Nepomuk
#include <Nepomuk/Utils/DateFacet>
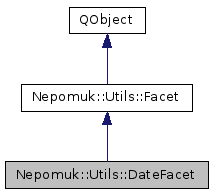
Public Types | |
enum | DateRangeCandidate { NoDateRange = 0x0, Anytime = 0x1, Today = 0x2, Yesterday = 0x4, ThisWeek = 0x8, LastWeek = 0x10, ThisMonth = 0x20, LastMonth = 0x40, ThisYear = 0x80, LastYear = 0x100, CustomDateRange = 0x10000, DefaultRanges = Anytime|Today|ThisWeek|ThisMonth|ThisYear|CustomDateRange } |
![]() | |
enum | SelectionMode { MatchAll, MatchAny, MatchOne } |
Public Slots | |
void | clearSelection () |
bool | selectFromTerm (const Nepomuk::Query::Term &queryTerm) |
void | setSelected (int index, bool selected=true) |
![]() | |
virtual void | clearSelection ()=0 |
virtual bool | selectFromTerm (const Nepomuk::Query::Term &queryTerm)=0 |
void | setClientQuery (const Nepomuk::Query::Query &query) |
virtual void | setSelected (int index, bool selected=true)=0 |
Public Member Functions | |
DateFacet (QObject *parent=0) | |
virtual | ~DateFacet () |
int | count () const |
DateRangeCandidates | dateRangeCandidates () const |
KGuiItem | guiItem (int index) const |
bool | isSelected (int index) const |
Query::Term | queryTerm () const |
SelectionMode | selectionMode () const |
void | setDateRangeCandidates (DateRangeCandidates ranges) |
![]() | |
Facet (QObject *parent=0) | |
virtual | ~Facet () |
Query::Query | clientQuery () const |
virtual QString | text (int index) const |
Protected Member Functions | |
virtual Query::Term | createDateRangeTerm (const DateRange &range) const |
virtual DateRange | extractDateRange (const Query::Term &queryTerm) const |
virtual DateRange | getCustomRange (bool *ok=0) const |
![]() | |
virtual void | handleClientQueryChange () |
Additional Inherited Members | |
![]() | |
void | layoutChanged (Nepomuk::Utils::Facet *facet) |
void | queryTermChanged (Nepomuk::Utils::Facet *facet, const Nepomuk::Query::Term &queryTerm) |
void | selectionChanged (Nepomuk::Utils::Facet *facet) |
![]() | |
static Facet * | createDateFacet (QObject *parent=0) |
static Facet * | createFileTypeFacet (QObject *parent=0) |
static Facet * | createPriorityFacet (QObject *parent=0) |
static Facet * | createRatingFacet (QObject *parent=0) |
static Facet * | createTagFacet (QObject *parent=0) |
static Facet * | createTypeFacet (QObject *parent=0) |
![]() | |
void | setLayoutChanged () |
void | setQueryTermChanged () |
void | setSelectionChanged () |
Detailed Description
A Facet that provides a set of date ranges.
Filtering search results on a date is a very typical problem. This could be handled by adding fixed Terms to a SimpleFacet. The big problem with that solution, however, is that these fixed terms would become inaccurate over time. Say, one wants to filter files that have been modified today. This is a very simple filter involving the nie:lastModified property and QDate::currentDate(). But as soon as the application runs while the current date is changing this fixed term becomes invalid since it is now referencing yesterday instead of today.
The DateFacet works around this problem by using the enumeration DateRangeCandidate instead of fixed terms and creating the terms on the fly based on the enum value.
By default it creates date terms via Nepomuk::Utils::dateRangeQuery() which includes the file modification time but also access times and content creation times (the latter includes the date a photo was taken). This can, however, be modified by reimplementing createDateRangeTerm() and its counterpart extractDateRange().
- Since
- 4.6
Definition at line 58 of file datefacet.h.
Member Enumeration Documentation
Using fixed QDate values would result in invalid queries as soon as an application runs over the span of two days.
Thus, DateFacet uses DateRangeCandidates to specify the list of choices and generates the QDate instances on the fly.
Definition at line 103 of file datefacet.h.
Constructor & Destructor Documentation
Nepomuk::Utils::DateFacet::DateFacet | ( | QObject * | parent = 0 | ) |
Creates a new date facet which contains the DefaultRanges date range candidates as choices.
|
virtual |
Destructor.
Member Function Documentation
|
slot |
Resets the current range to the default value which is the first in the list of candidates.
If Anytime is part of the configured candidates it will be selected.
|
virtual |
- Returns
- The number of date range candidates set via setDateRangeCandidates().
Implements Nepomuk::Utils::Facet.
|
protectedvirtual |
Create the query Term that reflects the dates specified in range
.
The default implementation uses Nepomuk::Utils::dateRangeQuery().
Subclasses may reimplement this method to create custom date ranges.
If this method is reimplemented extractDateRange() also needs to be implemented in order for selectFromTerm() to work properly.
DateRangeCandidates Nepomuk::Utils::DateFacet::dateRangeCandidates | ( | ) | const |
- Returns
- The date ranges configured via setDateRangeCandidates()
- See also
- DateRangeCandidate
|
protectedvirtual |
This is the counterpart method to createDateRangeTerm().
It should only extract valid date ranges from terms that have been created by createDateRangeTerm() or have the same structure.
A working implementation is required in order for selectFromTerm() to work properly.
The default implementation can handle query terms created via Nepomuk::Utils::dateRangeQuery().
|
protectedvirtual |
Retrieve a custom date range typically from the user.
The default implementation opens a popup at the current cursor position allowing the user to choose the range via appropriate GUI elements.
This method is called internally by DateFacet to handle the CustomRange date range candidate.
Reimplementing this method allows to provide a customized way to get a range for the CustomRange date range candidate.
- Parameters
-
ok The method should set this boolean according to the user feedback. This is necessary since returning an invalid DateRange is ambiguous.
- Returns
- The date range chosen by the user.
|
virtual |
The parameters used to render the choice at index
.
Reimplemented from Nepomuk::Utils::Facet.
|
virtual |
- Returns
true
if the choice atindex
is selected,false
otherwise.
Implements Nepomuk::Utils::Facet.
|
virtual |
Creates a query term for the currently selected date range.
This method makes use of createDateRangeTerm().
Implements Nepomuk::Utils::Facet.
|
slot |
Select the current date range based on term
.
This method relies on extractDateRange() to determine if term
can be used or not.
- Returns
true
ifterm
could be handled and the current range has been changed accordingly,false
otherwise.
|
virtual |
The selection mode in the date facet is fixed to MatchOne, ie.
it is an exclusive facet where a single date range must be selected at all time.
Implements Nepomuk::Utils::Facet.
void Nepomuk::Utils::DateFacet::setDateRangeCandidates | ( | DateRangeCandidates | ranges | ) |
Set the date ranges this facet should provide.
By default all date ranges from DefaultRanges are enabled.
|
slot |
Change the currently selected date range.
This method is typically triggered by user interaction.
The documentation for this class was generated from the following file:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:48:27 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.