Nepomuk
#include <Nepomuk/Utils/Facet>
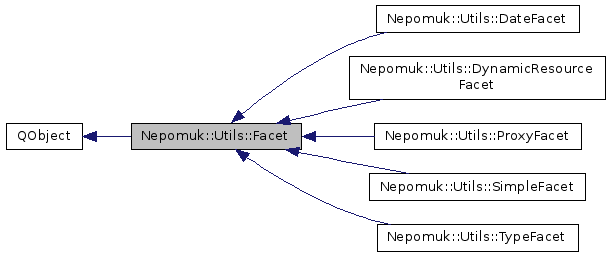
Public Types | |
enum | SelectionMode { MatchAll, MatchAny, MatchOne } |
Public Slots | |
virtual void | clearSelection ()=0 |
virtual bool | selectFromTerm (const Nepomuk::Query::Term &queryTerm)=0 |
void | setClientQuery (const Nepomuk::Query::Query &query) |
virtual void | setSelected (int index, bool selected=true)=0 |
Signals | |
void | layoutChanged (Nepomuk::Utils::Facet *facet) |
void | queryTermChanged (Nepomuk::Utils::Facet *facet, const Nepomuk::Query::Term &queryTerm) |
void | selectionChanged (Nepomuk::Utils::Facet *facet) |
Public Member Functions | |
Facet (QObject *parent=0) | |
virtual | ~Facet () |
Query::Query | clientQuery () const |
virtual int | count () const =0 |
virtual KGuiItem | guiItem (int index) const |
virtual bool | isSelected (int index) const =0 |
virtual Query::Term | queryTerm () const =0 |
virtual SelectionMode | selectionMode () const =0 |
virtual QString | text (int index) const |
Static Public Member Functions | |
static Facet * | createDateFacet (QObject *parent=0) |
static Facet * | createFileTypeFacet (QObject *parent=0) |
static Facet * | createPriorityFacet (QObject *parent=0) |
static Facet * | createRatingFacet (QObject *parent=0) |
static Facet * | createTagFacet (QObject *parent=0) |
static Facet * | createTypeFacet (QObject *parent=0) |
Protected Slots | |
void | setLayoutChanged () |
void | setQueryTermChanged () |
void | setSelectionChanged () |
Protected Member Functions | |
virtual void | handleClientQueryChange () |
Detailed Description
The base class for all facets used to create query filters.
A Facet represents one aspect of a query like the resource type or the modification date. By providing a list of facets to the user they can interactively construct a query by restricting the result set step by step.
A Facet can be seen as a list of choices presented to the user. Depending on the selection (which is typically triggered by user interaction) the Facet produces a resulting term() which is then used in the final query.
Each facet needs to implement a set of methods describing the choices: count(), guiItem(), isSelected(), setSelected(), and clearSelection(). In addition the term() method constructs the term this facet represents with its current selection.
Methods selectFromTerm() and setClientQuery() are optional but allow for a more advanced usage and a better user experience. See the method documentation for details.
Before implementing your own Facet subclass make sure that SimpleFacet or DynamicResourceFacet do not suit your needs.
Facet also provides a set of static factory methods like createTagFacet() or createFileTypeFacet() to create standard facets that are useful in most query UI situations.
- Since
- 4.6
Member Enumeration Documentation
A Facet can have one of three selection modes which is provided by selectionMode().
Enumerator | |
---|---|
MatchAll |
All of the choices need to be matched. This typically means that all choices' terms are combined using an AndTerm. |
MatchAny |
At least one of the choices needs to be matched. This typically means that all choices' terms are combined using an OrTerm. |
MatchOne |
Exactly one of the choices needs to match. This means that the Facet is exclusive, ie. only one choice can be selected at all times. |
Constructor & Destructor Documentation
Nepomuk::Utils::Facet::Facet | ( | QObject * | parent = 0 | ) |
Constructor.
|
virtual |
Destructor.
Member Function Documentation
|
pure virtualslot |
Clear the selection.
If selectionMode() is MatchOne the first choice should be selected.
Query::Query Nepomuk::Utils::Facet::clientQuery | ( | ) | const |
The client query set via setClientQuery().
See the documentation of setClientQuery() for details.
|
pure virtual |
The number of choices this facet provides.
Implemented in Nepomuk::Utils::DynamicResourceFacet, Nepomuk::Utils::DateFacet, Nepomuk::Utils::ProxyFacet, Nepomuk::Utils::SimpleFacet, and Nepomuk::Utils::TypeFacet.
Creates a new Facet that allows to filter on the date.
This includes the modification, creation, and access dates.
- See also
- Query::dateRangeQuery()
Creates a new Facet that allows to filter on the file type.
Normally this facet would be used in combination with a FileQuery.
- See also
- createTypeFacet()
Creates a new Facet that allows to sort the results by one of several criteria like last modification date or a score calculated by the Nepomuk system.
Using this facet does only make sense when displaying sorted results or when using a limit on the number of results.
Creates a new Facet that allows to filter on the rating of resources.
Creates a new Facet that allows to filter on tags, i.e.
search for resources that are tagged with the selected tags. The list of tags is dynamic. This means it has a default selection of the most often used ones and provides a means to access all tags through a dialog.
Creates a new Facet that allows to filter on the resource type without a restriction to files.
- See also
- createFileTypeFacet()
|
virtual |
The parameters used to render the choice at index
.
Reimplemented in Nepomuk::Utils::DynamicResourceFacet, Nepomuk::Utils::DateFacet, Nepomuk::Utils::ProxyFacet, Nepomuk::Utils::SimpleFacet, and Nepomuk::Utils::TypeFacet.
|
protectedvirtual |
This method is called from setClientQuery() and can be reimplemented by subclasses.
The default implementation does nothing.
Reimplemented in Nepomuk::Utils::DynamicResourceFacet, and Nepomuk::Utils::ProxyFacet.
|
pure virtual |
- Returns
true
if the choice atindex
is selected,false
otherwise.
Implemented in Nepomuk::Utils::DynamicResourceFacet, Nepomuk::Utils::SimpleFacet, Nepomuk::Utils::ProxyFacet, Nepomuk::Utils::DateFacet, and Nepomuk::Utils::TypeFacet.
|
signal |
Emitted when the layout of the facet changed, ie.
one of count(), guitItem(), or text() return different values.
Subclasses should call setLayoutChanged() instead of emitting this signal manually.
|
pure virtual |
The term currently produced by this facet.
This is dependant on the subclass implementation and the selectionMode(). The SimpleFacet for example uses an AndTerm or an OrTerm to combine all its terms in MatchAll or MatchAny mode while it returns the one selected term in MatchOne mode.
Implemented in Nepomuk::Utils::DynamicResourceFacet, Nepomuk::Utils::ProxyFacet, Nepomuk::Utils::DateFacet, Nepomuk::Utils::SimpleFacet, and Nepomuk::Utils::TypeFacet.
|
signal |
Emitted when the term of the facet changed, ie.
when the value returned by term() has changed.
Subclasses should call setTermChanged() instead of emitting this signal manually.
Be aware that in most situations queryTermChanged() and selectionChanged() will have to be emitted at the same time.
|
pure virtualslot |
If a client application provides several ways to construct a query (one could think of a query editor which allows to insert certain constraints or simply another application providing a base query) the user expects to be able to modify that query using the available facets.
Thus, a Facet should be able to extract parts from a query.
Implement this method to create a selection based on the contents of term
. The selection of the facet must only change if all of term
can be used. This means that if term
is for example an OrTerm and the Facet can only handle one of the sub terms it needs to ignore the whole term. FacetModel::setQuery() internally calls selectFromTerm() on all its facets.
A simple example is SimpleFacet which compares all its terms which have been added via SimpleFacet::addTerm() to term
. Depending on the selectionMode() it also checks for AndTerm or OrTerm.
- Warning
- Implementations of this method should never reset the selection before handling
term
. Instead the method should work similar to setSelected(), ie. in MatchAll or MatchAny facets calling it multiple times should select multiple choices.
- Returns
true
if all ofterm
could be used to select choices in this term,false
otherwise.
|
signal |
Emitted when the selection changed - normally triggered by a call to setSelected().
Subclasses should call setSelectionChanged() instead of emitting this signal manually.
Be aware that in most situations queryTermChanged() and selectionChanged() will have to be emitted at the same time.
|
pure virtual |
The selection mode used by this facet.
The GUI client can make use of this value to adjust the GUI accordingly. A typical example would be using radio buttons for MatchOne facets.
Implemented in Nepomuk::Utils::DynamicResourceFacet, Nepomuk::Utils::DateFacet, Nepomuk::Utils::SimpleFacet, Nepomuk::Utils::ProxyFacet, and Nepomuk::Utils::TypeFacet.
|
slot |
The FacetModel will set this to the final query that has been constructed from the facets and any other query part set by the client via FacetModel::setClientQuery().
The facet may use this information to filter the presented choices. A typical example is to only show terms that would actually change the result set. The latter is what DynamicResourceFacet does.
|
protectedslot |
Subclasses should call this method instead of emitting layoutChanged() manually.
|
protectedslot |
Subclasses should call this method instead of emitting termChanged() manually.
|
pure virtualslot |
Called by client code to change the selection.
- Parameters
-
index The index of the choice for which the selection should be changed. selected If true
the item should be selected, otherwise it should be deselected.
|
protectedslot |
Subclasses should call this method instead of emitting selectionChanged() manually.
|
virtual |
The text to be displayed at index
.
The default implementation makes use of guiItem(). Normally subclasses would implement guItem() instead of this method.
The documentation for this class was generated from the following file:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:48:27 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.