Nepomuk
#include <Nepomuk/Utils/FacetModel>
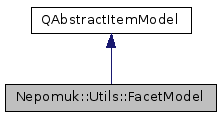
Public Types | |
enum | CustomRoles { FacetRole = 235265643 } |
Public Slots | |
void | addFacet (Nepomuk::Utils::Facet *facet) |
void | clear () |
void | clearSelection () |
Nepomuk::Query::Query | extractFacetsFromQuery (const Nepomuk::Query::Query &query) |
void | setClientQuery (const Nepomuk::Query::Query &query) |
void | setFacets (const QList< Nepomuk::Utils::Facet * > &facets) |
Signals | |
void | queryTermChanged (const Nepomuk::Query::Term &term) |
Public Member Functions | |
FacetModel (QObject *parent=0) | |
~FacetModel () | |
int | columnCount (const QModelIndex &parent=QModelIndex()) const |
QVariant | data (const QModelIndex &index, int role=Qt::DisplayRole) const |
QList< Facet * > | facets () const |
Qt::ItemFlags | flags (const QModelIndex &index) const |
bool | hasChildren (const QModelIndex &parent) const |
QModelIndex | index (int row, int column, const QModelIndex &parent=QModelIndex()) const |
QModelIndex | parent (const QModelIndex &index) const |
Query::Term | queryTerm () const |
int | rowCount (const QModelIndex &parent=QModelIndex()) const |
bool | setData (const QModelIndex &index, const QVariant &value, int role) |
Detailed Description
A FacetModel contains a list of facets that are provided in a tree structure.
The FacetModel manages a list of Facet instances that are layed out in a tree structure in which the leafs are checkable. This allows to easily configure the facets by clicking the tree.
Facets are added via setFacets() and addFacet().
The FacetModel can be used to create and augment queries using the queryTerm() method and connecting to the queryTermChanged() signal. In addition FacetModel provides the extractFacetsFromTerm() method which converts a query into facet selections. This is very convinient if the query comes from another source like a query bookmark or another application.
An improved user experience can be created by setting the final query used to list the results via setClientQuery(). This allows the FacetModel to filter the available choices, hiding those that do not make sense with the current result set or even showing facets that did not make sense before (compare the ProxyFacet example in Facet Examples).
Typically one would use FacetWidget instead of creating ones own FacetModel.
- Since
- 4.6
Definition at line 67 of file facetmodel.h.
Member Enumeration Documentation
Special roles FacetModel provides through data() in addition to the standard Qt roles.
- See also
- Qt::ItemDataRole
Enumerator | |
---|---|
FacetRole |
Provides a pointer to the Facet instance itself. Used internally. |
Definition at line 88 of file facetmodel.h.
Constructor & Destructor Documentation
Nepomuk::Utils::FacetModel::FacetModel | ( | QObject * | parent = 0 | ) |
Creates an empty facet model.
Nepomuk::Utils::FacetModel::~FacetModel | ( | ) |
Destructor.
Member Function Documentation
|
slot |
Add facet
to the model.
Used to populate the model with facets. Ownership of facet
is transferred to the model.
|
slot |
Remove all facets from the model.
|
slot |
Convenience method that clears the selection on all facets.
- See also
- Facet::clearSelection()
int Nepomuk::Utils::FacetModel::columnCount | ( | const QModelIndex & | parent = QModelIndex() | ) | const |
Reimplemented to provide facet data to Qt's model/view framework.
QVariant Nepomuk::Utils::FacetModel::data | ( | const QModelIndex & | index, |
int | role = Qt::DisplayRole |
||
) | const |
Reimplemented to provide facet data to Qt's model/view framework.
|
slot |
Extract as many facets from a query as possible.
This method is not able to handle all kinds of queries but works well on queries created via queryTerm().
Facets supported by this model will be extracted from query
and configured accordingly in the model.
Be aware that this method is not related to setClientQuery(). It is intended to split up a query in order to represent it graphically. It will, however change the client query in order to achieve the best possible term extraction. Thus, one would typically call setClientQuery after calling this method if query
does not already contain the full client query.
Typically a client would call this method and then try to handle the returned rest query in another way like converting it into a desktop user query string that can be shown in a search line edit. Another idea would be to use custom filters or a simple warning for the user that additional conditions are in place that could not be "translated" into facets.
- Returns
- The rest query after facets have been extracted.
QList<Facet*> Nepomuk::Utils::FacetModel::facets | ( | ) | const |
- Returns
- All Facet instances added via addFacet() and setFacets().
Qt::ItemFlags Nepomuk::Utils::FacetModel::flags | ( | const QModelIndex & | index | ) | const |
Reimplemented to provide facet data to Qt's model/view framework.
bool Nepomuk::Utils::FacetModel::hasChildren | ( | const QModelIndex & | parent | ) | const |
Reimplemented to provide facet data to Qt's model/view framework.
QModelIndex Nepomuk::Utils::FacetModel::index | ( | int | row, |
int | column, | ||
const QModelIndex & | parent = QModelIndex() |
||
) | const |
Reimplemented to provide facet data to Qt's model/view framework.
QModelIndex Nepomuk::Utils::FacetModel::parent | ( | const QModelIndex & | index | ) | const |
Reimplemented to provide facet data to Qt's model/view framework.
Query::Term Nepomuk::Utils::FacetModel::queryTerm | ( | ) | const |
Construct a query term from the selected facets in this model.
- Returns
- A new query which combines the facets in this model.
|
signal |
Emitted whenever the facets change, i.e.
when the user changes the selection or it is changed programmatically via extractFacetsFromQuery()
int Nepomuk::Utils::FacetModel::rowCount | ( | const QModelIndex & | parent = QModelIndex() | ) | const |
Reimplemented to provide facet data to Qt's model/view framework.
|
slot |
Can be used to set the full query the client is using (this includes facets created through this model).
It allows the facet system to disable certain choices that would not change the result set or do not make sense otherwise.
Be aware that this method is not related to extractFacetsFromTerm(). It is merely intended to improve the overall user experienceby filtering the facet choices depending on the current query.
Typically a client would call both extractFacetsFromTerm() and setClientQuery() seperately. However, they will often be called with the same query/term.
- See also
- Facet::setClientQuery()
bool Nepomuk::Utils::FacetModel::setData | ( | const QModelIndex & | index, |
const QVariant & | value, | ||
int | role | ||
) |
Reimplemented to provide facet data to Qt's model/view framework.
|
slot |
Set facets
as the list of facets used in this model.
Used to populate the model with facets. Ownership of the facets is transferred to the model.
The documentation for this class was generated from the following file:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:48:27 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.