Nepomuk
#include <Nepomuk/Utils/DynamicResourceFacet>
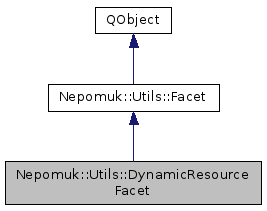
Public Slots | |
void | clearSelection () |
bool | selectFromTerm (const Nepomuk::Query::Term &queryTerm) |
void | setSelected (int index, bool selected=true) |
void | setSelected (const Nepomuk::Resource &res, bool selected=true) |
![]() | |
virtual void | clearSelection ()=0 |
virtual bool | selectFromTerm (const Nepomuk::Query::Term &queryTerm)=0 |
void | setClientQuery (const Nepomuk::Query::Query &query) |
virtual void | setSelected (int index, bool selected=true)=0 |
Public Member Functions | |
DynamicResourceFacet (QObject *parent=0) | |
virtual | ~DynamicResourceFacet () |
int | count () const |
KGuiItem | guiItem (int index) const |
bool | isSelected (int index) const |
int | maxRows () const |
Query::Term | queryTerm () const |
Types::Property | relation () const |
Nepomuk::Resource | resourceAt (int i) const |
Types::Class | resourceType () const |
QList< Resource > | selectedResources () const |
SelectionMode | selectionMode () const |
void | setMaxRows (int max) |
void | setRelation (const Types::Property &prop) |
void | setResourceType (const Types::Class &type) |
void | setSelectionMode (SelectionMode mode) |
![]() | |
Facet (QObject *parent=0) | |
virtual | ~Facet () |
Query::Query | clientQuery () const |
virtual QString | text (int index) const |
Protected Member Functions | |
virtual QList< Resource > | getMoreResources () const |
void | handleClientQueryChange () |
virtual Nepomuk::Resource | resourceForTerm (const Nepomuk::Query::Term &queryTerm) const |
virtual Query::Query | resourceQuery (const Query::Query &clientQuery) const |
virtual Nepomuk::Query::Term | termForResource (const Resource &res) const |
Additional Inherited Members | |
![]() | |
enum | SelectionMode { MatchAll, MatchAny, MatchOne } |
![]() | |
void | layoutChanged (Nepomuk::Utils::Facet *facet) |
void | queryTermChanged (Nepomuk::Utils::Facet *facet, const Nepomuk::Query::Term &queryTerm) |
void | selectionChanged (Nepomuk::Utils::Facet *facet) |
![]() | |
static Facet * | createDateFacet (QObject *parent=0) |
static Facet * | createFileTypeFacet (QObject *parent=0) |
static Facet * | createPriorityFacet (QObject *parent=0) |
static Facet * | createRatingFacet (QObject *parent=0) |
static Facet * | createTagFacet (QObject *parent=0) |
static Facet * | createTypeFacet (QObject *parent=0) |
![]() | |
void | setLayoutChanged () |
void | setQueryTermChanged () |
void | setSelectionChanged () |
Detailed Description
A facet that presents a dynamic lists of resources with the possibility to search for more.
The DynamicResourceFacet provides a way for the user to choose one or more resources which in combination with a property are used to construct Query::ComparisonTerm instances that make up the facet's term.
A typical use case would be the relation to a project or a person or a tag. The latter, however, is already provided via Facet::createTagFacet().
Usage is simple: set the property to use via setRelation() and optionally a resource type via setResourceType().
For customized query terms and resource listing DynamicResourceFacet might also be used as a base class for a new facet by reimplementing methods like resourceTerm() or getMoreResources().
- Warning
- DynamicResourceFacet will create a local event loop in order to show a dialog when the user wants to search for more resource candidates.
- Since
- 4.6
Definition at line 70 of file dynamicresourcefacet.h.
Constructor & Destructor Documentation
Nepomuk::Utils::DynamicResourceFacet::DynamicResourceFacet | ( | QObject * | parent = 0 | ) |
Create a new empty facet.
|
virtual |
Destructor.
Member Function Documentation
|
slot |
Clear the selection.
If selectionMode() is MatchOne the first choice will be selected.
|
virtual |
- Returns
- The number of choices in the facet.
Implements Nepomuk::Utils::Facet.
|
protectedvirtual |
Used to query additional resources if maxRows() is too small to fit all candidates.
The default implementation simply uses SearchWidget to let the user query additional resources.
- Warning
- The default implementation opens a dialog which will start a local event loop.
Reimplementations may use clientQuery() to optimize the resource selection.
- Returns
- The list of resources that should be selected in addition to the already selected ones.
|
virtual |
The parameters used to render the choice at index
.
Reimplemented from Nepomuk::Utils::Facet.
|
protectedvirtual |
Reimplemented to properly update the resource list on client query change.
Reimplemented from Nepomuk::Utils::Facet.
|
virtual |
- Returns
true
if the choice atindex
is selected,false
otherwise.
Implements Nepomuk::Utils::Facet.
int Nepomuk::Utils::DynamicResourceFacet::maxRows | ( | ) | const |
- Returns
- The number of maximum rows set via setMaxRows().
|
virtual |
- Returns
- The currently selected Term. Depending on the selectionMode() this is either a single Term, a combination through Query::AndTerm, or a combination through Query::OrTerm.
Implements Nepomuk::Utils::Facet.
Types::Property Nepomuk::Utils::DynamicResourceFacet::relation | ( | ) | const |
- Returns
- The property set via setRelation()
Nepomuk::Resource Nepomuk::Utils::DynamicResourceFacet::resourceAt | ( | int | i | ) | const |
- Returns
- The resource that is represented by the choice at index
i
or an invalid Resource in case is out of bounds or points to the "more" action.
|
protectedvirtual |
Used by selectFromTerm() to convert terms into resources.
This is the counterpart to termForResource(). The default implementation checks if term
is a Query::ComparisonTerm which uses relation().
When reimplementing this method termForResource() also needs to be reimplemented.
- Returns
- The resource that is represented by
term
if the latter has the form as terms generated by termForResource(), an invalid Resource otherwise.
|
protectedvirtual |
Construct the query that will be used to determine the resources that are presented as choices in the facet.
The default implementation uses resourceType() in combination with clientQuery
to determine the resources that can actually change the final query results.
- Parameters
-
clientQuery The query set via setClientQuery(). Reimplementations should use this to restrict the query to resources that actually change the final query results.
Reimplementations might also call the default implementation to simply add additional restrictions to the query like so:
- Returns
- The query that is used to fetch resources to be provided as choices in this facet.
Types::Class Nepomuk::Utils::DynamicResourceFacet::resourceType | ( | ) | const |
- Returns
- The resource type set via setResourceType() or the range of relation() in case no specific resource type was set.
QList<Resource> Nepomuk::Utils::DynamicResourceFacet::selectedResources | ( | ) | const |
- Returns
- The currently selected resources.
|
slot |
The term
is analyzed depending on the selectionMode().
- See also
- Facet::selectFromTerm()
|
virtual |
The selection mode set via setSelectionMode()
Implements Nepomuk::Utils::Facet.
void Nepomuk::Utils::DynamicResourceFacet::setMaxRows | ( | int | max | ) |
Set the maximum rows to show by default.
Further resources are accessible through a search dialog. By default the number of max rows is 5.
void Nepomuk::Utils::DynamicResourceFacet::setRelation | ( | const Types::Property & | prop | ) |
Set the property to use in the facet.
void Nepomuk::Utils::DynamicResourceFacet::setResourceType | ( | const Types::Class & | type | ) |
This is optional and defaults to the property's range if not set.
|
slot |
Select or deselect the resource at index
.
|
slot |
Select or deselect res
.
If res
is not of the correct type nothing happens.
void Nepomuk::Utils::DynamicResourceFacet::setSelectionMode | ( | SelectionMode | mode | ) |
Set the selection mode to use in this facet.
The default is MatchAny.
|
protectedvirtual |
Used by term() to construct the final query term this facet provides.
Reimplementing this method allows to customize the way the term is built.
The default implementation uses relation() in a Query::ComparisonTerm.
When reimplementing this method resourceForTerm() also needs to be reimplemented.
The documentation for this class was generated from the following file:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:48:27 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.