83 of file resourcewatcher.cpp.
Member Function Documentation
|
slot |
Add a property to be watched.
Every change to a value of this property will be signalled, depending on the configured resources() or types().
- See also
- setProperties()
Definition at line 176 of file resourcewatcher.cpp.
|
slot |
Add a resource to be watched.
Every change to this resource will be signalled, depending on the configured properties().
- See also
- setResources()
Definition at line 184 of file resourcewatcher.cpp.
|
slot |
Add a resource to be watched.
Every change to this resource will be signalled, depending on the configured properties().
- See also
- setResources()
Definition at line 189 of file resourcewatcher.cpp.
|
slot |
Add a type to be watched.
Every resource of this type will be watched for changes.
- See also
- setTypes()
Definition at line 198 of file resourcewatcher.cpp.
|
slot |
The properties that have been configured via addProperty() and setProperties().
Every change to a value of any of these properties will be signalled, depending on the configured resources() or types().
Definition at line 235 of file resourcewatcher.cpp.
|
signal |
This signal is emitted when a property value is added.
- Parameters
-
resource The changed resource. property The property which has a new value. value The newly added property value.
|
signal |
This signal is emitted when a property value is changed.
This signal is essentially a combination of the propertyAdded and propertyRemoved signals.
Be aware that removing and then adding a property will result in two separate propertyChanged signals. They are never combined.
Specially, since one could theoretically take forever between the removal and the setting of the property.
- Parameters
-
resource The changed resource. property The property which was changed. addedValues The values that have been added. removedValues The values that have been removed.
|
slot |
Return the number of properties that are being watched.
This method is slightly faster than called properties().count()
- See also
- properties addProperty setProperty
Definition at line 259 of file resourcewatcher.cpp.
|
signal |
This signal is emitted when a property value is removed.
- Parameters
-
resource The changed resource. property The property which was changed. value The removed property value.
|
slot |
Remove a property to be watched.
Every change to a value of this property will be signalled, depending on the configured resources() or types().
- See also
- setProperties()
Definition at line 206 of file resourcewatcher.cpp.
|
slot |
Remove a resource to be watched.
Every change to this resource will be signalled, depending on the configured properties().
- See also
- setResources()
Definition at line 214 of file resourcewatcher.cpp.
|
slot |
Remove a resource to be watched.
Every change to this resource will be signalled, depending on the configured properties().
- See also
- setResources()
Definition at line 219 of file resourcewatcher.cpp.
|
slot |
Remove a type to be watched.
Every resource of this type will be watched for changes.
- See also
- setTypes()
Definition at line 227 of file resourcewatcher.cpp.
|
slot |
Return the number of resources that are being watched.
This method is slightly faster than called resources().count()
- See also
- resources addResource setResources
Definition at line 264 of file resourcewatcher.cpp.
|
signal |
This signal is emitted when a new resource is created.
- Parameters
-
resource The newly created resource. types The types the new resource has. If types() have been configured this list will always contain one of the configured types.
|
signal |
This signal is emitted when a resource is deleted.
- Parameters
-
uri The resource URI of the removed resource. types The types the removed resource had. If types() have been configured this list will always contain one of the configured types.
|
slot |
The resources that have been configured via addResource() and setResources().
Every change to one of these resources will be signalled, depending on the configured properties().
Definition at line 243 of file resourcewatcher.cpp.
|
signal |
This signal is emitted when a type has been added to a resource.
This does not include creation which is signalled via resourceCreated(). It only applies to changes in a resource's types.
- Parameters
-
res The changed resource. type The newly added type. If types() have been configured it will be one of them.
|
signal |
This signal is emitted when a type has been removed from a resource.
This does not include removal of entire resources which is signalled via resourceRemoved(). It only applies to changes in a resource's types.
- Parameters
-
res The changed resource. type The removed type. If types() have been configured it will be one of them.
|
slot |
Set the properties to be watched.
Every change to a value of any of these properties will be signalled, depending on the configured resources() or types().
- See also
- addProperty()
Definition at line 275 of file resourcewatcher.cpp.
|
slot |
Set the resources to be watched.
Every change to one of these resources will be signalled, depending on the configured properties().
- See also
- addResource()
Definition at line 287 of file resourcewatcher.cpp.
|
slot |
Set the types to be watched.
Every resource having one of these types will be watched for changes.
- See also
- addType()
Definition at line 299 of file resourcewatcher.cpp.
|
slot |
Start the signalling of changes.
Before calling this method no signal will be emitted. In combination with stop() this allows to suspend the watching. Calling start() multiple times has no effect.
Definition at line 89 of file resourcewatcher.cpp.
|
slot |
Stop the signalling of changes.
Allows to stop the watcher which has been started via start(). Calling stop() multiple times has no effect.
Definition at line 162 of file resourcewatcher.cpp.
|
slot |
Return the number of types that are being watched.
This method is slightly faster than called types().count()
Definition at line 269 of file resourcewatcher.cpp.
|
slot |
The types that have been configured via addType() and setTypes().
Every resource having one of these types will be watched for changes.
Definition at line 251 of file resourcewatcher.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:48:09 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.