Plasma
#include <Plasma/Svg>
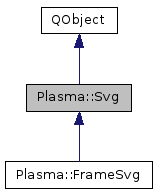
Signals | |
void | repaintNeeded () |
void | sizeChanged () |
Public Member Functions | |
~Svg () | |
bool | containsMultipleImages () const |
Q_INVOKABLE QString | elementAtPoint (const QPoint &point) const |
Q_INVOKABLE QRectF | elementRect (const QString &elementId) const |
Q_INVOKABLE QSize | elementSize (const QString &elementId) const |
Q_INVOKABLE bool | hasElement (const QString &elementId) const |
QString | imagePath () const |
bool | isUsingRenderingCache () const |
Q_INVOKABLE bool | isValid () const |
Q_INVOKABLE void | paint (QPainter *painter, const QPointF &point, const QString &elementID=QString()) |
Q_INVOKABLE void | paint (QPainter *painter, int x, int y, const QString &elementID=QString()) |
Q_INVOKABLE void | paint (QPainter *painter, const QRectF &rect, const QString &elementID=QString()) |
Q_INVOKABLE void | paint (QPainter *painter, int x, int y, int width, int height, const QString &elementID=QString()) |
Q_INVOKABLE QPixmap | pixmap (const QString &elementID=QString()) |
Q_INVOKABLE void | resize (qreal width, qreal height) |
Q_INVOKABLE void | resize (const QSizeF &size) |
Q_INVOKABLE void | resize () |
void | setContainsMultipleImages (bool multiple) |
void | setImagePath (const QString &svgFilePath) |
void | setTheme (Plasma::Theme *theme) |
void | setUsingRenderingCache (bool useCache) |
QSize | size () const |
Theme * | theme () const |
Properties | |
QString | imagePath |
bool | multipleImages |
QSize | size |
bool | usingRenderingCache |
Related Functions | |
(Note that these are not member functions.) | |
Svg (QObject *parent=0) | |
Detailed Description
A theme aware image-centric SVG class.
Plasma::Svg provides a class for rendering SVG images to a QPainter in a convenient manner. Unless an absolute path to a file is provided, it loads the SVG document using Plasma::Theme. It also provides a number of internal optimizations to help lower the cost of painting SVGs, such as caching.
- See also
- Plasma::FrameSvg
Constructor & Destructor Documentation
Member Function Documentation
bool Plasma::Svg::containsMultipleImages | ( | ) | const |
Whether the SVG contains multiple images.
If this is true
, the SVG will be treated as a collection of related images, rather than a consistent drawing.
- Returns
true
if the SVG will be treated as containing multiple images,false
if it will be treated as a coherent image.
QString Plasma::Svg::elementAtPoint | ( | const QPoint & | point | ) | const |
QRectF Plasma::Svg::elementRect | ( | const QString & | elementId | ) | const |
The bounding rect of a given element.
This is the bounding rect of the element with ID elementId
after the SVG has been scaled (see resize()). Note that this is unaffected by the containsMultipleImages property.
- Parameters
-
elementId the id of the element to check
- Returns
- the current rect of a given element, given the current size of the SVG
QSize Plasma::Svg::elementSize | ( | const QString & | elementId | ) | const |
Find the size of a given element.
This is the size of the element with ID elementId
after the SVG has been scaled (see resize()). Note that this is unaffected by the containsMultipleImages property.
- Parameters
-
elementId the id of the element to check
- Returns
- the size of a given element, given the current size of the SVG
bool Plasma::Svg::hasElement | ( | const QString & | elementId | ) | const |
QString Plasma::Svg::imagePath | ( | ) | const |
The SVG file to render.
If this SVG is themed, this will be a relative path, and will not include a file extension.
- Returns
- either an absolute path to an SVG file, or an image name
- See also
- Theme::imagePath()
bool Plasma::Svg::isUsingRenderingCache | ( | ) | const |
bool Plasma::Svg::isValid | ( | ) | const |
void Plasma::Svg::paint | ( | QPainter * | painter, |
const QPointF & | point, | ||
const QString & | elementID = QString() |
||
) |
Paints all or part of the SVG represented by this object.
The size of the painted area will be the size of this Svg object (size()) if containsMultipleImages is true
; otherwise, it will be the size of the requested element after the whole SVG has been scaled to size().
- Parameters
-
painter the QPainter to use point the position to start drawing; the entire svg will be drawn starting at this point. elementId the ID string of the element to render, or an empty string for the whole SVG (the default)
void Plasma::Svg::paint | ( | QPainter * | painter, |
int | x, | ||
int | y, | ||
const QString & | elementID = QString() |
||
) |
Paints all or part of the SVG represented by this object.
The size of the painted area will be the size of this Svg object (size()) if containsMultipleImages is true
; otherwise, it will be the size of the requested element after the whole SVG has been scaled to size().
- Parameters
-
painter the QPainter to use x the horizontal coordinate to start painting from y the vertical coordinate to start painting from elementId the ID string of the element to render, or an empty string for the whole SVG (the default)
void Plasma::Svg::paint | ( | QPainter * | painter, |
const QRectF & | rect, | ||
const QString & | elementID = QString() |
||
) |
Paints all or part of the SVG represented by this object.
- Parameters
-
painter the QPainter to use rect the rect to draw into; if smaller than the current size the drawing is starting at this point. elementId the ID string of the element to render, or an empty string for the whole SVG (the default)
void Plasma::Svg::paint | ( | QPainter * | painter, |
int | x, | ||
int | y, | ||
int | width, | ||
int | height, | ||
const QString & | elementID = QString() |
||
) |
Paints all or part of the SVG represented by this object.
- Parameters
-
painter the QPainter to use x the horizontal coordinate to start painting from y the vertical coordinate to start painting from width the width of the element to draw height the height of the element do draw elementId the ID string of the element to render, or an empty string for the whole SVG (the default)
QPixmap Plasma::Svg::pixmap | ( | const QString & | elementID = QString() | ) |
Returns a pixmap of the SVG represented by this object.
The size of the pixmap will be the size of this Svg object (size()) if containsMultipleImages is true
; otherwise, it will be the size of the requested element after the whole SVG has been scaled to size().
- Parameters
-
elementId the ID string of the element to render, or an empty string for the whole SVG (the default)
- Returns
- a QPixmap of the rendered SVG
|
signal |
Emitted whenever the SVG data has changed in such a way that a repaint is required.
Any usage of an Svg object that does the painting itself must connect to this signal and respond by updating the painting. Note that connected to Theme::themeChanged is incorrect in such a use case as the Svg itself may not be updated yet nor may theme change be the only case when a repaint is needed. Also note that classes or QML code which take Svg objects as parameters for their own painting all respond to this signal so that in those cases manually responding to the signal is unnecessary; ONLY when direct, manual painting with an Svg object is done in application code is this signal used.
void Plasma::Svg::resize | ( | qreal | width, |
qreal | height | ||
) |
Resizes the rendered image.
Rendering will actually take place on the next call to paint.
If containsMultipleImages is true
, each element of the SVG will be rendered at this size by default; otherwise, the entire image will be scaled to this size and each element will be scaled appropriately.
- Parameters
-
width the new width height the new height
void Plasma::Svg::resize | ( | const QSizeF & | size | ) |
Resizes the rendered image.
Rendering will actually take place on the next call to paint.
If containsMultipleImages is true
, each element of the SVG will be rendered at this size by default; otherwise, the entire image will be scaled to this size and each element will be scaled appropriately.
- Parameters
-
size the new size of the image
void Plasma::Svg::resize | ( | ) |
void Plasma::Svg::setContainsMultipleImages | ( | bool | multiple | ) |
Set whether the SVG contains a single image or multiple ones.
If this is set to true
, the SVG will be treated as a collection of related images, rather than a consistent drawing.
In particular, when individual elements are rendered, this affects whether the elements are resized to size() by default. See paint() and pixmap().
- Parameters
-
multiple true if the svg contains multiple images
void Plasma::Svg::setImagePath | ( | const QString & | svgFilePath | ) |
Set the SVG file to render.
Relative paths are looked for in the current Plasma theme, and should not include the file extension (.svg and .svgz files will be searched for). See Theme::imagePath().
If the parent object of this Svg is a Plasma::Applet, relative paths will be searched for in the applet's package first.
- Parameters
-
svgFilePath either an absolute path to an SVG file, or an image name
void Plasma::Svg::setTheme | ( | Plasma::Theme * | theme | ) |
Sets the Plasma::Theme to use with this Svg object.
By default, Svg objects use Plasma::Theme::default().
This determines how relative image paths are interpreted.
- Parameters
-
theme the theme object to use
- Since
- 4.3
void Plasma::Svg::setUsingRenderingCache | ( | bool | useCache | ) |
Sets whether or not to cache the results of rendering to pixmaps.
If the SVG is resized and re-rendered often (and does not keep using the same small set of pixmap dimensions), then it may be less efficient to do disk caching. A good example might be a progress meter that uses an Svg object to paint itself: the meter will be changing often enough, with enough unpredictability and without re-use of the previous pixmaps to not get a gain from caching.
Most Svg objects should use the caching feature, however. Therefore, the default is to use the render cache.
- Parameters
-
useCache true to cache rendered pixmaps
- Since
- 4.3
QSize Plasma::Svg::size | ( | ) | const |
The size of the SVG.
If the SVG has been resized with resize(), that size will be returned; otherwise, the natural size of the SVG will be returned.
If containsMultipleImages is true
, each element of the SVG will be rendered at this size by default.
- Returns
- the current size of the SVG
|
signal |
Theme * Plasma::Svg::theme | ( | ) | const |
The Plasma::Theme used by this Svg object.
This determines how relative image paths are interpreted.
- Returns
- the theme used by this Svg
Friends And Related Function Documentation
|
related |
Constructs an SVG object that implicitly shares and caches rendering.
Unlike QSvgRenderer, which this class uses internally, Plasma::Svg represents an image generated from an SVG. As such, it has a related size and transform matrix (the latter being provided by the painter used to paint the image).
The size is initialized to be the SVG's native size.
- Parameters
-
parent options QObject to parent this to
Property Documentation
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 22:48:35 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.