Plasma
#include <Plasma/FrameSvg>
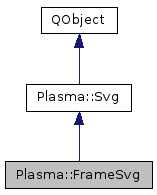
Public Types | |
enum | EnabledBorder { NoBorder = 0, TopBorder = 1, BottomBorder = 2, LeftBorder = 4, RightBorder = 8, AllBorders = TopBorder | BottomBorder | LeftBorder | RightBorder } |
Public Member Functions | |
~FrameSvg () | |
QPixmap | alphaMask () const |
Q_INVOKABLE bool | cacheAllRenderedFrames () const |
Q_INVOKABLE void | clearCache () |
Q_INVOKABLE QRectF | contentsRect () const |
EnabledBorders | enabledBorders () const |
Q_INVOKABLE QPixmap | framePixmap () |
Q_INVOKABLE QSizeF | frameSize () const |
Q_INVOKABLE void | getMargins (qreal &left, qreal &top, qreal &right, qreal &bottom) const |
Q_INVOKABLE bool | hasElementPrefix (const QString &prefix) const |
Q_INVOKABLE bool | hasElementPrefix (Plasma::Location location) const |
Q_INVOKABLE qreal | marginSize (const Plasma::MarginEdge edge) const |
Q_INVOKABLE QRegion | mask () const |
Q_INVOKABLE void | paintFrame (QPainter *painter, const QRectF &target, const QRectF &source=QRectF()) |
Q_INVOKABLE void | paintFrame (QPainter *painter, const QPointF &pos=QPointF(0, 0)) |
Q_INVOKABLE QString | prefix () |
Q_INVOKABLE void | resizeFrame (const QSizeF &size) |
Q_INVOKABLE void | setCacheAllRenderedFrames (bool cache) |
Q_INVOKABLE void | setElementPrefix (Plasma::Location location) |
Q_INVOKABLE void | setElementPrefix (const QString &prefix) |
void | setEnabledBorders (const EnabledBorders borders) |
Q_INVOKABLE void | setImagePath (const QString &path) |
![]() | |
~Svg () | |
bool | containsMultipleImages () const |
Q_INVOKABLE QString | elementAtPoint (const QPoint &point) const |
Q_INVOKABLE QRectF | elementRect (const QString &elementId) const |
Q_INVOKABLE QSize | elementSize (const QString &elementId) const |
Q_INVOKABLE bool | hasElement (const QString &elementId) const |
QString | imagePath () const |
bool | isUsingRenderingCache () const |
Q_INVOKABLE bool | isValid () const |
Q_INVOKABLE void | paint (QPainter *painter, const QPointF &point, const QString &elementID=QString()) |
Q_INVOKABLE void | paint (QPainter *painter, int x, int y, const QString &elementID=QString()) |
Q_INVOKABLE void | paint (QPainter *painter, const QRectF &rect, const QString &elementID=QString()) |
Q_INVOKABLE void | paint (QPainter *painter, int x, int y, int width, int height, const QString &elementID=QString()) |
Q_INVOKABLE QPixmap | pixmap (const QString &elementID=QString()) |
Q_INVOKABLE void | resize (qreal width, qreal height) |
Q_INVOKABLE void | resize (const QSizeF &size) |
Q_INVOKABLE void | resize () |
void | setContainsMultipleImages (bool multiple) |
void | setImagePath (const QString &svgFilePath) |
void | setTheme (Plasma::Theme *theme) |
void | setUsingRenderingCache (bool useCache) |
QSize | size () const |
Theme * | theme () const |
Properties | |
EnabledBorders | enabledBorders |
![]() | |
QString | imagePath |
bool | multipleImages |
QSize | size |
bool | usingRenderingCache |
Related Functions | |
(Note that these are not member functions.) | |
FrameSvg (QObject *parent=0) | |
![]() | |
Svg (QObject *parent=0) | |
Additional Inherited Members | |
![]() | |
void | repaintNeeded () |
void | sizeChanged () |
Detailed Description
Provides an SVG with borders.
When using SVG images for a background of an object that may change its aspect ratio, such as a dialog, simply scaling a single image may not be enough.
FrameSvg allows SVGs to provide several elements for borders as well as a central element, each of which are scaled individually. These elements should be named
center
- the central element, which will be scaled in both directionstop
- the top border; the height is fixed, but it will be scaled horizontally to the same width ascenter
bottom
- the bottom border; scaled in the same way astop
left
- the left border; the width is fixed, but it will be scaled vertically to the same height ascenter
right
- the right border; scaled in the same way asleft
topleft
- fixed size; must be the same height astop
and the same width asleft
bottomleft
,topright
,bottomright
- similar totopleft
center
must exist, but all the others are optional. topleft
and topright
will be ignored if top
does not exist, and similarly for bottomleft
and bottomright
.
- See also
- Plasma::Svg
Definition at line 76 of file framesvg.h.
Member Enumeration Documentation
These flags represents what borders should be drawn.
Enumerator | |
---|---|
NoBorder | |
TopBorder | |
BottomBorder | |
LeftBorder | |
RightBorder | |
AllBorders |
Definition at line 87 of file framesvg.h.
Constructor & Destructor Documentation
Plasma::FrameSvg::~FrameSvg | ( | ) |
Definition at line 57 of file framesvg.cpp.
Member Function Documentation
QPixmap Plasma::FrameSvg::alphaMask | ( | ) | const |
- Returns
- a pixmap whose alpha channel is the opacity of the frame. It may be the frame itself or a special frame with the mask- prefix
Definition at line 444 of file framesvg.cpp.
bool Plasma::FrameSvg::cacheAllRenderedFrames | ( | ) | const |
- Returns
- if all the different prefixes should be kept in a cache when rendered
Definition at line 473 of file framesvg.cpp.
void Plasma::FrameSvg::clearCache | ( | ) |
Deletes the internal cache freeing memory: use this if you want to switch the rendered element and you don't plan to switch back to the previous one for a long time and you used setUsingRenderingCache(true)
Definition at line 478 of file framesvg.cpp.
QRectF Plasma::FrameSvg::contentsRect | ( | ) | const |
- Returns
- the rectangle of the center element, taking the margins into account.
Definition at line 429 of file framesvg.cpp.
EnabledBorders Plasma::FrameSvg::enabledBorders | ( | ) | const |
Convenience method to get the enabled borders.
- Returns
- what borders are painted
QPixmap Plasma::FrameSvg::framePixmap | ( | ) |
Returns a pixmap of the SVG represented by this object.
- Parameters
-
elelementId the ID string of the element to render, or an empty string for the whole SVG (the default)
- Returns
- a QPixmap of the rendered SVG
Definition at line 499 of file framesvg.cpp.
QSizeF Plasma::FrameSvg::frameSize | ( | ) | const |
- Returns
- the size of the frame
Definition at line 377 of file framesvg.cpp.
void Plasma::FrameSvg::getMargins | ( | qreal & | left, |
qreal & | top, | ||
qreal & | right, | ||
qreal & | bottom | ||
) | const |
Convenience method that extracts the size of the four margins in the four output parameters.
- Parameters
-
left left margin size top top margin size right right margin size bottom bottom margin size
Definition at line 414 of file framesvg.cpp.
bool Plasma::FrameSvg::hasElementPrefix | ( | const QString & | prefix | ) | const |
- Returns
- true if the svg has the necessary elements with the given prefix to draw a frame
- Parameters
-
prefix the given prefix we want to check if drawable
Definition at line 276 of file framesvg.cpp.
bool Plasma::FrameSvg::hasElementPrefix | ( | Plasma::Location | location | ) | const |
This is an overloaded method provided for convenience equivalent to hasElementPrefix("north"), hasElementPrefix("south") hasElementPrefix("west") and hasElementPrefix("east")
- Returns
- true if the svg has the necessary elements with the given prefix to draw a frame.
- Parameters
-
location the given prefix we want to check if drawable
Definition at line 287 of file framesvg.cpp.
qreal Plasma::FrameSvg::marginSize | ( | const Plasma::MarginEdge | edge | ) | const |
Returns the margin size given the margin edge we want.
- Parameters
-
edge the margin edge we want, top, bottom, left or right
- Returns
- the margin size
Definition at line 388 of file framesvg.cpp.
QRegion Plasma::FrameSvg::mask | ( | ) | const |
Returns a mask that tightly contains the fully opaque areas of the svg.
- Returns
- a region of opaque areas
Definition at line 450 of file framesvg.cpp.
void Plasma::FrameSvg::paintFrame | ( | QPainter * | painter, |
const QRectF & | target, | ||
const QRectF & | source = QRectF() |
||
) |
Paints the loaded SVG with the elements that represents the border.
- Parameters
-
painter the QPainter to use target the target rectangle on the paint device source the portion rectangle of the source image
Definition at line 512 of file framesvg.cpp.
void Plasma::FrameSvg::paintFrame | ( | QPainter * | painter, |
const QPointF & | pos = QPointF(0, 0) |
||
) |
Paints the loaded SVG with the elements that represents the border This is an overloaded member provided for convenience.
- Parameters
-
painter the QPainter to use pos where to paint the svg
Definition at line 525 of file framesvg.cpp.
QString Plasma::FrameSvg::prefix | ( | ) |
Returns the prefix for SVG elements of the FrameSvg.
- Returns
- the prefix
Definition at line 308 of file framesvg.cpp.
void Plasma::FrameSvg::resizeFrame | ( | const QSizeF & | size | ) |
Resize the frame maintaining the same border size.
- Parameters
-
size the new size of the frame
Definition at line 317 of file framesvg.cpp.
void Plasma::FrameSvg::setCacheAllRenderedFrames | ( | bool | cache | ) |
Sets whether saving all the rendered prefixes in a cache or not.
- Parameters
-
cache if use the cache or not
Definition at line 464 of file framesvg.cpp.
void Plasma::FrameSvg::setElementPrefix | ( | Plasma::Location | location | ) |
Sets the prefix (.
- See also
- setElementPrefix) to 'north', 'south', 'west' and 'east' when the location is TopEdge, BottomEdge, LeftEdge and RightEdge, respectively. Clears the prefix in other cases.
The prefix must exist in the SVG document, which means that this can only be called successfully after setImagePath is called.
- Parameters
-
location location in the UI this frame will be drawn
Definition at line 184 of file framesvg.cpp.
void Plasma::FrameSvg::setElementPrefix | ( | const QString & | prefix | ) |
Sets the prefix for the SVG elements to be used for painting.
For example, if prefix is 'active', then instead of using the 'top' element of the SVG file to paint the top border, 'active-top' element will be used. The same goes for other SVG elements.
If the elements with prefixes are not present, the default ones are used. (for the sake of speed, the test is present only for the 'center' element)
Setting the prefix manually resets the location to Floating.
The prefix must exist in the SVG document, which means that this can only be called successfully after setImagePath is called.
- Parameters
-
prefix prefix for the SVG elements that make up the frame
Definition at line 207 of file framesvg.cpp.
void Plasma::FrameSvg::setEnabledBorders | ( | const EnabledBorders | borders | ) |
Sets what borders should be painted.
- Parameters
-
flags borders we want to paint
Definition at line 119 of file framesvg.cpp.
void Plasma::FrameSvg::setImagePath | ( | const QString & | path | ) |
Friends And Related Function Documentation
|
related |
Constructs a new FrameSvg that paints the proper named subelements as borders.
It may also be used as a regular Plasma::Svg object for direct access to elements in the Svg.
- Parameters
-
parent options QObject to parent this to
Definition at line 49 of file framesvg.cpp.
Property Documentation
|
readwrite |
Definition at line 81 of file framesvg.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:48:35 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.