Plasma
#include <Plasma/Animator>
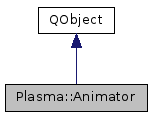
Public Types | |
enum | Animation { AppearAnimation = 0, DisappearAnimation, ActivateAnimation, FadeAnimation, GrowAnimation, PulseAnimation, RotationAnimation, RotationStackedAnimation, SlideAnimation, GeometryAnimation, ZoomAnimation, PixmapTransitionAnimation, WaterAnimation, LastAnimation = 1024 } |
enum | CurveShape { EaseInCurve = 0, EaseOutCurve, EaseInOutCurve, LinearCurve, PendularCurve } |
enum | Movement { SlideInMovement = 0, SlideOutMovement, FastSlideInMovement, FastSlideOutMovement } |
Signals | |
void | animationFinished (QGraphicsItem *item, Plasma::Animator::Animation anim) |
void | customAnimationFinished (int id) |
void | elementAnimationFinished (int id) |
void | movementFinished (QGraphicsItem *item) |
void | scrollStateChanged (QGraphicsWidget *widget, QAbstractAnimation::State newState, QAbstractAnimation::State oldState) |
Public Member Functions | |
Q_INVOKABLE int | animateElement (QGraphicsItem *obj, Animation) |
Q_INVOKABLE int | animateItem (QGraphicsItem *item, Animation anim) |
Q_INVOKABLE QPixmap | currentPixmap (int id) |
Q_INVOKABLE int | customAnimation (int frames, int duration, Animator::CurveShape curve, QObject *receiver, const char *method) |
Q_INVOKABLE bool | isAnimating () const |
Q_INVOKABLE int | moveItem (QGraphicsItem *item, Movement movement, const QPoint &destination) |
void | registerScrollingManager (QGraphicsWidget *widget) |
Q_INVOKABLE void | setInitialPixmap (int id, const QPixmap &pixmap) |
Q_INVOKABLE void | stopCustomAnimation (int id) |
Q_INVOKABLE void | stopElementAnimation (int id) |
Q_INVOKABLE void | stopItemAnimation (int id) |
Q_INVOKABLE void | stopItemMovement (int id) |
void | unregisterScrollingManager (QGraphicsWidget *widget) |
Static Public Member Functions | |
static Plasma::Animation * | create (Animator::Animation type, QObject *parent=0) |
static Plasma::Animation * | create (const QString &animationName, QObject *parent=0) |
static QEasingCurve | create (Animator::CurveShape type) |
static Animator * | self () |
Protected Member Functions | |
void | timerEvent (QTimerEvent *event) |
Detailed Description
A system for applying effects to Plasma elements.
Definition at line 46 of file animator.h.
Member Enumeration Documentation
Definition at line 55 of file animator.h.
Enumerator | |
---|---|
EaseInCurve | |
EaseOutCurve | |
EaseInOutCurve | |
LinearCurve | |
PendularCurve |
Definition at line 73 of file animator.h.
Enumerator | |
---|---|
SlideInMovement | |
SlideOutMovement | |
FastSlideInMovement | |
FastSlideOutMovement |
Definition at line 81 of file animator.h.
Member Function Documentation
int Plasma::Animator::animateElement | ( | QGraphicsItem * | obj, |
Animation | animation | ||
) |
Definition at line 431 of file deprecated/animator.cpp.
int Plasma::Animator::animateItem | ( | QGraphicsItem * | item, |
Animation | anim | ||
) |
Starts a standard animation on a QGraphicsItem.
- Parameters
-
item the item to animate in some fashion anim the type of animation to perform
- Returns
- the id of the animation
- Deprecated:
- use new Animator API with Qt Kinetic
Definition at line 230 of file deprecated/animator.cpp.
|
signal |
|
static |
Factory to build new animation objects.
To control their behavior, check AbstractAnimation properties.
- Since
- 4.4
Definition at line 61 of file animator.cpp.
|
static |
Factory to build new animation objects from Javascript files.
To control their behavior, check AbstractAnimation properties.
- Since
- 4.5
Definition at line 184 of file animator.cpp.
|
static |
QPixmap Plasma::Animator::currentPixmap | ( | int | id | ) |
Definition at line 503 of file deprecated/animator.cpp.
int Plasma::Animator::customAnimation | ( | int | frames, |
int | duration, | ||
Animator::CurveShape | curve, | ||
QObject * | receiver, | ||
const char * | method | ||
) |
Starts a custom animation, preventing the need to create a timeline with its own timer tick.
- Parameters
-
frames the number of frames this animation should persist for duration the length, in milliseconds, the animation will take curve the curve applied to the frame rate receive the object that will handle the actual animation method the method name of slot to be invoked on each update. It must take a qreal. So if the slot is animate(qreal), pass in "animate" as the method parameter. It has an optional integer paramenter that takes an integer that reapresents the animation id, useful if you want to manage multiple animations with a sigle slot
- Returns
- an id that can be used to identify this animation.
- Deprecated:
- use new Animator API with Qt Kinetic
Definition at line 340 of file deprecated/animator.cpp.
|
signal |
|
signal |
bool Plasma::Animator::isAnimating | ( | ) | const |
Can be used to query if there are other animations happening.
This way heavy operations can be delayed until all animations are finished.
- Returns
- true if there are animations going on.
- Since
- 4.1
- Deprecated:
- use new Animator API with Qt Kinetic
Definition at line 535 of file deprecated/animator.cpp.
int Plasma::Animator::moveItem | ( | QGraphicsItem * | item, |
Movement | movement, | ||
const QPoint & | destination | ||
) |
Starts a standard animation on a QGraphicsItem.
- Parameters
-
item the item to animate in some fashion anim the type of animation to perform
- Returns
- the id of the animation
- Deprecated:
- use new Animator API with Qt Kinetic
Definition at line 286 of file deprecated/animator.cpp.
|
signal |
void Plasma::Animator::registerScrollingManager | ( | QGraphicsWidget * | widget | ) |
Register a widget as a scrolling widget.
This function is deprecated: use a ScrollWidget, with setWidget() as your widget instead.
- Parameters
-
widget the widget that offers a scrolling behaviour
- Since
- 4.4
Definition at line 764 of file deprecated/animator.cpp.
|
signal |
|
static |
Singleton accessor.
Definition at line 142 of file deprecated/animator.cpp.
void Plasma::Animator::setInitialPixmap | ( | int | id, |
const QPixmap & | pixmap | ||
) |
Definition at line 491 of file deprecated/animator.cpp.
void Plasma::Animator::stopCustomAnimation | ( | int | id | ) |
Stops a custom animation.
Note that it is not necessary to call this on object destruction, as custom animations associated with a given QObject are cleaned up automatically on QObject destruction.
- Parameters
-
id the id of the animation as returned by customAnimation
- Deprecated:
- use new Animator API with Qt Kinetic
Definition at line 379 of file deprecated/animator.cpp.
void Plasma::Animator::stopElementAnimation | ( | int | id | ) |
Definition at line 476 of file deprecated/animator.cpp.
void Plasma::Animator::stopItemAnimation | ( | int | id | ) |
Stops an item animation before the animation is complete.
Note that it is not necessary to call this on normal completion of the animation.
- Parameters
-
id the id of the animation as returned by animateItem
- Deprecated:
- use new Animator API with Qt Kinetic
Definition at line 395 of file deprecated/animator.cpp.
void Plasma::Animator::stopItemMovement | ( | int | id | ) |
Stops an item movement before the animation is complete.
Note that it is not necessary to call this on normal completion of the animation.
- Parameters
-
id the id of the animation as returned by moveItem
- Deprecated:
- use new Animator API with Qt Kinetic
Definition at line 413 of file deprecated/animator.cpp.
|
protected |
Definition at line 543 of file deprecated/animator.cpp.
void Plasma::Animator::unregisterScrollingManager | ( | QGraphicsWidget * | widget | ) |
unregister the scrolling manager of a certain widget This function is deprecated: use ScrollWidget instead.
- Parameters
-
widget the widget we don't want no longer animated
- Since
- 4.4
Definition at line 775 of file deprecated/animator.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:48:34 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.