Plasma
#include <Plasma/Corona>
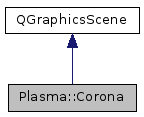
Public Slots | |
ImmutabilityType | immutability () const |
void | initializeLayout (const QString &config=QString()) |
void | layoutContainments () |
void | loadLayout (const QString &config=QString()) |
void | requestConfigSync () |
void | requireConfigSync () |
void | saveLayout (const QString &config=QString()) const |
void | setImmutability (const ImmutabilityType immutable) |
Signals | |
void | availableScreenRegionChanged () |
void | configSynced () |
void | containmentAdded (Plasma::Containment *containment) |
void | immutabilityChanged (Plasma::ImmutabilityType immutability) |
void | releaseVisualFocus () |
void | screenOwnerChanged (int wasScreen, int isScreen, Plasma::Containment *containment) |
void | shortcutsChanged () |
Public Member Functions | |
Corona (QObject *parent=0) | |
~Corona () | |
QAction * | action (QString name) const |
QList< QAction * > | actions () const |
void | addAction (QString name, QAction *action) |
KAction * | addAction (QString name) |
Containment * | addContainment (const QString &name, const QVariantList &args=QVariantList()) |
Containment * | addContainmentDelayed (const QString &name, const QVariantList &args=QVariantList()) |
void | addOffscreenWidget (QGraphicsWidget *widget) |
void | addShortcuts (KActionCollection *newShortcuts) |
QString | appletMimeType () |
virtual QRegion | availableScreenRegion (int id) const |
void | clearContainments () |
KSharedConfig::Ptr | config () const |
ContainmentActionsPluginsConfig | containmentActionsDefaults (Containment::Type containmentType) |
Containment * | containmentForScreen (int screen, int desktop=-1) const |
Containment * | containmentForScreen (int screen, int desktop, const QString &defaultPluginIfNonExistent, const QVariantList &defaultArgs=QVariantList()) |
QList< Containment * > | containments () const |
QString | defaultContainmentPlugin () const |
AbstractDialogManager * | dialogManager () |
void | enableAction (const QString &name, bool enable) |
void | exportLayout (KConfigGroup &config, QList< Containment * > containments) |
QList< Plasma::Location > | freeEdges (int screen) const |
QList< Plasma::Containment * > | importLayout (const KConfigBase &config) |
QList< Plasma::Containment * > | importLayout (const KConfigGroup &config) |
virtual int | numScreens () const |
QList< QGraphicsWidget * > | offscreenWidgets () const |
QPoint | popupPosition (const QGraphicsItem *item, const QSize &size) |
QPoint | popupPosition (const QGraphicsItem *item, const QSize &size, Qt::AlignmentFlag alignment) |
QString | preferredToolBoxPlugin (const Containment::Type type) const |
void | removeOffscreenWidget (QGraphicsWidget *widget) |
virtual QRect | screenGeometry (int id) const |
void | setAppletMimeType (const QString &mimetype) |
void | setContainmentActionsDefaults (Containment::Type containmentType, const ContainmentActionsPluginsConfig &config) |
void | setDialogManager (AbstractDialogManager *manager) |
void | updateShortcuts () |
Protected Member Functions | |
void | dragEnterEvent (QGraphicsSceneDragDropEvent *event) |
void | dragLeaveEvent (QGraphicsSceneDragDropEvent *event) |
void | dragMoveEvent (QGraphicsSceneDragDropEvent *event) |
virtual void | loadDefaultLayout () |
void | mapAnimation (Animator::Animation from, Animator::Animation to) |
void | mapAnimation (Animator::Animation from, const QString &to) |
void | setDefaultContainmentPlugin (const QString &name) |
void | setPreferredToolBoxPlugin (const Containment::Type type, const QString &plugin) |
Detailed Description
A QGraphicsScene for Plasma::Applets.
Constructor & Destructor Documentation
|
explicit |
Definition at line 64 of file corona.cpp.
Plasma::Corona::~Corona | ( | ) |
Definition at line 74 of file corona.cpp.
Member Function Documentation
QAction * Plasma::Corona::action | ( | QString | name | ) | const |
Returns the QAction with the given name from our collection.
Definition at line 716 of file corona.cpp.
QList< QAction * > Plasma::Corona::actions | ( | ) | const |
Returns all the actions in our collection.
Definition at line 731 of file corona.cpp.
void Plasma::Corona::addAction | ( | QString | name, |
QAction * | action | ||
) |
Adds the action to our collection under the given name.
Definition at line 721 of file corona.cpp.
KAction * Plasma::Corona::addAction | ( | QString | name | ) |
- Since
- 4.3 Creates an action in our collection under the given name
- Returns
- the new action FIXME I'm wrapping so much of kactioncollection API now, maybe I should just expose the collection itself :P
Definition at line 726 of file corona.cpp.
Containment * Plasma::Corona::addContainment | ( | const QString & | name, |
const QVariantList & | args = QVariantList() |
||
) |
Adds a Containment to the Corona.
- Parameters
-
name the plugin name for the containment, as given by KPluginInfo::pluginName(). If an empty string is passed in, the default containment plugin will be used (usually DesktopContainment). If the string literal "null" is passed in, then no plugin will be loaded and a simple Containment object will be created instead. args argument list to pass to the containment
- Returns
- a pointer to the containment on success, or 0 on failure. Failure can be caused by too restrictive of an Immutability type, as containments cannot be added when widgets are locked, or if the requested containment plugin can not be located or successfully loaded.
Definition at line 349 of file corona.cpp.
Containment * Plasma::Corona::addContainmentDelayed | ( | const QString & | name, |
const QVariantList & | args = QVariantList() |
||
) |
Loads a containment with delayed initialization, primarily useful for implementations of loadDefaultLayout.
The caller is responsible for all initializating, saving and notification of a new containment.
- Parameters
-
name the plugin name for the containment, as given by KPluginInfo::pluginName(). If an empty string is passed in, the defalt containment plugin will be used (usually DesktopContainment). If the string literal "null" is passed in, then no plugin will be loaded and a simple Containment object will be created instead. args argument list to pass to the containment
- Returns
- a pointer to the containment on success, or 0 on failure. Failure can be caused by the Immutability type being too restrictive, as containments can't be added when widgets are locked, or if the requested containment plugin can not be located or successfully loaded.
- See also
- addContainment
Definition at line 358 of file corona.cpp.
void Plasma::Corona::addOffscreenWidget | ( | QGraphicsWidget * | widget | ) |
Adds a widget in the topleft quadrant in the scene.
Widgets in the topleft quadrant are normally never shown unless you specifically aim a view at it, which makes it ideal for toplevel views etc.
- Parameters
-
widget the widget to add.
Definition at line 377 of file corona.cpp.
void Plasma::Corona::addShortcuts | ( | KActionCollection * | newShortcuts | ) |
- Since
- 4.3 Adds a set of actions to the shortcut config dialog. don't use this on actions in the corona's own actioncollection, those are handled automatically. this is for stuff outside of that.
Definition at line 764 of file corona.cpp.
QString Plasma::Corona::appletMimeType | ( | ) |
The current mime type of Drag/Drop items.
Definition at line 91 of file corona.cpp.
|
virtual |
Returns the available region for a given screen.
The available region excludes panels and similar windows. Valid screen ids are 0 to numScreens()-1. By default this method returns a rectangular region equal to screenGeometry(id); subclasses that need another behavior should override this method.
Definition at line 454 of file corona.cpp.
|
signal |
This signal inicates that a change in available screen goemetry occurred.
void Plasma::Corona::clearContainments | ( | ) |
Clear the Corona from all applets.
Definition at line 333 of file corona.cpp.
KSharedConfigPtr Plasma::Corona::config | ( | ) | const |
Returns the config file used to store the configuration for this Corona.
Definition at line 340 of file corona.cpp.
|
signal |
This signal indicates that the configuration file was flushed to disc.
ContainmentActionsPluginsConfig Plasma::Corona::containmentActionsDefaults | ( | Containment::Type | containmentType | ) |
- Since
- 4.4 Returns the default containmentactions plugins for the given containment type
Definition at line 777 of file corona.cpp.
|
signal |
This signal indicates a new containment has been added to the Corona.
Containment * Plasma::Corona::containmentForScreen | ( | int | screen, |
int | desktop = -1 |
||
) | const |
Returns the Containment, if any, for a given physical screen and desktop.
- Parameters
-
screen number of the physical screen to locate desktop the virtual desktop) to locate; if < 0 then it will simply return the first Containment associated with screen
Definition at line 296 of file corona.cpp.
Containment * Plasma::Corona::containmentForScreen | ( | int | screen, |
int | desktop, | ||
const QString & | defaultPluginIfNonExistent, | ||
const QVariantList & | defaultArgs = QVariantList() |
||
) |
Returns the Containment for a given physical screen and desktop, creating one if none exists.
- Parameters
-
screen number of the physical screen to locate desktop the virtual desktop) to locate; if < 0 then it will simply return the first Containment associated with screen defaultPluginIfNonExistent the plugin to load by default; "null" is an empty Containment and "default" creates the default plugin defaultArgs optional arguments to pass in when creating a Containment if needed
- Since
- 4.6
Definition at line 310 of file corona.cpp.
QList< Containment * > Plasma::Corona::containments | ( | ) | const |
- Returns
- all containments on this Corona
Definition at line 328 of file corona.cpp.
QString Plasma::Corona::defaultContainmentPlugin | ( | ) | const |
AbstractDialogManager * Plasma::Corona::dialogManager | ( | ) |
- Returns
- the AbstractDialogManager that will show dialogs used by applets, like configuration dialogs
- Since
- 4.5
Definition at line 787 of file corona.cpp.
|
protected |
Definition at line 643 of file corona.cpp.
|
protected |
Definition at line 648 of file corona.cpp.
|
protected |
Definition at line 653 of file corona.cpp.
void Plasma::Corona::enableAction | ( | const QString & | name, |
bool | enable | ||
) |
convenience function - enables or disables an action by name
- Parameters
-
name the name of the action in our collection enable true to enable, false to disable
Definition at line 736 of file corona.cpp.
void Plasma::Corona::exportLayout | ( | KConfigGroup & | config, |
QList< Containment * > | containments | ||
) |
Exports a set of containments to a config file.
- Parameters
-
config the config group to save to containments the list of containments to save
- Since
- 4.6
Definition at line 123 of file corona.cpp.
QList< Plasma::Location > Plasma::Corona::freeEdges | ( | int | screen | ) | const |
This method is useful in order to retrieve the list of available screen edges for panel type containments.
- Parameters
-
screen the id of the screen to look for free edges.
- Returns
- a list of free edges not filled with panel type containments.
Definition at line 700 of file corona.cpp.
|
slot |
- Returns
- The type of immutability of this Corona
Definition at line 658 of file corona.cpp.
|
signal |
emitted when immutability changes.
this is for use by things that don't get contraints events, like plasmaapp. it's NOT for containments or applets or any of the other stuff on the scene. if your code's not in shells/ it probably shouldn't be using it.
QList< Plasma::Containment * > Plasma::Corona::importLayout | ( | const KConfigBase & | config | ) |
Imports an applet layout from a config file.
The results will be added to the current set of Containments.
- Deprecated:
- Use the 4.6 version that takes a KConfigGroup
- Parameters
-
config the name of the config file to load from, or the default config file if QString()
- Returns
- the list of containments that were loaded
- Since
- 4.5
Definition at line 290 of file corona.cpp.
QList< Plasma::Containment * > Plasma::Corona::importLayout | ( | const KConfigGroup & | config | ) |
Imports an applet layout from a config file.
The results will be added to the current set of Containments.
- Parameters
-
config the name of the config file to load from, or the default config file if QString()
- Returns
- the list of containments that were loaded
- Since
- 4.6
Definition at line 284 of file corona.cpp.
|
slot |
Initializes the layout from a config file.
This will first clear any existing Containments, load a layout from the requested configuration file, request the default layout if needed and update immutability.
- Parameters
-
config the name of the config file to load from, or the default config file if QString()
Definition at line 179 of file corona.cpp.
|
slot |
- Since
- 4.5 Layout the containments on this corona. The default implementation organizes them in a grid-like view, but subclasses can reimplement this slot to provide their own layout.
Definition at line 206 of file corona.cpp.
|
protectedvirtual |
Loads the default (system wide) layout for this user.
Definition at line 628 of file corona.cpp.
|
slot |
Load applet layout from a config file.
The results will be added to the current set of Containments.
- Parameters
-
config the name of the config file to load from, or the default config file if QString()
Definition at line 272 of file corona.cpp.
|
protected |
Maps a stock animation to one of the semantic animations.
Used to control things such as what animation is used to make a Plasma::Appear appear in a containment.
- Parameters
-
from the animation to map a new value to to the animation value to map to from
- Since
- 4.5
Definition at line 367 of file corona.cpp.
|
protected |
Maps a loadable animation to one of the semantic animations.
Used to control things such as what animation is used to make a Plasma::Appear appear in a containment.
- Parameters
-
from the animation to map a new value to to the animation value to map to from; this must map to a Javascript animation
- Since
- 4.5
Definition at line 372 of file corona.cpp.
|
virtual |
Returns the number of screens available to plasma.
Subclasses should override this method as the default implementation returns a meaningless value.
Definition at line 436 of file corona.cpp.
QList< QGraphicsWidget * > Plasma::Corona::offscreenWidgets | ( | ) | const |
QPoint Plasma::Corona::popupPosition | ( | const QGraphicsItem * | item, |
const QSize & | size | ||
) |
Recommended position for a popup window like a menu or a tooltip given its size.
- Parameters
-
item the item that the popup should appear adjacent to (an applet, say) size size of the popup
- Returns
- reccomended position
Definition at line 459 of file corona.cpp.
QPoint Plasma::Corona::popupPosition | ( | const QGraphicsItem * | item, |
const QSize & | size, | ||
Qt::AlignmentFlag | alignment | ||
) |
- Since
- 4.4 Recommended position for a popup window like a menu or a tooltip given its size
- Parameters
-
item the item that the popup should appear adjacent to (an applet, say) size size of the popup alignment alignment of the popup, valid flags are Qt::AlignLeft, Qt::AlignRight and Qt::AlignCenter
- Returns
- reccomended position
Definition at line 464 of file corona.cpp.
QString Plasma::Corona::preferredToolBoxPlugin | ( | const Containment::Type | type | ) | const |
Returns the name of the preferred plugin to be used as containment toolboxes.
CustomContainments and CustomPanelContainments can still override it as their liking. It's also not guaranteed that the plugin will actually exist.
- Parameters
-
type the containment type of which we want to know the associated toolbox plugin
- Since
- 4.6
Definition at line 638 of file corona.cpp.
|
signal |
This signal indicates that an application launch, window creation or window focus event was triggered.
This is used, for instance, to ensure that the Dashboard view in Plasma hides when such an event is triggered by an item it is displaying.
void Plasma::Corona::removeOffscreenWidget | ( | QGraphicsWidget * | widget | ) |
Removes a widget from the topleft quadrant in the scene.
- Parameters
-
widget the widget to remove.
Definition at line 410 of file corona.cpp.
|
slot |
Schedules a flush-to-disk synchronization of the configuration state at the next convenient moment.
Definition at line 156 of file corona.cpp.
|
slot |
Schedules a time sensitive flush-to-disk synchronization of the configuration state.
Since this method does not provide any sort of event compression, it should only be used when an immediate disk sync is absolutely required. Otherwise, use
- See also
- requestConfigSync() which does do event compression.
Definition at line 174 of file corona.cpp.
|
slot |
Save applets layout to file.
- Parameters
-
config the file to save to, or the default config file if QString()
Definition at line 110 of file corona.cpp.
|
virtual |
Returns the geometry of a given screen.
Valid screen ids are 0 to numScreen()-1, or -1 for the full desktop geometry. Subclasses should override this method as the default implementation returns a meaningless value.
Definition at line 441 of file corona.cpp.
|
signal |
This signal indicates that a containment has been newly associated (or dissociated) with a physical screen.
- Parameters
-
wasScreen the screen it was associated with isScreen the screen it is now associated with containment the containment switching screens
void Plasma::Corona::setAppletMimeType | ( | const QString & | mimetype | ) |
Sets the mimetype of Drag/Drop items.
Default is text/x-plasmoidservicename
Definition at line 86 of file corona.cpp.
void Plasma::Corona::setContainmentActionsDefaults | ( | Containment::Type | containmentType, |
const ContainmentActionsPluginsConfig & | config | ||
) |
- Since
- 4.4 Sets the default containmentactions plugins for the given containment type
Definition at line 772 of file corona.cpp.
|
protected |
Sets the default containment plugin to try and load.
- Since
- 4.7
Definition at line 96 of file corona.cpp.
void Plasma::Corona::setDialogManager | ( | AbstractDialogManager * | manager | ) |
- Parameters
-
the AbstractDialogManager implementaion
- Since
- 4.5
Definition at line 782 of file corona.cpp.
|
slot |
Sets the immutability type for this Corona (not immutable, user immutable or system immutable)
- Parameters
-
immutable the new immutability type of this applet
Definition at line 663 of file corona.cpp.
|
protected |
- Returns
- The preferred toolbox plugin name for a given containment type.
- Parameters
-
type the containment type of which we want to know the preferred toolbox plugin. plugin the toolbox plugin name
- Since
- 4.6
Definition at line 632 of file corona.cpp.
|
signal |
- Since
- 4.3 emitted when the user changes keyboard shortcut settings connect to this if you've put some extra shortcuts in your app that are NOT in corona's actioncollection. if your code's not in shells/ it probably shouldn't be using this function.
- See also
- addShortcuts
void Plasma::Corona::updateShortcuts | ( | ) |
- Since
- 4.3 Updates keyboard shortcuts for all the corona's actions. If you've added actions to the corona you'll need to call this for them to be configurable.
Definition at line 745 of file corona.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:48:35 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.