Plasma
#include <Plasma/ContainmentActions>
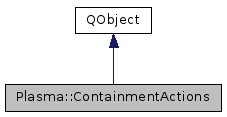
Public Member Functions | |
ContainmentActions (QObject *parent=0) | |
~ContainmentActions () | |
virtual void | configurationAccepted () |
bool | configurationRequired () const |
virtual void | contextEvent (QEvent *event) |
virtual QList< QAction * > | contextualActions () |
virtual QWidget * | createConfigurationInterface (QWidget *parent) |
Q_INVOKABLE DataEngine * | dataEngine (const QString &name) const |
bool | event (QEvent *e) |
QString | icon () const |
bool | isInitialized () const |
QString | name () const |
QString | pluginName () const |
QPoint | popupPosition (const QSize &s, QEvent *event) |
void | restore (const KConfigGroup &config) |
virtual void | save (KConfigGroup &config) |
void | setContainment (Containment *newContainment) |
Static Public Member Functions | |
static QString | eventToString (QEvent *event) |
static KPluginInfo::List | listContainmentActionsInfo () |
static ContainmentActions * | load (Containment *parent, const QString &name, const QVariantList &args=QVariantList()) |
static ContainmentActions * | load (Containment *parent, const KPluginInfo &info, const QVariantList &args=QVariantList()) |
static PackageStructure::Ptr | packageStructure () |
Protected Member Functions | |
ContainmentActions (QObject *parent, const QVariantList &args) | |
Containment * | containment () |
virtual void | init (const KConfigGroup &config) |
void | paste (QPointF scenePos, QPoint screenPos) |
void | setConfigurationRequired (bool needsConfiguring=true) |
Properties | |
QString | icon |
QString | name |
QString | pluginName |
Detailed Description
The base ContainmentActions class.
"ContainmentActions" are components that provide actions (usually displaying a contextmenu) in response to an event with a position (usually a mouse event).
ContainmentActions plugins are registered using .desktop files. These files should be named using the following naming scheme:
plasma-containmentactions-\<pluginname\>.desktop
Definition at line 55 of file containmentactions.h.
Constructor & Destructor Documentation
|
explicit |
Default constructor for an empty or null containmentactions.
Definition at line 47 of file containmentactions.cpp.
Plasma::ContainmentActions::~ContainmentActions | ( | ) |
Definition at line 68 of file containmentactions.cpp.
|
protected |
This constructor is to be used with the plugin loading systems found in KPluginInfo and KService.
The argument list is expected to have one element: the KService service ID for the desktop entry.
- Parameters
-
parent a QObject parent; you probably want to pass in 0 args a list of strings containing one entry: the service id
Definition at line 53 of file containmentactions.cpp.
Member Function Documentation
|
virtual |
This method is called when the user's configuration changes are accepted.
Definition at line 193 of file containmentactions.cpp.
bool Plasma::ContainmentActions::configurationRequired | ( | ) | const |
- Returns
- true if the containmentactions currently needs to be configured, otherwise, false
Definition at line 214 of file containmentactions.cpp.
|
protected |
- Returns
- the containment the plugin is associated with.
Definition at line 131 of file containmentactions.cpp.
|
virtual |
Implement this to respond to events.
The user can configure whatever button and modifier they like, so please don't look at those parameters. The event may be a QGraphicsSceneMouseEvent or a QGraphicsSceneWheelEvent.
Definition at line 198 of file containmentactions.cpp.
|
virtual |
Implement this to provide a list of actions that can be added to another menu for example, when right-clicking an applet, the "Activity Options" submenu is populated with this.
Definition at line 203 of file containmentactions.cpp.
Returns the widget used in the configuration dialog.
Add the configuration interface of the containmentactions to this widget.
Definition at line 187 of file containmentactions.cpp.
DataEngine * Plasma::ContainmentActions::dataEngine | ( | const QString & | name | ) | const |
Loads the given DataEngine.
Tries to load the data engine given by name
. Each engine is only loaded once, and that instance is re-used on all subsequent requests.
If the data engine was not found, an invalid data engine is returned (see DataEngine::isValid()).
Note that you should not delete the returned engine.
- Parameters
-
name Name of the data engine to load
- Returns
- pointer to the data engine if it was loaded, or an invalid data engine if the requested engine could not be loaded
Definition at line 209 of file containmentactions.cpp.
bool Plasma::ContainmentActions::event | ( | QEvent * | e | ) |
Definition at line 383 of file containmentactions.cpp.
|
static |
Turns a mouse or wheel event into a string suitable for a ContainmentActions.
- Returns
- the string representation of the event
Definition at line 225 of file containmentactions.cpp.
QString Plasma::ContainmentActions::icon | ( | ) | const |
Returns the icon related to this containmentactions.
|
protectedvirtual |
This method is called once the containmentactions is loaded or settings are changed.
- Parameters
-
config Config group to load settings
Definition at line 177 of file containmentactions.cpp.
bool Plasma::ContainmentActions::isInitialized | ( | ) | const |
- Returns
- true if initialized (usually by calling restore), false otherwise
Definition at line 166 of file containmentactions.cpp.
|
static |
Returns a list of all known containmentactions plugins.
- Returns
- list of containmentactions plugins
Definition at line 73 of file containmentactions.cpp.
|
static |
Attempts to load a containmentactions.
Returns a pointer to the containmentactions if successful. The caller takes responsibility for the containmentactions, including deleting it when no longer needed.
- Parameters
-
parent the parent containment.
- Since
- 4.6 null is allowed.
- Parameters
-
name the plugin name, as returned by KPluginInfo::pluginName() args to send the containmentactions extra arguments
- Returns
- a pointer to the loaded containmentactions, or 0 on load failure
Definition at line 81 of file containmentactions.cpp.
|
static |
Attempts to load a containmentactions.
Returns a pointer to the containmentactions if successful. The caller takes responsibility for the containmentactions, including deleting it when no longer needed.
- Parameters
-
parent the parent containment.
- Since
- 4.6 null is allowed.
- Parameters
-
info KPluginInfo object for the desired containmentactions args to send the containmentactions extra arguments
- Returns
- a pointer to the loaded containmentactions, or 0 on load failure
Definition at line 114 of file containmentactions.cpp.
QString Plasma::ContainmentActions::name | ( | ) | const |
Returns the user-visible name for the containmentactions, as specified in the .desktop file.
- Returns
- the user-visible name for the containmentactions.
|
static |
Returns the Package specialization for containmentactions.
Definition at line 122 of file containmentactions.cpp.
|
protected |
pastes the clipboard at a given location
Definition at line 293 of file containmentactions.cpp.
QString Plasma::ContainmentActions::pluginName | ( | ) | const |
Returns the plugin name for the containmentactions.
QPoint Plasma::ContainmentActions::popupPosition | ( | const QSize & | s, |
QEvent * | event | ||
) |
Returns a popup position appropriate to the event and the size.
- Parameters
-
s size of the popup event a pointer to the event that triggered the popup
- Returns
- the preferred top-left position for the popup
- Since
- 4.6
Definition at line 348 of file containmentactions.cpp.
void Plasma::ContainmentActions::restore | ( | const KConfigGroup & | config | ) |
This method should be called once the plugin is loaded or settings are changed.
- Parameters
-
config Config group to load settings
- See also
- init
Definition at line 171 of file containmentactions.cpp.
|
virtual |
This method is called when settings need to be saved.
- Parameters
-
config Config group to save settings
Definition at line 182 of file containmentactions.cpp.
|
protected |
When the containmentactions needs to be configured before being usable, this method can be called to denote that action is required.
- Parameters
-
needsConfiguring true if the applet needs to be configured, or false if it doesn't
Definition at line 219 of file containmentactions.cpp.
void Plasma::ContainmentActions::setContainment | ( | Containment * | newContainment | ) |
newContainment
the containment the plugin should be associated with.
- Since
- 4.6
Definition at line 389 of file containmentactions.cpp.
Property Documentation
|
read |
Definition at line 60 of file containmentactions.h.
|
read |
Definition at line 58 of file containmentactions.h.
|
read |
Definition at line 59 of file containmentactions.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:48:35 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.