Nepomuk
#include <Nepomuk/Resource>
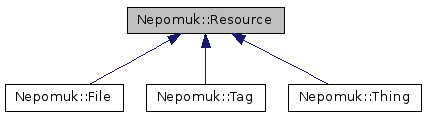
Public Member Functions | |
Resource () | |
Resource (ResourceManager *manager) | |
Resource (const Resource &) | |
Resource (const QString &pathOrIdentifier, const QUrl &type=QUrl()) | |
Resource (const QString &pathOrIdentifier, const QUrl &type, ResourceManager *manager) | |
Resource (const QString &pathOrIdentifier, const QString &type) | |
Resource (const QUrl &uri, const QUrl &type=QUrl()) | |
Resource (const QUrl &uri, const QUrl &type, ResourceManager *manager) | |
Resource (ResourceData *) | |
virtual | ~Resource () |
void | addAltLabel (const QString &value) |
void | addAnnotation (const Resource &value) |
void | addIdentifier (const QString &value) |
void | addIsRelated (const Resource &value) |
void | addIsTopicOf (const Resource &value) |
void | addProperty (const QUrl &uri, const Variant &value) |
void | addSymbol (const QString &value) |
void | addTag (const Tag &value) |
void | addTopic (const Resource &value) |
void | addType (const QUrl &type) |
QHash< QString, Variant > | allProperties () const |
QStringList | altLabels () const |
QList< Resource > | annotationOf () const |
QList< Resource > | annotations () const |
QString | className () const |
QString | description () const |
bool | exists () const |
QString | genericDescription () const |
QString | genericIcon () const |
QString | genericLabel () const |
bool | hasProperty (const QUrl &uri) const |
bool | hasProperty (const Types::Property &p, const Variant &v) const |
bool | hasProperty (const QString &uri) const |
bool | hasType (const QUrl &typeUri) const |
QStringList | identifiers () const |
void | increaseUsageCount () |
bool | isFile () const |
QList< Resource > | isRelatedOf () const |
QList< Resource > | isRelateds () const |
QList< Resource > | isTopicOfs () const |
bool | isValid () const |
QString | label () const |
ResourceManager * | manager () const |
bool | operator!= (const Resource &) const |
Resource & | operator= (const Resource &other) |
Resource & | operator= (const QUrl &uri) |
bool | operator== (const Resource &) const |
Thing | pimoThing () |
QHash< QUrl, Variant > | properties () const |
Variant | property (const QUrl &uri) const |
Variant | property (const QString &uri) const |
quint32 | rating () const |
void | remove () |
void | removeProperty (const QUrl &uri) |
void | removeProperty (const QUrl &uri, const Variant &value) |
void | removeProperty (const QString &uri) |
QUrl | resourceType () const |
QUrl | resourceUri () const |
void | setAltLabels (const QStringList &value) |
void | setAnnotations (const QList< Resource > &value) |
void | setDescription (const QString &value) |
void | setIdentifiers (const QStringList &value) |
void | setIsRelateds (const QList< Resource > &value) |
void | setIsTopicOfs (const QList< Resource > &value) |
void | setLabel (const QString &value) |
void | setProperty (const QUrl &uri, const Variant &value) |
void | setProperty (const QString &uri, const Variant &value) |
void | setRating (const quint32 &value) |
void | setSymbols (const QStringList &value) |
void | setTags (const QList< Tag > &value) |
void | setTopics (const QList< Resource > &value) |
void | setTypes (const QList< QUrl > &types) |
QStringList | symbols () const |
QList< Tag > | tags () const |
File | toFile () const |
QList< Resource > | topics () const |
QString | type () const |
QList< QUrl > | types () const |
QString | uri () const |
int | usageCount () const |
Static Public Member Functions | |
static QList< Resource > | allResources () |
static QString | altLabelUri () |
static QString | annotationUri () |
static QString | descriptionUri () |
static Resource | fromResourceUri (const KUrl &uri, const Nepomuk::Types::Class &type=Nepomuk::Types::Class(), ResourceManager *manager=0) |
static QString | identifierUri () |
static QString | isRelatedUri () |
static QString | isTopicOfUri () |
static QString | labelUri () |
static QString | ratingUri () |
static QString | symbolUri () |
static QString | tagUri () |
static QString | topicUri () |
Detailed Description
Resource is the central object type in Nepomuk.
It represents a piece of information of any kind.
Resources are identified by their unique URI (which correlates directly with the URI in the local NEPOMUK RDF storage.
Resource objects with the same URI share their data.
All methods in Resource are thread-safe.
See Using Nepomuk for details on how to use Resource.
- See also
- ResourceManager
Special case: file URLs
file:/
URLs are handled as a special case in Nepomuk. Starting with KDE 4.4 they are no longer used to identify the Nepomuk resource but only stored as nie:url property. All resources have nepomuk:/res/
<UUID> URIs. The Resource constructors handle this automatically. Thus, one can still use file URLs to construct the objects. But be aware of the following example:
Here fileUrl
and fileResUri
are NOT equal. The latter is the resource URI of the form nepomuk:/res/
<UUID>.
Definition at line 94 of file resource.h.
Constructor & Destructor Documentation
Nepomuk::Resource::Resource | ( | ) |
Creates an empty invalid Resource.
An invalid resource will become valid (i.e. get a new random URI) once setProperty is called.
Nepomuk::Resource::Resource | ( | ResourceManager * | manager | ) |
Creates an empty invalid Resource.
An invalid resource will become valid (i.e. get a new random URI) once setProperty is called.
- Parameters
-
manager The resource manager to use. This allows to mix resources from different managers and, thus, different models.
- Since
- 4.3
Nepomuk::Resource::Resource | ( | const Resource & | ) |
Copy constructor.
Nepomuk::Resource::Resource | ( | const QString & | pathOrIdentifier, |
const QUrl & | type = QUrl() |
||
) |
Creates a new Resource object.
The actual resource data is loaded on demand. Thus, it is possible to work with Resources as if they were in memory all the time.
- Parameters
-
pathOrIdentifier The path to a file or an arbitrary identifier of the resource. The following cases are handled: - A local file path is converted to a local file URL
- A URI which already exist in Nepomuk results in loading of that particular resource.
- A string which already exists as the nao:identifier of a resource results in loading of that particular resource.
- Any other string is used as nao:identifier for a new resource. This resource can later be loaded again by using the same identifier with this constructor.
type The URI identifying the type of the resource. If it is empty Resource falls back to http://www.w3.org/2000/01/rdf-schema#Resource or in case the resource already exists the type will be read from the store.
Example:
The best way to understand the path or identifier system is through tags. There are two ways to create a resource that represents an existing tag. The first is the low level one: use the unique URI of the tag with the Resource(QUrl,QUrl) constructor. The second one is to use this constructor with the name of the tag as its identifier:
This will result in Resource loading the tag with nao:identifier "Nepomuk".
Nepomuk::Resource::Resource | ( | const QString & | pathOrIdentifier, |
const QUrl & | type, | ||
ResourceManager * | manager | ||
) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
- Parameters
-
manager The resource manager to use. This allows to mix resources from different managers and, thus, different models.
- Since
- 4.3
Nepomuk::Resource::Resource | ( | const QString & | pathOrIdentifier, |
const QString & | type | ||
) |
Nepomuk::Resource::Resource | ( | const QUrl & | uri, |
const QUrl & | type = QUrl() |
||
) |
Creates a new Resource object.
- Parameters
-
uri The URI of the resource. If no resource with this URI exists, a new one is created. Using an empty QUrl will result in a new resource with a random URI being created on the first call to setProperty.
See the Special case: file URLs Special file URL handling.
- Parameters
-
type The URI identifying the type of the resource. If it is empty Resource falls back to http://www.w3.org/2000/01/rdf-schema#Resource or in case the resource already exists the type will be read from the store.
Nepomuk::Resource::Resource | ( | const QUrl & | uri, |
const QUrl & | type, | ||
ResourceManager * | manager | ||
) |
This is an overloaded member function, provided for convenience. It differs from the above function only in what argument(s) it accepts.
- Parameters
-
manager The resource manager to use. This allows to mix resources from different managers and, thus, different models.
- Since
- 4.3
Nepomuk::Resource::Resource | ( | ResourceData * | ) |
Constructor used internally.
|
virtual |
Destructor.
Member Function Documentation
void Nepomuk::Resource::addAltLabel | ( | const QString & | value | ) |
Add a value to property 'altLabel'.
void Nepomuk::Resource::addAnnotation | ( | const Resource & | value | ) |
Add a value to property 'annotation'.
void Nepomuk::Resource::addIdentifier | ( | const QString & | value | ) |
Add a value to property 'identifier'.
void Nepomuk::Resource::addIsRelated | ( | const Resource & | value | ) |
Add a value to property 'isRelated'.
void Nepomuk::Resource::addIsTopicOf | ( | const Resource & | value | ) |
Add a value to property 'isTopicOf'.
void Nepomuk::Resource::addProperty | ( | const QUrl & | uri, |
const Variant & | value | ||
) |
Add a property value to the existing values.
- Parameters
-
uri The URI identifying the property. value The value of the property (i.e. the object of the RDF triple(s))
- Since
- 4.3
void Nepomuk::Resource::addSymbol | ( | const QString & | value | ) |
Add a value to property 'Symbol'.
Each resource can have a symbol assigned. For now this is a simple string which can either be the path to an actual pixmap file or just the name of an icon as defined by the freedesktop.org standard.
void Nepomuk::Resource::addTag | ( | const Tag & | value | ) |
void Nepomuk::Resource::addTopic | ( | const Resource & | value | ) |
Add a value to property 'Topic'.
void Nepomuk::Resource::addType | ( | const QUrl & | type | ) |
Add a type to the list of types.
- Since
- 4.2
QHash<QString, Variant> Nepomuk::Resource::allProperties | ( | ) | const |
|
static |
Retrieve a list of all available Resource resources.
This list consists of all resource of type Resource that are stored in the local Nepomuk meta data storage and any changes made locally. Be aware that in some cases this list can get very big. Then it might be better to use libKNep directly.
- See also
- ResourceManager::allResources
- Warning
- This list will be very big. Usage of this method is discouraged. Use Query::QueryServiceClient in combination with an empty Query::Query instead.
QStringList Nepomuk::Resource::altLabels | ( | ) | const |
Get property 'altLabel'.
|
static |
- Returns
- The URI of the property 'altLabel'.
QList<Resource> Nepomuk::Resource::annotationOf | ( | ) | const |
Get all resources that have this resource set as property 'annotation'.
QList<Resource> Nepomuk::Resource::annotations | ( | ) | const |
Get property 'annotation'.
|
static |
- Returns
- The URI of the property 'annotation'.
QString Nepomuk::Resource::className | ( | ) | const |
The name of the class this Resource represents an object of.
The classname is derived from the type URI (see Resource::uri). For a translated user readable name of the resource see Ontology::typeName.
- See also
- type()
QString Nepomuk::Resource::description | ( | ) | const |
Get property 'description'.
Everything can be annotated with a simple string comment.
|
static |
- Returns
- The URI of the property 'description'.
bool Nepomuk::Resource::exists | ( | ) | const |
- Returns
- true if this resource (i.e. the uri of this resource) exists in the local NEPOMUK RDF store.
|
static |
Allows to quickly load a resource from its resource URI without any additional checks.
This is mostly used for optimized code within Nepomuk.
In most situations the construtor Resource( QUrl, QUrl ) is better suited.
- Since
- 4.5
QString Nepomuk::Resource::genericDescription | ( | ) | const |
Tries very hard to find a suitable human-readable description of the resource.
This description is supposed to be longer than genericLabel() and includes such properties as nao:description, xesam:comment, rdfs:comment
- Returns
- A human readable description of the resource or an empty string if none could be found.
QString Nepomuk::Resource::genericIcon | ( | ) | const |
Tries very hard to find an icon suitable for this resource.
- Returns
- An icon name to be used with KIcon or an empty string if none was found.
QString Nepomuk::Resource::genericLabel | ( | ) | const |
Tries very hard to find a suitable human-readable label for this resource.
It looks for properties such as nao:prefLabel, rdfs:label, or nao:identifier, or even the fileName of File resources.
- Returns
- A human readable label or if all fails the URI of the resource.
bool Nepomuk::Resource::hasProperty | ( | const QUrl & | uri | ) | const |
Check if property identified by uri is defined for this resource.
- Parameters
-
uri The URI identifying the property.
- Returns
- true if property uri has a value set.
bool Nepomuk::Resource::hasProperty | ( | const Types::Property & | p, |
const Variant & | v | ||
) | const |
Check if the resource has a property p
with value v
.
- Since
- 4.5
bool Nepomuk::Resource::hasProperty | ( | const QString & | uri | ) | const |
bool Nepomuk::Resource::hasType | ( | const QUrl & | typeUri | ) | const |
Check if the resource is of a certain type.
The type hierarchy is checked including subclass relations.
QStringList Nepomuk::Resource::identifiers | ( | ) | const |
Get property 'identifier'.
|
static |
- Returns
- The URI of the property 'identifier'.
void Nepomuk::Resource::increaseUsageCount | ( | ) |
Increase the usage count of this resource and also update the last used date to the current date and time.
- Since
- 4.5
bool Nepomuk::Resource::isFile | ( | ) | const |
- Returns
true
if this resource represents a file. Use toFile() to retrieve the corresponding file resource which provides convinience methods to handle file resources.
- Since
- 4.6
QList<Resource> Nepomuk::Resource::isRelatedOf | ( | ) | const |
Get all resources that have this resource set as property 'isRelated'.
QList<Resource> Nepomuk::Resource::isRelateds | ( | ) | const |
Get property 'isRelated'.
|
static |
- Returns
- The URI of the property 'isRelated'.
QList<Resource> Nepomuk::Resource::isTopicOfs | ( | ) | const |
Get property 'isTopicOf'.
|
static |
- Returns
- The URI of the property 'isTopicOf'.
bool Nepomuk::Resource::isValid | ( | ) | const |
- Returns
- true if this Resource object is valid, i.e. has a proper URI and type and can be synced with the local NEPOMUK RDF store.
An invalid resource will become valid (i.e. get a new random URI) once setProperty is called.
QString Nepomuk::Resource::label | ( | ) | const |
Get property 'label'.
|
static |
- Returns
- The URI of the property 'label'.
ResourceManager* Nepomuk::Resource::manager | ( | ) | const |
The Resource manager that manages this resource.
- Since
- 4.3
bool Nepomuk::Resource::operator!= | ( | const Resource & | ) | const |
Operator to compare two Resource objects.
- Since
- 4.4
Makes this instance of Resource a copy of other.
Resource& Nepomuk::Resource::operator= | ( | const QUrl & | uri | ) |
Same as operator=( Resource( uri ) )
bool Nepomuk::Resource::operator== | ( | const Resource & | ) | const |
Operator to compare two Resource objects.
Thing Nepomuk::Resource::pimoThing | ( | ) |
QHash<QUrl, Variant> Nepomuk::Resource::properties | ( | ) | const |
- Returns
- A list of all defined properties
Variant Nepomuk::Resource::property | ( | const QUrl & | uri | ) | const |
Retrieve the value of property uri.
If the property is not defined for this resource an invalid, empty Variant object is returned.
- Parameters
-
uri The URI identifying the property.
Variant Nepomuk::Resource::property | ( | const QString & | uri | ) | const |
quint32 Nepomuk::Resource::rating | ( | ) | const |
Get property 'Rating'.
|
static |
- Returns
- The URI of the property 'Rating'.
void Nepomuk::Resource::remove | ( | ) |
Remove this resource completely.
CAUTION: After calling this method the resource will have been removed from the store without any trace.
void Nepomuk::Resource::removeProperty | ( | const QUrl & | uri | ) |
Remove property uri from this resource object.
- Parameters
-
uri The URI identifying the property.
void Nepomuk::Resource::removeProperty | ( | const QUrl & | uri, |
const Variant & | value | ||
) |
Remove value from property uri of this resource object.
- Parameters
-
uri The URI identifying the property. value The value to remove
- Since
- 4.3
void Nepomuk::Resource::removeProperty | ( | const QString & | uri | ) |
QUrl Nepomuk::Resource::resourceType | ( | ) | const |
The main type of the resource.
Nepomuk tries hard to make this the type furthest down the hierarchy. In case the resource has only one type, this is no problem. However, if the resource has multiple types from different type hierarchies, there is no guarantee which one will be used here.
QUrl Nepomuk::Resource::resourceUri | ( | ) | const |
The URI of the resource, uniquely identifying it.
This URI in most cases is a virtual one which has been created from a generic base namespace and some identifier.
The most important thing to remember is that the URI of for example a file does not necessarily have a relation to its local path.
- Returns
- The resource URI of the resource or an empty url if the resource does not exist() yet.
- See also
- getIdentifiers()
void Nepomuk::Resource::setAltLabels | ( | const QStringList & | value | ) |
Set property 'altLabel'.
void Nepomuk::Resource::setAnnotations | ( | const QList< Resource > & | value | ) |
Set property 'annotation'.
void Nepomuk::Resource::setDescription | ( | const QString & | value | ) |
Set property 'description'.
Everything can be annotated with a simple string comment.
void Nepomuk::Resource::setIdentifiers | ( | const QStringList & | value | ) |
Set property 'identifier'.
void Nepomuk::Resource::setIsRelateds | ( | const QList< Resource > & | value | ) |
Set property 'isRelated'.
void Nepomuk::Resource::setIsTopicOfs | ( | const QList< Resource > & | value | ) |
Set property 'isTopicOf'.
void Nepomuk::Resource::setLabel | ( | const QString & | value | ) |
Set property 'label'.
void Nepomuk::Resource::setProperty | ( | const QUrl & | uri, |
const Variant & | value | ||
) |
Set a property of the resource.
- Parameters
-
uri The URI identifying the property. value The value of the property (i.e. the object of the RDF triple(s))
void Nepomuk::Resource::setProperty | ( | const QString & | uri, |
const Variant & | value | ||
) |
- Deprecated:
- use setProperty( const QUrl& )
void Nepomuk::Resource::setRating | ( | const quint32 & | value | ) |
Set property 'Rating'.
void Nepomuk::Resource::setSymbols | ( | const QStringList & | value | ) |
Set property 'Symbol'.
Each resource can have a symbol assigned. For now this is a simple string which can either be the path to an actual pixmap file or just the name of an icon as defined by the freedesktop.org standard.
void Nepomuk::Resource::setTags | ( | const QList< Tag > & | value | ) |
void Nepomuk::Resource::setTopics | ( | const QList< Resource > & | value | ) |
Set property 'Topic'.
void Nepomuk::Resource::setTypes | ( | const QList< QUrl > & | types | ) |
Set the types of the resource.
Previous types will be overwritten.
- Since
- 4.2
QStringList Nepomuk::Resource::symbols | ( | ) | const |
Get property 'Symbol'.
Each resource can have a symbol assigned. For now this is a simple string which can either be the path to an actual pixmap file or just the name of an icon as defined by the freedesktop.org standard.
|
static |
- Returns
- The URI of the property 'Symbol'.
QList<Tag> Nepomuk::Resource::tags | ( | ) | const |
|
static |
- Returns
- The URI of the property 'Tag'.
File Nepomuk::Resource::toFile | ( | ) | const |
QList<Resource> Nepomuk::Resource::topics | ( | ) | const |
Get property 'Topic'.
|
static |
- Returns
- The URI of the property 'Topic'.
QString Nepomuk::Resource::type | ( | ) | const |
The main type of the resource.
Nepomuk tries hard to make this the type furthest down the hierarchy. In case the resource has only one type, this is no problem. However, if the resource has multiple types from different type hierarchies, there is no guarantee which one will be used here.
For historical reasons the method does return a URI as QString instead of QUrl. The value equals resourceType().toString().
- Deprecated:
- use resourceType instead
QList<QUrl> Nepomuk::Resource::types | ( | ) | const |
QString Nepomuk::Resource::uri | ( | ) | const |
The URI of the resource, uniquely identifying it.
This URI in most cases is a virtual one which has been created from a generic base namespace and some identifier.
The most important thing to remember is that the URI of for example a file does not necessarily have a relation to its local path. (Although Nepomuk tries to keep the URI of file resources in sync with the file URL for convenience.)
For historical reasons the method does return a URI as QString instead of QUrl. The value equals resourceUri().toString().
- See also
- resourceUri(), getIdentifiers()
- Deprecated:
- use resourceUri() instead
int Nepomuk::Resource::usageCount | ( | ) | const |
The documentation for this class was generated from the following file:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:48:27 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.